Exploring Computational Geometry: Applying Algorithms to Solve Geometric Problems
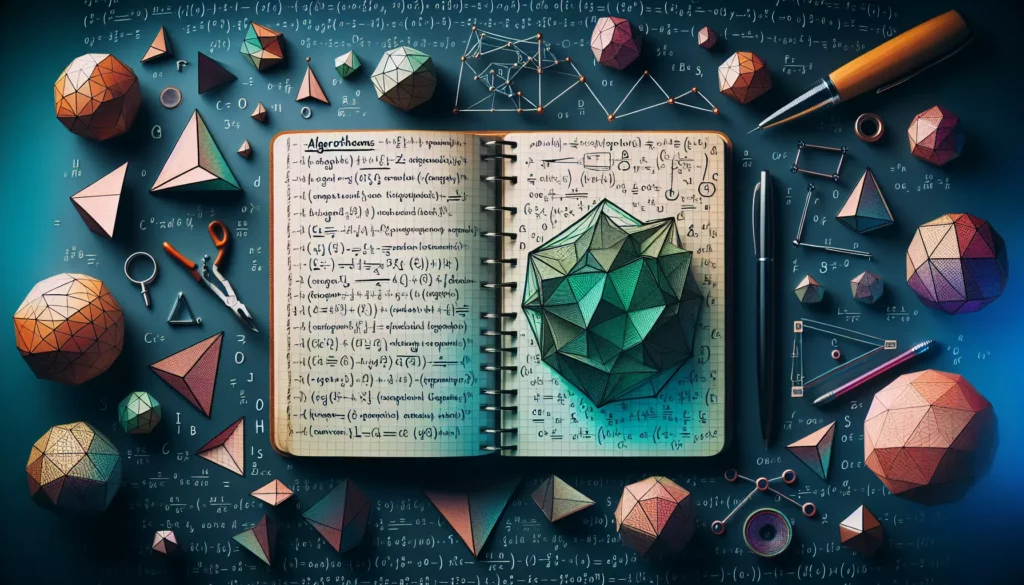
Computational geometry is a fascinating field that combines the principles of geometry with the power of algorithms to solve complex spatial problems. In this comprehensive guide, we’ll dive deep into the world of computational geometry, exploring its applications, key concepts, and essential algorithms. Whether you’re a beginner programmer or an experienced developer looking to expand your skills, this article will provide valuable insights into this crucial area of computer science.
What is Computational Geometry?
Computational geometry is a branch of computer science that focuses on the study of algorithms for solving geometric problems. It deals with the design and analysis of efficient algorithms and data structures for manipulating and processing geometric objects. These objects can include points, lines, polygons, and higher-dimensional shapes.
The field of computational geometry has numerous practical applications, including:
- Computer graphics and animation
- Geographic Information Systems (GIS)
- Robotics and motion planning
- Computer-Aided Design (CAD) and manufacturing
- Image processing and computer vision
- Virtual reality and augmented reality
- Scientific simulations and modeling
Fundamental Concepts in Computational Geometry
Before diving into specific algorithms, it’s essential to understand some fundamental concepts in computational geometry:
1. Points and Vectors
Points are the most basic geometric objects, typically represented by their coordinates in a Cartesian plane. Vectors are directed line segments that can be used to represent displacement or direction.
2. Lines and Line Segments
Lines are infinite in both directions and can be represented by equations or two points. Line segments are finite portions of lines, defined by their endpoints.
3. Polygons
Polygons are closed shapes formed by connecting a series of points (vertices) with line segments (edges). Common types include triangles, rectangles, and more complex shapes.
4. Convex Hull
The convex hull of a set of points is the smallest convex polygon that encloses all the points. It’s a fundamental concept in many computational geometry algorithms.
5. Voronoi Diagrams
A Voronoi diagram partitions a plane into regions based on the distance to a given set of points. Each region contains all points closer to a specific input point than to any other input point.
Essential Algorithms in Computational Geometry
Now that we’ve covered the basics, let’s explore some of the most important algorithms in computational geometry:
1. Line Intersection
Determining whether two line segments intersect is a fundamental problem in computational geometry. Here’s a simple algorithm to check for line segment intersection:
def orientation(p, q, r):
val = (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
if val == 0:
return 0 # Collinear
return 1 if val > 0 else 2 # Clockwise or Counterclockwise
def do_intersect(p1, q1, p2, q2):
o1 = orientation(p1, q1, p2)
o2 = orientation(p1, q1, q2)
o3 = orientation(p2, q2, p1)
o4 = orientation(p2, q2, q1)
if o1 != o2 and o3 != o4:
return True
if o1 == 0 and on_segment(p1, p2, q1):
return True
if o2 == 0 and on_segment(p1, q2, q1):
return True
if o3 == 0 and on_segment(p2, p1, q2):
return True
if o4 == 0 and on_segment(p2, q1, q2):
return True
return False
def on_segment(p, q, r):
return (q[0] <= max(p[0], r[0]) and q[0] >= min(p[0], r[0]) and
q[1] <= max(p[1], r[1]) and q[1] >= min(p[1], r[1]))
# Example usage
p1, q1 = (1, 1), (10, 1)
p2, q2 = (1, 2), (10, 2)
print(do_intersect(p1, q1, p2, q2)) # False
p3, q3 = (10, 0), (0, 10)
p4, q4 = (0, 0), (10, 10)
print(do_intersect(p3, q3, p4, q4)) # True
This algorithm uses the concept of orientation to determine if two line segments intersect. It’s efficient and works for all cases, including collinear segments.
