Egg Drop Problem: A Comprehensive Guide to This Classic Algorithmic Challenge
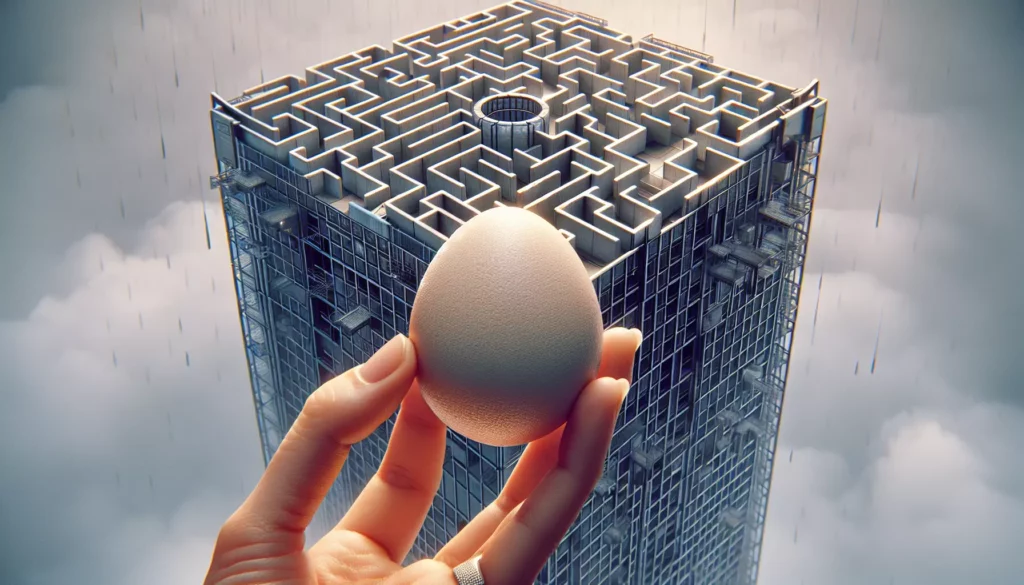
When diving into the world of algorithmic challenges, the Egg Drop problem stands as a fascinating puzzle that combines elements of optimization, dynamic programming, and binary search. This classic problem has become a staple in technical interviews, particularly at major tech companies, due to its ability to test a candidate’s problem-solving approach and algorithm design skills.
In this comprehensive guide, we’ll explore the Egg Drop problem from various angles, providing clear explanations, solution strategies, and code implementations that will help you master this challenging algorithm.
What is the Egg Drop Problem?
The Egg Drop problem can be stated as follows:
You are given k identical eggs and a building with n floors. You need to determine the highest floor from which an egg can be dropped without breaking. When an egg is dropped and doesn’t break, you can reuse it. However, once an egg breaks, it cannot be used again.
The goal is to find the minimum number of egg drops required to determine the critical floor (the highest safe floor) in the worst-case scenario.
Why is the Egg Drop Problem Important?
The Egg Drop problem is more than just a brain teaser; it represents a class of optimization problems where we need to minimize the worst-case scenario. Understanding this problem helps develop skills in:
- Dynamic programming
- Binary search optimizations
- Decision tree algorithms
- Recursive thinking
- Mathematical induction
These skills are directly applicable to real-world scenarios in software development, particularly when designing algorithms that need to make optimal decisions with limited resources.
Naive Approach: Linear Search
The simplest approach would be to start from the first floor and move up one floor at a time until the egg breaks. This approach would require at most n drops but uses only one egg. However, if we have multiple eggs, we can do better.
Binary Search Approach (When Eggs Are Plentiful)
If we have an unlimited supply of eggs, we could use a binary search approach:
function findCriticalFloor(n) {
let low = 1;
let high = n;
while (low <= high) {
let mid = Math.floor((low + high) / 2);
// Drop an egg from the middle floor
let result = dropEgg(mid);
if (result === "BREAKS") {
high = mid - 1;
} else {
low = mid + 1;
}
}
return high; // The highest floor where egg doesn't break
}
This approach would require at most log2(n) drops, but it assumes we have enough eggs to perform the binary search.
Dynamic Programming Solution
The optimal solution for the general case (limited eggs, many floors) uses dynamic programming. Let’s define:
dp[i][j] = minimum number of attempts needed to find the critical floor with i eggs and j floors in the worst case.
For each floor k, we have two possibilities:
- The egg breaks: We now have i-1 eggs and need to check floors 1 to k-1.
- The egg doesn’t break: We still have i eggs and need to check floors k+1 to j.
