Essential Tools for Programmers: Boosting Productivity and Efficiency
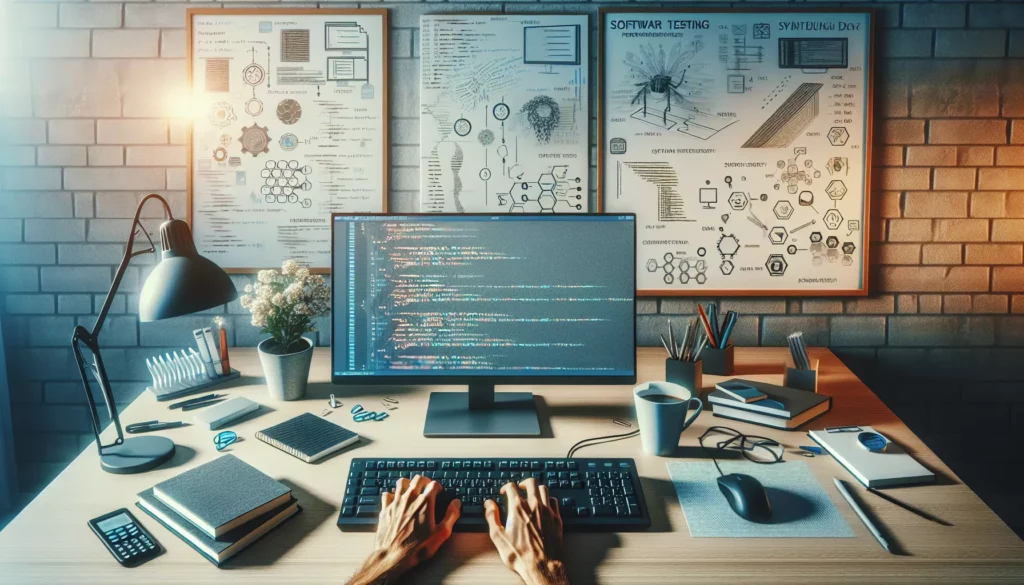
In the ever-evolving world of software development, having the right tools at your disposal can make all the difference in your productivity, efficiency, and overall success as a programmer. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, understanding and utilizing the essential tools of the trade is crucial. In this comprehensive guide, we’ll explore the must-have tools for programmers, covering everything from integrated development environments (IDEs) to version control systems and beyond.
1. Integrated Development Environments (IDEs)
An IDE is arguably the most important tool in a programmer’s arsenal. It combines multiple development tools into a single, user-friendly interface, streamlining the coding process and enhancing productivity. Some popular IDEs include:
- Visual Studio Code: A lightweight, highly customizable IDE that supports multiple programming languages and has a vast ecosystem of extensions.
- IntelliJ IDEA: A powerful Java IDE that also supports other languages like Kotlin, Scala, and JavaScript.
- PyCharm: An IDE specifically designed for Python development, offering advanced features like code analysis and debugging.
- Eclipse: A versatile IDE primarily used for Java development but supports many other languages through plugins.
These IDEs offer features like code completion, syntax highlighting, debugging tools, and integrated terminal access, making them indispensable for efficient coding.
2. Version Control Systems
Version control systems are essential for managing code changes, collaborating with team members, and maintaining project history. The most widely used version control system is Git, often used in conjunction with platforms like:
- GitHub: A web-based hosting service for Git repositories, offering features like pull requests, issue tracking, and project management tools.
- GitLab: Similar to GitHub but with additional features for continuous integration and deployment.
- Bitbucket: Another Git repository management solution, popular for its integration with other Atlassian tools.
Learning to use Git effectively is crucial for any programmer, as it allows for easy collaboration, code review, and version management.
3. Text Editors
While IDEs are comprehensive development environments, sometimes a lightweight text editor is all you need for quick edits or working with simple scripts. Popular text editors include:
- Sublime Text: A fast, feature-rich text editor with a minimalist interface.
- Atom: An open-source, highly customizable text editor developed by GitHub.
- Notepad++: A free, lightweight text editor for Windows with support for multiple programming languages.
These editors often support plugins and extensions, allowing you to customize them to suit your needs.
4. Package Managers
Package managers simplify the process of installing, updating, and managing libraries and dependencies for your projects. Some essential package managers include:
- npm (Node Package Manager): The default package manager for JavaScript and Node.js.
- pip: The package installer for Python, used to install and manage Python packages.
- Maven: A popular build automation and project management tool for Java projects.
- Composer: A dependency manager for PHP.
Using package managers effectively can save you time and ensure consistency across different development environments.
5. Debugging Tools
Debugging is an essential part of the development process, and having the right tools can make it much easier. While many IDEs come with built-in debuggers, some standalone debugging tools include:
- Chrome DevTools: A set of web developer tools built directly into the Google Chrome browser.
- GDB (GNU Debugger): A powerful debugger for C, C++, and other languages.
- Postman: While primarily an API development tool, Postman can be invaluable for debugging HTTP requests and responses.
Mastering debugging tools can significantly reduce the time spent identifying and fixing bugs in your code.
6. Containerization Tools
Containerization has become increasingly important in modern software development, allowing for consistent deployment across different environments. Key tools in this area include:
- Docker: The most popular containerization platform, used for creating, deploying, and running applications in containers.
- Kubernetes: An open-source system for automating deployment, scaling, and management of containerized applications.
Understanding containerization can greatly enhance your ability to develop scalable, portable applications.
