Exploring Bit Manipulation Techniques: Mastering the Art of Binary Operations
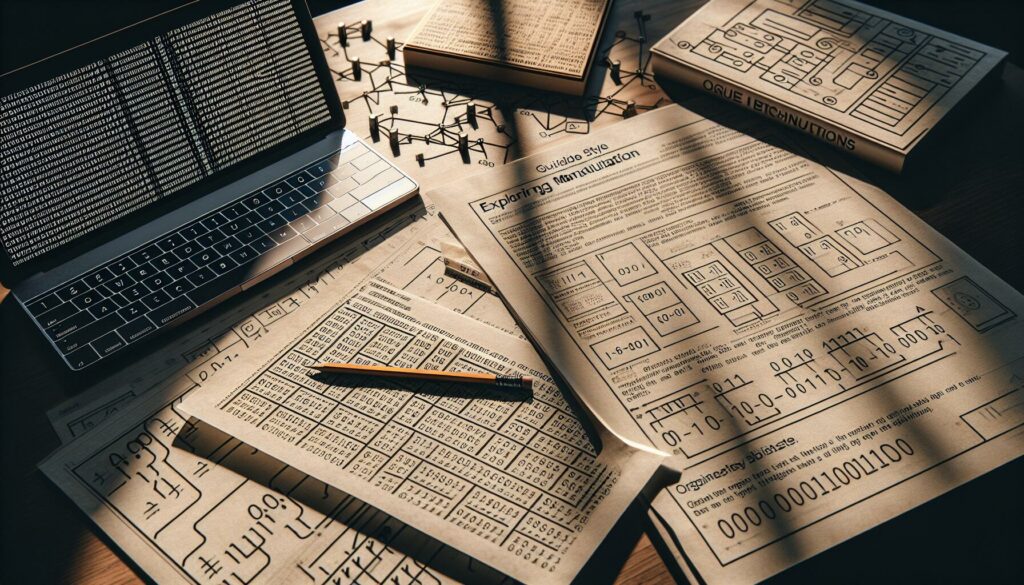
In the world of computer science and programming, bit manipulation is a powerful technique that allows developers to perform operations at the most fundamental level of computing: individual bits. Understanding and mastering bit manipulation can lead to more efficient algorithms, optimized code, and a deeper comprehension of how computers process information. In this comprehensive guide, we’ll explore various bit manipulation techniques, their applications, and how they can be used to solve complex problems in programming.
What is Bit Manipulation?
Bit manipulation refers to the process of applying logical operations on a sequence of bits to achieve a desired result. These operations are performed on the binary representation of numbers, which is the fundamental way computers store and process data. By manipulating individual bits, we can perform various tasks more efficiently than traditional arithmetic operations.
Why is Bit Manipulation Important?
Bit manipulation techniques are crucial for several reasons:
- Efficiency: Bit operations are typically faster than arithmetic operations.
- Space optimization: Bit manipulation can help in reducing memory usage.
- Low-level programming: It’s essential for systems programming and embedded systems.
- Algorithmic problem-solving: Many complex problems can be solved efficiently using bit manipulation.
- Cryptography: Bit operations are fundamental in encryption and decryption algorithms.
Basic Bitwise Operators
Before diving into advanced techniques, let’s review the basic bitwise operators available in most programming languages:
- AND (&): Returns 1 if both bits are 1, otherwise 0.
- OR (|): Returns 1 if at least one of the bits is 1, otherwise 0.
- XOR (^): Returns 1 if the bits are different, otherwise 0.
- NOT (~): Inverts all the bits.
- Left Shift (<<): Shifts all bits to the left by a specified number of positions.
- Right Shift (>>): Shifts all bits to the right by a specified number of positions.
