Effective Ways to Debug Code: A Comprehensive Guide for Programmers
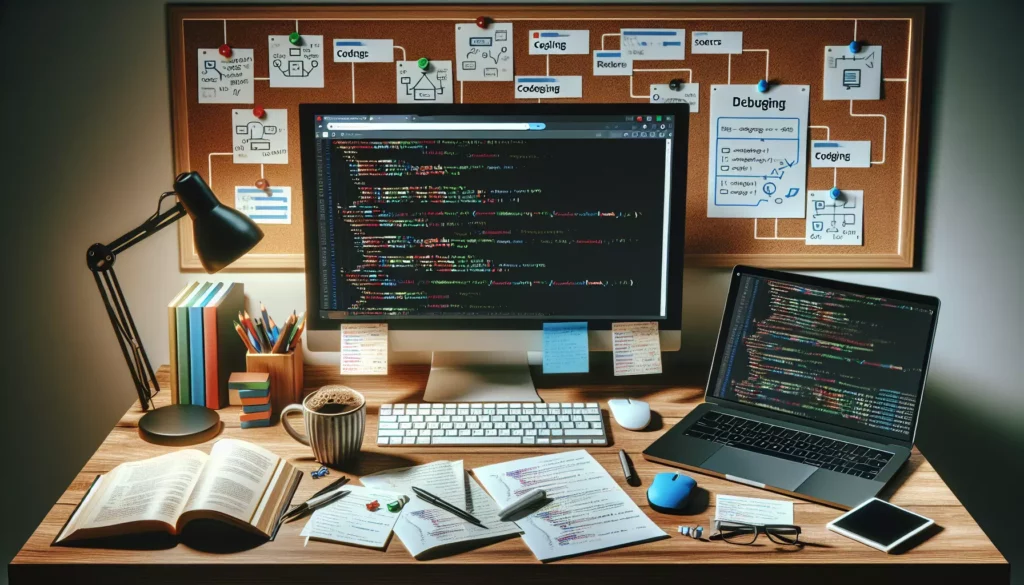
Debugging is an essential skill for every programmer, from beginners to seasoned professionals. It’s the process of identifying, isolating, and fixing errors or “bugs” in your code. Effective debugging can save you countless hours of frustration and help you deliver more reliable software. In this comprehensive guide, we’ll explore various techniques and tools to make your debugging process more efficient and productive.
1. Understanding the Importance of Debugging
Before diving into specific debugging techniques, it’s crucial to understand why debugging is so important in the software development process:
- Improves code quality
- Enhances software reliability
- Saves time and resources in the long run
- Helps developers learn and improve their coding skills
- Ensures better user experience in the final product
2. Common Types of Bugs
To effectively debug your code, it’s helpful to be familiar with common types of bugs you might encounter:
- Syntax errors: Mistakes in the code structure that prevent it from compiling or running
- Logic errors: Issues with the program’s logic that cause incorrect results
- Runtime errors: Errors that occur during program execution
- Semantic errors: Mistakes in the code’s meaning or intent
- Integration errors: Issues that arise when different parts of a program interact
3. Effective Debugging Techniques
3.1. Print Debugging
One of the simplest and most widely used debugging techniques is print debugging. This method involves adding print statements to your code to track variable values, execution flow, and program state.
Example in Python:
def calculate_average(numbers):
print(f"Input: {numbers}") # Debug print
total = sum(numbers)
count = len(numbers)
print(f"Total: {total}, Count: {count}") # Debug print
average = total / count
print(f"Average: {average}") # Debug print
return average
result = calculate_average([1, 2, 3, 4, 5])
print(f"Result: {result}")
While simple, print debugging can be very effective for quickly identifying issues in your code.
