Embedded Linux Development: Creating Applications for Linux-Powered Devices
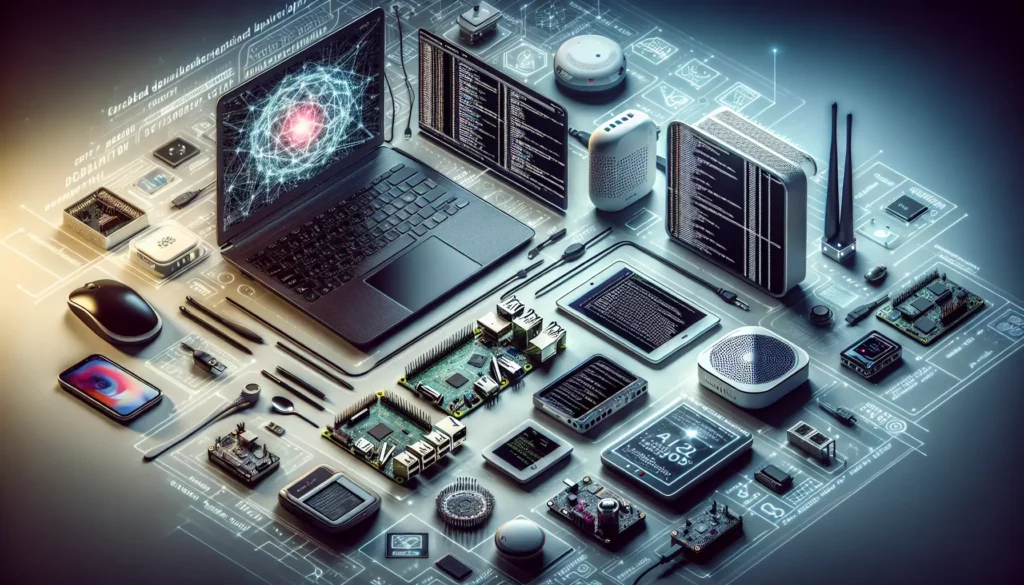
In today’s interconnected world, embedded systems are everywhere. From smart home devices to industrial automation systems, these specialized computer systems are designed to perform specific tasks with high efficiency and reliability. Among the various operating systems used in embedded devices, Linux stands out due to its flexibility, robustness, and open-source nature. This comprehensive guide will walk you through the fascinating world of embedded Linux development, equipping you with the knowledge and skills to create powerful applications for Linux-powered devices.
Table of Contents
- Introduction to Embedded Linux
- Advantages of Using Linux in Embedded Systems
- Getting Started with Embedded Linux Development
- Essential Tools for Embedded Linux Development
- Building an Embedded Linux System
- Embedded Linux Application Development
- Debugging Embedded Linux Applications
- Optimizing Embedded Linux Applications
- Real-World Examples of Embedded Linux Applications
- Challenges in Embedded Linux Development
- Future Trends in Embedded Linux
- Conclusion
1. Introduction to Embedded Linux
Embedded Linux refers to the use of the Linux operating system in embedded devices. These devices are typically designed for specific purposes and have limited resources compared to general-purpose computers. Embedded Linux combines the power and flexibility of the Linux kernel with custom-built root filesystems and applications tailored to the specific needs of the embedded device.
Some key characteristics of embedded Linux systems include:
- Resource constraints (limited memory, processing power, and storage)
- Real-time performance requirements
- Long-term stability and reliability
- Customizability and scalability
- Support for a wide range of hardware architectures
2. Advantages of Using Linux in Embedded Systems
Linux has become increasingly popular in the embedded systems world due to several compelling advantages:
- Open-source nature: Linux’s source code is freely available, allowing developers to modify and customize it for specific hardware and requirements.
- Wide hardware support: Linux supports a vast array of hardware architectures and peripherals, making it suitable for diverse embedded applications.
- Rich ecosystem: The Linux community provides a wealth of tools, libraries, and resources for embedded development.
- Scalability: Linux can be scaled down for resource-constrained devices or scaled up for more powerful systems.
- Security: Regular updates and a large community contribute to the overall security of Linux-based systems.
- Cost-effectiveness: Using Linux can significantly reduce licensing costs compared to proprietary embedded operating systems.
