Diving into Search Algorithms: Linear vs. Binary Search
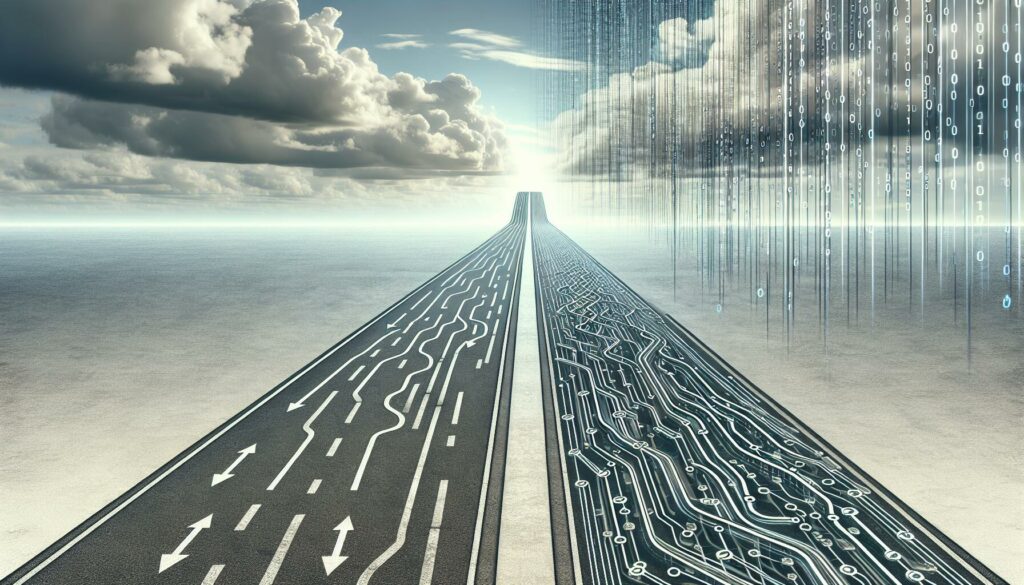
In the vast world of computer science and programming, search algorithms play a crucial role in finding specific elements within data structures. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, understanding search algorithms is essential. In this comprehensive guide, we’ll dive deep into two fundamental search algorithms: linear search and binary search. We’ll explore their implementations, compare their efficiency, and discuss when to use each one.
What are Search Algorithms?
Before we delve into the specifics of linear and binary search, let’s first understand what search algorithms are and why they’re important.
Search algorithms are step-by-step procedures designed to locate a specific item (often called the “target”) within a collection of data. These algorithms are fundamental to computer science and are used in various applications, from finding a contact in your phone’s address book to searching for products on e-commerce websites.
The efficiency of search algorithms is crucial, especially when dealing with large datasets. A well-implemented search algorithm can significantly reduce the time and computational resources required to find the desired information.
Linear Search: The Straightforward Approach
Linear search, also known as sequential search, is the simplest search algorithm. It works by checking each element in the data structure one by one until the target element is found or the end of the structure is reached.
How Linear Search Works
- Start from the first element of the array or list.
- Compare the current element with the target value.
- If they match, return the index of the current element.
- If they don’t match, move to the next element.
- Repeat steps 2-4 until the target is found or the end of the array is reached.
- If the target is not found, return a special value (e.g., -1) to indicate that the element is not present.
Implementing Linear Search in Python
Here’s a simple implementation of linear search in Python:
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i # Return the index if the target is found
return -1 # Return -1 if the target is not found
# Example usage
numbers = [4, 2, 7, 1, 9, 5, 3]
target = 7
result = linear_search(numbers, target)
if result != -1:
print(f"Target {target} found at index {result}")
else:
print(f"Target {target} not found in the array")
Time Complexity of Linear Search
The time complexity of linear search is O(n), where n is the number of elements in the array. This means that in the worst-case scenario (when the target is at the end of the array or not present), the algorithm will need to check every element once.
Advantages of Linear Search
- Simple to understand and implement
- Works on unsorted arrays
- Efficient for small datasets
- No need to preprocess the data
Disadvantages of Linear Search
- Inefficient for large datasets
- Time complexity increases linearly with the size of the input
Binary Search: Divide and Conquer
Binary search is a more efficient algorithm that works on sorted arrays. It uses a divide-and-conquer approach to repeatedly divide the search interval in half, significantly reducing the number of comparisons needed to find the target.
