Essential Programming Concepts for Tech Interviews
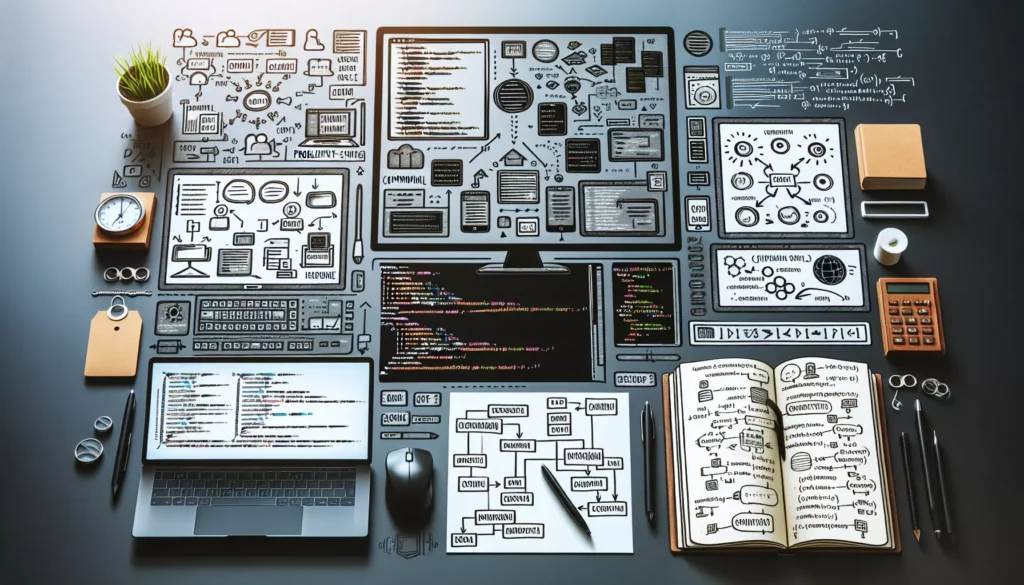
In today’s competitive tech landscape, mastering fundamental programming concepts is crucial for acing technical interviews, especially when aiming for positions at top companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Whether you’re a coding novice or looking to brush up on your skills, understanding these core principles can significantly boost your chances of success. In this comprehensive guide, we’ll explore the essential programming basics that interviewers often expect candidates to know.
1. Variables and Data Types
At the heart of programming lies the concept of variables and data types. Variables are containers for storing data, while data types define the kind of information a variable can hold.
Common Data Types:
- Integers: Whole numbers (e.g., 1, 42, -10)
- Floating-point numbers: Decimal numbers (e.g., 3.14, -0.5)
- Strings: Text (e.g., “Hello, World!”)
- Booleans: True or False values
- Arrays/Lists: Collections of items
Understanding how to declare variables, assign values, and work with different data types is fundamental. Here’s a simple example in Python:
# Variable declaration and assignment
age = 25
name = "Alice"
is_student = True
grades = [85, 90, 78, 92]
# Printing variables
print(f"{name} is {age} years old.")
print(f"Student status: {is_student}")
print(f"Grades: {grades}")
Mastering variables and data types is essential, as they form the building blocks of more complex programming concepts. Platforms like AlgoCademy offer interactive tutorials that help reinforce these fundamentals through hands-on coding exercises.
2. Control Structures
Control structures are the backbone of programming logic, allowing you to control the flow of your code. The three main types of control structures are:
a. Conditional Statements
Conditional statements allow your program to make decisions based on certain conditions. The most common forms are if, else if, and else statements.
# Example of conditional statements in Python
score = 85
if score >= 90:
print("A grade")
elif score >= 80:
print("B grade")
elif score >= 70:
print("C grade")
else:
print("Need improvement")
b. Loops
Loops allow you to repeat a block of code multiple times. The two primary types of loops are for loops and while loops.
# For loop example
for i in range(5):
print(f"Iteration {i + 1}")
# While loop example
count = 0
while count < 5:
print(f"Count is {count}")
count += 1
c. Switch Statements
While not available in all languages (like Python), switch statements provide an alternative to long if-else chains for multiple condition checking.
// Switch statement example in JavaScript
let day = 3;
switch (day) {
case 1:
console.log("Monday");
break;
case 2:
console.log("Tuesday");
break;
case 3:
console.log("Wednesday");
break;
default:
console.log("Another day");
}
AlgoCademy’s interactive platform offers a variety of exercises to practice these control structures, helping you build a solid foundation in programming logic.
3. Functions and Methods
Functions (also known as methods in object-oriented programming) are reusable blocks of code that perform specific tasks. They help in organizing code, promoting reusability, and breaking down complex problems into smaller, manageable parts.
# Function definition in Python
def greet(name):
return f"Hello, {name}!"
# Function call
message = greet("Alice")
print(message) # Output: Hello, Alice!
# Function with multiple parameters
def calculate_area(length, width):
return length * width
area = calculate_area(5, 3)
print(f"The area is: {area}") # Output: The area is: 15
Understanding how to define functions, pass parameters, and return values is crucial for writing efficient and maintainable code. AlgoCademy’s curriculum includes in-depth tutorials on functions, helping you master this essential concept through practical examples and coding challenges.
4. Data Structures
Data structures are ways of organizing and storing data for efficient access and modification. Knowing when and how to use different data structures is crucial for solving complex programming problems and optimizing code performance.
