Efficient Algorithms for Matrix Operations: Mastering the Fundamentals
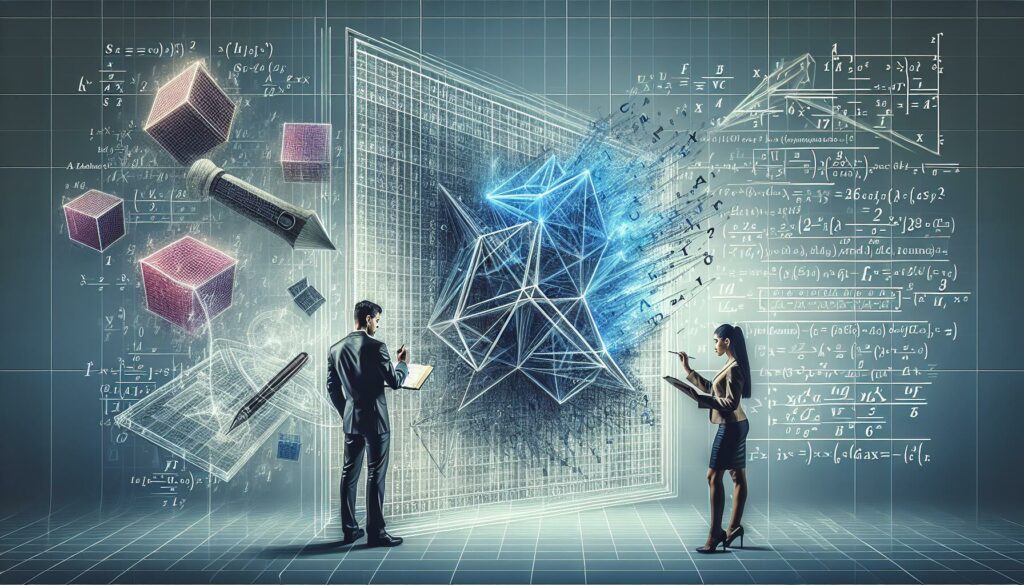
In the world of computer science and programming, matrices play a crucial role in various applications, from computer graphics to machine learning. Understanding and implementing efficient algorithms for matrix operations is essential for any aspiring software engineer or data scientist. In this comprehensive guide, we’ll explore the most important matrix operations and the algorithms that make them efficient, helping you to level up your coding skills and prepare for technical interviews at top tech companies.
1. Introduction to Matrices
Before diving into the algorithms, let’s quickly review what matrices are and why they’re important in computer science:
- A matrix is a rectangular array of numbers, symbols, or expressions arranged in rows and columns.
- Matrices are used to represent linear transformations, solve systems of linear equations, and store data in a structured format.
- In programming, matrices are typically implemented as 2D arrays or lists of lists.
Now, let’s explore some of the most common matrix operations and the efficient algorithms used to perform them.
2. Matrix Addition and Subtraction
Matrix addition and subtraction are the simplest matrix operations. They involve adding or subtracting corresponding elements of two matrices of the same dimensions.
Algorithm:
- Check if the dimensions of both matrices are the same.
- Iterate through each element of both matrices simultaneously.
- Add (or subtract) the corresponding elements and store the result in a new matrix.
Python Implementation:
def matrix_addition(matrix1, matrix2):
if len(matrix1) != len(matrix2) or len(matrix1[0]) != len(matrix2[0]):
raise ValueError("Matrices must have the same dimensions")
result = []
for i in range(len(matrix1)):
row = []
for j in range(len(matrix1[0])):
row.append(matrix1[i][j] + matrix2[i][j])
result.append(row)
return result
# Example usage
matrix1 = [[1, 2], [3, 4]]
matrix2 = [[5, 6], [7, 8]]
result = matrix_addition(matrix1, matrix2)
print(result) # Output: [[6, 8], [10, 12]]
Time Complexity: O(n * m), where n is the number of rows and m is the number of columns.