Common Bit Manipulation Techniques
1. Checking if a number is even or odd
One of the simplest applications of bit manipulation is checking whether a number is even or odd. We can do this by examining the least significant bit (LSB) of the number.
bool isEven(int num) {
return !(num & 1);
}
bool isOdd(int num) {
return num & 1;
}
In binary, even numbers always end with 0, while odd numbers end with 1. By performing an AND operation with 1, we isolate the LSB. If the result is 0, the number is even; otherwise, it’s odd.
2. Multiplying or dividing by powers of 2
Left shifting a number by n positions is equivalent to multiplying it by 2^n, while right shifting is equivalent to dividing by 2^n (integer division).
int multiplyByPowerOf2(int num, int power) {
return num << power;
}
int divideByPowerOf2(int num, int power) {
return num >> power;
}
This technique is much faster than traditional multiplication or division operations.
3. Setting, clearing, and toggling bits
These operations are fundamental in bit manipulation and are used frequently in various algorithms.
int setBit(int num, int position) {
return num | (1 << position);
}
int clearBit(int num, int position) {
return num & ~(1 << position);
}
int toggleBit(int num, int position) {
return num ^ (1 << position);
}
4. Checking if a number is a power of 2
A number that is a power of 2 has only one bit set in its binary representation. We can use this property to check if a number is a power of 2.
bool isPowerOf2(int num) {
return num && !(num & (num - 1));
}
This function returns true for powers of 2 and false for other numbers. It works because subtracting 1 from a power of 2 will flip all the bits after the set bit, and performing an AND operation with the original number will result in 0.
5. Counting set bits (population count)
Counting the number of set bits (1s) in a binary number is a common problem in bit manipulation. Here’s an efficient method known as Brian Kernighan’s algorithm:
int countSetBits(int num) {
int count = 0;
while (num) {
num &= (num - 1);
count++;
}
return count;
}
This algorithm works by repeatedly clearing the least significant set bit until the number becomes 0.
Advanced Bit Manipulation Techniques
1. Finding the rightmost set bit
To find the position of the rightmost set bit in a number, we can use the following technique:
int findRightmostSetBit(int num) {
return num & -num;
}
This works because the two’s complement of a number will have all bits flipped up to and including the rightmost set bit. When we AND this with the original number, only the rightmost set bit remains.
2. Isolating the rightmost 0 bit
Similarly, we can isolate the rightmost 0 bit in a number:
int isolateRightmost0Bit(int num) {
return ~num & (num + 1);
}
This technique works by flipping all bits and then adding 1, which will set all bits up to and including the rightmost 0. ANDing this with the complement of the original number isolates the rightmost 0.
3. Swapping values without using a temporary variable
We can use XOR to swap two values without using a temporary variable:
void swapWithoutTemp(int &a, int &b) {
a = a ^ b;
b = a ^ b;
a = a ^ b;
}
This works because XOR is its own inverse operation. The intermediate steps store the XOR of both values, which can then be used to recover each original value.
4. Finding the missing number in a sequence
Given an array containing n distinct numbers taken from 0, 1, 2, …, n, find the one that is missing from the array. We can use XOR to solve this efficiently:
int findMissingNumber(vector<int> &nums) {
int n = nums.size();
int result = n;
for (int i = 0; i < n; i++) {
result ^= i ^ nums[i];
}
return result;
}
This solution works because XOR is commutative and associative, and XORing a number with itself results in 0. By XORing all numbers from 0 to n with all numbers in the array, the missing number will be the only one left.
5. Gray code generation
Gray code is a sequence of binary numbers where adjacent numbers differ by only one bit. We can generate Gray code using bit manipulation:
vector<int> grayCode(int n) {
vector<int> result;
for (int i = 0; i < (1 << n); i++) {
result.push_back(i ^ (i >> 1));
}
return result;
}
This technique works by XORing each number with itself right-shifted by 1, which effectively flips the appropriate bit to create the Gray code sequence.
