Exploring Edge AI: Implementing Artificial Intelligence Directly on Edge Devices
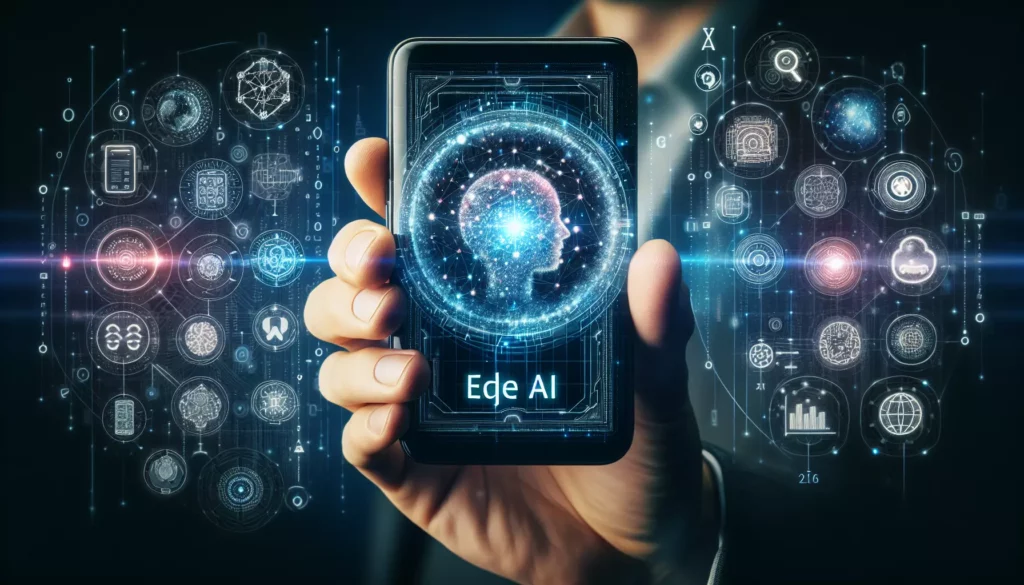
In the rapidly evolving landscape of technology, Edge AI has emerged as a game-changing paradigm that brings the power of artificial intelligence directly to edge devices. This innovative approach to AI implementation is revolutionizing how we process data, make decisions, and interact with smart devices in our daily lives. In this comprehensive guide, we’ll dive deep into the world of Edge AI, exploring its concepts, applications, benefits, and implementation strategies.
What is Edge AI?
Edge AI, short for Edge Artificial Intelligence, refers to the deployment and execution of AI algorithms directly on edge devices, rather than in centralized cloud servers. Edge devices are hardware units located at the “edge” of a network, closer to the source of data generation. These can include smartphones, IoT devices, sensors, cameras, and other smart gadgets.
The core idea behind Edge AI is to process data and make decisions locally on these devices, minimizing the need to send data back to centralized servers for analysis. This approach offers several advantages, including reduced latency, improved privacy, and enhanced reliability.
The Evolution from Cloud AI to Edge AI
To understand the significance of Edge AI, it’s essential to trace its evolution from traditional cloud-based AI systems:
- Cloud AI: Initially, AI models were primarily deployed on powerful cloud servers. Devices would send data to these servers for processing and wait for results.
- Hybrid AI: As edge devices became more powerful, a hybrid approach emerged, where some processing occurred on the device, and more complex tasks were offloaded to the cloud.
- Edge AI: The latest evolution involves running sophisticated AI models directly on edge devices, enabling real-time processing and decision-making without relying on cloud connectivity.
Key Benefits of Edge AI
The shift towards Edge AI brings several significant advantages:
1. Reduced Latency
By processing data locally, Edge AI dramatically reduces the time between data collection and decision-making. This near-instantaneous response is crucial for applications requiring real-time actions, such as autonomous vehicles or industrial robotics.
2. Enhanced Privacy and Security
Since data is processed on the device itself, sensitive information doesn’t need to be transmitted over networks, reducing the risk of data breaches and enhancing overall privacy.
