Does Learning Algorithms Matter for Beginners? A Comprehensive Guide
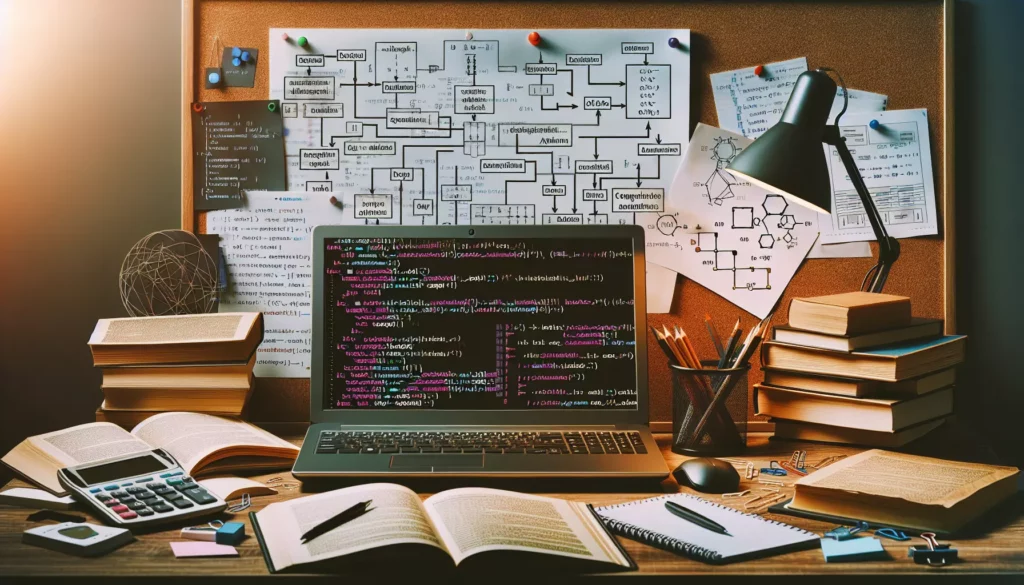
In the ever-evolving world of programming and software development, beginners often find themselves at a crossroads: should they focus on learning programming languages or dive into the complex world of algorithms? This question is particularly relevant in the context of platforms like AlgoCademy, which emphasizes algorithmic thinking and problem-solving skills. In this comprehensive guide, we’ll explore why learning algorithms matters for beginners and how it can shape your journey as a programmer.
The Importance of Algorithms in Programming
Before we delve into why algorithms matter for beginners, let’s first understand what algorithms are and their role in programming.
What Are Algorithms?
An algorithm is a step-by-step procedure or formula for solving a problem. In the context of computer science, algorithms are the foundation of all efficient solutions to computational problems. They are language-agnostic, meaning the same algorithm can be implemented in various programming languages.
The Role of Algorithms in Software Development
Algorithms play a crucial role in software development for several reasons:
- Efficiency: Well-designed algorithms can significantly improve the performance of software applications.
- Problem-solving: Algorithms provide structured approaches to solving complex problems.
- Scalability: Efficient algorithms allow applications to handle larger datasets and more users.
- Optimization: Understanding algorithms helps in optimizing code and improving resource utilization.
Why Learning Algorithms Matters for Beginners
Now that we understand what algorithms are and their importance in programming, let’s explore why beginners should consider learning algorithms early in their coding journey.
1. Developing a Problem-Solving Mindset
Learning algorithms helps beginners develop a structured approach to problem-solving. It teaches you to break down complex problems into smaller, manageable parts – a skill that is invaluable in all aspects of programming and software development.
2. Understanding Efficiency and Optimization
Algorithms introduce beginners to the concept of efficiency in programming. You learn to consider factors like time complexity and space complexity, which are crucial for writing optimized code. This understanding becomes increasingly important as you work on larger, more complex projects.
3. Preparing for Technical Interviews
Many technical interviews, especially for positions at major tech companies (often referred to as FAANG – Facebook, Amazon, Apple, Netflix, Google), focus heavily on algorithmic problem-solving. By learning algorithms early, beginners can better prepare themselves for these challenging interviews.
4. Enhancing Language-Agnostic Skills
Algorithmic thinking is not tied to any specific programming language. By focusing on algorithms, beginners develop skills that are transferable across different languages and technologies. This versatility is incredibly valuable in the ever-changing landscape of technology.
5. Building a Strong Foundation
Understanding algorithms provides a solid foundation for more advanced topics in computer science. It prepares you for concepts like data structures, design patterns, and system design – all of which are crucial for advancing in your programming career.
6. Improving Code Quality
Knowledge of algorithms often leads to writing cleaner, more efficient code. You learn to choose the right tool for the job, whether it’s selecting the appropriate sorting algorithm or implementing an efficient search method.
How to Start Learning Algorithms as a Beginner
Now that we’ve established the importance of learning algorithms, let’s look at how beginners can start their journey into the world of algorithms.
1. Start with Basic Concepts
Begin with fundamental algorithmic concepts such as:
- Time and space complexity
- Big O notation
- Basic data structures (arrays, linked lists, stacks, queues)
- Sorting and searching algorithms
2. Practice Problem-Solving
Platforms like AlgoCademy offer interactive coding tutorials and problem-solving exercises. Regularly practicing algorithmic problems helps reinforce your understanding and improves your problem-solving skills.
