Exploring Blockchains and Cryptocurrency Programming: Developing Decentralized Applications (dApps) on Ethereum
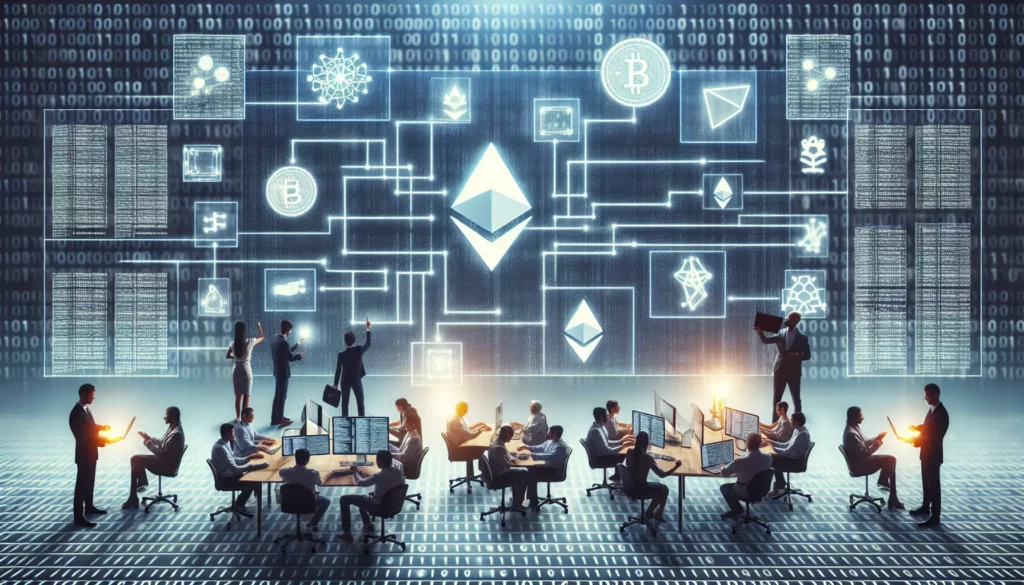
In the ever-evolving landscape of technology, blockchain and cryptocurrency have emerged as transformative forces, reshaping industries and challenging traditional paradigms. As coding education platforms like AlgoCademy continue to equip learners with essential programming skills, it’s crucial to delve into the world of blockchain development. This article will explore the fascinating realm of blockchain technology, with a specific focus on developing decentralized applications (dApps) using the Ethereum platform.
Understanding Blockchain Technology
Before we dive into the intricacies of dApp development, it’s essential to grasp the fundamentals of blockchain technology. At its core, a blockchain is a distributed, immutable ledger that records transactions across a network of computers. This decentralized approach eliminates the need for intermediaries and ensures transparency, security, and trust in digital interactions.
Key characteristics of blockchain technology include:
- Decentralization: No single entity controls the network
- Transparency: All transactions are visible to network participants
- Immutability: Once recorded, data cannot be altered without consensus
- Security: Cryptographic techniques ensure data integrity
Introduction to Ethereum
Ethereum, launched in 2015 by Vitalik Buterin, is a blockchain-based platform that extends beyond simple cryptocurrency transactions. It introduces the concept of smart contracts, self-executing agreements with the terms of the contract directly written into code. This innovation has paved the way for the development of decentralized applications (dApps) that can operate without centralized control.
Key Components of Ethereum
- Ether (ETH): The native cryptocurrency of the Ethereum network
- Smart Contracts: Self-executing code that runs on the Ethereum Virtual Machine (EVM)
- Gas: A measure of computational effort required to execute operations on the network
- Solidity: The primary programming language for writing smart contracts on Ethereum
Getting Started with Ethereum Development
To begin developing decentralized applications on Ethereum, you’ll need to set up your development environment. Here are the essential tools and frameworks you’ll need:
1. Node.js and npm
Install Node.js and npm (Node Package Manager) to manage dependencies and run JavaScript-based tools.
2. Truffle Suite
Truffle is a popular development framework for Ethereum that simplifies the process of building and testing smart contracts. Install Truffle globally using npm:
npm install -g truffle
3. Ganache
Ganache provides a local Ethereum blockchain for testing and development purposes. It allows you to deploy contracts, develop applications, and run tests without incurring real costs.
4. MetaMask
MetaMask is a browser extension that serves as an Ethereum wallet and allows you to interact with dApps directly from your browser.
5. Solidity
Solidity is the primary programming language for writing smart contracts on Ethereum. Familiarize yourself with its syntax and concepts.
Creating Your First Smart Contract
Let’s create a simple smart contract to demonstrate the basics of Ethereum development. We’ll create a “Hello, World!” contract that stores a greeting message and allows it to be updated.
pragma solidity ^0.8.0;
contract HelloWorld {
string public greeting;
constructor() {
greeting = "Hello, World!";
}
function setGreeting(string memory _greeting) public {
greeting = _greeting;
}
function getGreeting() public view returns (string memory) {
return greeting;
}
}
This contract does the following:
- Declares a public string variable
greeting
- Initializes the greeting in the constructor
- Provides a function to update the greeting
- Includes a function to retrieve the current greeting
Deploying and Interacting with Smart Contracts
To deploy your smart contract, you’ll need to use Truffle and Ganache. Here’s a step-by-step guide:
1. Initialize a Truffle Project
mkdir hello-world-dapp
cd hello-world-dapp
truffle init
2. Create a New Contract File
Create a new file named HelloWorld.sol
in the contracts/
directory and paste the smart contract code from earlier.
