Effective Strategies for Dealing with Legacy Code: A Comprehensive Guide
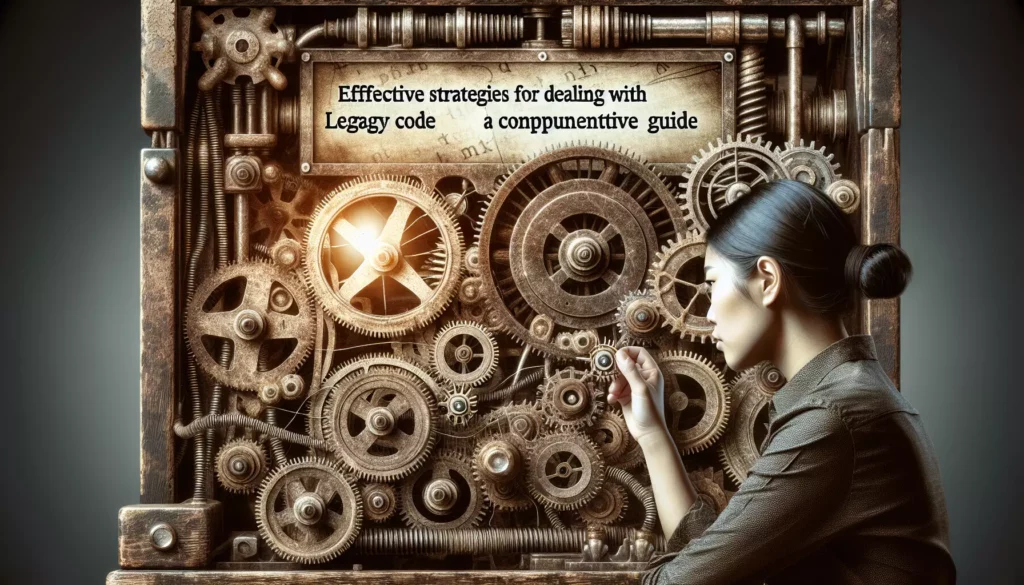
In the ever-evolving world of software development, legacy code is an inevitable reality that developers must face. Whether you’re a seasoned programmer or just starting your journey in coding, understanding how to effectively deal with legacy code is a crucial skill. This comprehensive guide will explore various strategies and best practices for managing, refactoring, and improving legacy code, helping you navigate the challenges it presents.
What is Legacy Code?
Before diving into strategies, let’s define what we mean by legacy code. Legacy code typically refers to source code that:
- Is inherited from previous developers or teams
- Uses outdated technologies or programming paradigms
- Lacks proper documentation or test coverage
- Is difficult to maintain, understand, or modify
- May be mission-critical to the organization
Legacy code often presents unique challenges, such as increased maintenance costs, decreased productivity, and higher risk of introducing bugs when making changes. However, with the right approach, these challenges can be overcome.
1. Understand the Existing Codebase
The first step in dealing with legacy code is to gain a thorough understanding of the existing codebase. This involves:
Code Analysis
Use static code analysis tools to get an overview of the codebase structure, dependencies, and potential issues. Tools like SonarQube, ESLint, or language-specific analyzers can provide valuable insights.
Documentation Review
If available, review any existing documentation, comments, or README files. While often outdated, these can still provide context and historical information about the codebase.
Version Control History
Examine the version control history to understand how the code has evolved over time. This can help identify key changes, contributors, and the reasons behind certain design decisions.
2. Establish a Testing Framework
Before making any changes to legacy code, it’s crucial to have a safety net in place. This is where testing comes in:
Write Characterization Tests
Characterization tests capture the current behavior of the system, even if that behavior is not ideal. These tests serve as a baseline to ensure that changes don’t inadvertently alter existing functionality.
Implement Unit Tests
As you work on specific parts of the code, write unit tests to cover individual functions or components. This will help prevent regressions and make future changes easier.
Set Up Continuous Integration
Implement a CI/CD pipeline to automatically run tests on every code change. This ensures that new modifications don’t break existing functionality.
3. Refactoring Techniques
Refactoring is the process of restructuring existing code without changing its external behavior. Here are some effective refactoring techniques for legacy code:
Extract Method
Break down large, complex functions into smaller, more manageable pieces. This improves readability and makes the code easier to test and maintain.
