Essential Math Skills for Programming: Mastering the Foundations
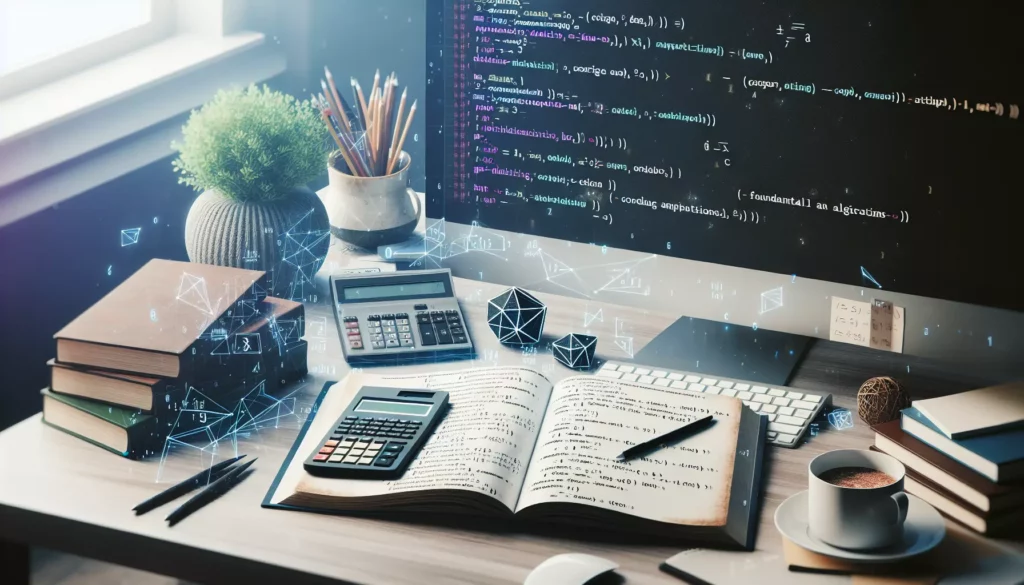
In the world of programming, mathematics plays a crucial role in shaping the way we think about and solve complex problems. Whether you’re a beginner just starting your coding journey or an experienced developer looking to enhance your skills, understanding the essential math concepts can significantly boost your programming prowess. In this comprehensive guide, we’ll explore the fundamental math skills needed for programming and how they apply to various aspects of software development.
1. Arithmetic and Basic Operations
At the core of programming lies the ability to perform basic arithmetic operations. While it may seem elementary, a solid grasp of addition, subtraction, multiplication, and division is essential for writing efficient code.
Why It’s Important:
- Calculating values and manipulating data
- Implementing algorithms that require numerical computations
- Optimizing code performance through efficient calculations
Programming Application:
Consider a simple example of calculating the average of a list of numbers:
def calculate_average(numbers):
total = sum(numbers)
count = len(numbers)
average = total / count
return average
scores = [85, 92, 78, 90, 88]
avg_score = calculate_average(scores)
print(f"The average score is: {avg_score}")
In this example, we use addition (sum), division, and the concept of counting (len) to calculate the average score.
2. Algebra and Variables
Algebra forms the backbone of programming logic. Understanding variables, equations, and algebraic expressions is crucial for writing dynamic and flexible code.
Why It’s Important:
- Representing and manipulating data through variables
- Solving equations and implementing mathematical models
- Creating reusable and modular code
Programming Application:
Let’s look at a simple algebraic equation implemented in code:
def quadratic_equation(a, b, c, x):
return a * x**2 + b * x + c
result = quadratic_equation(2, 3, -5, 4)
print(f"The result of the quadratic equation is: {result}")
This function represents the quadratic equation ax² + bx + c, where we can input different values for a, b, c, and x to calculate the result.
3. Logic and Boolean Algebra
Boolean algebra and logical operations are fundamental to programming, as they form the basis of decision-making in code.
Why It’s Important:
- Implementing conditional statements and control flow
- Evaluating complex conditions and making decisions
- Optimizing algorithms through logical simplification
Programming Application:
Here’s an example of using boolean logic in a simple login system:
def check_login(username, password):
correct_username = "user123"
correct_password = "securepass"
is_username_correct = (username == correct_username)
is_password_correct = (password == correct_password)
return is_username_correct and is_password_correct
login_successful = check_login("user123", "securepass")
print(f"Login successful: {login_successful}")
In this example, we use boolean comparisons and the logical AND operator to determine if the login credentials are correct.
4. Functions and Graphing
Understanding functions and their graphical representations is crucial for modeling relationships between variables and implementing complex algorithms.
Why It’s Important:
- Visualizing data and understanding relationships
- Implementing mathematical models in code
- Analyzing algorithm performance and complexity
Programming Application:
Here’s an example of plotting a simple function using Python’s matplotlib library:
import matplotlib.pyplot as plt
import numpy as np
def plot_function(func, x_range):
x = np.linspace(x_range[0], x_range[1], 100)
y = func(x)
plt.plot(x, y)
plt.title(f"Graph of {func.__name__}")
plt.xlabel("x")
plt.ylabel("y")
plt.grid(True)
plt.show()
def quadratic(x):
return x**2 - 4*x + 4
plot_function(quadratic, [-2, 6])
This code defines a function to plot any given function and then uses it to visualize a quadratic equation.
5. Statistics and Probability
Statistics and probability are essential for data analysis, machine learning, and making informed decisions based on data.
