Embedded JavaScript Engines: Powering Your Applications with V8 and SpiderMonkey
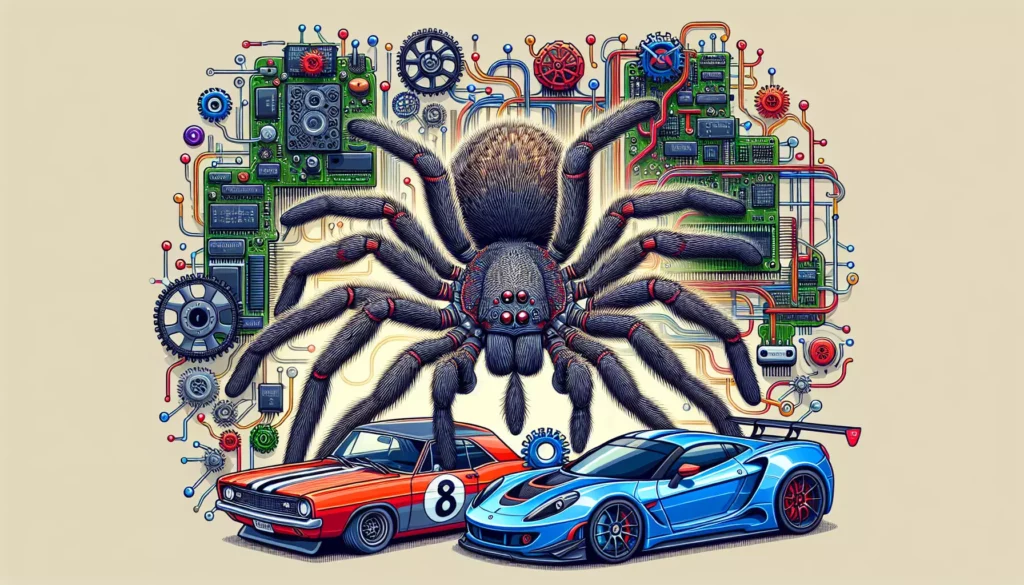
In the ever-evolving landscape of software development, the ability to incorporate scripting capabilities into applications has become increasingly important. This is where embedded JavaScript engines come into play, offering developers powerful tools to enhance their applications with dynamic scripting capabilities. In this comprehensive guide, we’ll delve deep into the world of embedded JavaScript engines, with a particular focus on two industry giants: V8 and SpiderMonkey.
Understanding Embedded JavaScript Engines
Before we dive into the specifics of V8 and SpiderMonkey, let’s first understand what embedded JavaScript engines are and why they’re crucial in modern application development.
What are Embedded JavaScript Engines?
Embedded JavaScript engines are standalone implementations of JavaScript interpreters and runtime environments that can be integrated into larger applications. These engines allow developers to execute JavaScript code within their applications, enabling dynamic scripting capabilities and extending the functionality of their software.
Why Use Embedded JavaScript Engines?
There are several compelling reasons to incorporate embedded JavaScript engines into your applications:
- Extensibility: Allow users to customize and extend your application’s functionality through scripting.
- Dynamic Content: Generate dynamic content or perform complex calculations on the fly.
- Plugin Systems: Create robust plugin architectures that enable third-party developers to enhance your application.
- Cross-platform Compatibility: Leverage JavaScript’s widespread support to ensure your scripting features work across different platforms.
- Rapid Prototyping: Quickly implement and test new features using JavaScript before committing to native code implementations.
V8: Google’s High-Performance JavaScript Engine
V8 is an open-source JavaScript engine developed by Google for the Chrome browser. It has gained immense popularity due to its high performance and has found applications beyond the browser, including server-side JavaScript execution with Node.js.
Key Features of V8
- Just-In-Time (JIT) Compilation: V8 compiles JavaScript code to native machine code before execution, significantly improving performance.
- Efficient Garbage Collection: V8 employs advanced garbage collection techniques to manage memory efficiently.
- Optimizing Compiler: The engine includes an optimizing compiler that can further improve the performance of hot code paths.
- Inline Caching: V8 uses inline caching to speed up property access and method calls.
- Hidden Classes: A mechanism to optimize the way JavaScript objects are represented in memory.
Embedding V8 in Your Application
To embed V8 in your application, you’ll need to follow these general steps:
- Download and build the V8 library for your target platform.
- Include the necessary V8 headers in your project.
- Initialize the V8 engine in your application.
- Create a V8 context for script execution.
- Compile and run JavaScript code within the context.