Space Complexity: O(n * m) to store the result matrix.
3. Matrix Multiplication
Matrix multiplication is a fundamental operation in linear algebra and has numerous applications in computer science. The standard algorithm for matrix multiplication is straightforward but can be inefficient for large matrices.
Standard Algorithm:
- Check if the number of columns in the first matrix equals the number of rows in the second matrix.
- Create a result matrix with dimensions (rows of first matrix) x (columns of second matrix).
- For each element in the result matrix, compute the dot product of the corresponding row from the first matrix and column from the second matrix.
Python Implementation:
def matrix_multiplication(matrix1, matrix2):
if len(matrix1[0]) != len(matrix2):
raise ValueError("Number of columns in first matrix must equal number of rows in second matrix")
result = [[0 for _ in range(len(matrix2[0]))] for _ in range(len(matrix1))]
for i in range(len(matrix1)):
for j in range(len(matrix2[0])):
for k in range(len(matrix2)):
result[i][j] += matrix1[i][k] * matrix2[k][j]
return result
# Example usage
matrix1 = [[1, 2], [3, 4]]
matrix2 = [[5, 6], [7, 8]]
result = matrix_multiplication(matrix1, matrix2)
print(result) # Output: [[19, 22], [43, 50]]
Time Complexity: O(n * m * p), where n is the number of rows in the first matrix, m is the number of columns in the first matrix (and rows in the second matrix), and p is the number of columns in the second matrix.
Space Complexity: O(n * p) to store the result matrix.
Strassen’s Algorithm for Matrix Multiplication
For large matrices, Strassen’s algorithm provides a more efficient approach to matrix multiplication. It reduces the number of recursive calls from 8 (in the standard divide-and-conquer approach) to 7, resulting in a better time complexity.
The key idea behind Strassen’s algorithm is to perform clever combinations of matrix additions and subtractions to reduce the number of recursive multiplications.
Steps of Strassen’s Algorithm:
- Divide the input matrices into four submatrices each.
- Compute seven products using these submatrices with clever combinations.
- Compute the four quadrants of the result matrix using these products.
- Combine the quadrants to get the final result.
Python Implementation of Strassen’s Algorithm:
import numpy as np
def strassen(x, y):
if len(x) <= 32: # Base case: use standard multiplication for small matrices
return x.dot(y)
n = len(x)
# Splitting the matrices into quadrants
a11 = x[:n//2, :n//2]
a12 = x[:n//2, n//2:]
a21 = x[n//2:, :n//2]
a22 = x[n//2:, n//2:]
b11 = y[:n//2, :n//2]
b12 = y[:n//2, n//2:]
b21 = y[n//2:, :n//2]
b22 = y[n//2:, n//2:]
# Computing the 7 products
p1 = strassen(a11 + a22, b11 + b22)
p2 = strassen(a21 + a22, b11)
p3 = strassen(a11, b12 - b22)
p4 = strassen(a22, b21 - b11)
p5 = strassen(a11 + a12, b22)
p6 = strassen(a21 - a11, b11 + b12)
p7 = strassen(a12 - a22, b21 + b22)
# Computing the 4 quadrants of the result
c11 = p1 + p4 - p5 + p7
c12 = p3 + p5
c21 = p2 + p4
c22 = p1 - p2 + p3 + p6
# Combining the quadrants
result = np.vstack((np.hstack((c11, c12)), np.hstack((c21, c22))))
return result
# Example usage
matrix1 = np.array([[1, 2, 3, 4], [5, 6, 7, 8], [9, 10, 11, 12], [13, 14, 15, 16]])
matrix2 = np.array([[17, 18, 19, 20], [21, 22, 23, 24], [25, 26, 27, 28], [29, 30, 31, 32]])
result = strassen(matrix1, matrix2)
print(result)
Time Complexity: O(n^log2(7)) ≈ O(n^2.8074), which is better than the standard O(n^3) algorithm for large matrices.
