Essential Tools for Programmers: Boosting Productivity and Efficiency
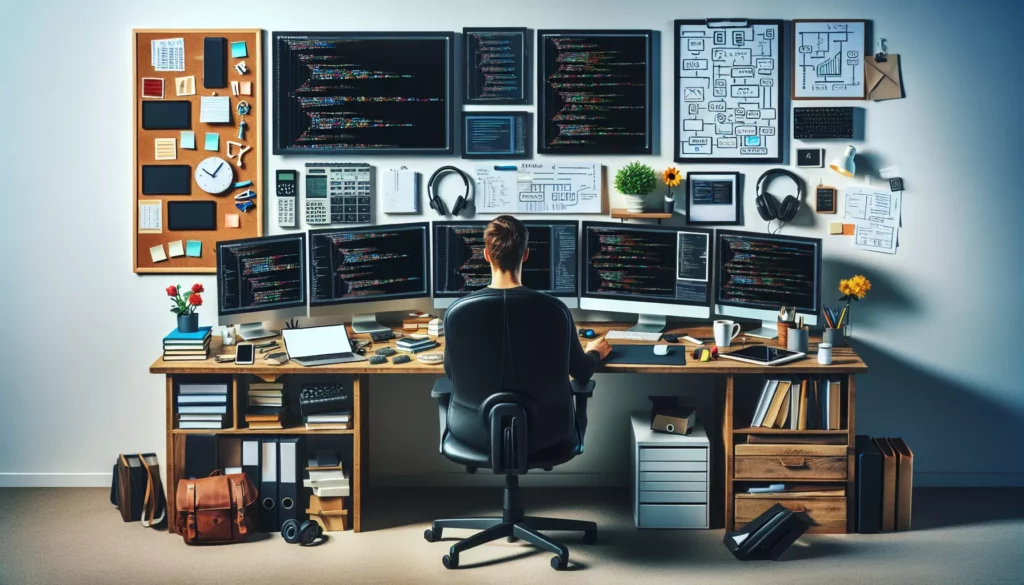
In the ever-evolving world of programming, having the right tools at your disposal can make a significant difference in your productivity, efficiency, and overall success as a developer. Whether you’re a beginner just starting your coding journey or an experienced programmer preparing for technical interviews at top tech companies, having a well-equipped toolkit is crucial. In this comprehensive guide, we’ll explore the essential tools that every programmer should have in their arsenal, from integrated development environments (IDEs) to version control systems and beyond.
1. Integrated Development Environments (IDEs)
An IDE is perhaps the most fundamental tool for any programmer. It’s a software application that provides comprehensive facilities for software development, combining features like code editing, debugging, and compilation into a single, user-friendly interface.
Popular IDEs:
- Visual Studio Code (VS Code): A free, open-source, and highly customizable IDE that supports multiple programming languages.
- IntelliJ IDEA: A powerful Java IDE that also supports other JVM languages like Kotlin and Scala.
- PyCharm: Specifically designed for Python development, offering advanced features for data science and web development.
- Eclipse: A versatile IDE primarily used for Java development but supports many other languages through plugins.
Choosing the right IDE depends on your primary programming language and personal preferences. Many IDEs offer features like code completion, syntax highlighting, and integrated debugging tools that can significantly speed up your development process.
2. Version Control Systems
Version control systems are essential for tracking changes in your code, collaborating with other developers, and managing different versions of your projects. They allow you to revert to previous states, create branches for experimentation, and merge changes from multiple contributors.
Popular Version Control Systems:
- Git: The most widely used distributed version control system.
- GitHub: A web-based platform for hosting Git repositories, offering additional collaboration features.
- GitLab: Similar to GitHub, but with more emphasis on CI/CD pipelines and DevOps features.
- Bitbucket: Another Git-based version control system, popular for its integration with other Atlassian tools.
Learning to use Git effectively is crucial for any programmer, as it’s become the industry standard for version control. Understanding concepts like branching, merging, and resolving conflicts will greatly enhance your ability to work on complex projects and collaborate with other developers.
3. Text Editors
While IDEs offer comprehensive development environments, sometimes you need a lightweight tool for quick edits or working with individual files. This is where text editors come in handy.
Popular Text Editors:
- Sublime Text: A fast and feature-rich text editor with a large ecosystem of plugins.
- Atom: A highly customizable open-source text editor developed by GitHub.
- Notepad++: A free, lightweight text editor for Windows with support for multiple programming languages.
