Exploring Digital Signal Processing (DSP): From Basics to Advanced Applications
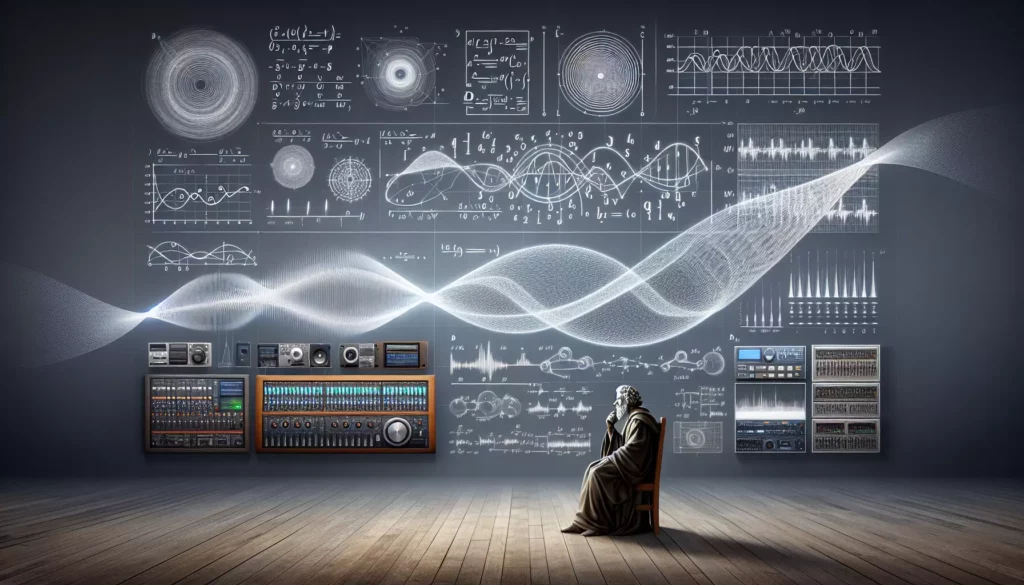
In today’s digital age, the processing and manipulation of signals play a crucial role in various technological advancements. Digital Signal Processing (DSP) is a fundamental concept that underlies many of the technologies we use daily, from smartphones to medical imaging devices. This comprehensive guide will take you through the basics of DSP, its applications, and how it relates to coding and algorithm development.
What is Digital Signal Processing?
Digital Signal Processing (DSP) is the use of digital computing to process signals that have been digitized. These signals can represent various physical phenomena, such as sound, images, temperature, or pressure. DSP techniques are used to improve, modify, or analyze these signals for a wide range of purposes.
The core idea behind DSP is to take an analog signal, convert it to a digital format, process it using digital techniques, and then convert it back to an analog form if necessary. This process allows for more precise and flexible manipulation of signals compared to analog processing methods.
Key Concepts in DSP
1. Sampling and Quantization
The first step in DSP is converting an analog signal to a digital one. This process involves two main steps:
- Sampling: Taking measurements of the analog signal at regular intervals.
- Quantization: Assigning discrete numerical values to the sampled measurements.
The Nyquist-Shannon sampling theorem states that to accurately reconstruct a signal, the sampling rate must be at least twice the highest frequency component of the signal.
2. Fourier Transform
The Fourier Transform is a fundamental tool in DSP. It allows us to convert a signal from the time domain to the frequency domain and vice versa. This transformation provides insights into the frequency components of a signal, which is crucial for many DSP applications.
In DSP, we often use the Discrete Fourier Transform (DFT) and its efficient implementation, the Fast Fourier Transform (FFT).
3. Filters
Filters are essential components in DSP systems. They are used to remove unwanted components or features from a signal. Common types of filters include:
- Low-pass filters
- High-pass filters
- Band-pass filters
- Notch filters
These filters can be implemented using various techniques, such as Finite Impulse Response (FIR) and Infinite Impulse Response (IIR) filters.
Applications of Digital Signal Processing
DSP has a wide range of applications across various fields. Let’s explore some of the most common and innovative uses of DSP:
1. Audio Processing
DSP is extensively used in audio applications, including:
- Noise reduction in recordings
- Audio compression (e.g., MP3 format)
- Voice recognition systems
- Sound effect generation
- Equalizers and audio enhancers
2. Image and Video Processing
In the realm of visual media, DSP techniques are crucial for:
- Image enhancement and restoration
- Video compression (e.g., MPEG formats)
- Face recognition systems
- Medical imaging (CT scans, MRI)
- Computer vision applications
3. Communications
DSP plays a vital role in modern communication systems:
- Modulation and demodulation in digital communications
- Error detection and correction in data transmission
- Echo cancellation in telephones
- Spread spectrum techniques for secure communications
- Software-defined radio
4. Biomedical Applications
In the medical field, DSP contributes to:
- ECG signal analysis
- Brain-computer interfaces
- Hearing aids and cochlear implants
- Analysis of EEG signals
- Medical image processing
5. Control Systems
DSP techniques are used in various control systems:
- Automotive control systems
- Industrial process control
- Robotics and automation
- Aerospace control systems
DSP and Coding: Implementing DSP Algorithms
For programmers and aspiring developers, understanding DSP opens up a world of exciting possibilities. Implementing DSP algorithms requires strong coding skills and a good grasp of mathematical concepts. Let’s look at some common DSP algorithms and how they can be implemented in code.