Space Complexity: O(n^2) due to the recursive nature of the algorithm.
While Strassen’s algorithm has a better asymptotic time complexity, it’s worth noting that it’s often slower for small to medium-sized matrices due to the overhead of recursion and additional memory usage. In practice, it’s typically used for very large matrices or as part of a hybrid algorithm that switches to standard multiplication for smaller submatrices.
4. Matrix Transposition
Matrix transposition is the operation of flipping a matrix over its diagonal, switching the row and column indices of each element. This operation is frequently used in various matrix algorithms and has applications in areas like computer graphics and machine learning.
Algorithm:
- Create a new matrix with dimensions swapped (rows become columns and vice versa).
- Iterate through each element of the original matrix.
- Place each element in the new matrix with its indices swapped.
Python Implementation:
def matrix_transpose(matrix):
rows = len(matrix)
cols = len(matrix[0])
# Create a new matrix with swapped dimensions
result = [[0 for _ in range(rows)] for _ in range(cols)]
for i in range(rows):
for j in range(cols):
result[j][i] = matrix[i][j]
return result
# Example usage
matrix = [[1, 2, 3], [4, 5, 6]]
transposed = matrix_transpose(matrix)
print(transposed) # Output: [[1, 4], [2, 5], [3, 6]]
Time Complexity: O(n * m), where n is the number of rows and m is the number of columns in the original matrix.
Space Complexity: O(n * m) to store the transposed matrix.
In-place Transposition for Square Matrices
For square matrices, we can perform the transposition in-place, which saves memory but is slightly more complex:
def in_place_transpose(matrix):
n = len(matrix)
for i in range(n):
for j in range(i + 1, n):
matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
return matrix
# Example usage
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
transposed = in_place_transpose(matrix)
print(transposed) # Output: [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
Time Complexity: O(n^2) for a square matrix of size n x n.
Space Complexity: O(1) as we’re modifying the matrix in-place.
5. Matrix Determinant
The determinant of a square matrix is a scalar value that provides important information about the matrix’s properties. It’s used in various applications, including solving systems of linear equations and finding inverse matrices.
Recursive Algorithm (for small matrices):
- If the matrix is 1×1, return its only element.
- If the matrix is 2×2, return ad – bc for matrix [[a, b], [c, d]].
- For larger matrices:
- Choose a row or column (typically the first row).
- For each element in the chosen row/column:
- Calculate the cofactor (determinant of submatrix * (-1)^(i+j)).
- Multiply the element by its cofactor.
- Sum these products to get the determinant.
Python Implementation (Recursive):
def matrix_determinant(matrix):
n = len(matrix)
# Base case: 1x1 matrix
if n == 1:
return matrix[0][0]
# Base case: 2x2 matrix
if n == 2:
return matrix[0][0] * matrix[1][1] - matrix[0][1] * matrix[1][0]
det = 0
for j in range(n):
# Create submatrix by removing first row and j-th column
submatrix = [row[:j] + row[j+1:] for row in matrix[1:]]
# Calculate cofactor and add to determinant
cofactor = (-1) ** j * matrix[0][j] * matrix_determinant(submatrix)
det += cofactor
return det
# Example usage
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
det = matrix_determinant(matrix)
print(det) # Output: 0
Time Complexity: O(n!), where n is the size of the matrix. This recursive algorithm is inefficient for large matrices.
Space Complexity: O(n^2) due to the recursive calls and creation of submatrices.
LU Decomposition for Efficient Determinant Calculation
For larger matrices, LU decomposition provides a more efficient method to calculate the determinant. The idea is to decompose the matrix A into a lower triangular matrix L and an upper triangular matrix U, such that A = LU. The determinant of A is then the product of the diagonal elements of U (or L, as det(L) = 1).