- Vim: A highly configurable text editor built to enable efficient text editing, popular among experienced developers.
Text editors are often preferred for their speed and simplicity when working with individual files or making quick edits. Many developers use a combination of IDEs and text editors depending on the task at hand.
4. Package Managers
Package managers are tools that automate the process of installing, upgrading, configuring, and removing software packages or libraries. They are essential for managing dependencies in your projects and keeping your development environment organized.
Popular Package Managers:
- npm (Node Package Manager): The default package manager for JavaScript and Node.js.
- pip: The package installer for Python, used to install and manage Python packages.
- Maven: A build automation and project management tool primarily used for Java projects.
- Composer: A dependency manager for PHP, used to declare and manage project dependencies.
Understanding how to use package managers effectively can save you a lot of time and effort in managing project dependencies and keeping your development environment consistent across different machines.
5. Debugging Tools
Debugging is an essential part of the development process, and having the right tools can make identifying and fixing bugs much easier. While many IDEs come with built-in debuggers, there are also standalone debugging tools that can provide additional functionality.
Popular Debugging Tools:
- Chrome DevTools: A set of web developer tools built directly into the Google Chrome browser.
- GDB (GNU Debugger): A powerful debugger for C, C++, and other languages.
- Valgrind: An instrumentation framework for building dynamic analysis tools, particularly useful for memory debugging.
- Postman: While primarily an API development tool, it’s also useful for debugging and testing HTTP requests.
Learning to use debugging tools effectively can significantly reduce the time you spend tracking down and fixing bugs in your code. It’s an essential skill for any programmer, regardless of experience level.
6. Code Analysis Tools
Code analysis tools help maintain code quality by identifying potential issues, enforcing coding standards, and suggesting improvements. They can catch bugs early in the development process and help ensure consistent code style across a project.
Popular Code Analysis Tools:
- ESLint: A pluggable linting utility for JavaScript and JSX.
- Pylint: A source code analyzer for Python.
- SonarQube: An open-source platform for continuous inspection of code quality.
- Checkstyle: A development tool to help programmers write Java code that adheres to a coding standard.
Integrating code analysis tools into your development workflow can help you catch potential issues early and maintain a high standard of code quality throughout your projects.
7. Collaboration and Project Management Tools
In today’s interconnected world, collaboration is key to successful software development. Having the right tools to communicate with team members, track tasks, and manage projects is essential for any programmer working in a team environment.
Popular Collaboration and Project Management Tools:
- Slack: A messaging app for team communication and collaboration.
- Trello: A web-based Kanban-style list-making application for project management.
- Jira: A proprietary issue tracking product developed by Atlassian, used for bug tracking, issue tracking, and project management.
- Asana: A web and mobile application designed to help teams organize, track, and manage their work.
Effective use of these tools can greatly enhance team productivity and ensure that everyone is on the same page regarding project goals and progress.
8. Documentation Tools
Good documentation is crucial for maintaining and scaling software projects. Documentation tools help create and maintain clear, organized documentation for your code and projects.
Popular Documentation Tools:
- Swagger: A tool for documenting RESTful APIs.
- Doxygen: A documentation generator for C++, C, Java, and other languages.
- Sphinx: A tool that makes it easy to create intelligent and beautiful documentation for Python projects.
- JSDoc: An API documentation generator for JavaScript.
Proper documentation is essential for maintaining code over time, onboarding new team members, and ensuring that your projects are usable and understandable by others.
9. Containerization and Virtualization Tools
Containerization and virtualization tools help create consistent development environments, simplify deployment processes, and ensure that applications run consistently across different systems.
Popular Containerization and Virtualization Tools:
- Docker: A platform for developing, shipping, and running applications in containers.
- Kubernetes: An open-source system for automating deployment, scaling, and management of containerized applications.
- Vagrant: A tool for building and managing virtual machine environments.
- VirtualBox: A free and open-source hosted hypervisor for x86 virtualization.
Understanding and using these tools can greatly simplify the process of setting up development environments and deploying applications, especially in complex, distributed systems.
10. Continuous Integration/Continuous Deployment (CI/CD) Tools
CI/CD tools automate the process of integrating code changes, running tests, and deploying applications. They are essential for maintaining code quality and streamlining the development process, especially in team environments.
Popular CI/CD Tools:
- Jenkins: An open-source automation server that enables developers to build, test, and deploy their software.
- Travis CI: A hosted continuous integration service used to build and test software projects hosted on GitHub.
- GitLab CI/CD: A part of the GitLab platform that provides a complete CI/CD toolchain.
