Dynamic Programming Simplified: What You Need to Know
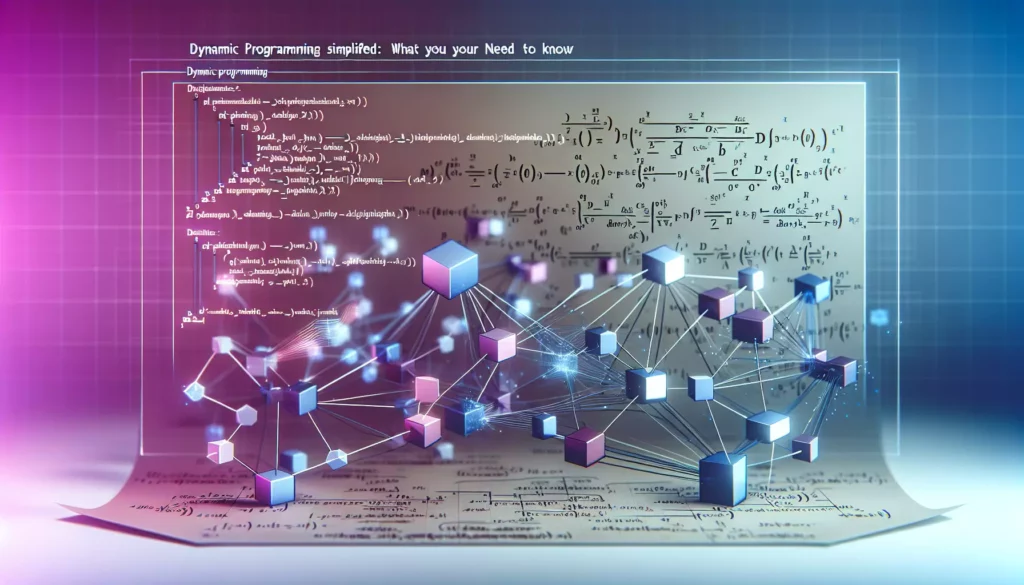
Dynamic Programming (DP) is a powerful problem-solving technique that can significantly improve the efficiency of algorithms. It’s a method that breaks down complex problems into smaller subproblems and stores the results for future use. While it may seem daunting at first, understanding dynamic programming can be a game-changer in your coding journey, especially when preparing for technical interviews at top tech companies.
In this comprehensive guide, we’ll demystify dynamic programming, explore its core concepts, and walk through several examples to help you grasp this essential technique. Whether you’re a beginner looking to expand your problem-solving toolkit or an experienced programmer aiming to refine your skills, this article will provide valuable insights into the world of dynamic programming.
Table of Contents
- What is Dynamic Programming?
- Key Concepts in Dynamic Programming
- When to Use Dynamic Programming
- Top-Down vs. Bottom-Up Approaches
- Dynamic Programming Examples
- Common Dynamic Programming Patterns
- Optimizing Dynamic Programming Solutions
- Practice Problems and Resources
- Conclusion
1. What is Dynamic Programming?
Dynamic Programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is a method for solving optimization problems by caching subproblem solutions to avoid redundant computations. The term “dynamic programming” was coined by Richard Bellman in the 1950s, although the core ideas have been around for much longer.
At its essence, dynamic programming is about:
- Identifying overlapping subproblems
- Storing solutions to these subproblems
- Reusing these solutions to build the final answer
The “programming” in dynamic programming refers to a tabular method, not to writing computer code. It’s about filling in a table (or an array) with solutions to subproblems, which can then be combined to solve the original problem.
2. Key Concepts in Dynamic Programming
To effectively use dynamic programming, it’s crucial to understand its fundamental concepts:
Optimal Substructure
A problem has optimal substructure if an optimal solution can be constructed from optimal solutions of its subproblems. This property is essential for dynamic programming to work. It means that the overall optimal solution can be built from the optimal solutions of smaller instances of the same problem.
Overlapping Subproblems
In dynamic programming, the same subproblems are solved multiple times. By storing the results of these subproblems, we can avoid redundant computations and significantly improve the efficiency of our algorithm.
Memoization
Memoization is the technique of storing the results of expensive function calls and returning the cached result when the same inputs occur again. In dynamic programming, this often involves using a data structure (like an array or a hash table) to store computed results.