Since we want to minimize the maximum number of attempts in the worst case, our recurrence relation becomes:
dp[i][j] = 1 + min(max(dp[i-1][k-1], dp[i][j-k])) for all k from 1 to j
Here’s the implementation:
function eggDrop(eggs, floors) {
// Create a 2D array for dynamic programming
const dp = Array(eggs + 1).fill().map(() => Array(floors + 1).fill(0));
// Base cases:
// 1. If there are 0 floors, 0 trials needed
// 2. If there is 1 floor, 1 trial needed
// 3. If there are 0 eggs, maximum trials needed (impossible)
// If we have only one egg, we need j trials for j floors
for (let j = 1; j <= floors; j++) {
dp[1][j] = j;
}
// Fill the rest of the table
for (let i = 2; i <= eggs; i++) {
for (let j = 1; j <= floors; j++) {
dp[i][j] = Number.MAX_SAFE_INTEGER;
for (let k = 1; k <= j; k++) {
// Maximum of egg breaks or egg doesn't break scenarios
let result = 1 + Math.max(dp[i - 1][k - 1], dp[i][j - k]);
// Minimize the worst case
dp[i][j] = Math.min(dp[i][j], result);
}
}
}
return dp[eggs][floors];
}
// Example usage
console.log(eggDrop(2, 10)); // Output: Minimum number of trials needed with 2 eggs and 10 floors
Optimizing the Dynamic Programming Solution
The solution above has a time complexity of O(eggs × floors²), which can be slow for large inputs. We can optimize it by observing that as we increase k (the floor we drop from), dp[i-1][k-1] increases and dp[i][j-k] decreases. The optimal k is where these two values are closest.
This leads to a binary search optimization:
function eggDropOptimized(eggs, floors) {
const dp = Array(eggs + 1).fill().map(() => Array(floors + 1).fill(0));
for (let j = 1; j <= floors; j++) {
dp[1][j] = j;
}
for (let i = 2; i <= eggs; i++) {
for (let j = 1; j <= floors; j++) {
dp[i][j] = Number.MAX_SAFE_INTEGER;
let left = 1;
let right = j;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
let eggBreaks = dp[i - 1][mid - 1];
let eggSurvives = dp[i][j - mid];
let result = 1 + Math.max(eggBreaks, eggSurvives);
dp[i][j] = Math.min(dp[i][j], result);
if (eggBreaks < eggSurvives) {
left = mid + 1;
} else {
right = mid - 1;
}
}
}
}
return dp[eggs][floors];
}
This optimization reduces the time complexity to O(eggs × floors × log(floors)).
Mathematical Solution
For the special case of 2 eggs, there’s an elegant mathematical solution. The optimal strategy is to use what’s called the “drop schedule” approach:
- Drop the first egg at floor x, then at floor x+(x-1), then at floor x+(x-1)+(x-2), and so on.
- If the first egg breaks, use the second egg to check each floor in the range one by one.
The optimal value of x is the solution to the equation x + (x-1) + (x-2) + … + 1 ≥ n, which simplifies to x(x+1)/2 ≥ n.
Solving for x, we get x ≈ √(2n).
function eggDrop2Eggs(floors) {
return Math.ceil(Math.sqrt(2 * floors));
}
// Example usage
console.log(eggDrop2Eggs(100)); // Output: Minimum number of trials needed with 2 eggs and 100 floors
Practical Applications of the Egg Drop Problem
The Egg Drop problem might seem abstract, but its principles apply to many real-world scenarios:
1. Software Testing and Debugging
When tracking down a bug that appears at a certain version, the Egg Drop algorithm can help minimize the number of versions you need to check.
2. Network Packet Size Optimization
Finding the maximum transmission unit (MTU) for a network path involves a similar binary search approach.
3. Resource Allocation
Determining how to distribute limited resources across multiple tasks can use similar dynamic programming approaches.
4. System Design
When designing systems with limited fault tolerance, the concepts from the Egg Drop problem help determine optimal checkpoint frequencies.
Common Variations of the Egg Drop Problem
Variation 1: Egg Drop with Fixed Number of Floors
Given exactly k eggs and n floors, find the minimum number of drops needed in the worst case.
Variation 2: Egg Drop with Variable Breaking Points
Each egg has a different breaking threshold, making the problem more complex.
Variation 3: Egg Drop with a Cost Function
Different floors have different costs for dropping eggs, and you need to minimize the total cost.
Interview Tips for the Egg Drop Problem
If you encounter the Egg Drop problem in a technical interview, keep these tips in mind:
- Start with small examples: Work through cases with 1 or 2 eggs and a small number of floors to build intuition.
- Identify the recurrence relation: Explain how the solution for n floors relates to smaller subproblems.
- Consider edge cases: What if there’s only 1 egg? What if there are 0 floors?
- Analyze time and space complexity: Be prepared to discuss the efficiency of your solution.
- Optimize incrementally: Start with a working solution, then look for optimizations.
Common Mistakes to Avoid
- Confusing worst case with average case: The problem asks for the minimum number of drops in the worst-case scenario.
- Ignoring the state of eggs: Remember that broken eggs cannot be reused.
- Overlooking the optimal drop strategy: The drop strategy should adapt based on whether the previous egg broke.
- Implementing inefficient recursion: Without memoization, a recursive solution will time out for large inputs.
Conclusion
The Egg Drop problem is a fascinating algorithmic challenge that combines several important concepts in computer science. By understanding the various approaches to solve it, from naive linear search to optimized dynamic programming, you’ll develop valuable skills that extend far beyond this specific problem.
Whether you’re preparing for technical interviews at major tech companies or simply looking to improve your algorithmic thinking, mastering the Egg Drop problem is a significant achievement. The problem-solving strategies you learn here will serve you well across many different programming challenges.
Remember, the key to solving complex problems like the Egg Drop is to break them down into smaller, manageable subproblems and build your solution step by step. With practice and persistence, you’ll develop the algorithmic intuition needed to tackle even the most challenging coding problems.
Happy coding, and may all your eggs land safely!