Practical Applications of Bit Manipulation
1. Optimizing space usage
Bit manipulation can be used to optimize space usage in various scenarios. For example, we can use a single integer to represent a set of boolean flags:
class Flags {
int flags;
public:
void setFlag(int position) { flags |= (1 << position); }
void clearFlag(int position) { flags &= ~(1 << position); }
bool getFlag(int position) { return flags & (1 << position); }
};
This approach can save significant memory when dealing with large numbers of boolean values.
2. Fast multiplication and division
As mentioned earlier, left and right shifts can be used for fast multiplication and division by powers of 2. This technique is often used in performance-critical code:
int fastMultiply(int a, int b) {
int result = 0;
while (b) {
if (b & 1) result += a;
a <<= 1;
b >>= 1;
}
return result;
}
This algorithm performs multiplication using only shifts and additions, which can be faster than traditional multiplication on some hardware.
3. Implementing data structures
Bit manipulation is often used in implementing efficient data structures. For example, we can implement a trie (prefix tree) using bit manipulation for space efficiency:
class TrieNode {
TrieNode* children[26];
bool isEndOfWord;
public:
TrieNode() {
memset(children, 0, sizeof(children));
isEndOfWord = false;
}
};
class Trie {
TrieNode* root;
public:
Trie() { root = new TrieNode(); }
void insert(string word) {
TrieNode* current = root;
for (char c : word) {
int index = c - 'a';
if (!current->children[index])
current->children[index] = new TrieNode();
current = current->children[index];
}
current->isEndOfWord = true;
}
};
This implementation uses an array of pointers to represent children nodes, which can be more space-efficient than using a map or unordered_map for small alphabets.
4. Cryptography and hashing
Bit manipulation is extensively used in cryptography and hashing algorithms. For example, the XOR operation is often used in simple encryption schemes:
string xorEncrypt(string text, char key) {
string result = text;
for (char &c : result) {
c ^= key;
}
return result;
}
string xorDecrypt(string ciphertext, char key) {
return xorEncrypt(ciphertext, key); // XOR is its own inverse
}
While this is a very basic example and not secure for real-world use, it demonstrates the principle of using XOR in cryptography.
5. Graphics programming
Bit manipulation is often used in graphics programming for tasks such as color manipulation and pixel operations. For example, we can use bit manipulation to extract individual color channels from an RGB value:
int getRed(int rgb) { return (rgb >> 16) & 0xFF; }
int getGreen(int rgb) { return (rgb >> 8) & 0xFF; }
int getBlue(int rgb) { return rgb & 0xFF; }
int combineRGB(int r, int g, int b) {
return (r << 16) | (g << 8) | b;
}
These operations are much faster than using division and modulo operations to extract color components.
Common Pitfalls and Best Practices
While bit manipulation can lead to efficient and elegant solutions, it’s important to be aware of potential pitfalls:
- Readability: Bit manipulation code can be hard to read and understand. Always add clear comments explaining the logic.
- Portability: Be cautious when using bit manipulation on signed integers, as the behavior can vary between different systems.
- Overflow: Be aware of potential overflow issues, especially when shifting bits.
- Premature optimization: Don’t sacrifice code clarity for minor performance gains. Use bit manipulation when it significantly improves performance or solves a problem more elegantly.
- Testing: Thoroughly test bit manipulation code, as errors can be subtle and hard to detect.
Conclusion
Bit manipulation is a powerful tool in a programmer’s arsenal. It allows for efficient solutions to a wide range of problems and is particularly useful in systems programming, algorithm optimization, and low-level operations. By mastering bit manipulation techniques, you can write more efficient code and gain a deeper understanding of how computers process information at the most fundamental level.
As you continue your journey in programming and computer science, remember that bit manipulation is just one of many tools available to you. The key is to understand when and how to apply these techniques effectively. Practice regularly with coding challenges that involve bit manipulation, and you’ll find yourself becoming more comfortable with these powerful operations.
Remember, the goal is not just to write code that works, but to write code that is efficient, maintainable, and elegant. Bit manipulation, when used appropriately, can help you achieve all of these objectives. Happy coding!