Embracing Feedback: Turning Criticism into Growth Opportunities in Coding Education
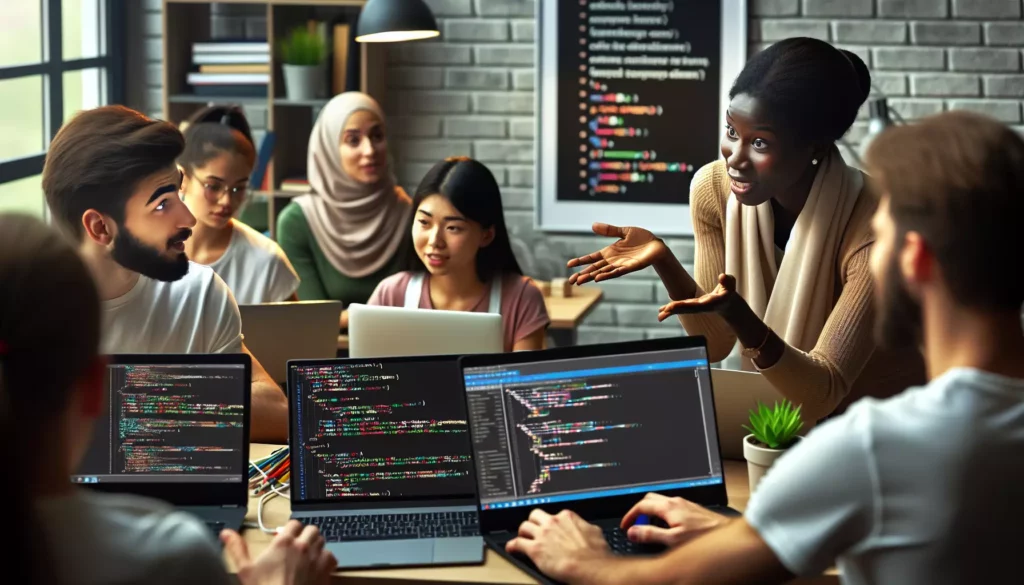
In the world of coding education and programming skills development, feedback is an essential component of growth. Whether you’re a beginner just starting your journey or an experienced developer preparing for technical interviews at major tech companies, the ability to embrace feedback and turn criticism into opportunities for improvement is crucial. This article will explore the importance of feedback in coding education, strategies for receiving and processing criticism effectively, and how platforms like AlgoCademy can help you leverage feedback to enhance your programming skills.
The Importance of Feedback in Coding Education
Feedback plays a vital role in the learning process, especially in a field as complex and ever-evolving as programming. Here’s why feedback is crucial in coding education:
- Identifying blind spots: Feedback helps learners recognize areas of improvement that they might not have noticed on their own.
- Reinforcing good practices: Positive feedback reinforces effective coding techniques and encourages learners to continue using them.
- Correcting misconceptions: Constructive criticism can help address misunderstandings about programming concepts or best practices.
- Accelerating learning: Timely feedback allows learners to make adjustments quickly, speeding up the learning process.
- Preparing for real-world scenarios: Feedback in educational settings mimics the code review process in professional environments, preparing learners for future careers.
Types of Feedback in Coding Education
In the context of coding education, feedback can come in various forms:
- Automated feedback: Generated by coding platforms or integrated development environments (IDEs) that check for syntax errors, code style violations, or performance issues.
- Peer feedback: Provided by fellow learners through code reviews or pair programming sessions.
- Instructor feedback: Given by teachers, mentors, or tutors who review code and provide personalized guidance.
- AI-powered feedback: Offered by advanced platforms like AlgoCademy, which use artificial intelligence to provide detailed, context-aware suggestions and explanations.
- Self-assessment: Reflection on one’s own code and problem-solving approaches.
Strategies for Receiving and Processing Criticism Effectively
Receiving criticism can be challenging, but it’s an essential skill for growth in the programming field. Here are some strategies to help you embrace feedback and turn it into opportunities for improvement:
1. Cultivate a Growth Mindset
Adopting a growth mindset is crucial when dealing with feedback. This means viewing challenges and criticism as opportunities to learn and improve, rather than as threats to your abilities or self-worth. Remember that even the most experienced programmers continue to learn and grow throughout their careers.
2. Separate Your Identity from Your Code
It’s important to recognize that criticism of your code is not a criticism of you as a person. Your code is a product of your current knowledge and skills, which are constantly evolving. By separating your identity from your work, you can approach feedback more objectively and use it as a tool for improvement.
3. Listen Actively and Ask Questions
When receiving feedback, listen actively and try to understand the perspective of the person providing the criticism. Ask clarifying questions to ensure you fully grasp the feedback and its implications. This approach demonstrates your willingness to learn and can lead to more detailed and helpful insights.