How Binary Search Works
- Start with the entire sorted array.
- Find the middle element of the current search range.
- Compare the middle element with the target value.
- If they match, return the index of the middle element.
- If the target is less than the middle element, repeat the search on the left half of the array.
- If the target is greater than the middle element, repeat the search on the right half of the array.
- Repeat steps 2-6 until the target is found or the search range becomes empty.
Implementing Binary Search in Python
Here’s an implementation of binary search in Python:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid # Return the index if the target is found
elif arr[mid] < target:
left = mid + 1 # Target is in the right half
else:
right = mid - 1 # Target is in the left half
return -1 # Return -1 if the target is not found
# Example usage
sorted_numbers = [1, 2, 3, 4, 5, 7, 9]
target = 7
result = binary_search(sorted_numbers, target)
if result != -1:
print(f"Target {target} found at index {result}")
else:
print(f"Target {target} not found in the array")
Time Complexity of Binary Search
The time complexity of binary search is O(log n), where n is the number of elements in the array. This logarithmic time complexity makes binary search much more efficient than linear search for large datasets.
Advantages of Binary Search
- Highly efficient for large datasets
- Logarithmic time complexity
- Requires fewer comparisons than linear search
Disadvantages of Binary Search
- Requires a sorted array
- Not suitable for unsorted data
- Slightly more complex to implement than linear search
Comparing Linear Search and Binary Search
Now that we’ve explored both linear and binary search algorithms, let’s compare them side by side to understand their strengths and weaknesses.
Aspect | Linear Search | Binary Search |
---|---|---|
Time Complexity | O(n) | O(log n) |
Data Structure | Works on sorted and unsorted arrays | Requires sorted array |
Efficiency for Large Datasets | Poor | Excellent |
Implementation Complexity | Simple | Moderate |
Space Complexity | O(1) – Constant | O(1) – Constant for iterative, O(log n) for recursive |
Best Case Scenario | O(1) – Target at the beginning | O(1) – Target at the middle |
Worst Case Scenario | O(n) – Target at the end or not present | O(log n) – Target not present |
When to Use Linear Search vs. Binary Search
Choosing the right search algorithm depends on various factors. Here are some guidelines to help you decide:
Use Linear Search When:
- The array is small (typically less than 100 elements)
- The array is unsorted, and sorting would be too costly
- You need to search through the entire array regardless of finding the target (e.g., finding all occurrences)
- The data structure doesn’t support random access (e.g., linked lists)
- Simplicity is more important than efficiency
Use Binary Search When:
- The array is large (typically more than 100 elements)
- The array is already sorted or can be efficiently sorted
- You need to perform multiple searches on the same dataset
- The data structure supports random access (e.g., arrays)
- Efficiency is crucial, especially for large datasets
Practical Examples and Use Cases
To better understand how these search algorithms are applied in real-world scenarios, let’s look at some practical examples:
1. Phone Contact List
In a phone’s contact list, names are typically sorted alphabetically. When you search for a contact, the phone likely uses a binary search algorithm to quickly find the name you’re looking for, especially if you have hundreds or thousands of contacts.
2. Dictionary Lookup
When you look up a word in a physical dictionary or a digital one, the process mimics a binary search. You start in the middle, then move to the left or right half based on alphabetical order, quickly narrowing down to the desired word.
3. Database Queries
In database management systems, binary search trees or more advanced data structures based on binary search principles are often used to index data, allowing for quick retrieval of records based on key values.
4. E-commerce Product Search
When you search for products on an e-commerce website, the system might use a combination of search algorithms. While the initial search might involve more complex algorithms, sorting and filtering results often utilize principles similar to binary search for efficient data retrieval.
5. File Systems
Operating systems use search algorithms to locate files and directories. While the exact implementation may vary, the concept of efficiently searching through a large number of files often involves principles similar to binary search.
Optimizing Search Algorithms
While linear and binary search are fundamental algorithms, there are ways to optimize them and even develop more advanced search techniques for specific use cases:
1. Jump Search
Jump search is a technique that combines elements of linear and binary search. It works on sorted arrays by jumping ahead by fixed steps and then performing a linear search. Its time complexity is O(√n), which is between linear and binary search.