2. Convex Hull – Graham Scan Algorithm
The Graham Scan algorithm is an efficient method for computing the convex hull of a set of points. Here’s an implementation in Python:
def orientation(p, q, r):
return (q[1] - p[1]) * (r[0] - q[0]) - (q[0] - p[0]) * (r[1] - q[1])
def graham_scan(points):
n = len(points)
if n < 3:
return points
# Find the bottommost point (and leftmost if there are multiple)
bottom_point = min(points, key=lambda p: (p[1], p[0]))
# Sort points based on polar angle with respect to the bottom point
sorted_points = sorted(points, key=lambda p: (math.atan2(p[1] - bottom_point[1], p[0] - bottom_point[0]), p))
stack = [bottom_point, sorted_points[0], sorted_points[1]]
for i in range(2, n):
while len(stack) > 1 and orientation(stack[-2], stack[-1], sorted_points[i]) <= 0:
stack.pop()
stack.append(sorted_points[i])
return stack
# Example usage
points = [(0, 3), (2, 2), (1, 1), (2, 1), (3, 0), (0, 0), (3, 3)]
convex_hull = graham_scan(points)
print(convex_hull) # [(0, 0), (3, 0), (3, 3), (0, 3)]
The Graham Scan algorithm works by first finding the bottommost point, then sorting the remaining points based on their polar angle with respect to this point. It then builds the convex hull by iteratively adding points and removing those that create concavities.
3. Closest Pair of Points
Finding the closest pair of points in a set is another fundamental problem in computational geometry. Here’s an efficient divide-and-conquer algorithm to solve this problem:
import math
def distance(p1, p2):
return math.sqrt((p1[0] - p2[0])**2 + (p1[1] - p2[1])**2)
def brute_force(points):
n = len(points)
min_dist = float('inf')
for i in range(n):
for j in range(i+1, n):
dist = distance(points[i], points[j])
if dist < min_dist:
min_dist = dist
return min_dist
def strip_closest(strip, d):
strip.sort(key=lambda point: point[1])
min_dist = d
for i in range(len(strip)):
j = i + 1
while j < len(strip) and (strip[j][1] - strip[i][1]) < min_dist:
min_dist = min(min_dist, distance(strip[i], strip[j]))
j += 1
return min_dist
def closest_pair(points):
n = len(points)
if n <= 3:
return brute_force(points)
mid = n // 2
mid_point = points[mid]
left = points[:mid]
right = points[mid:]
d_left = closest_pair(left)
d_right = closest_pair(right)
d = min(d_left, d_right)
strip = [p for p in points if abs(p[0] - mid_point[0]) < d]
return min(d, strip_closest(strip, d))
# Example usage
points = [(2, 3), (12, 30), (40, 50), (5, 1), (12, 10), (3, 4)]
points.sort() # Sort points based on x-coordinate
min_distance = closest_pair(points)
print(f"The closest pair of points are {min_distance:.2f} units apart.")
This algorithm uses a divide-and-conquer approach, recursively dividing the points into two halves, solving the problem for each half, and then combining the results. It achieves an average time complexity of O(n log n).
4. Point in Polygon
Determining whether a point lies inside a polygon is a common problem in computational geometry. The ray casting algorithm is an efficient solution:
def is_point_in_polygon(point, polygon):
x, y = point
n = len(polygon)
inside = False
p1x, p1y = polygon[0]
for i in range(n + 1):
p2x, p2y = polygon[i % n]
if y > min(p1y, p2y):
if y <= max(p1y, p2y):
if x <= max(p1x, p2x):
if p1y != p2y:
xinters = (y - p1y) * (p2x - p1x) / (p2y - p1y) + p1x
if p1x == p2x or x <= xinters:
inside = not inside
p1x, p1y = p2x, p2y
return inside
# Example usage
polygon = [(0, 0), (5, 0), (5, 5), (0, 5)]
point1 = (2, 2)
point2 = (6, 6)
print(f"Is {point1} inside the polygon? {is_point_in_polygon(point1, polygon)}") # True
print(f"Is {point2} inside the polygon? {is_point_in_polygon(point2, polygon)}") # False
This algorithm works by casting a ray from the point and counting the number of times it intersects with the polygon’s edges. If the number of intersections is odd, the point is inside the polygon.