3. Getting Started with Embedded Linux Development
To begin your journey in embedded Linux development, you’ll need to set up a development environment and familiarize yourself with the basic concepts. Here’s a step-by-step guide to get you started:
- Choose a development board: Select a suitable hardware platform for your projects, such as Raspberry Pi, BeagleBone, or other single-board computers designed for embedded development.
- Set up a cross-compilation environment: Install a cross-compilation toolchain to build applications for your target architecture on your development machine.
- Learn the basics of the Linux kernel: Understand the architecture of the Linux kernel, its boot process, and how it interacts with hardware.
- Familiarize yourself with embedded Linux distributions: Explore popular embedded Linux distributions like Yocto Project, Buildroot, or OpenWrt.
- Study device drivers and hardware interfacing: Learn how to write and integrate device drivers for various peripherals.
- Explore embedded-specific programming concepts: Understand real-time programming, memory management, and power optimization techniques.
4. Essential Tools for Embedded Linux Development
To effectively develop applications for embedded Linux systems, you’ll need to master a set of essential tools:
- Cross-compilation toolchain: A set of tools that allow you to compile code on your development machine for the target embedded system.
- Build systems: Tools like Make, CMake, or Autotools to manage the compilation process of your projects.
- Version control systems: Git or SVN for managing your source code and collaborating with other developers.
- Integrated Development Environments (IDEs): Eclipse CDT, Qt Creator, or Visual Studio Code with appropriate plugins for embedded development.
- Debuggers: GDB (GNU Debugger) and its remote debugging capabilities for troubleshooting your applications.
- Emulators and simulators: QEMU or other emulators to test your applications without physical hardware.
- Profiling tools: Valgrind, perf, or gprof for analyzing and optimizing your application’s performance.
5. Building an Embedded Linux System
Creating a custom embedded Linux system involves several steps:
- Kernel configuration: Customize the Linux kernel for your target hardware and required features.
- Root filesystem creation: Build a minimal root filesystem containing essential system utilities and libraries.
- Bootloader setup: Configure and compile a bootloader (e.g., U-Boot) for your target hardware.
- Device tree configuration: Create or modify device tree files to describe the hardware configuration.
- Cross-compiling applications: Compile your custom applications and any required libraries for the target architecture.
- Image creation: Package the kernel, root filesystem, and bootloader into a bootable image for your embedded device.
Here’s a simple example of cross-compiling a “Hello, World!” application for an ARM-based embedded Linux system:
#include <stdio.h>
int main() {
printf("Hello, Embedded Linux World!\n");
return 0;
}
To cross-compile this program, you would use a command similar to:
arm-linux-gnueabihf-gcc -o hello hello.c
6. Embedded Linux Application Development
Developing applications for embedded Linux systems requires consideration of the unique constraints and requirements of embedded environments. Here are some key aspects to focus on:
- Resource efficiency: Optimize your code for minimal memory and CPU usage.
- Real-time performance: Implement real-time features when required, using appropriate scheduling and synchronization mechanisms.
- Hardware interfacing: Utilize Linux APIs and device drivers to interact with hardware peripherals.
- Power management: Implement power-saving features to extend battery life in portable devices.
- Security: Apply secure coding practices and utilize Linux security features to protect your applications and data.
Here’s an example of a simple embedded Linux application that reads data from a GPIO pin:
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define GPIO_PATH "/sys/class/gpio/gpio17/value"
int main() {
int fd, value;
char buffer[2];
fd = open(GPIO_PATH, O_RDONLY);
if (fd < 0) {
perror("Failed to open GPIO device");
return 1;
}
if (read(fd, buffer, sizeof(buffer)) < 0) {
perror("Failed to read GPIO value");
close(fd);
return 1;
}
value = atoi(buffer);
printf("GPIO value: %d\n", value);
close(fd);
return 0;
}
7. Debugging Embedded Linux Applications
Debugging embedded Linux applications can be challenging due to limited resources and remote execution. Here are some techniques and tools to help you debug effectively:
- Remote debugging with GDB: Use GDB’s remote debugging capabilities to debug applications running on the target device from your development machine.
- Log analysis: Implement comprehensive logging in your applications and analyze log files for troubleshooting.
- Core dump analysis: Configure your system to generate core dumps on crashes and analyze them using GDB.
- Tracing tools: Utilize tools like strace or ftrace to trace system calls and kernel functions.
- Memory debugging: Use tools like Valgrind or AddressSanitizer to detect memory-related issues.
