Epic Games Interview Questions: A Comprehensive Guide for Aspiring Game Developers
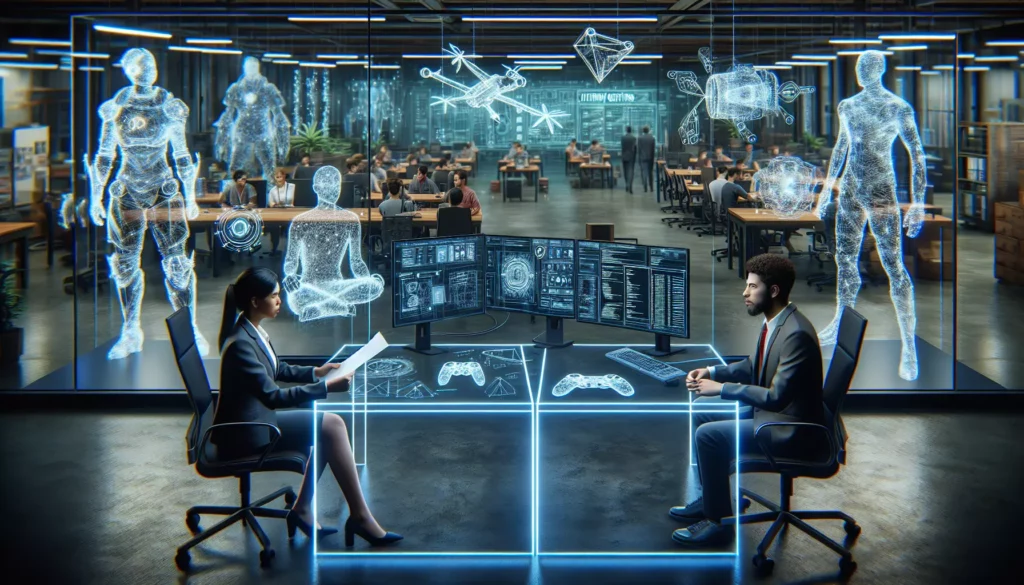
Are you dreaming of joining the team behind Fortnite, Unreal Engine, and other groundbreaking games and technologies? Landing a job at Epic Games is a goal for many aspiring game developers and programmers. To help you prepare for this exciting opportunity, we’ve compiled a comprehensive guide to Epic Games interview questions. This article will cover various aspects of the interview process, including technical questions, behavioral interviews, and tips to help you stand out from the competition.
Table of Contents
- Introduction to Epic Games Interviews
- Technical Interview Questions
- Coding Challenges and Problem-Solving
- Game Development-Specific Questions
- Behavioral Interview Questions
- Unreal Engine-Related Questions
- System Design and Architecture
- Graphics Programming Questions
- Networking and Multiplayer
- Performance Optimization
- Tools and Technologies
- Tips for Success in Epic Games Interviews
- Conclusion
1. Introduction to Epic Games Interviews
Epic Games, founded in 1991, has become a powerhouse in the gaming industry, known for its innovative games and cutting-edge technology. The company’s interview process is designed to identify candidates who are not only technically proficient but also passionate about game development and capable of thriving in a fast-paced, creative environment.
The interview process at Epic Games typically consists of several stages:
- Initial screening or phone interview
- Technical assessment or coding challenge
- On-site interviews (or virtual interviews, depending on circumstances)
- Final round with team leads or management
Throughout these stages, you’ll be evaluated on your technical skills, problem-solving abilities, creativity, and cultural fit. Let’s dive into the types of questions you might encounter during this process.
2. Technical Interview Questions
Technical questions form the core of Epic Games interviews, especially for engineering and programming roles. These questions assess your fundamental knowledge of computer science concepts and programming languages. Here are some examples of technical questions you might face:
C++ Questions
- Explain the difference between stack and heap memory allocation.
- What are virtual functions in C++, and how do they work?
- Describe the concept of move semantics in C++11 and its benefits.
- How does multiple inheritance work in C++, and what are its potential pitfalls?
- Explain the rule of three (or rule of five) in C++.
Data Structures and Algorithms
- Implement a function to reverse a linked list.
- Explain the time and space complexity of quicksort. How can it be optimized?
- Describe how a hash table works and discuss collision resolution techniques.
- Implement a binary search tree and write functions for insertion and deletion.
- Explain the concept of dynamic programming and provide an example of its application.