Advanced Topics in Computational Geometry
As you progress in your understanding of computational geometry, you may want to explore more advanced topics and algorithms:
1. Delaunay Triangulation
Delaunay triangulation is a way of connecting a set of points to form a triangular mesh. It has the property that no point in the set is inside the circumcircle of any triangle in the mesh. Delaunay triangulations are widely used in computer graphics and terrain modeling.
2. Voronoi Diagrams
Voronoi diagrams are dual to Delaunay triangulations and have numerous applications in various fields, including computer graphics, robotics, and epidemiology. They partition a plane into regions based on the distance to a set of points.
3. Spatial Data Structures
Efficient data structures for storing and querying geometric data are crucial in computational geometry. Some important spatial data structures include:
- K-d trees
- Quad trees
- R-trees
- Bounding Volume Hierarchies (BVH)
4. Geometric Intersection Problems
More complex intersection problems involve multiple geometric objects, such as:
- Line segment intersection: Finding all intersections in a set of line segments
- Polygon intersection: Determining the intersection of two or more polygons
- Ray tracing: Finding intersections between rays and geometric objects
5. Motion Planning
Motion planning algorithms use computational geometry techniques to find collision-free paths for robots or virtual characters in complex environments. Some popular algorithms include:
- Rapidly-exploring Random Trees (RRT)
- Probabilistic Roadmaps (PRM)
- Potential Field methods
Practical Applications of Computational Geometry
Computational geometry has a wide range of practical applications across various industries:
1. Computer Graphics and Animation
In computer graphics, computational geometry is used for tasks such as:
- Collision detection in video games and simulations
- Ray tracing for realistic lighting and shadows
- Mesh generation and simplification for 3D models
- Path planning for character movement
2. Geographic Information Systems (GIS)
GIS applications rely heavily on computational geometry for:
- Map overlay and spatial analysis
- Terrain modeling and visibility analysis
- Route planning and optimization
- Spatial indexing for efficient querying of geographic data
3. Computer-Aided Design (CAD) and Manufacturing
In CAD and manufacturing, computational geometry is used for:
- Solid modeling and boolean operations on 3D objects
- Tool path generation for CNC machines
- Tolerance analysis and interference checking
- Nesting and packing problems for efficient material usage
4. Robotics and Automation
Robotics applications of computational geometry include:
- Path planning and obstacle avoidance
- Simultaneous Localization and Mapping (SLAM)
- Grasp planning for robotic manipulators
- Coverage path planning for autonomous cleaning robots
5. Computer Vision and Image Processing
In computer vision, computational geometry is used for:
- Feature detection and matching
- Image segmentation and object recognition
- 3D reconstruction from multiple views
- Camera calibration and pose estimation
Learning Resources and Tools
To further your understanding of computational geometry, consider exploring these resources:
1. Books
- “Computational Geometry: Algorithms and Applications” by Mark de Berg, et al.
- “Geometric Algorithms and Combinatorial Optimization” by Martin Grötschel, et al.
- “Computational Geometry in C” by Joseph O’Rourke
2. Online Courses
- Coursera: “Computational Geometry” by École Normale Supérieure
- edX: “Computational Geometry” by Tsinghua University
- Udacity: “Computational Photography” (includes relevant geometry topics)
3. Libraries and Tools
- CGAL (Computational Geometry Algorithms Library): A powerful C++ library for computational geometry
- Shapely: A Python library for manipulation and analysis of geometric objects
- GeoTools: An open-source Java library for geospatial data manipulation
- OpenSCAD: A 3D modeling software that uses a scripting language for creating solid 3D CAD objects
Conclusion
Computational geometry is a fascinating field that combines mathematical principles with algorithmic thinking to solve complex spatial problems. As we’ve explored in this article, it has numerous applications across various industries and plays a crucial role in many modern technologies.
By understanding the fundamental concepts and algorithms of computational geometry, you’ll be better equipped to tackle spatial problems in your own projects and applications. Whether you’re working on computer graphics, robotics, or geographic information systems, the principles of computational geometry will prove invaluable.
As you continue your journey in programming and computer science, consider diving deeper into computational geometry. The skills and knowledge you gain will not only expand your problem-solving toolkit but also open up new opportunities in cutting-edge fields like augmented reality, autonomous vehicles, and advanced manufacturing.
Remember, the key to mastering computational geometry, like any area of computer science, is practice. Implement the algorithms discussed in this article, experiment with different geometric problems, and challenge yourself to optimize and improve your solutions. With dedication and persistence, you’ll soon be solving complex geometric problems with ease and confidence.