Exploring Functional Programming Algorithms: A Comprehensive Guide
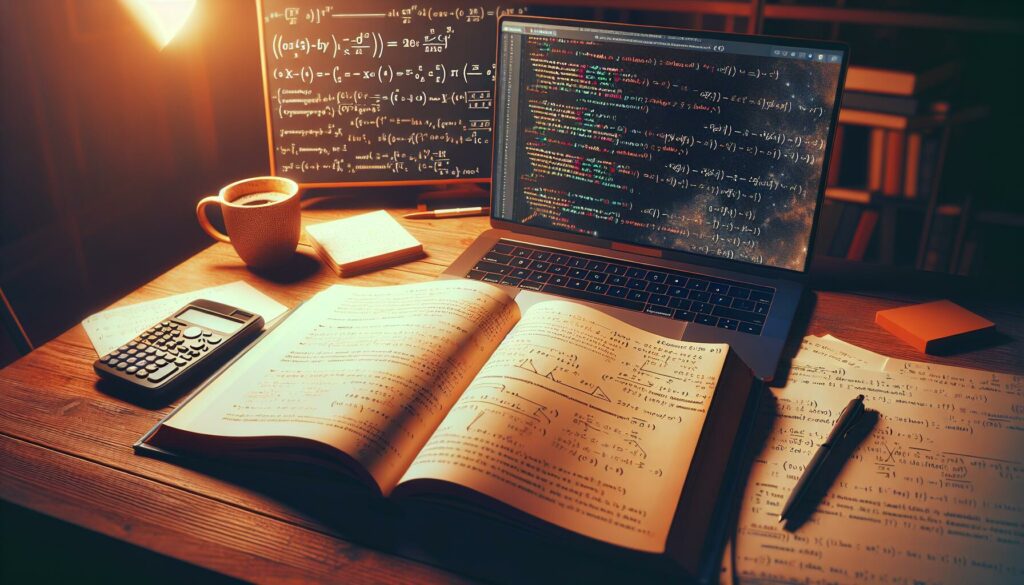
In the ever-evolving landscape of software development, functional programming has gained significant traction due to its emphasis on immutability, pure functions, and declarative code. As we delve into the world of functional programming algorithms, we’ll explore how these concepts can lead to more robust, maintainable, and efficient code. This comprehensive guide will take you through the fundamental principles of functional programming and demonstrate how they can be applied to solve common algorithmic problems.
Table of Contents
- Introduction to Functional Programming
- Core Concepts of Functional Programming
- Recursion in Functional Programming
- Higher-Order Functions and Their Applications
- Map, Filter, and Reduce: The Holy Trinity
- Lazy Evaluation and Infinite Sequences
- Immutability and Persistent Data Structures
- Pattern Matching in Algorithms
- Monads and Their Role in Functional Programming
- Real-World Examples of Functional Algorithms
- Conclusion and Future Trends
1. Introduction to Functional Programming
Functional programming is a paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the application of functions to inputs to produce outputs without modifying state. This approach can lead to code that is easier to understand, test, and debug.
Key characteristics of functional programming include:
- First-class and higher-order functions
- Pure functions with no side effects
- Immutability
- Declarative rather than imperative style
- Recursion instead of looping
Languages that support functional programming include Haskell, Scala, F#, Clojure, and even JavaScript to some extent. As we explore functional programming algorithms, we’ll use a mix of these languages to illustrate concepts, with a focus on JavaScript for its widespread use and accessibility.
2. Core Concepts of Functional Programming
Before diving into specific algorithms, it’s crucial to understand the core concepts that make functional programming unique and powerful.
Pure Functions
Pure functions are the building blocks of functional programming. A function is considered pure if:
- It always returns the same output for the same input
- It has no side effects (doesn’t modify external state)
Here’s an example of a pure function in JavaScript:
const add = (a, b) => a + b;
console.log(add(2, 3)); // Always returns 5
console.log(add(2, 3)); // Always returns 5, no matter how many times it's called
Immutability
Immutability is the practice of not changing data once it’s created. Instead of modifying existing data structures, functional programming creates new ones with the desired changes. This leads to more predictable code and easier tracking of state changes.
Example of immutability in JavaScript:
const originalArray = [1, 2, 3];
const newArray = [...originalArray, 4]; // Creates a new array instead of modifying the original
console.log(originalArray); // [1, 2, 3]
console.log(newArray); // [1, 2, 3, 4]
Function Composition
Function composition is the process of combining two or more functions to produce a new function. This allows for building complex operations from simpler ones.
const compose = (f, g) => x => f(g(x));
const addOne = x => x + 1;
const double = x => x * 2;
const addOneThenDouble = compose(double, addOne);
console.log(addOneThenDouble(3)); // (3 + 1) * 2 = 8
3. Recursion in Functional Programming
Recursion is a fundamental concept in functional programming, often used in place of imperative loops. It involves a function calling itself until it reaches a base case.