Sample C++ Code Question
Here’s an example of a C++ coding question you might encounter:
// Implement a function to find the longest palindromic substring in a given string
class Solution {
public:
string longestPalindrome(string s) {
// Your implementation here
}
};
A possible solution to this problem could be:
class Solution {
public:
string longestPalindrome(string s) {
if (s.empty()) return "";
int start = 0, max_length = 1;
int n = s.length();
vector<vector<bool>> dp(n, vector<bool>(n, false));
// All substrings of length 1 are palindromes
for (int i = 0; i < n; i++) {
dp[i][i] = true;
}
// Check for substrings of length 2
for (int i = 0; i < n - 1; i++) {
if (s[i] == s[i+1]) {
dp[i][i+1] = true;
start = i;
max_length = 2;
}
}
// Check for lengths greater than 2
for (int len = 3; len <= n; len++) {
for (int i = 0; i < n - len + 1; i++) {
int j = i + len - 1;
if (s[i] == s[j] && dp[i+1][j-1]) {
dp[i][j] = true;
if (len > max_length) {
start = i;
max_length = len;
}
}
}
}
return s.substr(start, max_length);
}
};
This solution uses dynamic programming to efficiently find the longest palindromic substring in O(n^2) time complexity and O(n^2) space complexity.
3. Coding Challenges and Problem-Solving
In addition to technical questions, you may be asked to complete coding challenges or solve complex problems during your Epic Games interview. These challenges are designed to assess your problem-solving skills, coding ability, and approach to tackling difficult tasks.
Types of Coding Challenges
- Algorithm Implementation: You might be asked to implement a specific algorithm, such as pathfinding (A* or Dijkstra’s algorithm) or sorting algorithms.
- Data Structure Design: Design and implement a custom data structure to solve a particular problem efficiently.
- Game Mechanics: Implement a simple game mechanic or physics simulation.
- Optimization Problems: Optimize an existing piece of code or algorithm for better performance.
- Debugging: Identify and fix bugs in a given piece of code.
Sample Problem-Solving Question
Here’s an example of a problem-solving question you might encounter:
Design a system for a multiplayer game that can efficiently handle collision detection between thousands of moving objects in real-time. Consider performance, scalability, and network limitations.
When approaching this problem, consider the following aspects:
- Spatial partitioning techniques (e.g., quadtrees, octrees, or grid-based systems)
- Broad-phase and narrow-phase collision detection
- Parallelization and multi-threading
- Network synchronization and prediction
- Optimization techniques like sweep and prune or bounding volume hierarchies
Be prepared to discuss your approach, explain your reasoning, and consider trade-offs between different solutions.
4. Game Development-Specific Questions
Epic Games is, of course, primarily focused on game development. You should expect questions that relate directly to game development concepts and practices. Here are some examples:
- Explain the game loop and its importance in game development.
- Describe the components of a basic entity-component system (ECS) and its benefits in game architecture.
- How would you implement a basic particle system for visual effects?
- Discuss different techniques for game AI, such as behavior trees, state machines, or goal-oriented action planning.
- Explain the concept of delta time and why it’s crucial in game programming.
- How would you approach optimizing the rendering pipeline for a large open-world game?
- Describe the process of implementing a save/load system for a complex game with multiple levels and persistent player data.
Sample Game Development Code Question
Here’s an example of a game development-related coding question:
// Implement a simple 2D vector class with basic operations
class Vector2D {
public:
// Constructor
Vector2D(float x, float y) : x(x), y(y) {}
// Add two vectors
Vector2D operator+(const Vector2D& other) const {
// Your implementation here
}
// Subtract two vectors
Vector2D operator-(const Vector2D& other) const {
// Your implementation here
}
// Scalar multiplication
Vector2D operator*(float scalar) const {
// Your implementation here
}
// Dot product
float dot(const Vector2D& other) const {
// Your implementation here
}
// Magnitude (length) of the vector
float magnitude() const {
// Your implementation here
}
// Normalize the vector
Vector2D normalize() const {
// Your implementation here
}
private:
float x, y;
};
Implement the missing functions in this Vector2D class, which could be used in a 2D game engine for various calculations and operations.
5. Behavioral Interview Questions
In addition to technical skills, Epic Games values candidates who can work well in teams, communicate effectively, and adapt to changing environments. You may encounter behavioral questions that assess these qualities. Some examples include:
- Describe a challenging project you worked on and how you overcame obstacles.
- Tell me about a time when you had to meet a tight deadline. How did you manage your time and resources?
- Give an example of how you’ve worked effectively in a team environment.
- How do you stay updated with the latest trends and technologies in game development?
- Describe a situation where you had to explain a complex technical concept to a non-technical person.