State
The state in dynamic programming represents the current situation or condition of the problem. It encapsulates all the information needed to make a decision at a particular point in the problem-solving process.
Transition
Transitions define how we move from one state to another. In dynamic programming, this often involves defining recurrence relations that show how the solution to a larger problem can be expressed in terms of solutions to smaller subproblems.
3. When to Use Dynamic Programming
Dynamic programming is particularly useful for solving problems with the following characteristics:
- Overlapping subproblems: The problem can be broken down into subproblems which are reused several times.
- Optimal substructure: An optimal solution to the problem can be constructed from optimal solutions of its subproblems.
- The problem involves making a series of interconnected choices.
- The problem asks for optimization (minimization or maximization) of a certain quantity.
- The problem involves computing the number of ways to do something.
Common problem types that often lend themselves to dynamic programming solutions include:
- Fibonacci sequence and its variations
- Longest Common Subsequence
- Knapsack problems
- Matrix Chain Multiplication
- Shortest Path problems
- Edit Distance
- Coin Change problems
4. Top-Down vs. Bottom-Up Approaches
There are two main approaches to implementing dynamic programming solutions: top-down and bottom-up.
Top-Down Approach (Memoization)
The top-down approach, also known as memoization, starts with the original problem and recursively breaks it down into subproblems. As each subproblem is solved, its result is stored (memoized) for future use. If the same subproblem is encountered again, the stored result is returned instead of recomputing it.
Advantages of the top-down approach:
- It’s often more intuitive and closer to the natural recursive formulation of the problem.
- It only solves subproblems that are actually needed.
- It’s easier to debug as it follows the natural thought process.
Here’s a simple example of a top-down approach for calculating Fibonacci numbers:
def fib(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fib(n-1, memo) + fib(n-2, memo)
return memo[n]
print(fib(100)) # This will compute quickly even for large n
Bottom-Up Approach (Tabulation)
The bottom-up approach, also known as tabulation, starts by solving the smallest subproblems first and works its way up to the original problem. It typically involves filling a table (array) with solutions to subproblems.
Advantages of the bottom-up approach:
- It’s usually more efficient as it avoids the overhead of recursive calls.
- It’s easier to analyze the time and space complexity.
- It can sometimes use less memory if you only need to keep track of a few previous states.
Here’s the same Fibonacci example using a bottom-up approach:
def fib(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
print(fib(100)) # This will compute quickly for large n
5. Dynamic Programming Examples
Let’s explore a few classic dynamic programming problems to better understand how to apply this technique.
Longest Common Subsequence (LCS)
The Longest Common Subsequence problem asks to find the length of the longest subsequence that is common to two sequences. This is a classic dynamic programming problem that demonstrates the power of the technique.
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
X = "ABCDGH"
Y = "AEDFHR"
print(f"Length of LCS is {lcs(X, Y)}") # Output: Length of LCS is 3
In this example, we use a 2D table to store the lengths of longest common subsequences for different prefixes of the input strings. The final answer is in the bottom-right cell of the table.
0/1 Knapsack Problem
The 0/1 Knapsack problem is another classic dynamic programming problem. Given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit and the total value is as large as possible.
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
val = [60, 100, 120]
wt = [10, 20, 30]
W = 50
n = len(val)
print(f"Maximum value: {knapsack(W, wt, val, n)}") # Output: Maximum value: 220
This solution uses a 2D table to store the maximum values for different capacities and different numbers of items. The final answer is in the bottom-right cell of the table.
Coin Change Problem
The Coin Change problem asks for the number of ways to make a certain amount of money with a given set of coin denominations. This problem demonstrates how dynamic programming can be used to solve counting problems efficiently.
def coin_change(coins, amount):
dp = [0] * (amount + 1)
dp[0] = 1
for coin in coins:
for i in range(coin, amount + 1):
dp[i] += dp[i - coin]
return dp[amount]
coins = [1, 2, 5]
amount = 5
print(f"Number of ways to make {amount} cents: {coin_change(coins, amount)}")
# Output: Number of ways to make 5 cents: 4
In this solution, we use a 1D array to store the number of ways to make different amounts. We build up the solution iteratively, considering one coin denomination at a time.
6. Common Dynamic Programming Patterns
As you solve more dynamic programming problems, you’ll start to recognize common patterns. Here are some of the most frequently encountered patterns:
Linear Sequence
This pattern involves problems where the solution for index i depends on the solutions for some previous indices. The Fibonacci sequence is a classic example of this pattern.
