Edit Distance (Levenshtein Distance): A Comprehensive Guide
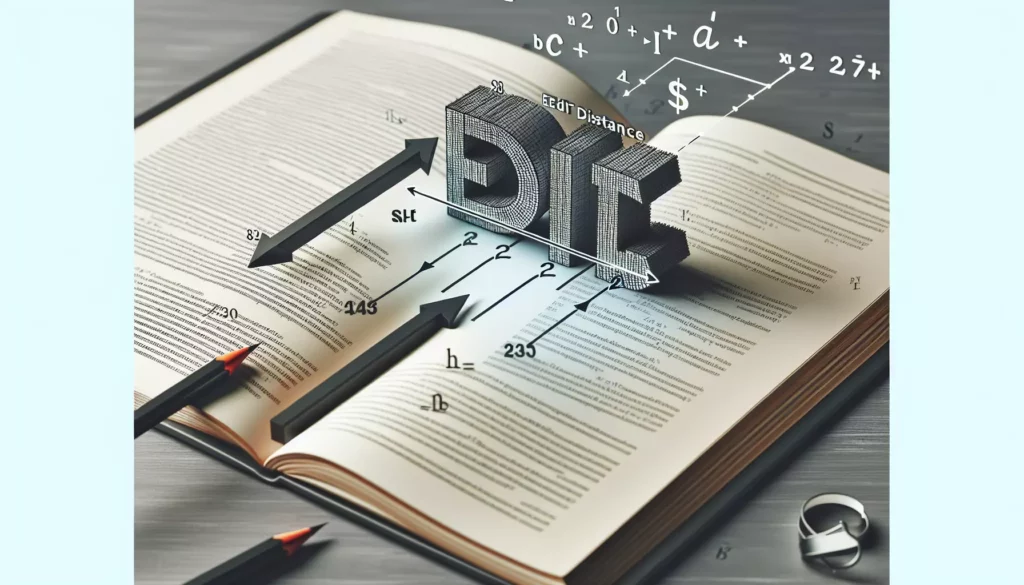
In the world of computer science and algorithmic problem-solving, the concept of Edit Distance, also known as Levenshtein Distance, plays a crucial role in various applications. This powerful algorithm is essential for anyone looking to enhance their coding skills, especially those preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll dive deep into the Edit Distance algorithm, exploring its implementation, applications, and significance in the realm of programming.
What is Edit Distance?
Edit Distance, or Levenshtein Distance, is a measure of the similarity between two strings. It quantifies how many single-character edits (insertions, deletions, or substitutions) are needed to transform one string into another. This concept was introduced by Vladimir Levenshtein in 1965 and has since become a fundamental algorithm in computer science.
For example, the Edit Distance between “kitten” and “sitting” is 3, as it takes three edits to transform “kitten” into “sitting”:
- Replace ‘k’ with ‘s’
- Replace ‘e’ with ‘i’
- Insert ‘g’ at the end
Understanding the Algorithm
The Edit Distance algorithm uses dynamic programming to efficiently calculate the minimum number of edits required. Let’s break down the steps:
- Create a matrix with dimensions (m+1) x (n+1), where m and n are the lengths of the two strings.
- Initialize the first row and column of the matrix.
- Fill the rest of the matrix using the following rule:
- If the characters match, copy the value from the diagonal.
- If they don’t match, take the minimum of the three adjacent cells and add 1.
- The value in the bottom-right cell of the matrix is the Edit Distance.
Implementing Edit Distance in Python
Let’s implement the Edit Distance algorithm in Python:
def edit_distance(str1, str2):
m, n = len(str1), len(str2)
dp = [[0] * (n + 1) for _ in range(m + 1)]
# Initialize first row and column
for i in range(m + 1):
dp[i][0] = i
for j in range(n + 1):
dp[0][j] = j
# Fill the matrix
for i in range(1, m + 1):
for j in range(1, n + 1):
if str1[i-1] == str2[j-1]:
dp[i][j] = dp[i-1][j-1]
else:
dp[i][j] = 1 + min(dp[i-1][j], # Deletion
dp[i][j-1], # Insertion
dp[i-1][j-1]) # Substitution
return dp[m][n]
# Example usage
print(edit_distance("kitten", "sitting")) # Output: 3
This implementation creates a 2D matrix (dp) to store intermediate results. The final Edit Distance is found in the bottom-right cell of the matrix.
Time and Space Complexity
The time complexity of this algorithm is O(mn), where m and n are the lengths of the two strings. This is because we need to fill each cell in the m x n matrix. The space complexity is also O(mn) due to the 2D matrix used to store intermediate results.