- Tell me about a time when you received critical feedback. How did you respond and what did you learn?
- How do you approach learning new technologies or programming languages?
When answering behavioral questions, use the STAR method (Situation, Task, Action, Result) to structure your responses and provide concrete examples from your past experiences.
6. Unreal Engine-Related Questions
Given Epic Games’ development and maintenance of Unreal Engine, you should be prepared for questions specific to this game engine, especially if you’re applying for a role that involves working with Unreal Engine. Here are some potential questions:
- Explain the difference between Blueprints and C++ in Unreal Engine. When would you use one over the other?
- Describe the role of the Gameplay Ability System in Unreal Engine and its benefits.
- How does Unreal Engine handle memory management, and what are some best practices for optimizing memory usage?
- Explain the concept of actor replication in Unreal Engine’s networking system.
- Describe the rendering pipeline in Unreal Engine and how it differs from other game engines.
- How would you optimize performance for an open-world game built in Unreal Engine?
- Explain the purpose and functionality of Unreal Engine’s behavior tree system.
Sample Unreal Engine Code Question
Here’s an example of an Unreal Engine-specific coding question:
// Implement a custom Actor class in Unreal Engine that represents a collectible item
UCLASS()
class MYGAME_API ACollectibleItem : public AActor
{
GENERATED_BODY()
public:
ACollectibleItem();
UPROPERTY(EditAnywhere, BlueprintReadWrite, Category = "Collectible")
int32 PointValue;
UFUNCTION(BlueprintCallable, Category = "Collectible")
void Collect(APlayerController* Collector);
protected:
virtual void BeginPlay() override;
private:
UPROPERTY(VisibleAnywhere, Category = "Components")
UStaticMeshComponent* MeshComponent;
UPROPERTY(VisibleAnywhere, Category = "Components")
USphereComponent* CollisionComponent;
// Implement the Collect function
void Collect_Implementation(APlayerController* Collector);
};
In this question, you would need to implement the Collect_Implementation
function to handle the logic when a player collects the item, such as adding points to the player’s score, playing a sound effect, and destroying the actor.
7. System Design and Architecture
For more senior positions or roles involving game architecture, you may be asked system design questions. These questions assess your ability to design scalable, efficient, and maintainable game systems. Some examples include:
- Design a inventory system for an MMORPG that can handle millions of concurrent players.
- Architect a quest system for an open-world game that supports branching storylines and player choices.
- Design a matchmaking system for a competitive multiplayer game that considers player skill, latency, and team balance.
- Create a data-driven ability system that allows designers to easily create and modify character abilities without programming.
- Design a save system for a large open-world game that can efficiently save and load player progress, world state, and quest information.
When answering system design questions, focus on:
- Scalability and performance considerations
- Data structures and algorithms used
- Trade-offs between different approaches
- Extensibility and maintainability of the system
- Integration with other game systems
8. Graphics Programming Questions
Epic Games is known for its cutting-edge graphics technology, so you may encounter questions related to computer graphics, especially if you’re applying for a graphics programming role. Some potential topics include:
- Explain the concept of deferred rendering and its advantages over forward rendering.
- Describe the process of implementing dynamic global illumination in a game engine.
- How would you implement a basic shader for cel-shaded (toon) rendering?
- Explain the concept of tessellation in modern graphics pipelines and its benefits.
- Describe the process of implementing screen-space ambient occlusion (SSAO).
- How would you optimize the rendering of a large number of dynamic lights in a scene?
- Explain the concept of physically-based rendering (PBR) and its importance in modern game graphics.
Sample Graphics Programming Question
Here’s an example of a graphics programming question you might encounter:
// Implement a basic vertex shader and fragment shader for a simple textured 3D object
// Vertex Shader
#version 330 core
layout (location = 0) in vec3 aPos;
layout (location = 1) in vec2 aTexCoord;
out vec2 TexCoord;
uniform mat4 model;
uniform mat4 view;
uniform mat4 projection;
void main()
{
// Your implementation here
}
// Fragment Shader
#version 330 core
out vec4 FragColor;
in vec2 TexCoord;
uniform sampler2D texture1;
void main()
{
// Your implementation here
}
In this question, you would need to implement the vertex and fragment shaders to correctly render a textured 3D object, applying the model, view, and projection transformations in the vertex shader and sampling the texture in the fragment shader.
9. Networking and Multiplayer
Given the importance of multiplayer gaming in many of Epic’s titles, you should be prepared for questions related to networking and multiplayer game development. Some potential topics include:
- Explain the difference between authoritative and non-authoritative networking models in multiplayer games.