- CircleCI: A cloud-based CI/CD tool that automates the software development process.
Implementing CI/CD practices with these tools can significantly improve the efficiency and reliability of your development process, catching issues early and ensuring that your code is always in a deployable state.
11. Performance Profiling Tools
Performance profiling tools help identify bottlenecks and optimize the performance of your applications. They are crucial for ensuring that your software runs efficiently and can handle the required load.
Popular Performance Profiling Tools:
- YourKit: A CPU and memory profiler for Java and .NET applications.
- Vtune: Intel’s performance profiler for C, C++, Fortran, Python, and Java.
- Xcode Instruments: A performance, analysis, and testing tool included with Apple’s Xcode IDE.
- dotTrace: A performance profiler for .NET applications.
Using these tools can help you identify performance issues in your code, optimize resource usage, and ensure that your applications run smoothly even under heavy load.
12. API Development and Testing Tools
For developers working on web services or applications that interact with APIs, having tools specifically designed for API development and testing is crucial.
Popular API Development and Testing Tools:
- Postman: A collaboration platform for API development that simplifies each step of building an API.
- Swagger: A suite of tools for API development, including Swagger UI for API documentation and testing.
- Insomnia: A cross-platform HTTP and GraphQL client that simplifies the process of interacting with and testing HTTP-based APIs.
- cURL: A command-line tool for transferring data using various protocols, often used for testing APIs.
These tools can greatly simplify the process of developing, testing, and documenting APIs, ensuring that your web services are robust and well-documented.
13. Database Management Tools
For developers working with databases, having tools to manage, query, and analyze database systems is essential.
Popular Database Management Tools:
- MySQL Workbench: A visual database design tool that integrates SQL development, administration, database design, creation, and maintenance into a single environment for the MySQL database system.
- pgAdmin: The most popular and feature-rich open-source administration and development platform for PostgreSQL.
- MongoDB Compass: The GUI for MongoDB, allowing you to explore and manipulate your data visually.
- DBeaver: A free, open-source universal database tool that supports multiple database management systems.
These tools can significantly simplify database management tasks, from designing database schemas to optimizing queries and monitoring database performance.
14. Machine Learning and Data Science Tools
With the growing importance of machine learning and data science in software development, having tools specifically designed for these tasks is becoming increasingly important for many programmers.
Popular Machine Learning and Data Science Tools:
- Jupyter Notebook: An open-source web application that allows you to create and share documents that contain live code, equations, visualizations, and narrative text.
- TensorFlow: An open-source software library for machine learning and artificial intelligence.
- PyTorch: An open-source machine learning library based on the Torch library, used for applications such as computer vision and natural language processing.
- Scikit-learn: A free software machine learning library for Python, featuring various classification, regression, and clustering algorithms.
These tools provide powerful capabilities for data analysis, machine learning model development, and data visualization, essential for any programmer working in the fields of AI and data science.
15. Cloud Development Tools
As more applications move to the cloud, having tools that facilitate cloud development and deployment is becoming increasingly important for programmers.
Popular Cloud Development Tools:
- AWS CLI: The Amazon Web Services Command Line Interface, used for managing AWS services.
- Google Cloud SDK: A set of tools for managing resources and applications hosted on Google Cloud Platform.
- Azure CLI: A cross-platform command-line tool for managing Azure resources.
- Terraform: An open-source infrastructure as code software tool that enables you to safely and predictably create, change, and improve infrastructure.
These tools can greatly simplify the process of developing, deploying, and managing applications in cloud environments, which is becoming an increasingly important skill for modern programmers.
Conclusion
The tools mentioned in this article represent just a fraction of the vast ecosystem of programming tools available today. As a programmer, it’s important to continually explore and experiment with different tools to find the ones that best suit your needs and workflow. Remember that the most important tool is your knowledge and problem-solving ability – these tools are meant to enhance your capabilities, not replace them.
At AlgoCademy, we understand the importance of having the right tools and knowledge to succeed in programming. Our platform is designed to help you develop your coding skills, from basic concepts to advanced algorithms, preparing you for technical interviews at top tech companies. We provide interactive coding tutorials, AI-powered assistance, and step-by-step guidance to help you master the essential skills and tools needed in today’s competitive tech industry.
Whether you’re just starting your coding journey or preparing for technical interviews at FAANG companies, remember that the right combination of knowledge, practice, and tools is key to your success. Keep learning, keep practicing, and don’t be afraid to explore new tools and technologies. Happy coding!