Exploring Computer Graphics Programming: A Deep Dive into OpenGL and DirectX
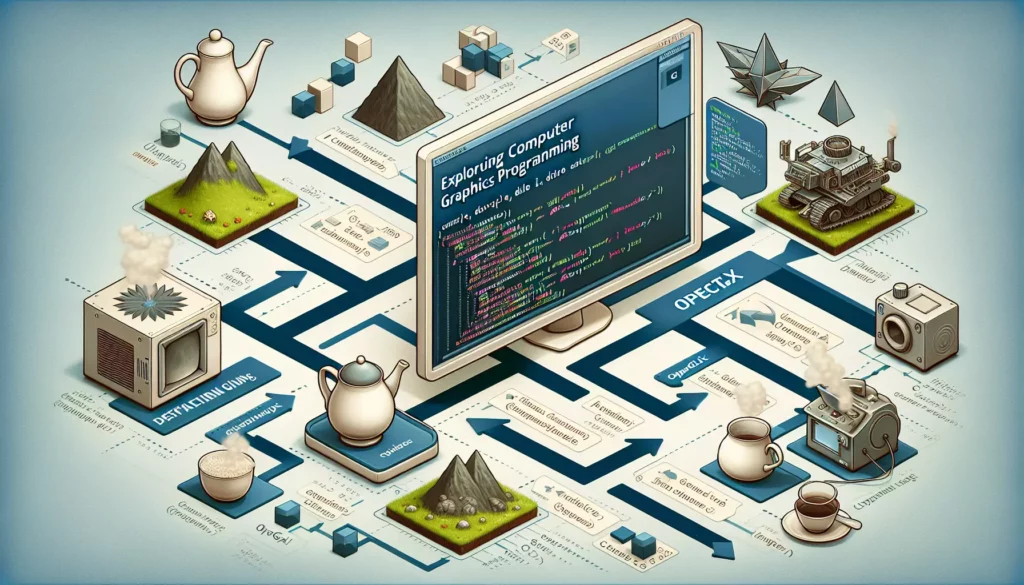
Computer graphics programming is a fascinating field that combines artistry with technical prowess, allowing developers to create stunning visual experiences in both 2D and 3D environments. Whether you’re interested in game development, scientific visualization, or creating immersive user interfaces, understanding graphics programming is an essential skill. In this comprehensive guide, we’ll explore two of the most popular graphics APIs: OpenGL and DirectX. We’ll delve into their features, use cases, and provide practical examples to help you get started on your journey into the world of computer graphics.
Understanding Computer Graphics Programming
Before we dive into the specifics of OpenGL and DirectX, it’s important to understand what computer graphics programming entails. At its core, graphics programming is about creating visual content using computer algorithms and specialized hardware. This can range from simple 2D shapes to complex 3D scenes with realistic lighting, textures, and animations.
Graphics programming involves several key concepts:
- Rendering: The process of generating an image from a model
- Shaders: Programs that determine how 3D scenes are rendered
- Texturing: Applying 2D images to 3D surfaces
- Lighting: Simulating how light interacts with objects in a scene
- Animation: Creating the illusion of movement
- Geometry: Defining the shape and structure of objects
With these concepts in mind, let’s explore the two major graphics APIs: OpenGL and DirectX.
OpenGL: The Open Graphics Library
OpenGL (Open Graphics Library) is a cross-platform, language-independent API for rendering 2D and 3D vector graphics. Developed by Silicon Graphics Inc. in 1992, OpenGL has become one of the most widely used graphics APIs in the industry.
Key Features of OpenGL
- Cross-platform compatibility (Windows, macOS, Linux, mobile devices)
- Support for multiple programming languages (C, C++, Java, Python, etc.)