2. Interpolation Search
Interpolation search improves upon binary search for uniformly distributed sorted arrays. It estimates the position of the target value, potentially reducing the number of comparisons. In the best case, it can achieve O(log log n) time complexity.
3. Exponential Search
Exponential search is useful when searching unbounded or infinite lists. It works by exponentially increasing the search range and then performing a binary search within that range. It’s particularly efficient when the target is closer to the beginning of the list.
4. Hashing
While not a traditional search algorithm, hashing provides a way to achieve average-case O(1) time complexity for search operations. However, it requires additional space and careful implementation to handle collisions.
Implementing Search Algorithms in Different Programming Languages
To give you a broader perspective, let’s look at how linear and binary search can be implemented in different programming languages:
Java Implementation
Linear Search in Java:
public class LinearSearch {
public static int linearSearch(int[] arr, int target) {
for (int i = 0; i < arr.length; i++) {
if (arr[i] == target) {
return i;
}
}
return -1;
}
public static void main(String[] args) {
int[] numbers = {4, 2, 7, 1, 9, 5, 3};
int target = 7;
int result = linearSearch(numbers, target);
if (result != -1) {
System.out.println("Target " + target + " found at index " + result);
} else {
System.out.println("Target " + target + " not found in the array");
}
}
}
Binary Search in Java:
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
public static void main(String[] args) {
int[] sortedNumbers = {1, 2, 3, 4, 5, 7, 9};
int target = 7;
int result = binarySearch(sortedNumbers, target);
if (result != -1) {
System.out.println("Target " + target + " found at index " + result);
} else {
System.out.println("Target " + target + " not found in the array");
}
}
}
JavaScript Implementation
Linear Search in JavaScript:
function linearSearch(arr, target) {
for (let i = 0; i < arr.length; i++) {
if (arr[i] === target) {
return i;
}
}
return -1;
}
const numbers = [4, 2, 7, 1, 9, 5, 3];
const target = 7;
const result = linearSearch(numbers, target);
if (result !== -1) {
console.log(`Target ${target} found at index ${result}`);
} else {
console.log(`Target ${target} not found in the array`);
}
Binary Search in JavaScript:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
const mid = Math.floor((left + right) / 2);
if (arr[mid] === target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
const sortedNumbers = [1, 2, 3, 4, 5, 7, 9];
const target = 7;
const result = binarySearch(sortedNumbers, target);
if (result !== -1) {
console.log(`Target ${target} found at index ${result}`);
} else {
console.log(`Target ${target} not found in the array`);
}
Common Pitfalls and Best Practices
When implementing search algorithms, it’s important to be aware of common pitfalls and follow best practices:
Pitfalls to Avoid:
- Using binary search on an unsorted array
- Not handling edge cases (e.g., empty arrays, single-element arrays)
- Integer overflow in the calculation of the middle index in binary search
- Incorrect handling of the search range in binary search
- Using linear search for large, frequently searched datasets
Best Practices:
- Always verify that the array is sorted before applying binary search
- Use appropriate data types to handle the size of your dataset
- Consider the trade-offs between time and space complexity
- Test your implementation with various input sizes and edge cases
- Use built-in library functions when available for optimal performance
- Document your code, explaining the algorithm and any specific implementation details
Conclusion
Understanding search algorithms is crucial for any programmer, whether you’re just starting out or preparing for technical interviews at major tech companies. Linear search and binary search are fundamental algorithms that serve as building blocks for more complex search techniques.
Linear search, with its simplicity and versatility, is ideal for small datasets and unsorted arrays. Binary search, on the other hand, shines when dealing with large, sorted datasets, offering logarithmic time complexity that can significantly improve performance.
As you continue your journey in coding education and programming skills development, remember that mastering these search algorithms is just the beginning. They provide a solid foundation for understanding more advanced algorithms and data structures, which are essential for solving complex problems efficiently.
Practice implementing these algorithms in different programming languages, experiment with various input sizes, and challenge yourself to optimize your code. By doing so, you’ll not only improve your coding skills but also develop the algorithmic thinking necessary to excel in technical interviews and real-world programming challenges.
Keep exploring, keep coding, and remember that every search is an opportunity to learn and grow as a programmer!