// Before refactoring
function processOrder(order) {
// 100 lines of code doing multiple things
}
// After refactoring
function processOrder(order) {
validateOrder(order);
calculateTotalPrice(order);
applyDiscounts(order);
createInvoice(order);
sendConfirmationEmail(order);
}
function validateOrder(order) {
// Validation logic
}
function calculateTotalPrice(order) {
// Price calculation logic
}
// ... other extracted methods
Introduce Parameter Object
When a method has too many parameters, group related parameters into a single object. This simplifies method signatures and makes the code more maintainable.
// Before refactoring
function createUser(name, email, age, address, phone) {
// User creation logic
}
// After refactoring
function createUser(userDetails) {
const { name, email, age, address, phone } = userDetails;
// User creation logic
}
// Usage
createUser({
name: "John Doe",
email: "john@example.com",
age: 30,
address: "123 Main St",
phone: "555-1234"
});
Replace Conditional with Polymorphism
Convert complex conditional statements into a more object-oriented structure using polymorphism. This can make the code more extensible and easier to maintain.
// Before refactoring
function calculateShippingCost(packageType, weight) {
if (packageType === "standard") {
return weight * 0.5;
} else if (packageType === "express") {
return weight * 1.5;
} else if (packageType === "overnight") {
return weight * 2.5;
}
}
// After refactoring
class ShippingCalculator {
calculateCost(weight) {
throw new Error("Method not implemented");
}
}
class StandardShipping extends ShippingCalculator {
calculateCost(weight) {
return weight * 0.5;
}
}
class ExpressShipping extends ShippingCalculator {
calculateCost(weight) {
return weight * 1.5;
}
}
class OvernightShipping extends ShippingCalculator {
calculateCost(weight) {
return weight * 2.5;
}
}
// Usage
const calculator = getShippingCalculator(packageType);
const cost = calculator.calculateCost(weight);
4. Improve Code Organization
Organizing legacy code can significantly improve its maintainability and readability. Consider the following strategies:
Apply the Single Responsibility Principle
Ensure that each class or module has a single, well-defined responsibility. This makes the code easier to understand, test, and modify.
Use Design Patterns
Implement appropriate design patterns to solve common architectural problems. Patterns like Factory, Strategy, or Observer can help improve code structure and flexibility.
Modularize the Codebase
Break down the monolithic codebase into smaller, more manageable modules or microservices. This can improve scalability and make it easier to work on specific parts of the system.
5. Modernize Dependencies and Technologies
Legacy code often relies on outdated libraries or technologies. Modernizing these dependencies can bring significant benefits:
Upgrade Libraries and Frameworks
Gradually update third-party libraries and frameworks to their latest stable versions. This can improve performance, security, and access to new features.
Migrate to Modern Language Features
If the legacy code is written in an older version of a programming language, consider migrating to newer language features. For example, if working with JavaScript, you might update from ES5 to ES6+ syntax.
// ES5
var sum = function(a, b) {
return a + b;
};
// ES6+
const sum = (a, b) => a + b;
Adopt Contemporary Best Practices
Implement modern development practices such as containerization, infrastructure as code, or serverless architectures where appropriate.
6. Documentation and Knowledge Transfer
Proper documentation is crucial when dealing with legacy code. It helps preserve knowledge and makes it easier for future developers to understand and maintain the system.
Create Technical Documentation
Document the system architecture, key components, and important workflows. Use tools like draw.io or Mermaid to create visual diagrams.
Implement Code Comments
Add meaningful comments to explain complex logic, non-obvious decisions, or potential pitfalls. However, avoid over-commenting or stating the obvious.
// Good comment
// Calculate the discount based on the customer's loyalty tier
// Platinum: 20%, Gold: 15%, Silver: 10%, Bronze: 5%
function calculateDiscount(customer) {
// Implementation
}
// Avoid comments like this
// Increment i by 1
i++;
Maintain a Knowledge Base
Create and maintain a centralized knowledge base or wiki that contains information about the system, common issues, and their solutions.