- Extensive documentation and community support
- Flexible and customizable pipeline
- Hardware-accelerated rendering
Getting Started with OpenGL
To begin working with OpenGL, you’ll need to set up your development environment. Here’s a basic guide to get you started:
- Install a C++ compiler (e.g., GCC, Clang, or Visual Studio)
- Download and install the OpenGL library and its extensions (GLEW, GLFW)
- Set up your project and link the necessary libraries
Here’s a simple example of creating a window and rendering a triangle using OpenGL and GLFW:
#include <GL/glew.h>
#include <GLFW/glfw3.h>
#include <iostream>
const char* vertexShaderSource = R"(
#version 330 core
layout (location = 0) in vec3 aPos;
void main() {
gl_Position = vec4(aPos.x, aPos.y, aPos.z, 1.0);
}
)";
const char* fragmentShaderSource = R"(
#version 330 core
out vec4 FragColor;
void main() {
FragColor = vec4(1.0f, 0.5f, 0.2f, 1.0f);
}
)";
int main() {
// Initialize GLFW
if (!glfwInit()) {
std::cerr << "Failed to initialize GLFW" << std::endl;
return -1;
}
// Create a windowed mode window and its OpenGL context
GLFWwindow* window = glfwCreateWindow(800, 600, "OpenGL Triangle", NULL, NULL);
if (!window) {
std::cerr << "Failed to create GLFW window" << std::endl;
glfwTerminate();
return -1;
}
// Make the window's context current
glfwMakeContextCurrent(window);
// Initialize GLEW
if (glewInit() != GLEW_OK) {
std::cerr << "Failed to initialize GLEW" << std::endl;
return -1;
}
// Compile and link shaders
unsigned int vertexShader = glCreateShader(GL_VERTEX_SHADER);
glShaderSource(vertexShader, 1, &vertexShaderSource, NULL);
glCompileShader(vertexShader);
unsigned int fragmentShader = glCreateShader(GL_FRAGMENT_SHADER);
glShaderSource(fragmentShader, 1, &fragmentShaderSource, NULL);
glCompileShader(fragmentShader);
unsigned int shaderProgram = glCreateProgram();
glAttachShader(shaderProgram, vertexShader);
glAttachShader(shaderProgram, fragmentShader);
glLinkProgram(shaderProgram);
glDeleteShader(vertexShader);
glDeleteShader(fragmentShader);
// Set up vertex data and buffers
float vertices[] = {
-0.5f, -0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
0.0f, 0.5f, 0.0f
};
unsigned int VBO, VAO;
glGenVertexArrays(1, &VAO);
glGenBuffers(1, &VBO);
glBindVertexArray(VAO);
glBindBuffer(GL_ARRAY_BUFFER, VBO);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 3 * sizeof(float), (void*)0);
glEnableVertexAttribArray(0);
// Render loop
while (!glfwWindowShouldClose(window)) {
// Clear the screen
glClearColor(0.2f, 0.3f, 0.3f, 1.0f);
glClear(GL_COLOR_BUFFER_BIT);
// Draw the triangle
glUseProgram(shaderProgram);
glBindVertexArray(VAO);
glDrawArrays(GL_TRIANGLES, 0, 3);
// Swap front and back buffers
glfwSwapBuffers(window);
// Poll for and process events
glfwPollEvents();
}
// Clean up
glDeleteVertexArrays(1, &VAO);
glDeleteBuffers(1, &VBO);
glDeleteProgram(shaderProgram);
glfwTerminate();
return 0;
}
This example demonstrates the basic structure of an OpenGL program, including window creation, shader compilation, and rendering a simple triangle.
Advanced OpenGL Techniques
As you become more comfortable with OpenGL, you can explore more advanced techniques:
- Texture mapping
- Lighting and shading models
- 3D model loading and rendering
- Particle systems
- Post-processing effects
These techniques will allow you to create more complex and visually appealing graphics applications.
DirectX: Microsoft’s Graphics API
DirectX is a collection of APIs developed by Microsoft for handling tasks related to multimedia, especially game and video programming, on Microsoft platforms. While it includes several components, we’ll focus on Direct3D, which is used for rendering 3D graphics.