7. Continuous Integration/Continuous Deployment (CI/CD) Tools
CI/CD tools automate the process of building, testing, and deploying code, ensuring that changes are integrated smoothly and frequently. Popular CI/CD tools include:
- Jenkins: An open-source automation server that supports building, deploying, and automating any project.
- Travis CI: A hosted continuous integration service used to build and test software projects hosted on GitHub.
- GitLab CI/CD: Integrated CI/CD features within the GitLab platform.
Implementing CI/CD practices can lead to faster development cycles and more reliable software releases.
8. Code Quality and Analysis Tools
Maintaining code quality is crucial for long-term project success. Tools that help in this area include:
- SonarQube: An open-source platform for continuous inspection of code quality.
- ESLint: A static code analysis tool for identifying problematic patterns in JavaScript code.
- Checkstyle: A development tool to help programmers write Java code that adheres to a coding standard.
Regularly using code quality tools can help maintain clean, maintainable code and catch potential issues early in the development process.
9. API Development and Testing Tools
For developers working with APIs, specialized tools can greatly simplify the development and testing process:
- Postman: A popular tool for API development, allowing you to design, test, and document APIs.
- Swagger: An open-source framework backed by a large ecosystem of tools that helps developers design, build, document, and consume RESTful web services.
- Insomnia: A cross-platform HTTP and GraphQL client, great for debugging and testing APIs.
These tools can significantly speed up API development and ensure that your APIs are well-documented and easy to use.
10. Performance Profiling Tools
Optimizing code performance is a critical skill for any programmer. Performance profiling tools can help identify bottlenecks and areas for improvement:
- JProfiler: A Java profiler that helps developers identify performance bottlenecks, memory leaks, and other issues in Java applications.
- Valgrind: An instrumentation framework for building dynamic analysis tools, particularly useful for C and C++ programs.
- Chrome DevTools Performance tab: Offers in-depth performance analysis for web applications.
Regular use of profiling tools can help you write more efficient, performant code.
11. Database Management Tools
For developers working with databases, specialized management tools can be incredibly helpful:
- MySQL Workbench: A visual database design tool that integrates SQL development, administration, database design, creation, and maintenance into a single environment for the MySQL database system.
- pgAdmin: The most popular and feature-rich open-source administration and development platform for PostgreSQL.
- MongoDB Compass: The GUI for MongoDB, allowing you to explore and manipulate your data visually.
These tools can simplify database operations, from designing schemas to writing and optimizing queries.
12. Cloud Platforms and Services
As cloud computing continues to grow in importance, familiarity with major cloud platforms is becoming essential for many developers:
- Amazon Web Services (AWS): Offers a broad set of global cloud-based products including computing, storage, databases, analytics, networking, mobile, developer tools, management tools, IoT, security, and enterprise applications.
- Microsoft Azure: Microsoft’s cloud computing service for building, testing, deploying, and managing applications and services through Microsoft-managed data centers.
- Google Cloud Platform (GCP): A suite of cloud computing services that runs on the same infrastructure that Google uses internally for its end-user products.
Understanding these platforms can open up new possibilities for deploying and scaling your applications.
13. Collaboration and Project Management Tools
Effective collaboration is crucial in software development. Tools that facilitate teamwork and project management include:
- Jira: A popular issue tracking and project management tool, particularly useful for agile development methodologies.
- Trello: A web-based Kanban-style list-making application that can be used for project management and task organization.
- Slack: A channel-based messaging platform that many development teams use for communication and collaboration.
These tools can help keep your team organized, improve communication, and increase overall productivity.
14. Documentation Tools
Good documentation is essential for maintaining and sharing code. Tools that can help with documentation include:
- Doxygen: A documentation generator for various programming languages that can produce documentation from source code comments.
- Sphinx: A tool that makes it easy to create intelligent and beautiful documentation, originally created for Python documentation.
- Javadoc: A documentation generator for Java that generates API documentation in HTML format from Java source code.
Consistent use of documentation tools can make your code more accessible and easier to maintain over time.
15. Learning and Practice Platforms
Continuous learning is a key aspect of a programmer’s career. Platforms that can help you improve your skills include:
- LeetCode: A platform to help you enhance your skills, expand your knowledge and prepare for technical interviews.
- HackerRank: A technology company that focuses on competitive programming challenges for both consumers and businesses.
- Codecademy: An online interactive platform that offers free coding classes in multiple programming languages.
- AlgoCademy: A platform focused on algorithmic thinking and problem-solving, offering interactive coding tutorials and AI-powered assistance to help prepare for technical interviews.
Regularly practicing on these platforms can help you stay sharp and prepare for technical interviews, especially for positions at major tech companies.
Conclusion
The tools mentioned in this guide represent just a fraction of the resources available to modern programmers. The key is to find the right combination of tools that work best for your specific needs and workflow. As you progress in your programming journey, you’ll likely discover additional tools that can further enhance your productivity and capabilities.
Remember, while tools are important, they are just that – tools. The most critical assets for any programmer are problem-solving skills, algorithmic thinking, and a deep understanding of programming concepts. Platforms like AlgoCademy can be invaluable in developing these fundamental skills, offering interactive tutorials, AI-powered assistance, and a focus on practical coding skills that are essential for success in technical interviews and beyond.
As you explore these tools and continue to develop your skills, keep in mind that the field of programming is constantly evolving. Stay curious, keep learning, and don’t be afraid to experiment with new tools and technologies. With the right combination of skills, knowledge, and tools, you’ll be well-equipped to tackle any programming challenge that comes your way.