Common Data Structures:
- Arrays/Lists
- Linked Lists
- Stacks
- Queues
- Hash Tables (Dictionaries)
- Trees
- Graphs
Let’s look at examples of some basic data structures:
# List (Array) in Python
fruits = ["apple", "banana", "cherry"]
print(fruits[1]) # Output: banana
# Dictionary (Hash Table) in Python
person = {
"name": "John",
"age": 30,
"city": "New York"
}
print(person["name"]) # Output: John
# Stack implementation using a list
stack = []
stack.append("a")
stack.append("b")
stack.append("c")
print(stack.pop()) # Output: c
AlgoCademy offers comprehensive tutorials on various data structures, providing clear explanations and practical exercises to help you understand their implementations and use cases.
5. Object-Oriented Programming (OOP)
Object-Oriented Programming is a programming paradigm based on the concept of “objects,” which can contain data and code. The main principles of OOP are:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Here’s a basic example of a class in Python demonstrating some OOP concepts:
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
pass
class Dog(Animal):
def speak(self):
return f"{self.name} says Woof!"
class Cat(Animal):
def speak(self):
return f"{self.name} says Meow!"
# Creating objects
dog = Dog("Buddy")
cat = Cat("Whiskers")
print(dog.speak()) # Output: Buddy says Woof!
print(cat.speak()) # Output: Whiskers says Meow!
Understanding OOP principles is crucial for designing scalable and maintainable software systems. AlgoCademy’s curriculum includes detailed lessons on OOP, helping you grasp these concepts through interactive coding exercises and real-world examples.
6. Algorithms and Problem-Solving
Algorithms are step-by-step procedures for solving problems or performing tasks. Developing strong problem-solving skills and understanding common algorithms is essential for succeeding in technical interviews.
Key Algorithmic Concepts:
- Searching (e.g., Binary Search)
- Sorting (e.g., Quick Sort, Merge Sort)
- Recursion
- Dynamic Programming
- Graph Algorithms
Here’s an example of a simple binary search algorithm:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15]
result = binary_search(sorted_array, 7)
print(f"Index of 7: {result}") # Output: Index of 7: 3
AlgoCademy excels in teaching algorithms and problem-solving techniques. Their platform offers a wide range of algorithmic challenges, from basic to advanced levels, helping you build the skills necessary to tackle complex coding problems in interviews.
7. Time and Space Complexity
Understanding time and space complexity is crucial for writing efficient code and optimizing algorithms. This knowledge is often tested in technical interviews, especially at top tech companies.
Key Concepts:
- Big O notation
- Time complexity analysis
- Space complexity analysis
- Trade-offs between time and space
For example, the time complexity of the binary search algorithm shown earlier is O(log n), which is much more efficient than a linear search O(n) for large datasets.
AlgoCademy’s curriculum includes in-depth coverage of time and space complexity, helping you analyze and optimize your code effectively. Their interactive exercises allow you to practice applying these concepts to real coding problems.
8. Error Handling and Debugging
Proper error handling and debugging skills are essential for writing robust and reliable code. Understanding how to anticipate, catch, and handle errors can significantly improve the quality of your programs.
# Example of error handling in Python
def divide(a, b):
try:
result = a / b
return result
except ZeroDivisionError:
return "Error: Division by zero"
except TypeError:
return "Error: Invalid input types"
print(divide(10, 2)) # Output: 5.0
print(divide(10, 0)) # Output: Error: Division by zero
print(divide("10", 2)) # Output: Error: Invalid input types
AlgoCademy provides comprehensive tutorials on error handling and debugging techniques, equipping you with the skills to write more reliable code and efficiently troubleshoot issues.
9. Version Control with Git
While not strictly a programming concept, understanding version control systems, particularly Git, is crucial for modern software development. Many technical interviews may include questions about Git or require you to use it during coding challenges.
Key Git Concepts:
- Repositories
- Commits
- Branches
- Merging
- Pull requests
AlgoCademy integrates Git concepts into its curriculum, helping you understand how to use version control effectively in your coding projects.
10. Testing and Test-Driven Development (TDD)
Writing tests for your code is a crucial skill in professional software development. Understanding testing methodologies and practicing test-driven development can set you apart in technical interviews.
# Example of a simple unit test in Python using unittest
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add(-1, -1), -2)
def test_add_mixed_numbers(self):
self.assertEqual(add(-1, 1), 0)
if __name__ == '__main__':
unittest.main()
AlgoCademy’s platform includes lessons on testing methodologies and provides opportunities to practice writing tests for your code, helping you develop this essential skill.
Conclusion: Mastering Programming Basics with AlgoCademy
Understanding these fundamental programming concepts is crucial for succeeding in technical interviews, especially when aiming for positions at top tech companies. However, truly mastering these concepts requires more than just reading about them – it demands hands-on practice and guided learning.
This is where AlgoCademy shines. As a comprehensive coding education platform, AlgoCademy offers:
- Interactive coding tutorials that cover all the concepts discussed in this article and more
- AI-powered assistance to help you learn at your own pace
- Step-by-step guidance through complex programming problems
- A focus on algorithmic thinking and problem-solving skills
- Preparation specifically tailored for technical interviews at major tech companies
By leveraging AlgoCademy’s resources, you can progress from beginner-level coding to confidently tackling technical interviews. The platform’s emphasis on practical coding skills, combined with its comprehensive coverage of fundamental concepts, makes it an invaluable tool for aspiring programmers and experienced developers alike.
Remember, the key to success in programming interviews is not just knowing the concepts, but being able to apply them effectively to solve real-world problems. With AlgoCademy, you’ll have the opportunity to practice these skills in a supportive, interactive environment, preparing you for success in your coding journey and future technical interviews.
Start your journey with AlgoCademy today and take the first step towards mastering the essential programming concepts that will set you apart in technical interviews and beyond!