Dynamic Programming: Mastering the Art of Efficient Problem Solving
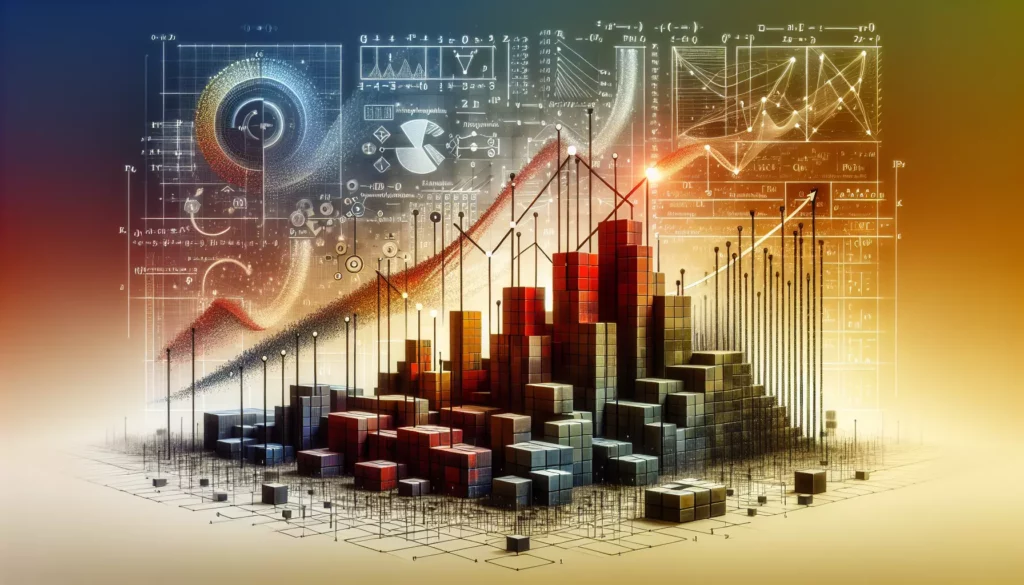
In the world of computer science and algorithmic problem-solving, dynamic programming (DP) stands out as a powerful technique that can dramatically improve the efficiency of certain algorithms. Whether you’re preparing for technical interviews at top tech companies or simply looking to enhance your coding skills, mastering dynamic programming is an essential step in your journey as a programmer. In this comprehensive guide, we’ll explore what dynamic programming is, why it’s important, and how you can master this crucial skill.
What is Dynamic Programming?
Dynamic programming is an algorithmic paradigm that solves complex problems by breaking them down into simpler subproblems. It is a method for solving optimization problems by combining the solutions to subproblems. The key idea behind dynamic programming is to store the results of subproblems so that we don’t have to recompute them when needed later.
The term “dynamic programming” was coined by Richard Bellman in the 1950s. Despite its name, it has nothing to do with dynamic computer programming. Instead, the word “dynamic” refers to the way the method works: by dynamically filling in a table with solutions to subproblems.
Key Characteristics of Dynamic Programming
- Overlapping Subproblems: The problem can be broken down into subproblems which are reused several times.
- Optimal Substructure: An optimal solution to the problem can be constructed efficiently from optimal solutions of its subproblems.
Why is Dynamic Programming Important?
Dynamic programming is crucial for several reasons:
- Efficiency: DP can significantly reduce the time complexity of algorithms, often from exponential to polynomial time.
- Optimization: It’s particularly useful for optimization problems, where we need to find the best solution among many possible ones.
- Versatility: DP can be applied to a wide range of problems in various domains, from computer science to economics.
- Interview Preparation: DP problems are common in technical interviews, especially at top tech companies like Google, Facebook, Amazon, and others.
Common Dynamic Programming Patterns
While each DP problem is unique, there are several common patterns that you’ll encounter:
1. Fibonacci Sequence
The Fibonacci sequence is a classic example of a problem that can be solved efficiently using DP. Without DP, calculating the nth Fibonacci number has a time complexity of O(2^n). With DP, we can reduce it to O(n).
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
2. Longest Common Subsequence (LCS)
The LCS problem aims to find the longest subsequence common to all sequences in a set of sequences. This problem has applications in bioinformatics and version control systems.
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
3. Knapsack Problem
The Knapsack problem is a problem in combinatorial optimization. Given a set of items, each with a weight and a value, determine the number of each item to include in a collection so that the total weight is less than or equal to a given limit and the total value is as large as possible.
def knapsack(W, wt, val, n):
K = [[0 for x in range(W + 1)] for x in range(n + 1)]
for i in range(n + 1):
for w in range(W + 1):
if i == 0 or w == 0:
K[i][w] = 0
elif wt[i-1] <= w:
K[i][w] = max(val[i-1] + K[i-1][w-wt[i-1]], K[i-1][w])
else:
K[i][w] = K[i-1][w]
return K[n][W]
How to Master Dynamic Programming
Mastering dynamic programming requires practice, patience, and a systematic approach. Here are some steps to help you on your journey:
1. Understand the Fundamentals
Before diving into complex DP problems, make sure you have a solid understanding of the basic concepts:
- Recursion
- Memoization
- Tabulation
- Time and space complexity analysis
2. Start with Simple Problems
Begin with straightforward DP problems like the Fibonacci sequence or climbing stairs problem. These will help you understand the basic principles of DP without overwhelming you with complexity.