3. Implement Algorithms in Your Preferred Language
Try implementing common algorithms in the programming language you’re most comfortable with. This practice helps you understand how theoretical concepts translate into actual code. Here’s a simple example of a bubble sort algorithm in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
unsorted_list = [64, 34, 25, 12, 22, 11, 90]
sorted_list = bubble_sort(unsorted_list)
print("Sorted array:", sorted_list)
4. Utilize Online Resources
Take advantage of online resources like:
- MOOCs (Massive Open Online Courses) on algorithms
- YouTube tutorials
- Coding challenge websites
- Algorithm visualization tools
5. Read Books on Algorithms
While online resources are great, don’t underestimate the value of a good book. Some popular choices for beginners include:
- “Grokking Algorithms” by Aditya Bhargava
- “Introduction to Algorithms” by Thomas H. Cormen et al.
- “Algorithms” by Robert Sedgewick and Kevin Wayne
Common Algorithms Every Beginner Should Know
As you start your journey into algorithms, there are several fundamental algorithms that every beginner should familiarize themselves with:
1. Sorting Algorithms
- Bubble Sort
- Selection Sort
- Insertion Sort
- Merge Sort
- Quick Sort
2. Searching Algorithms
- Linear Search
- Binary Search
3. Graph Algorithms
- Breadth-First Search (BFS)
- Depth-First Search (DFS)
4. Dynamic Programming
- Fibonacci Sequence
- Longest Common Subsequence
5. String Manipulation Algorithms
- String Reversal
- Palindrome Check
Balancing Algorithm Learning with Practical Coding
While learning algorithms is important, it’s crucial to strike a balance between theoretical knowledge and practical coding skills. Here are some tips to maintain this balance:
1. Apply Algorithms to Real Projects
Try to incorporate the algorithms you learn into real-world projects. This practice helps you understand how algorithms are used in practical scenarios.
2. Focus on Problem-Solving
Don’t just memorize algorithms. Instead, focus on understanding the problem-solving process. This approach will help you tackle new, unfamiliar problems more effectively.
3. Learn Data Structures Alongside Algorithms
Algorithms and data structures go hand in hand. As you learn algorithms, make sure to understand the data structures they operate on. This knowledge will give you a more comprehensive understanding of efficient programming.
4. Practice Coding Regularly
While studying algorithms, continue to practice general coding. Build small projects, contribute to open-source, or solve coding challenges. This practice ensures that your practical coding skills remain sharp.
5. Understand the Limitations
Remember that while algorithms are important, they’re not everything in programming. Understanding software design principles, coding best practices, and specific technologies are also crucial for becoming a well-rounded programmer.
The Role of Platforms like AlgoCademy
Platforms like AlgoCademy play a crucial role in helping beginners learn algorithms effectively. Here’s how they contribute to the learning process:
1. Structured Learning Path
These platforms often provide a structured curriculum that takes you from basic to advanced algorithmic concepts. This guided approach ensures that you build a solid foundation before moving on to more complex topics.
2. Interactive Coding Environments
Many platforms offer interactive coding environments where you can practice implementing algorithms. This hands-on approach is crucial for reinforcing your understanding of algorithmic concepts.
3. Real-time Feedback and Assistance
Features like AI-powered assistance can provide immediate feedback on your code, helping you identify and correct mistakes quickly. This rapid feedback loop accelerates the learning process.
4. Problem Sets and Challenges
Curated problem sets and coding challenges help you apply your algorithmic knowledge to various scenarios. These exercises are often designed to mimic real interview questions, preparing you for technical interviews.
5. Community and Support
Many platforms foster a community of learners where you can discuss problems, share solutions, and learn from peers. This collaborative environment can be incredibly beneficial for beginners.
Common Misconceptions About Learning Algorithms
As you embark on your journey to learn algorithms, it’s important to address some common misconceptions:
1. “I need to be a math genius to understand algorithms”
While some algorithms involve mathematical concepts, many don’t require advanced math skills. Basic logical thinking and problem-solving abilities are often sufficient to start learning algorithms.
2. “Algorithms are only useful for competitive programming”
While algorithms are indeed crucial in competitive programming, they’re equally important in everyday software development. Efficient algorithms can significantly improve the performance and scalability of your applications.
3. “I can rely on libraries and frameworks, so I don’t need to learn algorithms”
While libraries and frameworks can handle many algorithmic tasks, understanding the underlying principles helps you choose the right tools and optimize their use. Moreover, you’ll often need to implement custom algorithms for specific problems.
4. “Learning algorithms is too difficult for beginners”
With the right approach and resources, beginners can absolutely learn algorithms. Starting with basic concepts and gradually progressing to more complex topics makes the learning process manageable and rewarding.
Conclusion: Embracing Algorithmic Thinking
In conclusion, learning algorithms does matter for beginners in programming. It develops crucial problem-solving skills, enhances your understanding of efficiency and optimization, prepares you for technical interviews, and provides a strong foundation for advanced topics in computer science.
However, it’s important to approach algorithm learning as part of a holistic programming education. Balance your study of algorithms with practical coding projects, and leverage resources like AlgoCademy to guide your learning journey.
Remember, becoming proficient in algorithms is a gradual process. Start with the basics, practice regularly, and don’t be discouraged by initial challenges. With persistence and the right resources, you’ll find that understanding algorithms not only improves your coding skills but also opens up new ways of thinking about and solving problems in the world of programming.
As you continue your programming journey, let algorithmic thinking become a powerful tool in your developer toolkit. Embrace the challenge, enjoy the problem-solving process, and watch as your skills grow and evolve. Happy coding!