Let’s implement a factorial function using recursion:
const factorial = n => {
if (n === 0 || n === 1) {
return 1;
}
return n * factorial(n - 1);
};
console.log(factorial(5)); // 120
While this is a simple example, recursion can be used to solve complex problems elegantly. However, it’s important to be aware of the potential for stack overflow errors with deep recursion. Many functional languages optimize for tail recursion to mitigate this issue.
Tail Recursion
Tail recursion is a special case of recursion where the recursive call is the last operation in the function. This allows for optimization by the compiler or interpreter, effectively turning the recursion into iteration.
Here’s the factorial function rewritten with tail recursion:
const factorialTail = (n, acc = 1) => {
if (n === 0 || n === 1) {
return acc;
}
return factorialTail(n - 1, n * acc);
};
console.log(factorialTail(5)); // 120
4. Higher-Order Functions and Their Applications
Higher-order functions are functions that can take other functions as arguments or return functions as results. They are a powerful tool in functional programming, enabling the creation of more abstract and reusable code.
Function as Argument
A common use of higher-order functions is to pass behavior as an argument. Here’s an example of a function that applies a given operation to an array of numbers:
const applyOperation = (numbers, operation) => {
return numbers.map(operation);
};
const double = x => x * 2;
const square = x => x * x;
const numbers = [1, 2, 3, 4, 5];
console.log(applyOperation(numbers, double)); // [2, 4, 6, 8, 10]
console.log(applyOperation(numbers, square)); // [1, 4, 9, 16, 25]
Returning a Function
Higher-order functions can also return new functions, allowing for powerful abstractions:
const multiply = x => y => x * y;
const double = multiply(2);
const triple = multiply(3);
console.log(double(5)); // 10
console.log(triple(5)); // 15
5. Map, Filter, and Reduce: The Holy Trinity
Map, filter, and reduce are three higher-order functions that form the backbone of functional programming. They provide a declarative way to transform data without mutating the original source.
Map
Map applies a function to each element of an array, creating a new array with the results.
const numbers = [1, 2, 3, 4, 5];
const squared = numbers.map(x => x * x);
console.log(squared); // [1, 4, 9, 16, 25]
Filter
Filter creates a new array with all elements that pass a certain test implemented by the provided function.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evens = numbers.filter(x => x % 2 === 0);
console.log(evens); // [2, 4, 6, 8, 10]
Reduce
Reduce applies a function against an accumulator and each element in the array to reduce it to a single value.
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((acc, curr) => acc + curr, 0);
console.log(sum); // 15
These functions can be combined to create powerful data processing pipelines:
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const sumOfSquaresOfEvens = numbers
.filter(x => x % 2 === 0)
.map(x => x * x)
.reduce((acc, curr) => acc + curr, 0);
console.log(sumOfSquaresOfEvens); // 220
6. Lazy Evaluation and Infinite Sequences
Lazy evaluation is a strategy that delays the evaluation of an expression until its value is needed. This can lead to performance improvements and allows for working with infinite sequences.
While JavaScript doesn’t natively support lazy evaluation, we can simulate it using generator functions:
function* fibonacci() {
let a = 0, b = 1;
while (true) {
yield a;
[a, b] = [b, a + b];
}
}
const fib = fibonacci();
console.log(fib.next().value); // 0
console.log(fib.next().value); // 1
console.log(fib.next().value); // 1
console.log(fib.next().value); // 2
console.log(fib.next().value); // 3
This generator function creates an infinite sequence of Fibonacci numbers, but only calculates them as they are requested.
7. Immutability and Persistent Data Structures
Immutability is a core principle of functional programming. Persistent data structures are a way to achieve immutability efficiently by sharing structure between versions of data.
While JavaScript doesn’t have built-in persistent data structures, libraries like Immutable.js provide them. Here’s an example using Immutable.js:
const { List } = require('immutable');
const list1 = List([1, 2, 3]);
const list2 = list1.push(4);
console.log(list1.toArray()); // [1, 2, 3]
console.log(list2.toArray()); // [1, 2, 3, 4]
In this example, list2
is a new list that shares most of its structure with list1
, but has an additional element. The original list1
remains unchanged.