- Handle the results and potential exceptions.
Here’s a basic example of how to embed V8 in a C++ application:
#include <v8.h>
int main(int argc, char* argv[]) {
// Initialize V8
v8::V8::InitializeICUDefaultLocation(argv[0]);
v8::V8::InitializeExternalStartupData(argv[0]);
std::unique_ptr<v8::Platform> platform = v8::platform::NewDefaultPlatform();
v8::V8::InitializePlatform(platform.get());
v8::V8::Initialize();
// Create a new Isolate and make it the current one
v8::Isolate::CreateParams create_params;
create_params.array_buffer_allocator = v8::ArrayBuffer::Allocator::NewDefaultAllocator();
v8::Isolate* isolate = v8::Isolate::New(create_params);
{
v8::Isolate::Scope isolate_scope(isolate);
v8::HandleScope handle_scope(isolate);
// Create a new context
v8::Local<v8::Context> context = v8::Context::New(isolate);
v8::Context::Scope context_scope(context);
// Create a string containing the JavaScript source code
v8::Local<v8::String> source = v8::String::NewFromUtf8(isolate, "'Hello, World!'", v8::NewStringType::kNormal).ToLocalChecked();
// Compile the source code
v8::Local<v8::Script> script = v8::Script::Compile(context, source).ToLocalChecked();
// Run the script to get the result
v8::Local<v8::Value> result = script->Run(context).ToLocalChecked();
// Convert the result to a UTF8 string and print it
v8::String::Utf8Value utf8(isolate, result);
printf("%s\n", *utf8);
}
// Dispose the isolate and tear down V8
isolate->Dispose();
v8::V8::Dispose();
v8::V8::ShutdownPlatform();
delete create_params.array_buffer_allocator;
return 0;
}
This example demonstrates the basic steps of initializing V8, creating a context, compiling and running a simple JavaScript script, and handling the result.
SpiderMonkey: Mozilla’s Versatile JavaScript Engine
SpiderMonkey is the JavaScript engine developed by Mozilla for the Firefox browser. It’s known for its compliance with ECMAScript standards and its extensive set of features.
Key Features of SpiderMonkey
- JIT Compilation: Like V8, SpiderMonkey uses JIT compilation to optimize JavaScript execution.
- Garbage Collection: SpiderMonkey implements efficient garbage collection algorithms to manage memory.
- ECMAScript Compliance: The engine is known for its strict adherence to ECMAScript standards.
- Debugger Support: SpiderMonkey includes built-in support for debugging JavaScript code.
- WASM Support: The engine supports WebAssembly, allowing for near-native performance of code compiled from languages like C++ and Rust.
Embedding SpiderMonkey in Your Application
To embed SpiderMonkey in your application, you’ll follow a similar process to V8:
- Download and build the SpiderMonkey library.
- Include the necessary SpiderMonkey headers in your project.
- Initialize the SpiderMonkey runtime.
- Create a context for script execution.
- Compile and execute JavaScript code.
- Handle the results and potential exceptions.
Here’s a basic example of embedding SpiderMonkey in a C++ application:
#include <jsapi.h>
#include <js/Initialization.h>
static JSBool
print(JSContext* cx, unsigned argc, jsval* vp)
{
JS::CallArgs args = CallArgsFromVp(argc, vp);
for (unsigned i = 0; i < args.length(); i++) {
JSString* str = args[i].toString();
char* bytes = JS_EncodeString(cx, str);
if (!bytes)
return false;
printf("%s", bytes);
JS_free(cx, bytes);
}
printf("\n");
args.rval().setUndefined();
return true;
}
int main(int argc, const char *argv[])
{
JS_Init();
JSRuntime* rt = JS_NewRuntime(8L * 1024 * 1024);
if (!rt)
return 1;
JSContext* cx = JS_NewContext(rt, 8192);
if (!cx)
return 1;
JS::RootedObject global(cx, JS_NewGlobalObject(cx, &global_class, nullptr, JS::FireOnNewGlobalHook));
if (!global)
return 1;
JS::RootedValue rval(cx);
{
JSAutoCompartment ac(cx, global);
JS_InitStandardClasses(cx, global);
JS_DefineFunction(cx, global, "print", print, 0, 0);
const char* script = "'Hello, World!';";
JS::CompileOptions options(cx);
options.setFileAndLine("noname", 1);
JS::Evaluate(cx, options, script, strlen(script), &rval);
}
JS::RootedString str(cx, rval.toString());
char* bytes = JS_EncodeString(cx, str);
if (!bytes)
return 1;
printf("%s\n", bytes);
JS_free(cx, bytes);
JS_DestroyContext(cx);
JS_DestroyRuntime(rt);
JS_ShutDown();
return 0;
}
This example shows how to set up SpiderMonkey, create a global object, define a custom print function, and execute a simple JavaScript script.