Optimizing Space Complexity
We can optimize the space complexity to O(min(m,n)) by only keeping track of the current and previous rows in the matrix. Here’s an optimized version:
def edit_distance_optimized(str1, str2):
m, n = len(str1), len(str2)
# Ensure str1 is the shorter string
if m > n:
return edit_distance_optimized(str2, str1)
# Initialize current row
current_row = list(range(n + 1))
for i in range(1, m + 1):
previous_row, current_row = current_row, [i] + [0] * n
for j in range(1, n + 1):
if str1[i-1] == str2[j-1]:
current_row[j] = previous_row[j-1]
else:
current_row[j] = 1 + min(previous_row[j], # Deletion
current_row[j-1], # Insertion
previous_row[j-1]) # Substitution
return current_row[n]
# Example usage
print(edit_distance_optimized("kitten", "sitting")) # Output: 3
This optimized version reduces the space complexity to O(min(m,n)) while maintaining the same time complexity of O(mn).
Applications of Edit Distance
The Edit Distance algorithm has numerous practical applications across various domains:
- Spell Checking: It can suggest corrections for misspelled words by finding words with the smallest Edit Distance.
- DNA Sequence Alignment: In bioinformatics, it’s used to compare DNA sequences and identify similarities.
- Plagiarism Detection: It can help identify similarities between texts, potentially indicating plagiarism.
- Fuzzy String Matching: Useful in search engines and databases for finding approximate matches.
- Natural Language Processing: It’s used in various NLP tasks, including machine translation and speech recognition.
Variations of Edit Distance
There are several variations of the Edit Distance algorithm, each with its own specific use cases:
1. Hamming Distance
Hamming Distance is a simpler version that only considers substitution operations and works on strings of equal length. It’s often used in information theory and error detection.
def hamming_distance(str1, str2):
if len(str1) != len(str2):
raise ValueError("Strings must be of equal length")
return sum(c1 != c2 for c1, c2 in zip(str1, str2))
# Example usage
print(hamming_distance("karolin", "kathrin")) # Output: 3
2. Damerau-Levenshtein Distance
This variation extends the Levenshtein Distance by including transposition of adjacent characters as a valid operation. It’s particularly useful for spell checking.
def damerau_levenshtein_distance(str1, str2):
d = {}
lenstr1 = len(str1)
lenstr2 = len(str2)
for i in range(-1, lenstr1 + 1):
d[(i, -1)] = i + 1
for j in range(-1, lenstr2 + 1):
d[(-1, j)] = j + 1
for i in range(lenstr1):
for j in range(lenstr2):
if str1[i] == str2[j]:
cost = 0
else:
cost = 1
d[(i, j)] = min(
d[(i - 1, j)] + 1, # Deletion
d[(i, j - 1)] + 1, # Insertion
d[(i - 1, j - 1)] + cost # Substitution
)
if i > 0 and j > 0 and str1[i] == str2[j - 1] and str1[i - 1] == str2[j]:
d[(i, j)] = min(d[(i, j)], d[i - 2, j - 2] + cost) # Transposition
return d[lenstr1 - 1, lenstr2 - 1]
# Example usage
print(damerau_levenshtein_distance("ca", "abc")) # Output: 2
Edit Distance in Technical Interviews
The Edit Distance algorithm is a popular topic in technical interviews, especially at major tech companies. Here are some tips for tackling Edit Distance problems in interviews:
- Understand the problem: Make sure you grasp what the question is asking. Is it a standard Edit Distance problem, or a variation?
- Start with a brute force approach: Begin by explaining a recursive solution, even if it’s not optimal. This shows your problem-solving process.
- Optimize with dynamic programming: Explain how you can use memoization or tabulation to optimize the solution.
- Consider space optimization: If asked, discuss how you can optimize the space complexity.
- Analyze time and space complexity: Be prepared to discuss the complexity of your solution.
- Handle edge cases: Consider what happens with empty strings or strings of very different lengths.
- Implement cleanly: Write clean, well-commented code that’s easy to understand.
- Test your solution: Provide test cases and walk through your code to ensure it works correctly.
Practice Problems
To further enhance your understanding and prepare for interviews, here are some practice problems related to Edit Distance:
- One Edit Distance: Given two strings, determine if they are one edit distance apart.
- Delete Operation for Two Strings: Find the minimum number of steps required to make two strings the same.
- Minimum ASCII Delete Sum for Two Strings: Find the lowest ASCII sum of deleted characters to make two strings equal.
- Longest Common Subsequence: Find the length of the longest subsequence present in both strings.
- Wildcard Matching: Implement wildcard pattern matching with support for ‘?’ and ‘*’.
Conclusion
The Edit Distance algorithm is a fundamental concept in computer science with wide-ranging applications. Understanding this algorithm and its variations is crucial for anyone looking to excel in coding interviews and develop strong problem-solving skills.
As you continue your journey in coding education and skills development, remember that mastering algorithms like Edit Distance is just one piece of the puzzle. Keep practicing, exploring new concepts, and applying your knowledge to real-world problems. Platforms like AlgoCademy can provide valuable resources and guidance as you progress from beginner-level coding to tackling complex algorithmic challenges.
By thoroughly understanding Edit Distance and similar dynamic programming concepts, you’ll be well-prepared for technical interviews at major tech companies and equipped to solve a wide range of programming problems. Keep honing your skills, and don’t hesitate to dive deep into the intricacies of algorithms – it’s the key to becoming a proficient and confident programmer.