Key Features of DirectX
- Optimized for Windows and Xbox platforms
- Tightly integrated with Windows operating system
- Excellent performance on supported hardware
- Comprehensive set of tools and debugging features
- Regular updates and improvements from Microsoft
Getting Started with DirectX
To begin working with DirectX, you’ll need to set up your development environment:
- Install Visual Studio (Community edition is free and sufficient)
- Install the Windows SDK, which includes DirectX
- Set up a new DirectX project in Visual Studio
Here’s a basic example of initializing DirectX and clearing the screen:
#include <windows.h>
#include <d3d11.h>
#include <dxgi.h>
#include <d3dcompiler.h>
#include <directxmath.h>
#include <directxcolors.h>
using namespace DirectX;
// DirectX pointers
ID3D11Device* g_pd3dDevice = nullptr;
ID3D11DeviceContext* g_pImmediateContext = nullptr;
IDXGISwapChain* g_pSwapChain = nullptr;
ID3D11RenderTargetView* g_pRenderTargetView = nullptr;
// Window handle
HWND g_hWnd = nullptr;
// Forward declarations
HRESULT InitWindow(HINSTANCE hInstance, int nCmdShow);
HRESULT InitDevice();
void CleanupDevice();
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
void Render();
// Entry point
int WINAPI wWinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPWSTR lpCmdLine, int nCmdShow) {
UNREFERENCED_PARAMETER(hPrevInstance);
UNREFERENCED_PARAMETER(lpCmdLine);
if (FAILED(InitWindow(hInstance, nCmdShow)))
return 0;
if (FAILED(InitDevice())) {
CleanupDevice();
return 0;
}
// Main message loop
MSG msg = {0};
while (WM_QUIT != msg.message) {
if (PeekMessage(&msg, nullptr, 0, 0, PM_REMOVE)) {
TranslateMessage(&msg);
DispatchMessage(&msg);
} else {
Render();
}
}
CleanupDevice();
return (int)msg.wParam;
}
// Initialize the window
HRESULT InitWindow(HINSTANCE hInstance, int nCmdShow) {
// Register class
WNDCLASSEX wcex;
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.style = CS_HREDRAW | CS_VREDRAW;
wcex.lpfnWndProc = WndProc;
wcex.cbClsExtra = 0;
wcex.cbWndExtra = 0;
wcex.hInstance = hInstance;
wcex.hIcon = LoadIcon(hInstance, (LPCTSTR)IDI_APPLICATION);
wcex.hCursor = LoadCursor(nullptr, IDC_ARROW);
wcex.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wcex.lpszMenuName = nullptr;
wcex.lpszClassName = L"DirectXWindowClass";
wcex.hIconSm = LoadIcon(wcex.hInstance, (LPCTSTR)IDI_APPLICATION);
if (!RegisterClassEx(&wcex))
return E_FAIL;
// Create window
g_hWnd = CreateWindow(L"DirectXWindowClass", L"DirectX Tutorial", WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 800, 600, nullptr, nullptr, hInstance, nullptr);
if (!g_hWnd)
return E_FAIL;
ShowWindow(g_hWnd, nCmdShow);
return S_OK;
}
// Initialize Direct3D
HRESULT InitDevice() {
HRESULT hr = S_OK;
RECT rc;
GetClientRect(g_hWnd, &rc);
UINT width = rc.right - rc.left;
UINT height = rc.bottom - rc.top;
UINT createDeviceFlags = 0;
#ifdef _DEBUG
createDeviceFlags |= D3D11_CREATE_DEVICE_DEBUG;
#endif
D3D_DRIVER_TYPE driverTypes[] = {
D3D_DRIVER_TYPE_HARDWARE,
D3D_DRIVER_TYPE_WARP,
D3D_DRIVER_TYPE_REFERENCE,
};
UINT numDriverTypes = ARRAYSIZE(driverTypes);
D3D_FEATURE_LEVEL featureLevels[] = {
D3D_FEATURE_LEVEL_11_0,
D3D_FEATURE_LEVEL_10_1,
D3D_FEATURE_LEVEL_10_0,
};
UINT numFeatureLevels = ARRAYSIZE(featureLevels);
DXGI_SWAP_CHAIN_DESC sd;
ZeroMemory(&sd, sizeof(sd));
sd.BufferCount = 1;
sd.BufferDesc.Width = width;
sd.BufferDesc.Height = height;
sd.BufferDesc.Format = DXGI_FORMAT_R8G8B8A8_UNORM;
sd.BufferDesc.RefreshRate.Numerator = 60;
sd.BufferDesc.RefreshRate.Denominator = 1;
sd.BufferUsage = DXGI_USAGE_RENDER_TARGET_OUTPUT;
sd.OutputWindow = g_hWnd;
sd.SampleDesc.Count = 1;
sd.SampleDesc.Quality = 0;
sd.Windowed = TRUE;
for (UINT driverTypeIndex = 0; driverTypeIndex < numDriverTypes; driverTypeIndex++) {
D3D_DRIVER_TYPE driverType = driverTypes[driverTypeIndex];
hr = D3D11CreateDeviceAndSwapChain(nullptr, driverType, nullptr, createDeviceFlags, featureLevels, numFeatureLevels,
D3D11_SDK_VERSION, &sd, &g_pSwapChain, &g_pd3dDevice, nullptr, &g_pImmediateContext);
if (SUCCEEDED(hr))
break;
}
if (FAILED(hr))
return hr;
// Create a render target view
ID3D11Texture2D* pBackBuffer = nullptr;
hr = g_pSwapChain->GetBuffer(0, __uuidof(ID3D11Texture2D), (LPVOID*)&pBackBuffer);
if (FAILED(hr))
return hr;
hr = g_pd3dDevice->CreateRenderTargetView(pBackBuffer, nullptr, &g_pRenderTargetView);