7. Gradual Rewrite vs. Complete Overhaul
When dealing with legacy code, you’ll often face the decision of whether to gradually improve the existing codebase or completely rewrite the system. Both approaches have their merits and drawbacks:
Gradual Rewrite
Pros:
- Lower risk and cost
- Continuous delivery of improvements
- Easier to manage alongside ongoing maintenance
Cons:
- Slower overall progress
- May perpetuate some existing architectural issues
- Requires careful planning to avoid creating a hybrid system
Complete Overhaul
Pros:
- Opportunity to redesign the entire system with modern best practices
- Can lead to significant improvements in performance and maintainability
- Allows for a clean break from legacy technologies
Cons:
- Higher risk and cost
- Longer time to deliver value
- May introduce new bugs or miss important edge cases handled by the legacy system
The decision between these approaches depends on factors such as the size of the codebase, available resources, business priorities, and the overall health of the existing system.
8. Performance Optimization
Legacy code often suffers from performance issues due to outdated algorithms or inefficient data structures. Here are some strategies to improve performance:
Profiling and Benchmarking
Use profiling tools to identify performance bottlenecks in the code. This can help you focus your optimization efforts on the areas that will have the most significant impact.
Optimize Database Queries
Review and optimize database queries, especially in data-intensive applications. This may involve adding indexes, denormalizing data, or using caching mechanisms.
Implement Caching
Use caching strategies to reduce the load on your system and improve response times. This can be particularly effective for frequently accessed, computationally expensive, or slow-changing data.
// Example of a simple memoization cache in JavaScript
const memoize = (fn) => {
const cache = new Map();
return (...args) => {
const key = JSON.stringify(args);
if (cache.has(key)) {
return cache.get(key);
}
const result = fn(...args);
cache.set(key, result);
return result;
};
};
// Usage
const expensiveCalculation = memoize((x, y) => {
// Simulating a time-consuming calculation
let result = 0;
for (let i = 0; i < 1000000; i++) {
result += x * y;
}
return result;
});
console.time("First call");
expensiveCalculation(5, 10);
console.timeEnd("First call");
console.time("Second call (cached)");
expensiveCalculation(5, 10);
console.timeEnd("Second call (cached)");
9. Security Considerations
Legacy code may contain security vulnerabilities due to outdated practices or unpatched dependencies. Address security concerns by:
Conducting Security Audits
Regularly perform security audits of the codebase to identify potential vulnerabilities. Use tools like OWASP ZAP or Snyk to scan for common security issues.
Updating Dependencies
Keep all dependencies up to date, especially those with known security vulnerabilities. Use tools like npm audit (for Node.js projects) to identify and fix security issues in dependencies.
Implementing Secure Coding Practices
Apply secure coding practices such as input validation, proper error handling, and secure authentication mechanisms. Educate the development team on common security pitfalls and how to avoid them.
10. Managing Technical Debt
Legacy code often accumulates technical debt over time. Here are strategies to manage and reduce technical debt:
Prioritize Debt Repayment
Identify areas of the codebase with the highest technical debt and prioritize their improvement. Focus on changes that will have the most significant impact on maintainability and development speed.
Boy Scout Rule
Encourage developers to follow the “Boy Scout Rule”: Leave the code better than you found it. This means making small improvements whenever working on a piece of code, gradually reducing technical debt over time.
Allocate Time for Refactoring
Set aside dedicated time for refactoring and improving the codebase. This can be a percentage of each sprint or specific refactoring sprints.
Conclusion
Dealing with legacy code is a common challenge in software development, but with the right strategies and mindset, it can be managed effectively. By understanding the existing codebase, establishing a robust testing framework, applying refactoring techniques, and gradually modernizing the system, you can transform legacy code into a more maintainable and efficient codebase.
Remember that improving legacy code is often an ongoing process rather than a one-time task. It requires patience, persistence, and a commitment to continuous improvement. By following the strategies outlined in this guide, you’ll be well-equipped to tackle legacy code challenges and contribute to the long-term success of your software projects.
As you apply these strategies, keep in mind that every codebase is unique, and what works best may vary depending on your specific situation. Be prepared to adapt these approaches to fit your team’s needs and the particular challenges of your legacy system. With practice and experience, you’ll develop a keen sense for when and how to apply these techniques most effectively.