Comparing V8 and SpiderMonkey
While both V8 and SpiderMonkey are powerful JavaScript engines, they have some differences that may influence your choice:
Feature | V8 | SpiderMonkey |
---|---|---|
Performance | Generally considered faster for most benchmarks | Competitive performance, especially in recent versions |
Memory Usage | Typically uses less memory | May use more memory in some scenarios |
ECMAScript Compliance | High, but may lag behind in some newer features | Very high, often leading in implementing new ECMAScript features |
Debugging Support | Good debugging capabilities | Excellent built-in debugging support |
Community and Resources | Large community, especially due to Node.js | Smaller but dedicated community |
Best Practices for Embedding JavaScript Engines
When integrating V8, SpiderMonkey, or any other JavaScript engine into your application, consider the following best practices:
- Security: Be cautious when executing user-provided scripts. Implement proper sandboxing and limit access to sensitive APIs.
- Memory Management: Pay attention to memory usage, especially when dealing with long-running scripts or large datasets.
- Error Handling: Implement robust error handling to gracefully manage JavaScript exceptions and prevent crashes.
- Performance Optimization: Profile your application to identify bottlenecks and optimize critical code paths.
- API Design: Carefully design the interface between your application and the JavaScript environment to ensure ease of use and maintainability.
- Version Management: Keep the embedded engine up-to-date to benefit from performance improvements and security fixes.
- Cross-platform Considerations: If your application targets multiple platforms, ensure compatibility across different operating systems and architectures.
Real-world Applications of Embedded JavaScript Engines
Embedded JavaScript engines have found their way into various applications and industries. Here are some notable examples:
- Game Engines: Unity and Unreal Engine use embedded JavaScript (or similar scripting languages) for game logic and modding.
- Desktop Applications: Electron, which powers apps like Visual Studio Code and Atom, embeds V8 to run JavaScript on the desktop.
- Server-side Applications: Node.js uses V8 to run JavaScript on the server, enabling full-stack JavaScript development.
- Databases: Some NoSQL databases like MongoDB use JavaScript engines for query processing and server-side scripting.
- Embedded Systems: IoT devices and smart appliances often use embedded JavaScript engines for scripting and automation.
- Content Creation Tools: Adobe Creative Suite applications use embedded JavaScript engines for extensibility and automation.
Future Trends in Embedded JavaScript Engines
As we look to the future, several trends are shaping the landscape of embedded JavaScript engines:
- WebAssembly Integration: Closer integration with WebAssembly (WASM) is allowing for even better performance and interoperability with other languages.
- Improved Security Features: Enhanced sandboxing and security measures are being developed to make script execution safer in embedded environments.
- AI and Machine Learning: JavaScript engines are being optimized for AI and machine learning workloads, opening up new possibilities for embedded scripting.
- IoT and Edge Computing: Lightweight versions of JavaScript engines are being developed for resource-constrained devices, enabling scripting capabilities in IoT and edge computing scenarios.
- Real-time Capabilities: Improvements in garbage collection and overall performance are making JavaScript engines more suitable for real-time applications.
Conclusion
Embedded JavaScript engines like V8 and SpiderMonkey offer powerful tools for enhancing applications with dynamic scripting capabilities. By understanding their features, strengths, and best practices for integration, developers can leverage these engines to create more flexible, extensible, and powerful software.
As the JavaScript ecosystem continues to evolve, embedded engines will play an increasingly important role in application development across various domains. Whether you’re building a game engine, a desktop application, or an IoT device, the ability to incorporate JavaScript scripting can provide significant benefits in terms of flexibility, rapid development, and user customization.
By mastering the use of embedded JavaScript engines, developers can stay at the forefront of modern application development, creating software that is both powerful and adaptable to changing user needs. As you embark on your journey with embedded JavaScript engines, remember to keep security, performance, and cross-platform compatibility at the forefront of your development process.
The world of embedded JavaScript engines is rich with possibilities, and by harnessing their power, you can take your applications to new heights of functionality and user engagement. Whether you choose V8, SpiderMonkey, or another JavaScript engine, the key is to understand their capabilities and apply them effectively to solve real-world problems and create innovative software solutions.