3. Improved Reliability
Edge AI systems can continue to function even when network connectivity is poor or unavailable, ensuring consistent performance in various environments.
4. Bandwidth Efficiency
By processing data locally, Edge AI reduces the amount of data that needs to be transmitted to central servers, leading to significant bandwidth savings.
5. Cost-Effectiveness
While initial hardware costs may be higher, Edge AI can lead to long-term cost savings by reducing cloud computing and data transmission expenses.
Applications of Edge AI
The versatility of Edge AI has led to its adoption across various industries and use cases:
1. Autonomous Vehicles
Edge AI enables real-time decision-making for self-driving cars, processing sensor data and making split-second driving choices without relying on cloud connectivity.
2. Smart Home Devices
Voice assistants and smart home gadgets use Edge AI to respond to commands quickly and operate even when internet connectivity is limited.
3. Industrial IoT
In manufacturing and industrial settings, Edge AI powers predictive maintenance, quality control, and real-time monitoring of equipment.
4. Healthcare
Wearable devices and medical equipment leverage Edge AI for real-time health monitoring and early detection of medical conditions.
5. Retail
Edge AI enables smart inventory management, personalized shopping experiences, and automated checkout systems in retail environments.
6. Security and Surveillance
AI-powered cameras and security systems use Edge AI for real-time threat detection and analysis.
Implementing Edge AI: Key Considerations
While the benefits of Edge AI are compelling, implementing it effectively requires careful planning and consideration of several factors:
1. Hardware Selection
Choosing the right hardware is crucial for Edge AI implementation. Factors to consider include:
- Processing power
- Energy efficiency
- Memory capacity
- Thermal management
- Form factor
Popular hardware options for Edge AI include:
- NVIDIA Jetson series
- Intel Neural Compute Stick
- Google Coral TPU
- Raspberry Pi with AI accelerators
2. Model Optimization
AI models designed for cloud deployment often need to be optimized for edge devices. Techniques for model optimization include:
- Quantization: Reducing the precision of model weights to decrease memory footprint and computational requirements.
- Pruning: Removing unnecessary connections in neural networks to reduce model size.
- Knowledge distillation: Creating smaller, more efficient models that mimic the behavior of larger, more complex ones.
3. Software Frameworks
Several frameworks and tools are available for developing and deploying Edge AI applications:
- TensorFlow Lite: A lightweight version of TensorFlow designed for mobile and embedded devices.
- ONNX Runtime: An open-source inference engine for deploying AI models across different hardware platforms.
- Edge Impulse: A development platform for creating and deploying machine learning models on edge devices.
- Apache TVM: An open-source machine learning compiler framework for CPUs, GPUs, and accelerators.
4. Power Management
Edge devices often have limited power resources, making energy efficiency a critical consideration. Strategies for power management include:
- Using low-power modes when AI processing is not required
- Implementing efficient scheduling algorithms for AI tasks
- Leveraging hardware-specific power optimization features
5. Security Considerations
While Edge AI enhances data privacy, it also introduces new security challenges. Key security considerations include:
- Implementing robust authentication and encryption mechanisms
- Ensuring secure firmware updates
- Protecting AI models from tampering or reverse engineering
- Implementing secure boot processes
Implementing Edge AI: A Step-by-Step Guide
Now that we’ve covered the key considerations, let’s walk through a step-by-step process for implementing Edge AI:
Step 1: Define Your Use Case
Clearly outline the problem you’re trying to solve and the specific requirements of your Edge AI application. Consider factors such as:
- Required response time
- Type of data being processed (images, audio, sensor data, etc.)
- Environmental constraints (power availability, network connectivity, etc.)
- Scalability requirements
Step 2: Choose Your Hardware
Based on your use case requirements, select the appropriate edge device or development board. Consider factors like processing power, energy efficiency, and cost.
Step 3: Develop and Train Your AI Model
Create and train your AI model using a suitable framework like TensorFlow or PyTorch. Start with a larger, more complex model and then optimize it for edge deployment.