- Describe techniques for minimizing the impact of network latency in fast-paced multiplayer games.
- How would you implement a reliable UDP protocol for a multiplayer game?
- Explain the concept of client-side prediction and server reconciliation in networked games.
- Describe approaches to cheat prevention in multiplayer games.
- How would you design a scalable server architecture for a massively multiplayer online game?
- Explain the challenges and solutions for implementing cross-platform multiplayer functionality.
Sample Networking Question
Here’s an example of a networking-related question:
// Implement a basic client-side prediction system for player movement
class PlayerController {
public:
void Update(float deltaTime) {
// Apply local input and update position
// Your implementation here
// Send input to server
SendInputToServer();
// Receive server updates and reconcile if necessary
ReconcileWithServer();
}
private:
Vector3 position;
Vector3 velocity;
void SendInputToServer() {
// Your implementation here
}
void ReconcileWithServer() {
// Your implementation here
}
};
In this question, you would need to implement client-side prediction for smooth player movement, while also handling server reconciliation to correct any discrepancies between the client and server states.
10. Performance Optimization
Performance is crucial in game development, and Epic Games places a high value on optimization. You may be asked questions about various optimization techniques and strategies. Some examples include:
- Describe techniques for optimizing memory usage in a large open-world game.
- How would you profile and optimize CPU performance in a game engine?
- Explain the concept of level of detail (LOD) and how it’s used to optimize rendering performance.
- Describe strategies for optimizing asset loading and streaming in a large game world.
- How would you optimize physics calculations for a game with many dynamic objects?
- Explain techniques for reducing draw calls in a complex 3D scene.
- Describe approaches to optimizing shaders for better GPU performance.
Sample Optimization Question
Here’s an example of an optimization-related question:
// Optimize the following function for better performance
void UpdateEntities(std::vector<Entity*>& entities, float deltaTime) {
for (int i = 0; i < entities.size(); i++) {
if (entities[i]->isActive()) {
entities[i]->update(deltaTime);
if (entities[i]->getHealth() <= 0) {
delete entities[i];
entities.erase(entities.begin() + i);
i--;
}
}
}
}
In this question, you would need to identify and implement optimizations such as:
- Using a more efficient container (e.g.,
std::vector<std::unique_ptr<Entity>>
) - Implementing the erase-remove idiom for more efficient removal
- Considering parallelization for the update loop
- Potentially using a object pool for entity management
11. Tools and Technologies
Epic Games uses a variety of tools and technologies in their development process. You may be asked about your experience with or knowledge of specific tools. Some areas to be familiar with include:
- Version control systems (e.g., Git, Perforce)
- Build systems and continuous integration/continuous deployment (CI/CD) pipelines
- Profiling and debugging tools
- 3D modeling and animation software (e.g., Maya, Blender)
- Asset management systems
- Scripting languages (e.g., Python, Lua)
- Cross-platform development considerations
Be prepared to discuss your experience with these tools and how you’ve used them in past projects.
12. Tips for Success in Epic Games Interviews
To increase your chances of success in an Epic Games interview, consider the following tips:
- Prepare thoroughly: Review computer science fundamentals, brush up on C++ and game development concepts, and practice coding problems.
- Showcase your passion: Demonstrate your enthusiasm for game development and your knowledge of Epic Games’ products and technologies.
- Highlight relevant projects: Be ready to discuss personal or professional projects that showcase your skills and creativity in game development.
- Practice problem-solving out loud: Get comfortable explaining your thought process as you work through problems.
- Ask thoughtful questions: Prepare questions about the role, team, and company to show your genuine interest.
- Stay updated: Keep up with the latest trends and advancements in game development and Epic Games’ technologies.
- Be honest about your knowledge: If you’re unsure about something, admit it and explain how you would go about finding the answer.
- Show adaptability: Emphasize your ability to learn quickly and adapt to new technologies and challenges.
13. Conclusion
Preparing for an interview at Epic Games can be challenging, but with the right preparation and mindset, you can increase your chances of success. Remember that the interview process is not just about showcasing your technical skills, but also demonstrating your passion for game development, your ability to work in a team, and your potential to contribute to Epic Games’ innovative projects.
By familiarizing yourself with the types of questions and topics covered in this guide, practicing your problem-solving skills, and staying up-to-date with the latest in game development technology, you’ll be well-equipped to tackle your Epic Games interview with confidence.
Good luck with your interview, and may your journey in game development be as epic as the company you’re aiming to join!