Grid Traversal
Problems involving traversal of a 2D grid often fall into this category. Examples include finding the number of unique paths from the top-left to the bottom-right of a grid, or calculating the minimum path sum in a grid.
String Manipulation
Many string-related problems, such as the Longest Common Subsequence, Edit Distance, or Palindrome-related problems, can be solved using dynamic programming.
Decision Making
Problems where you need to make a series of decisions to optimize some quantity often use dynamic programming. The 0/1 Knapsack problem is a prime example of this pattern.
Interval Problems
These problems involve finding optimal solutions over different intervals of a sequence. Examples include Matrix Chain Multiplication and Optimal Binary Search Tree.
Partition Problems
These problems involve dividing a set of elements into subsets to optimize some criterion. The Equal Subset Sum Partition problem is an example of this pattern.
7. Optimizing Dynamic Programming Solutions
While dynamic programming can significantly improve the time complexity of algorithms, there are often ways to further optimize DP solutions:
Space Optimization
Many DP solutions use 2D arrays to store results, but often, you only need the results from the previous row or column. In such cases, you can reduce the space complexity from O(n^2) to O(n) by using a 1D array and updating it in place.
For example, in the 0/1 Knapsack problem, we can optimize the space usage like this:
def knapsack_optimized(W, wt, val, n):
dp = [0 for i in range(W + 1)]
for i in range(n):
for w in range(W, wt[i] - 1, -1):
dp[w] = max(dp[w], dp[w - wt[i]] + val[i])
return dp[W]
val = [60, 100, 120]
wt = [10, 20, 30]
W = 50
n = len(val)
print(f"Maximum value: {knapsack_optimized(W, wt, val, n)}") # Output: Maximum value: 220
State Reduction
Sometimes, you can reduce the number of states in your DP solution by recognizing patterns or symmetries in the problem. This can lead to significant improvements in both time and space complexity.
Preprocessing
In some cases, preprocessing the input data can lead to more efficient DP solutions. For example, in problems involving strings, computing hash values or suffix arrays beforehand can speed up subsequent operations.
8. Practice Problems and Resources
To master dynamic programming, consistent practice is key. Here are some resources and practice problems to help you improve your skills:
Online Platforms
- LeetCode: Has a dedicated Dynamic Programming section with problems of varying difficulty.
- HackerRank: Offers a Dynamic Programming track with explanations and challenges.
- Codeforces: Contains many competitive programming problems, including DP challenges.
- GeeksforGeeks: Provides a comprehensive list of DP problems with detailed explanations.
Books
- “Introduction to Algorithms” by Cormen, Leiserson, Rivest, and Stein: Contains a chapter dedicated to Dynamic Programming.
- “Algorithms” by Robert Sedgewick and Kevin Wayne: Includes sections on dynamic programming with clear explanations.
- “Dynamic Programming for Coding Interviews” by Meenakshi and Kamal Rawat: Focuses specifically on DP problems commonly asked in coding interviews.
Practice Problems
Here are some classic DP problems to get you started:
- Longest Increasing Subsequence
- Edit Distance
- Maximum Subarray Sum
- Coin Change (both counting and minimizing coins)
- Longest Palindromic Subsequence
- Minimum Path Sum in a Grid
- Palindrome Partitioning
- Word Break Problem
- Rod Cutting
- Egg Dropping Puzzle
9. Conclusion
Dynamic Programming is a powerful technique that can transform seemingly intractable problems into efficiently solvable ones. By breaking down complex problems into simpler subproblems and storing their solutions, DP allows us to avoid redundant computations and dramatically improve the efficiency of our algorithms.
While mastering dynamic programming takes time and practice, the skills you develop will be invaluable in your journey as a programmer. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your problem-solving abilities, a strong foundation in dynamic programming will serve you well.
Remember, the key to success with dynamic programming is to practice regularly, start with simpler problems, and gradually work your way up to more complex ones. As you solve more problems, you’ll start to recognize common patterns and develop intuition for when and how to apply dynamic programming techniques.
Keep coding, keep learning, and don’t be discouraged if you find some DP problems challenging at first. With persistence and practice, you’ll soon find yourself confidently tackling even the most complex dynamic programming challenges!