Why It’s Important:
- Analyzing and interpreting data
- Implementing statistical algorithms and models
- Making predictions and estimations in various applications
Programming Application:
Here’s a simple example of calculating basic statistics:
import statistics
data = [2, 4, 4, 4, 5, 5, 7, 9]
mean = statistics.mean(data)
median = statistics.median(data)
mode = statistics.mode(data)
std_dev = statistics.stdev(data)
print(f"Mean: {mean}")
print(f"Median: {median}")
print(f"Mode: {mode}")
print(f"Standard Deviation: {std_dev}")
This code calculates basic statistical measures like mean, median, mode, and standard deviation for a given dataset.
6. Linear Algebra
Linear algebra is fundamental in many areas of computer science, including computer graphics, machine learning, and optimization algorithms.
Why It’s Important:
- Representing and manipulating multidimensional data
- Solving systems of linear equations
- Implementing algorithms for data transformation and analysis
Programming Application:
Here’s an example of matrix multiplication using NumPy:
import numpy as np
# Define two matrices
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
# Perform matrix multiplication
C = np.dot(A, B)
print("Matrix A:")
print(A)
print("\nMatrix B:")
print(B)
print("\nResult of A * B:")
print(C)
This code demonstrates how to perform matrix multiplication, a fundamental operation in linear algebra, using NumPy.
7. Discrete Mathematics
Discrete mathematics is crucial for understanding the theoretical foundations of computer science and is essential for areas like algorithm design and cryptography.
Why It’s Important:
- Understanding set theory and its applications in programming
- Analyzing algorithm complexity and efficiency
- Implementing graph algorithms and data structures
Programming Application:
Here’s an example of implementing a simple set operation:
def symmetric_difference(set1, set2):
return (set1 - set2) | (set2 - set1)
A = {1, 2, 3, 4, 5}
B = {4, 5, 6, 7, 8}
result = symmetric_difference(A, B)
print(f"Symmetric difference of A and B: {result}")
This code implements the symmetric difference operation, which is a concept from set theory in discrete mathematics.
8. Calculus
While not always directly applied in day-to-day programming, calculus concepts are crucial for understanding advanced algorithms, particularly in fields like machine learning and computer graphics.
Why It’s Important:
- Optimizing functions and finding minimum/maximum values
- Understanding rates of change and their applications
- Implementing numerical methods for solving complex problems
Programming Application:
Here’s a simple example of numerical differentiation:
def numerical_derivative(func, x, h=1e-5):
return (func(x + h) - func(x)) / h
def f(x):
return x**2
x = 3
derivative = numerical_derivative(f, x)
print(f"The approximate derivative of f(x) = x^2 at x = {x} is: {derivative}")
This code implements a basic numerical method to approximate the derivative of a function, a fundamental concept in calculus.
9. Number Theory
Number theory is essential for cryptography, random number generation, and solving various algorithmic problems.
Why It’s Important:
- Implementing encryption algorithms and secure communication protocols
- Solving problems related to divisibility and prime numbers
- Optimizing algorithms that deal with integer arithmetic
Programming Application:
Here’s an example of implementing the Sieve of Eratosthenes algorithm to find prime numbers:
def sieve_of_eratosthenes(n):
primes = [True] * (n + 1)
primes[0] = primes[1] = False
for i in range(2, int(n**0.5) + 1):
if primes[i]:
for j in range(i*i, n+1, i):
primes[j] = False
return [i for i in range(2, n+1) if primes[i]]
limit = 50
prime_numbers = sieve_of_eratosthenes(limit)
print(f"Prime numbers up to {limit}: {prime_numbers}")
This algorithm efficiently finds all prime numbers up to a given limit, showcasing the application of number theory concepts in programming.
10. Geometry and Trigonometry
Geometry and trigonometry are crucial for computer graphics, game development, and various scientific simulations.
Why It’s Important:
- Implementing 2D and 3D graphics algorithms
- Calculating distances, angles, and positions in space
- Solving problems related to spatial relationships and transformations
Programming Application:
Here’s an example of calculating the distance between two points in 2D space:
import math
def distance_between_points(x1, y1, x2, y2):
return math.sqrt((x2 - x1)**2 + (y2 - y1)**2)
point1 = (0, 0)
point2 = (3, 4)
dist = distance_between_points(*point1, *point2)
print(f"The distance between {point1} and {point2} is: {dist}")
This code uses the Pythagorean theorem to calculate the distance between two points, demonstrating the application of geometry in programming.
Conclusion: Building a Strong Mathematical Foundation for Programming
While it’s true that not every programming task requires advanced mathematical knowledge, having a solid foundation in these essential math skills can significantly enhance your ability to solve complex problems, optimize algorithms, and understand the underlying principles of computer science.
As you progress in your programming journey, you’ll find that different areas of software development may require varying levels of mathematical proficiency. For instance:
- Web development often relies more on logic and basic arithmetic
- Game development frequently uses geometry, trigonometry, and linear algebra
- Data science and machine learning heavily utilize statistics, linear algebra, and calculus
- Cryptography and security rely on number theory and discrete mathematics
The key is to build a strong foundation in these core mathematical concepts and then deepen your knowledge in specific areas as needed for your particular field of interest or project requirements.
Remember, mathematics in programming is not just about performing calculations; it’s about developing a logical and analytical mindset that allows you to break down complex problems into manageable parts. This problem-solving approach is invaluable in all aspects of programming, from designing efficient algorithms to architecting scalable software systems.
As you continue to learn and grow as a programmer, don’t shy away from mathematical concepts. Embrace them as powerful tools that can enhance your coding skills and open up new possibilities in your software development career. With practice and persistence, you’ll find that the intersection of mathematics and programming can lead to innovative solutions and a deeper understanding of the technology that powers our digital world.
Keep coding, keep learning, and let mathematics be your ally in the exciting journey of programming!