3. Create a Migration Script
In the migrations/
directory, create a new file named 2_deploy_contracts.js
with the following content:
const HelloWorld = artifacts.require("HelloWorld");
module.exports = function(deployer) {
deployer.deploy(HelloWorld);
};
4. Configure Truffle
Update the truffle-config.js
file to connect to your local Ganache instance:
module.exports = {
networks: {
development: {
host: "127.0.0.1",
port: 7545,
network_id: "*"
}
},
compilers: {
solc: {
version: "0.8.0"
}
}
};
5. Compile and Deploy
Run the following commands to compile and deploy your smart contract:
truffle compile
truffle migrate
6. Interact with the Contract
Use the Truffle console to interact with your deployed contract:
truffle console
// Get the deployed contract instance
let instance = await HelloWorld.deployed()
// Get the current greeting
await instance.getGreeting()
// Set a new greeting
await instance.setGreeting("Hello, Ethereum!")
// Verify the new greeting
await instance.getGreeting()
Building a Frontend for Your dApp
To create a user-friendly interface for your dApp, you’ll need to build a frontend application that interacts with your smart contract. Here’s a simple example using HTML, JavaScript, and Web3.js:
1. Set Up the HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Hello World dApp</title>
</head>
<body>
<h1>Hello World dApp</h1>
<p>Current Greeting: <span id="greeting"></span></p>
<input type="text" id="newGreeting" placeholder="Enter new greeting">
<button onclick="setGreeting()">Set Greeting</button>
<script src="https://cdn.jsdelivr.net/npm/web3@1.5.2/dist/web3.min.js"></script>
<script src="app.js"></script>
</body>
</html>
2. Implement the JavaScript Logic
Create an app.js
file with the following content:
const contractAddress = "YOUR_CONTRACT_ADDRESS";
const contractABI = [
// Add your contract ABI here
];
let web3;
let contract;
async function init() {
if (typeof window.ethereum !== "undefined") {
web3 = new Web3(window.ethereum);
try {
await window.ethereum.enable();
contract = new web3.eth.Contract(contractABI, contractAddress);
updateGreeting();
} catch (error) {
console.error("User denied account access");
}
} else {
console.log("Please install MetaMask");
}
}
async function updateGreeting() {
const greeting = await contract.methods.getGreeting().call();
document.getElementById("greeting").textContent = greeting;
}
async function setGreeting() {
const newGreeting = document.getElementById("newGreeting").value;
const accounts = await web3.eth.getAccounts();
await contract.methods.setGreeting(newGreeting).send({ from: accounts[0] });
updateGreeting();
}
init();
Remember to replace YOUR_CONTRACT_ADDRESS
with the actual address of your deployed contract and add the correct ABI (Application Binary Interface) for your smart contract.
Best Practices for dApp Development
As you delve deeper into dApp development, keep these best practices in mind:
- Security First: Always prioritize security in your smart contracts. Use established patterns and libraries, and consider having your code audited by professionals.
- Gas Optimization: Ethereum transactions require gas, so optimize your code to minimize gas costs for users.
- Error Handling: Implement robust error handling in both your smart contracts and frontend code.
- Testing: Thoroughly test your smart contracts using Truffle’s testing framework and consider using tools like Ganache for local blockchain testing.
- Upgradability: Plan for contract upgrades from the beginning, as smart contracts are immutable once deployed.
- User Experience: Focus on creating intuitive user interfaces that abstract the complexities of blockchain interactions.
- Compliance: Be aware of regulatory requirements in your jurisdiction regarding cryptocurrency and blockchain applications.
Advanced Topics in Ethereum Development
As you progress in your Ethereum development journey, consider exploring these advanced topics:
1. Token Standards
Learn about various token standards like ERC-20 for fungible tokens and ERC-721 for non-fungible tokens (NFTs).
2. Decentralized Finance (DeFi)
Explore the world of decentralized finance, including concepts like automated market makers, yield farming, and lending protocols.
3. Layer 2 Solutions
Understand scaling solutions like Optimistic Rollups and zk-Rollups that aim to improve Ethereum’s transaction throughput and reduce gas costs.
4. Oracles
Learn how to integrate real-world data into your smart contracts using oracle services like Chainlink.
5. Interoperability
Explore cross-chain communication and interoperability solutions to connect different blockchain networks.
Conclusion
Developing decentralized applications on Ethereum opens up a world of possibilities for creating trustless, transparent, and innovative solutions. As you continue your journey in blockchain development, remember that platforms like AlgoCademy can provide valuable resources and guidance to enhance your coding skills and algorithmic thinking.
The field of blockchain and cryptocurrency programming is rapidly evolving, with new tools, frameworks, and best practices emerging regularly. Stay curious, keep learning, and don’t hesitate to experiment with different approaches and technologies. By mastering the fundamentals of smart contract development and staying up-to-date with the latest advancements, you’ll be well-equipped to build the decentralized applications of tomorrow.
As you progress in your blockchain development journey, consider how the problem-solving skills and coding practices you’ve learned through platforms like AlgoCademy can be applied to create more efficient, secure, and scalable dApps. The ability to think algorithmically and optimize code is crucial in the resource-constrained environment of blockchain networks.
Remember that blockchain development is not just about writing code; it’s about reimagining systems and processes in a decentralized context. As you build your dApps, always consider the broader implications of your work and how it can contribute to a more open, transparent, and equitable digital ecosystem.
The world of blockchain and cryptocurrency programming is full of challenges and opportunities. By combining your growing expertise in this field with the solid foundation of coding skills provided by platforms like AlgoCademy, you’ll be well-positioned to make significant contributions to this revolutionary technology landscape. Keep coding, keep exploring, and be part of the decentralized future!