4. Look for Patterns in Feedback
Pay attention to recurring themes in the feedback you receive. If multiple sources point out similar issues, it’s likely an area that requires focused attention and improvement. Identifying these patterns can help you prioritize your learning efforts.
5. Practice Gratitude
Express appreciation for the time and effort others put into providing feedback. Gratitude not only fosters positive relationships but also encourages others to continue offering valuable insights in the future.
6. Reflect and Plan
After receiving feedback, take time to reflect on the information and plan your next steps. Consider how you can incorporate the suggestions into your coding practice and set specific goals for improvement.
Leveraging AlgoCademy for Feedback-Driven Learning
Platforms like AlgoCademy are designed to provide a comprehensive learning experience that incorporates various forms of feedback. Here’s how you can use AlgoCademy to maximize your learning through feedback:
1. Utilize AI-Powered Assistance
AlgoCademy’s AI-powered assistance provides instant, contextual feedback as you work through coding challenges. This feature can help you identify errors, suggest optimizations, and explain complex concepts in real-time. To make the most of this feature:
- Pay close attention to the AI’s suggestions and explanations.
- Try to understand the reasoning behind each recommendation.
- Experiment with implementing the suggested changes to see how they affect your code.
2. Engage with Step-by-Step Guidance
The platform’s step-by-step guidance feature breaks down complex problems into manageable parts. This structured approach provides built-in feedback at each stage of the problem-solving process. To benefit from this feature:
- Complete each step thoroughly before moving on to the next.
- Reflect on what you’ve learned after each step.
- If you’re struggling, use the hints provided but try to understand why they’re helpful rather than simply copying the solution.
3. Participate in Code Reviews
Many coding education platforms, including AlgoCademy, offer opportunities for peer code reviews. This feature allows you to both give and receive feedback from other learners. To make the most of code reviews:
- Submit your code for review regularly, even if you think it’s not perfect.
- Provide thoughtful feedback on others’ code, which can reinforce your own understanding.
- Approach reviews with an open mind and be prepared to defend your choices or admit when you’ve made a mistake.
4. Track Your Progress
Use AlgoCademy’s progress tracking features to monitor your improvement over time. This self-assessment tool provides valuable feedback on your overall development. To leverage this feature effectively:
- Regularly review your progress metrics to identify areas of strength and weakness.
- Set specific goals based on your progress data.
- Celebrate your achievements and use setbacks as motivation to push harder.
5. Engage with the Community
Participate in AlgoCademy’s community forums or discussion boards. These platforms often provide a wealth of informal feedback and insights from peers and more experienced programmers. To benefit from community engagement:
- Ask questions when you’re stuck or need clarification.
- Share your own solutions and be open to suggestions for improvement.
- Engage in discussions about different approaches to solving problems.
Applying Feedback to Improve Algorithmic Thinking and Problem-Solving
Feedback is particularly crucial when developing algorithmic thinking and problem-solving skills, which are key focus areas of platforms like AlgoCademy. Here’s how you can apply feedback to enhance these critical skills:
1. Analyze Time and Space Complexity
When receiving feedback on your algorithms, pay close attention to comments about time and space complexity. Understanding and optimizing these aspects is crucial for technical interviews and real-world programming. Consider the following example:
// Original solution with O(n^2) time complexity
function findDuplicates(arr) {
let duplicates = [];
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] === arr[j] && !duplicates.includes(arr[i])) {
duplicates.push(arr[i]);
}
}
}
return duplicates;
}
// Optimized solution with O(n) time complexity
function findDuplicatesOptimized(arr) {
let seen = new Set();
let duplicates = new Set();
for (let num of arr) {
if (seen.has(num)) {
duplicates.add(num);
} else {
seen.add(num);
}
}
return Array.from(duplicates);
}
In this example, feedback might suggest using a Set to improve the time complexity from O(n^2) to O(n). By implementing this change, you not only solve the problem more efficiently but also demonstrate a deeper understanding of data structures and their applications.
2. Refactor for Readability and Maintainability
Feedback often addresses the readability and maintainability of your code. These aspects are crucial for working in team environments and for long-term project success. Consider this example of refactoring based on feedback:
// Original code
function calc(a,b,c) {
let x = a+b;
let y = x*c;
return y-a;
}
// Refactored code based on feedback
function calculateResult(baseValue, addend, multiplier) {
const sum = baseValue + addend;
const product = sum * multiplier;
return product - baseValue;
}
The refactored version improves readability through descriptive variable and function names, making the code’s purpose clearer and easier to maintain.