8. Pattern Matching in Algorithms
Pattern matching is a powerful feature in many functional programming languages that allows for concise and expressive code. While JavaScript doesn’t have native pattern matching, we can simulate it to some extent:
const match = (value, patterns) => {
for (let [pattern, result] of patterns) {
if (typeof pattern === 'function' && pattern(value)) {
return typeof result === 'function' ? result(value) : result;
}
if (pattern === value) {
return result;
}
}
throw new Error('No matching pattern');
};
const factorial = n => match(n, [
[0, 1],
[1, 1],
[x => x > 1, x => x * factorial(x - 1)]
]);
console.log(factorial(5)); // 120
This implementation of factorial uses a simple pattern matching function to handle different cases, making the code more declarative and easier to read.
9. Monads and Their Role in Functional Programming
Monads are a concept from category theory that has found practical applications in functional programming. They provide a way to structure computations in terms of values and sequences of operations. While the full theory of monads can be complex, we can understand their practical use through examples.
One common monad is the Maybe monad, which represents computations that might not produce a value. Here’s a simple implementation in JavaScript:
class Maybe {
constructor(value) {
this._value = value;
}
static of(value) {
return new Maybe(value);
}
isNothing() {
return this._value === null || this._value === undefined;
}
map(fn) {
return this.isNothing() ? Maybe.of(null) : Maybe.of(fn(this._value));
}
flatMap(fn) {
return this.isNothing() ? Maybe.of(null) : fn(this._value);
}
getOrElse(defaultValue) {
return this.isNothing() ? defaultValue : this._value;
}
}
// Usage
const safeDivide = (a, b) => b === 0 ? Maybe.of(null) : Maybe.of(a / b);
const result = Maybe.of(10)
.flatMap(x => safeDivide(x, 2))
.map(x => x + 5)
.getOrElse('Error');
console.log(result); // 10
In this example, the Maybe monad allows us to chain operations that might fail (like division by zero) without explicitly checking for errors at each step.
10. Real-World Examples of Functional Algorithms
Let’s explore some real-world applications of functional programming concepts in solving algorithmic problems.
Merge Sort
Here’s an implementation of the merge sort algorithm using functional programming principles:
const merge = (left, right) => {
const result = [];
let leftIndex = 0;
let rightIndex = 0;
while (leftIndex < left.length && rightIndex < right.length) {
if (left[leftIndex] < right[rightIndex]) {
result.push(left[leftIndex]);
leftIndex++;
} else {
result.push(right[rightIndex]);
rightIndex++;
}
}
return result.concat(left.slice(leftIndex)).concat(right.slice(rightIndex));
};
const mergeSort = arr => {
if (arr.length <= 1) return arr;
const middle = Math.floor(arr.length / 2);
const left = arr.slice(0, middle);
const right = arr.slice(middle);
return merge(mergeSort(left), mergeSort(right));
};
console.log(mergeSort([64, 34, 25, 12, 22, 11, 90])); // [11, 12, 22, 25, 34, 64, 90]
This implementation uses recursion and immutability, creating new arrays at each step instead of modifying existing ones.
Functional Graph Traversal
Here’s a depth-first search implementation using functional programming concepts:
const dfs = (graph, start, visited = new Set()) => {
console.log(start);
visited.add(start);
for (let neighbor of graph[start]) {
if (!visited.has(neighbor)) {
dfs(graph, neighbor, visited);
}
}
};
const graph = {
A: ['B', 'C'],
B: ['A', 'D', 'E'],
C: ['A', 'F'],
D: ['B'],
E: ['B', 'F'],
F: ['C', 'E']
};
dfs(graph, 'A');
This implementation uses recursion and treats the graph as an immutable data structure.
11. Conclusion and Future Trends
Functional programming offers a powerful paradigm for solving algorithmic problems. Its emphasis on immutability, pure functions, and declarative code can lead to more robust, maintainable, and often more efficient solutions. As we’ve seen, concepts like recursion, higher-order functions, and monads provide elegant ways to express complex algorithms.
Looking to the future, we can expect functional programming concepts to become even more prevalent in mainstream development. Many popular languages are incorporating functional features, and the rise of parallel and distributed computing makes functional programming’s emphasis on immutability and side-effect-free code increasingly valuable.
As you continue your journey in algorithm development, consider how functional programming principles can be applied to create cleaner, more robust solutions. Whether you’re working on data processing pipelines, implementing complex algorithms, or building reactive systems, the concepts we’ve explored in this guide will serve you well.
Remember, the goal is not to use functional programming exclusively, but to add it to your toolkit of problem-solving approaches. By understanding when and how to apply functional concepts, you’ll be better equipped to choose the right tool for each programming challenge you face.
Happy coding, and may your functions always be pure and your data immutable!