Here’s an example of how to set up remote debugging with GDB:
- On the target device, start the GDB server:
gdbserver :1234 ./your_application
- On your development machine, connect to the GDB server:
arm-linux-gnueabihf-gdb ./your_application
(gdb) target remote target_ip:1234
(gdb) continue
8. Optimizing Embedded Linux Applications
Optimizing applications for embedded Linux systems is crucial to ensure efficient use of limited resources. Consider the following optimization techniques:
- Code optimization: Use compiler optimization flags and write efficient algorithms.
- Memory optimization: Minimize dynamic memory allocation, use static allocation when possible, and implement efficient data structures.
- I/O optimization: Use efficient I/O methods, such as memory-mapped I/O or DMA, when appropriate.
- Power optimization: Implement sleep modes, dynamic frequency scaling, and other power-saving techniques.
- Size optimization: Strip unnecessary symbols, use link-time optimization, and consider using smaller libraries.
Here’s an example of using compiler optimization flags:
arm-linux-gnueabihf-gcc -O2 -ffunction-sections -fdata-sections -Wl,--gc-sections -o optimized_app your_app.c
9. Real-World Examples of Embedded Linux Applications
Embedded Linux is used in a wide variety of applications across different industries. Here are some real-world examples:
- Consumer electronics: Smart TVs, set-top boxes, and digital cameras
- Industrial automation: Programmable logic controllers (PLCs) and human-machine interfaces (HMIs)
- Automotive systems: In-vehicle infotainment systems and advanced driver-assistance systems (ADAS)
- Internet of Things (IoT) devices: Smart home appliances, environmental sensors, and wearable devices
- Networking equipment: Routers, switches, and network-attached storage (NAS) devices
- Medical devices: Patient monitoring systems and diagnostic equipment
10. Challenges in Embedded Linux Development
While embedded Linux development offers many advantages, it also comes with its own set of challenges:
- Resource constraints: Balancing functionality with limited memory, storage, and processing power
- Real-time requirements: Ensuring deterministic behavior in time-critical applications
- Hardware diversity: Supporting a wide range of hardware architectures and peripherals
- Power management: Implementing effective power-saving strategies for battery-powered devices
- Security: Protecting embedded systems from vulnerabilities and attacks
- Long-term support: Maintaining and updating embedded Linux systems over extended periods
- Licensing compliance: Ensuring compliance with open-source licenses when using and distributing Linux-based systems
11. Future Trends in Embedded Linux
The field of embedded Linux is constantly evolving. Here are some emerging trends to watch:
- Artificial Intelligence and Machine Learning: Integration of AI/ML capabilities in embedded Linux devices for edge computing applications
- Real-time Linux: Continued improvements in real-time Linux kernels for time-critical applications
- Security enhancements: Advanced security features and hardening techniques for embedded Linux systems
- Containerization: Adoption of lightweight containerization technologies for embedded systems
- Over-the-air (OTA) updates: Improved mechanisms for remote updates and maintenance of embedded Linux devices
- Energy harvesting: Integration of energy harvesting technologies with ultra-low-power embedded Linux systems
12. Conclusion
Embedded Linux development offers a powerful and flexible approach to creating applications for a wide range of devices and industries. By leveraging the open-source nature of Linux, developers can create custom, efficient, and scalable solutions for embedded systems. As you embark on your journey in embedded Linux development, remember to focus on the unique constraints and requirements of embedded environments, such as resource efficiency, real-time performance, and hardware interfacing.
To become proficient in embedded Linux development, it’s essential to gain hands-on experience with various development boards, tools, and techniques. Start with simple projects and gradually work your way up to more complex applications. Stay up-to-date with the latest trends and best practices in the field, and don’t hesitate to engage with the vibrant embedded Linux community for support and knowledge sharing.
As the Internet of Things (IoT) and edge computing continue to grow, the demand for skilled embedded Linux developers is likely to increase. By mastering the art of creating applications for Linux-powered devices, you’ll be well-positioned to contribute to exciting projects across various industries and push the boundaries of what’s possible with embedded systems.
Remember that embedded Linux development is a continuous learning process. As new hardware architectures, tools, and techniques emerge, it’s crucial to adapt and expand your skillset. With dedication and practice, you’ll be able to create innovative and efficient embedded Linux applications that power the devices of tomorrow.