Python Implementation using NumPy:
import numpy as np
def efficient_determinant(matrix):
try:
# Perform LU decomposition
L, U = np.linalg.lu(matrix)
# Calculate determinant as product of diagonal elements of U
det = np.prod(np.diag(U))
return det
except np.linalg.LinAlgError:
# Matrix is singular (determinant is zero)
return 0
# Example usage
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
det = efficient_determinant(matrix)
print(det) # Output: -9.51619735392994e-16 (essentially zero due to floating-point precision)
Time Complexity: O(n^3) for the LU decomposition.
Space Complexity: O(n^2) to store the L and U matrices.
This method is much more efficient for large matrices compared to the recursive approach.
6. Matrix Inversion
Matrix inversion is the process of finding the inverse of a square matrix, if it exists. The inverse of a matrix A, denoted as A^(-1), is a matrix that, when multiplied with A, yields the identity matrix.
Gauss-Jordan Elimination Algorithm:
- Create an augmented matrix [A|I] where A is the input matrix and I is the identity matrix of the same size.
- Perform row operations to transform the left side (A) into the identity matrix:
- For each column k:
- Find a non-zero element in column k, row i >= k.
- Swap row i with row k if necessary.
- Divide row k by the element at (k, k) to make it 1.
- Subtract multiples of row k from other rows to make their column k elements 0.
- For each column k:
- The right side of the augmented matrix will now be A^(-1).
Python Implementation:
import numpy as np
def matrix_inverse(matrix):
n = len(matrix)
augmented = np.hstack((matrix, np.identity(n)))
for k in range(n):
# Find non-zero element in column k
if augmented[k, k] == 0:
for i in range(k + 1, n):
if augmented[i, k] != 0:
augmented[k], augmented[i] = augmented[i].copy(), augmented[k].copy()
break
else:
raise ValueError("Matrix is not invertible")
# Make the diagonal element 1
augmented[k] = augmented[k] / augmented[k, k]
# Make other elements in column k zero
for i in range(n):
if i != k:
augmented[i] -= augmented[k] * augmented[i, k]
return augmented[:, n:]
# Example usage
matrix = np.array([[1, 2], [3, 4]])
inverse = matrix_inverse(matrix)
print(inverse)
# Verify the result
print(np.allclose(np.dot(matrix, inverse), np.identity(2)))
Time Complexity: O(n^3), where n is the size of the matrix.
Space Complexity: O(n^2) to store the augmented matrix.
Using NumPy for Matrix Inversion
For practical applications, it’s often more efficient to use optimized libraries like NumPy:
import numpy as np
def numpy_matrix_inverse(matrix):
return np.linalg.inv(matrix)
# Example usage
matrix = np.array([[1, 2], [3, 4]])
inverse = numpy_matrix_inverse(matrix)
print(inverse)
# Verify the result
print(np.allclose(np.dot(matrix, inverse), np.identity(2)))
The NumPy implementation is typically faster and more numerically stable, especially for larger matrices.
7. Conclusion
Mastering efficient algorithms for matrix operations is crucial for any programmer working in fields like computer graphics, machine learning, or scientific computing. The algorithms we’ve covered here form the foundation for more advanced matrix operations and optimizations.
Key takeaways:
- Understanding the basic operations (addition, multiplication, transposition) is essential.
- For large matrices, consider using optimized algorithms like Strassen’s for multiplication.
- Determinant calculation can be significantly improved using LU decomposition for large matrices.
- Matrix inversion is a complex operation, and using optimized libraries like NumPy is often the best approach in practice.
- Always consider the trade-offs between implementation complexity, time complexity, and space complexity when choosing an algorithm.
As you continue to develop your skills in algorithmic thinking and problem-solving, remember that matrix operations are just one piece of the puzzle. Keep practicing, exploring new algorithms, and applying these concepts to real-world problems to become a proficient programmer ready for technical interviews at top tech companies.
Happy coding!