3.2. Using a Debugger
Most modern Integrated Development Environments (IDEs) come with built-in debuggers. These powerful tools allow you to:
- Set breakpoints in your code
- Step through code execution line by line
- Inspect variable values at different points in the program
- Modify variable values during runtime
- Analyze the call stack
Example of using a debugger in Python with Visual Studio Code:
- Set a breakpoint by clicking on the left margin of your code editor.
- Start debugging by pressing F5 or clicking the “Run and Debug” button.
- Use the debug controls to step through your code, examining variables and program flow.
3.3. Logging
Logging is a more advanced form of print debugging that allows you to record information about your program’s execution. Most programming languages have built-in logging libraries that offer various log levels (e.g., DEBUG, INFO, WARNING, ERROR).
Example in Python using the logging module:
import logging
logging.basicConfig(level=logging.DEBUG)
def calculate_average(numbers):
logging.debug(f"Input: {numbers}")
total = sum(numbers)
count = len(numbers)
logging.info(f"Total: {total}, Count: {count}")
average = total / count
logging.debug(f"Average: {average}")
return average
result = calculate_average([1, 2, 3, 4, 5])
logging.info(f"Result: {result}")
Logging provides a more structured and flexible approach to tracking program execution compared to simple print statements.
3.4. Rubber Duck Debugging
Rubber duck debugging is a technique where you explain your code line-by-line to an inanimate object (like a rubber duck). This process often helps you identify issues in your logic or implementation as you verbalize your thought process.
Steps for rubber duck debugging:
- Get a rubber duck (or any object)
- Explain your code to the duck, line by line
- As you explain, pay attention to any inconsistencies or unclear parts in your explanation
- Often, you’ll spot the issue yourself during this process
3.5. Code Review
Having another developer review your code can be an excellent way to catch bugs and improve code quality. Code reviews can be formal or informal and can help identify issues that you might have overlooked.
Benefits of code review:
- Fresh perspective on your code
- Identification of potential bugs or inefficiencies
- Knowledge sharing among team members
- Improved overall code quality
3.6. Unit Testing
Writing unit tests for your code can help catch bugs early and ensure that your code behaves as expected. Unit tests are small, focused tests that check individual components or functions of your program.
Example of a unit test in Python using the unittest module:
import unittest
def calculate_average(numbers):
return sum(numbers) / len(numbers)
class TestCalculateAverage(unittest.TestCase):
def test_calculate_average(self):
self.assertEqual(calculate_average([1, 2, 3, 4, 5]), 3)
self.assertEqual(calculate_average([0, 0, 0]), 0)
self.assertAlmostEqual(calculate_average([1.5, 2.5, 3.5]), 2.5)
if __name__ == '__main__':
unittest.main()
Regular unit testing can help you catch bugs early in the development process and make it easier to refactor your code with confidence.
4. Advanced Debugging Techniques
4.1. Static Code Analysis
Static code analysis tools examine your code without executing it, helping to identify potential issues, coding standard violations, and security vulnerabilities. These tools can catch many common programming errors before your code even runs.
Popular static analysis tools:
- For Python: Pylint, Flake8
- For JavaScript: ESLint, JSHint
- For Java: SonarQube, FindBugs
- For C/C++: Cppcheck, Clang Static Analyzer
4.2. Dynamic Analysis
Dynamic analysis involves examining your code as it runs. This can help identify issues that only occur during program execution, such as memory leaks or race conditions.
Tools for dynamic analysis:
- Valgrind (for C/C++)
- JProfiler (for Java)
- Python Memory Profiler
4.3. Remote Debugging
Remote debugging allows you to debug code running on a different machine or environment. This is particularly useful for debugging issues that only occur in production or on specific platforms.
Steps for remote debugging:
- Set up a debugging server on the remote machine
- Configure your local IDE to connect to the remote debugging server
- Set breakpoints and debug as you would locally
4.4. Time Travel Debugging
Time travel debugging, also known as reverse debugging, allows you to step backwards through your code’s execution. This can be incredibly useful for tracking down the source of a bug.