3. Implement Error Handling and Edge Cases
Feedback often highlights the importance of handling errors and edge cases, which is crucial for robust and reliable code. Here’s an example of implementing error handling based on feedback:
// Original function without error handling
function divide(a, b) {
return a / b;
}
// Improved function with error handling
function divideWithErrorHandling(dividend, divisor) {
if (typeof dividend !== 'number' || typeof divisor !== 'number') {
throw new TypeError('Both arguments must be numbers');
}
if (divisor === 0) {
throw new Error('Cannot divide by zero');
}
return dividend / divisor;
}
This improved version handles potential errors and edge cases, making the function more robust and reliable in various scenarios.
4. Optimize for Specific Use Cases
Feedback might suggest optimizing your code for specific use cases or constraints. This could involve choosing different data structures or algorithms based on the expected input or performance requirements. For example:
// Original general-purpose sorting function
function sortArray(arr) {
return arr.sort((a, b) => a - b);
}
// Optimized sorting for small arrays (<10 elements)
function sortSmallArray(arr) {
if (arr.length > 10) {
throw new Error('This function is optimized for arrays with 10 or fewer elements');
}
// Using insertion sort for small arrays
for (let i = 1; i < arr.length; i++) {
let current = arr[i];
let j = i - 1;
while (j >= 0 && arr[j] > current) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = current;
}
return arr;
}
In this case, feedback might suggest using insertion sort for small arrays due to its efficiency with limited data sets and good performance on nearly-sorted arrays.
Preparing for Technical Interviews with Feedback-Driven Learning
One of the key goals of platforms like AlgoCademy is to prepare learners for technical interviews, particularly for positions at major tech companies. Feedback plays a crucial role in this preparation process. Here’s how you can use feedback to enhance your interview readiness:
1. Mock Interviews and Code Reviews
Participate in mock interviews and code reviews that simulate real interview scenarios. The feedback from these sessions can help you:
- Identify areas where you need to improve your problem-solving approach
- Practice explaining your thought process clearly and concisely
- Get comfortable with the pressure of coding under time constraints
- Learn to ask clarifying questions and communicate effectively during the problem-solving process
2. Focus on Common Interview Topics
Use feedback to guide your focus on topics that frequently appear in technical interviews. For example, if you consistently receive feedback about struggling with graph algorithms, prioritize studying and practicing these types of problems. Common interview topics often include:
- Data Structures (Arrays, Linked Lists, Trees, Graphs, Hash Tables)
- Algorithms (Sorting, Searching, Dynamic Programming, Recursion)
- System Design
- Object-Oriented Programming
- Time and Space Complexity Analysis
3. Improve Your Problem-Solving Process
Use feedback to refine your problem-solving approach. A typical process might include:
- Understand the problem (ask clarifying questions)
- Identify the inputs and outputs
- Brainstorm possible approaches
- Choose an approach and explain your reasoning
- Write pseudocode
- Implement the solution
- Test and debug
- Analyze time and space complexity
- Discuss potential optimizations
Feedback can help you identify which steps you tend to rush through or neglect, allowing you to practice a more thorough and methodical approach.
4. Develop Your Communication Skills
Technical interviews often assess not just your coding skills, but also your ability to communicate complex ideas clearly. Use feedback to improve how you:
- Explain your thought process while solving problems
- Discuss trade-offs between different approaches
- Respond to hints or suggestions from the interviewer
- Ask meaningful questions about the problem or requirements
5. Build a Growth Narrative
Use the feedback you receive throughout your learning journey to build a narrative of growth and improvement. This can be valuable during behavioral interviews or when discussing your learning experiences. For example:
“When I first started learning about dynamic programming, I struggled with identifying subproblems. Through consistent practice and feedback from mentors on AlgoCademy, I developed a systematic approach to breaking down complex problems into smaller, manageable parts. This not only improved my skills in dynamic programming but also enhanced my overall problem-solving abilities.”
Conclusion: Embracing Feedback as a Lifelong Skill
In the rapidly evolving field of programming, the ability to embrace feedback and turn criticism into growth opportunities is not just a learning technique—it’s a lifelong skill that can significantly impact your career. By leveraging platforms like AlgoCademy and consistently applying the strategies discussed in this article, you can:
- Accelerate your learning process
- Develop robust problem-solving skills
- Prepare effectively for technical interviews
- Build resilience and adaptability in the face of challenges
- Foster a growth mindset that will serve you throughout your career
Remember that every piece of feedback, whether positive or critical, is an opportunity to refine your skills and deepen your understanding. By embracing feedback as an integral part of your coding education journey, you’re not just learning to code—you’re learning how to become a lifelong learner and a more effective problem solver.
As you continue your journey in coding education and prepare for technical interviews, maintain an open and receptive attitude towards feedback. Engage actively with the resources and community available through platforms like AlgoCademy, and don’t hesitate to seek out additional sources of feedback. With persistence, reflection, and a willingness to learn from every experience, you’ll be well-equipped to tackle the challenges of programming and thrive in your future career.