Step 4: Optimize Your Model
Apply optimization techniques to make your model suitable for edge deployment:
import tensorflow as tf
# Load your trained model
model = tf.keras.models.load_model('my_model.h5')
# Convert the model to TensorFlow Lite format
converter = tf.lite.TFLiteConverter.from_keras_model(model)
tflite_model = converter.convert()
# Quantize the model (optional)
converter.optimizations = [tf.lite.Optimize.DEFAULT]
quantized_model = converter.convert()
# Save the optimized model
with open('edge_model.tflite', 'wb') as f:
f.write(quantized_model)
Step 5: Deploy the Model to Your Edge Device
Transfer the optimized model to your edge device and set up the necessary runtime environment.
Step 6: Implement Inference Logic
Develop the code to perform inference on the edge device. Here’s a simple example using TensorFlow Lite:
import tflite_runtime.interpreter as tflite
# Load the TFLite model
interpreter = tflite.Interpreter(model_path="edge_model.tflite")
interpreter.allocate_tensors()
# Get input and output tensors
input_details = interpreter.get_input_details()
output_details = interpreter.get_output_details()
# Prepare input data
input_data = prepare_input_data() # Your function to prepare input
# Set the input tensor
interpreter.set_tensor(input_details[0]['index'], input_data)
# Run inference
interpreter.invoke()
# Get the output tensor
output_data = interpreter.get_tensor(output_details[0]['index'])
# Process the output
process_output(output_data) # Your function to handle the output
Step 7: Optimize Performance
Fine-tune your implementation for optimal performance on the edge device. This may involve:
- Adjusting batch sizes
- Implementing efficient data preprocessing
- Utilizing hardware-specific optimizations
Step 8: Implement Power Management
Develop strategies to manage power consumption, such as implementing sleep modes or dynamic frequency scaling.
Step 9: Ensure Security
Implement security measures to protect your Edge AI system, including secure boot, encrypted storage, and secure communication protocols.
Step 10: Test and Validate
Thoroughly test your Edge AI implementation in real-world conditions, considering factors like accuracy, latency, and reliability.
Challenges and Future Trends in Edge AI
While Edge AI offers numerous benefits, it also presents several challenges:
Challenges:
- Limited computational resources compared to cloud infrastructure
- Balancing model accuracy with efficiency
- Ensuring consistency across diverse edge hardware
- Managing and updating deployed models at scale
- Addressing privacy concerns in data collection and model training
Future Trends:
As Edge AI continues to evolve, several exciting trends are emerging:
- Federated Learning: Enabling collaborative model training across edge devices without centralizing data.
- Neuromorphic Computing: Developing AI hardware that mimics the structure and function of biological neural networks.
- Edge-Cloud Collaboration: Creating more sophisticated hybrid systems that leverage both edge and cloud resources optimally.
- AI-Specific Hardware: Developing more powerful and efficient AI accelerators tailored for edge deployment.
- Explainable AI on the Edge: Implementing techniques to make Edge AI decisions more transparent and interpretable.
Conclusion
Edge AI represents a significant leap forward in the field of artificial intelligence, bringing powerful computational capabilities directly to the devices we use every day. By processing data locally, Edge AI enables faster, more private, and more reliable AI-driven experiences across a wide range of applications.
As we’ve explored in this guide, implementing Edge AI requires careful consideration of hardware, software, and optimization techniques. However, with the right approach, developers can harness the power of Edge AI to create innovative solutions that push the boundaries of what’s possible in fields like IoT, autonomous systems, and smart devices.
The future of Edge AI is bright, with ongoing advancements in hardware, software frameworks, and AI algorithms continually expanding its capabilities. As edge devices become more powerful and AI models more efficient, we can expect to see even more sophisticated and impactful Edge AI applications in the years to come.
By staying informed about the latest developments in Edge AI and mastering the techniques for its implementation, developers and organizations can position themselves at the forefront of this exciting technological frontier, ready to create the next generation of intelligent, responsive, and efficient AI-powered systems.