Tools that support time travel debugging:
- GDB (GNU Debugger) with reverse debugging capabilities
- Microsoft’s Time Travel Debugging for Windows
- Undo LiveRecorder for Linux
5. Debugging Best Practices
To make your debugging process more efficient and effective, consider these best practices:
5.1. Reproduce the Bug
Before you start debugging, make sure you can consistently reproduce the bug. This might involve:
- Identifying the specific inputs or conditions that trigger the bug
- Creating a minimal test case that demonstrates the issue
- Documenting the steps to reproduce the bug
5.2. Isolate the Problem
Try to narrow down the source of the bug as much as possible:
- Use binary search techniques to identify the problematic section of code
- Comment out sections of code to isolate the issue
- Create simplified versions of your code to test specific components
5.3. Use Version Control
Leverage version control systems like Git to:
- Track changes in your code over time
- Revert to previous working versions if needed
- Create branches for experimenting with different bug fixes
5.4. Take Breaks
Debugging can be mentally taxing. Remember to:
- Take regular breaks to avoid burnout
- Step away from a problem if you’re stuck – a fresh perspective can work wonders
- Collaborate with colleagues to get new ideas and approaches
5.5. Learn from Your Bugs
Every bug is an opportunity to improve your coding skills:
- Analyze the root cause of each bug you encounter
- Document common issues and their solutions
- Share your learnings with your team to prevent similar bugs in the future
6. Debugging Tools and IDEs
Choosing the right debugging tools can significantly improve your productivity. Here are some popular debugging tools and IDEs for different programming languages:
6.1. Python
- PyCharm: A powerful IDE with advanced debugging features
- Visual Studio Code with Python extension: Offers a great balance of features and performance
- PDB (Python Debugger): A built-in command-line debugger for Python
6.2. JavaScript
- Chrome DevTools: Built-in developer tools for debugging JavaScript in the browser
- Visual Studio Code: Excellent support for JavaScript debugging
- WebStorm: A full-featured IDE for JavaScript development
6.3. Java
- IntelliJ IDEA: A comprehensive IDE with powerful debugging capabilities
- Eclipse: A popular open-source IDE with robust debugging features
- JDB (Java Debugger): A command-line debugger included with the JDK
6.4. C/C++
- Visual Studio: Microsoft’s IDE with excellent debugging support for C and C++
- GDB (GNU Debugger): A powerful command-line debugger for multiple languages
- CLion: JetBrains’ IDE for C and C++ development with advanced debugging features
7. Debugging in Different Environments
Debugging techniques may vary depending on the environment you’re working in. Here are some considerations for different scenarios:
7.1. Web Development
When debugging web applications, consider:
- Using browser developer tools to inspect HTML, CSS, and JavaScript
- Leveraging network monitoring tools to debug API calls and data transfers
- Utilizing cross-browser testing tools to identify browser-specific issues
7.2. Mobile Development
For mobile app debugging:
- Use platform-specific tools like Xcode for iOS or Android Studio for Android
- Employ device emulators or simulators for testing on various device configurations
- Utilize remote debugging tools to debug apps on physical devices
7.3. Server-Side Development
When debugging server-side applications:
- Use logging extensively to track program flow and state
- Leverage server monitoring tools to identify performance issues
- Employ remote debugging techniques for production environments
8. Conclusion
Debugging is an essential skill for any programmer, and mastering various debugging techniques can significantly improve your productivity and code quality. By understanding common types of bugs, leveraging appropriate tools, and following best practices, you can approach debugging with confidence and efficiency.
Remember that debugging is not just about fixing errors – it’s an opportunity to learn, improve your coding skills, and gain a deeper understanding of your software. Embrace the challenge of debugging, and you’ll become a more proficient and valuable programmer.
As you continue to develop your debugging skills, keep exploring new tools and techniques. The field of software development is constantly evolving, and staying up-to-date with the latest debugging methodologies will help you tackle even the most complex coding challenges with ease.