1. Implementing a Simple Moving Average Filter
A moving average filter is one of the simplest DSP algorithms. It’s used to smooth out short-term fluctuations and highlight longer-term trends. Here’s a simple implementation in Python:
def moving_average(data, window_size):
cumsum = numpy.cumsum(numpy.insert(data, 0, 0))
return (cumsum[window_size:] - cumsum[:-window_size]) / float(window_size)
# Example usage
import numpy as np
data = np.random.randn(1000) # Generate some random data
smoothed_data = moving_average(data, 5) # Apply 5-point moving average
2. Implementing the Fast Fourier Transform (FFT)
The FFT is a crucial algorithm in DSP. While most programming languages have built-in FFT functions, understanding the algorithm is important. Here’s a simple recursive implementation of the Cooley-Tukey FFT algorithm in Python:
import cmath
def fft(x):
N = len(x)
if N <= 1: return x
even = fft(x[0::2])
odd = fft(x[1::2])
T= [cmath.exp(-2j*cmath.pi*k/N) * odd[k] for k in range(N//2)]
return [even[k] + T[k] for k in range(N//2)] + \
[even[k] - T[k] for k in range(N//2)]
# Example usage
x = [1.0, 1.0, 1.0, 1.0, 0.0, 0.0, 0.0, 0.0]
X = fft(x)
print([abs(f) for f in X])
3. Implementing a Basic FIR Filter
Finite Impulse Response (FIR) filters are widely used in DSP. Here’s a simple implementation of a low-pass FIR filter:
import numpy as np
def fir_filter(x, h):
"""
Apply FIR filter to x using coefficients h
"""
y = np.zeros(len(x))
for n in range(len(x)):
for k in range(len(h)):
if n-k >= 0:
y[n] += h[k]*x[n-k]
return y
# Example usage
# Generate a simple signal
t = np.linspace(0, 1, 1000, False)
x = np.sin(2*np.pi*10*t) + 0.5*np.sin(2*np.pi*20*t)
# Define filter coefficients (simple low-pass filter)
h = np.ones(5) / 5.0
# Apply filter
y = fir_filter(x, h)
Advanced DSP Concepts and Techniques
As you delve deeper into DSP, you’ll encounter more advanced concepts and techniques. Some of these include:
1. Adaptive Filtering
Adaptive filters can automatically adjust their parameters based on the input signal. They’re used in applications like noise cancellation and system identification.
2. Multirate Signal Processing
This involves changing the sampling rate of a signal, which is useful in applications like audio and video compression.
3. Wavelet Transforms
Wavelet transforms provide time-frequency representation of signals and are particularly useful in image compression and denoising.
4. Statistical Signal Processing
This branch of DSP deals with the statistical analysis of signals and noise, often used in communications and radar systems.
5. Machine Learning in DSP
The integration of machine learning techniques with DSP has led to powerful new approaches in areas like speech recognition and computer vision.
DSP in the Context of Coding Education and Technical Interviews
Understanding DSP can be a valuable asset in your coding education and career, especially if you’re aiming for positions in tech companies that deal with signal processing, audio/video technologies, or communications.
DSP in Technical Interviews
While not all technical interviews will directly test DSP knowledge, understanding these concepts can give you an edge:
- Algorithm Optimization: Many DSP algorithms, like the FFT, are excellent examples of algorithmic optimization. Understanding these can help you approach other optimization problems.
- Time and Space Complexity: DSP algorithms often involve interesting trade-offs between time and space complexity, which is a common theme in technical interviews.
- Real-world Applications: Being able to discuss real-world applications of algorithms (like those in DSP) can demonstrate your ability to connect theoretical concepts with practical uses.
DSP Projects for Portfolio Building
Including DSP projects in your portfolio can showcase your skills in signal processing and algorithm implementation. Some ideas include:
- Building a simple audio equalizer
- Implementing a basic image processing tool (e.g., filters, edge detection)
- Creating a voice activity detection system
- Developing a simple software-defined radio application
Resources for Learning DSP
If you’re interested in diving deeper into DSP, here are some resources to get you started:
- Books:
- “The Scientist and Engineer’s Guide to Digital Signal Processing” by Steven W. Smith (available free online)
- “Understanding Digital Signal Processing” by Richard G. Lyons
- Online Courses:
- Coursera: “Digital Signal Processing” by EPFL
- edX: “Discrete Time Signal Processing” by MIT
- Websites and Tutorials:
- DSPGuide.com
- scipy.org (for Python implementations of DSP algorithms)
Conclusion
Digital Signal Processing is a vast and exciting field that underpins many of the technologies we use every day. From audio and video processing to communications and medical imaging, DSP techniques are everywhere. For programmers and aspiring developers, understanding DSP not only opens up new career opportunities but also provides valuable insights into algorithm design and optimization.
As you continue your coding journey, consider exploring DSP concepts and implementing some of the algorithms we’ve discussed. Not only will this enhance your programming skills, but it will also give you a deeper appreciation for the intricate processing that occurs in many of our digital devices.
Remember, the world of DSP is constantly evolving, with new applications and techniques emerging regularly. Stay curious, keep learning, and you’ll be well-equipped to tackle the exciting challenges in this field. Whether you’re aiming for a career in signal processing or simply want to broaden your coding horizons, DSP offers a rich landscape of possibilities to explore.