3. Recognize DP Problems
Learn to identify problems that can be solved using DP. Look for these characteristics:
- The problem asks for the optimal value (maximum or minimum)
- The problem has overlapping subproblems
- The problem exhibits optimal substructure
4. Practice the Top-Down and Bottom-Up Approaches
DP problems can typically be solved using two approaches:
- Top-Down (Memoization): Start with the main problem and break it down into subproblems. Use recursion with memoization to store and reuse solutions to subproblems.
- Bottom-Up (Tabulation): Start by solving the smallest subproblems and use their solutions to build up to the main problem. This usually involves filling a table (hence the name tabulation).
Practice both approaches to gain a deeper understanding of DP and to be able to choose the most suitable method for each problem.
5. Solve Classic DP Problems
Work your way through classic DP problems. Some examples include:
- Longest Increasing Subsequence
- Edit Distance
- Coin Change
- Matrix Chain Multiplication
- Longest Palindromic Subsequence
6. Analyze and Optimize Your Solutions
For each problem you solve:
- Analyze the time and space complexity of your solution
- Look for ways to optimize your code
- Compare your solution with other approaches
7. Use Visualization Tools
Visualizing the DP process can greatly enhance your understanding. Use tools like:
- Pen and paper to draw out the DP table
- Online visualization tools
- Debuggers to step through your code and observe how the DP table is filled
8. Study Real-World Applications
Understanding how DP is applied in real-world scenarios can provide motivation and context. Some applications include:
- Bioinformatics (sequence alignment)
- Natural Language Processing (speech recognition)
- Resource allocation in economics
- Optimization in operations research
9. Participate in Coding Competitions
Platforms like LeetCode, HackerRank, and Codeforces often feature DP problems in their contests. Participating in these competitions can:
- Expose you to a variety of DP problems
- Help you practice solving problems under time pressure
- Allow you to learn from other programmers’ solutions
10. Teach Others
One of the best ways to solidify your understanding of DP is to explain it to others. Consider:
- Writing blog posts about DP concepts and problems
- Creating video tutorials
- Mentoring other programmers
Common Pitfalls and How to Avoid Them
As you work on mastering dynamic programming, be aware of these common pitfalls:
1. Overcomplicating the Solution
Sometimes, programmers try to apply DP to problems that don’t require it, leading to unnecessarily complex solutions. Always consider simpler alternatives first.
2. Neglecting Base Cases
Forgetting to handle base cases properly is a common mistake in DP. Make sure your base cases are correct and comprehensive.
3. Incorrect State Definition
The success of a DP solution often hinges on correctly defining the state. Take time to carefully consider what information needs to be stored in each state.
4. Inefficient State Transitions
Even with the correct state definition, inefficient transitions between states can lead to suboptimal solutions. Always look for ways to optimize your state transitions.
5. Not Considering Space Complexity
While DP can significantly improve time complexity, it often comes at the cost of increased space complexity. Always consider whether the space trade-off is acceptable for your specific use case.
Advanced Dynamic Programming Concepts
Once you’ve mastered the basics, you can explore more advanced DP concepts:
1. Bitmasking DP
Bitmasking DP uses binary representations to efficiently handle subsets in DP problems. It’s particularly useful for problems involving small sets of elements.
2. Digit DP
Digit DP is used to solve problems that involve counting numbers with certain properties. It’s often applied to problems where the constraints are given in terms of the digits of numbers.
3. DP on Trees
DP can be applied to tree structures to solve problems like finding the maximum independent set in a tree or the minimum vertex cover.
4. DP with Probability
Some DP problems involve probability calculations. These problems require a good understanding of both DP and probability theory.
5. Multi-dimensional DP
While many DP problems use 1D or 2D arrays, some complex problems require higher-dimensional DP tables.
Conclusion
Dynamic programming is a powerful technique that can significantly improve the efficiency of algorithms for a wide range of problems. Mastering DP requires dedication, practice, and a systematic approach to learning. By understanding the fundamental concepts, recognizing DP problems, practicing both top-down and bottom-up approaches, and working through classic problems, you can develop a strong foundation in this essential algorithmic technique.
Remember that becoming proficient in DP is a journey. Don’t get discouraged if you find some problems challenging at first. With persistence and practice, you’ll gradually build your skills and intuition. As you progress, you’ll find that DP not only helps you solve complex problems more efficiently but also enhances your overall problem-solving abilities as a programmer.
Whether you’re preparing for technical interviews at top tech companies or simply aiming to become a better programmer, investing time in mastering dynamic programming will undoubtedly pay off in your coding journey. So, embrace the challenge, keep practicing, and watch as your problem-solving skills reach new heights with the power of dynamic programming!