pBackBuffer->Release();
if (FAILED(hr))
return hr;
g_pImmediateContext->OMSetRenderTargets(1, &g_pRenderTargetView, nullptr);
// Setup the viewport
D3D11_VIEWPORT vp;
vp.Width = (FLOAT)width;
vp.Height = (FLOAT)height;
vp.MinDepth = 0.0f;
vp.MaxDepth = 1.0f;
vp.TopLeftX = 0;
vp.TopLeftY = 0;
g_pImmediateContext->RSSetViewports(1, &vp);
return S_OK;
}
// Clean up the objects we've created
void CleanupDevice() {
if (g_pImmediateContext) g_pImmediateContext->ClearState();
if (g_pRenderTargetView) g_pRenderTargetView->Release();
if (g_pSwapChain) g_pSwapChain->Release();
if (g_pImmediateContext) g_pImmediateContext->Release();
if (g_pd3dDevice) g_pd3dDevice->Release();
}
// Called every time the application receives a message
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam) {
PAINTSTRUCT ps;
HDC hdc;
switch (message) {
case WM_PAINT:
hdc = BeginPaint(hWnd, &ps);
EndPaint(hWnd, &ps);
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hWnd, message, wParam, lParam);
}
return 0;
}
// Render a frame
void Render() {
// Clear the back buffer
g_pImmediateContext->ClearRenderTargetView(g_pRenderTargetView, Colors::MidnightBlue);
// Present the information rendered to the back buffer to the front buffer (the screen)
g_pSwapChain->Present(0, 0);
}
This example sets up a basic DirectX application that creates a window and clears the screen with a solid color.
Advanced DirectX Techniques
As you progress with DirectX, you can explore more advanced topics:
- Shader programming using HLSL (High-Level Shader Language)
- 3D model rendering and animation
- Advanced lighting techniques (e.g., deferred rendering, global illumination)
- Physics simulation
- DirectX 12 for improved performance and lower-level control
Choosing Between OpenGL and DirectX
When deciding between OpenGL and DirectX, consider the following factors:
- Platform support: OpenGL for cross-platform development, DirectX for Windows/Xbox
- Performance: Both offer excellent performance, but DirectX may have an edge on Windows
- Learning curve: OpenGL might be easier to start with due to its extensive documentation and community support
- Industry trends: Many game engines support both, but DirectX is more common in AAA game development
- Project requirements: Consider the specific needs of your application
Conclusion
Computer graphics programming is a vast and exciting field with endless possibilities. Whether you choose OpenGL or DirectX, you’ll be embarking on a journey that combines creativity with technical expertise. Both APIs offer powerful tools for creating stunning visual experiences, from simple 2D graphics to complex 3D environments.
As you continue your learning journey, remember that mastering graphics programming takes time and practice. Start with simple projects and gradually work your way up to more complex applications. Experiment with different techniques, study existing code, and don’t be afraid to push the boundaries of what’s possible.
In the context of AlgoCademy’s focus on coding education and programming skills development, exploring computer graphics programming can be an excellent way to enhance your problem-solving abilities and deepen your understanding of low-level programming concepts. The skills you develop in graphics programming, such as optimization, parallel processing, and working with complex APIs, are valuable across many areas of software development.
Whether you’re aiming to create the next blockbuster game, develop scientific visualizations, or push the boundaries of user interface design, the world of computer graphics programming offers endless opportunities for innovation and creativity. So dive in, start coding, and let your imagination run wild!
Additional Resources
To further your learning in computer graphics programming, consider exploring these resources:
- Learn OpenGL: A comprehensive, modern OpenGL tutorial
- Microsoft DirectX Documentation: Official documentation and tutorials for DirectX
- Khronos OpenGL Website: Official OpenGL resources and specifications
- Scratchapixel: In-depth articles on computer graphics concepts
- Real-Time Rendering: A comprehensive book on modern graphics techniques
Remember, the key to mastering computer graphics programming is consistent practice and a willingness to experiment. Happy coding!