Dive into Robotics Programming: Mastering ROS (Robot Operating System)
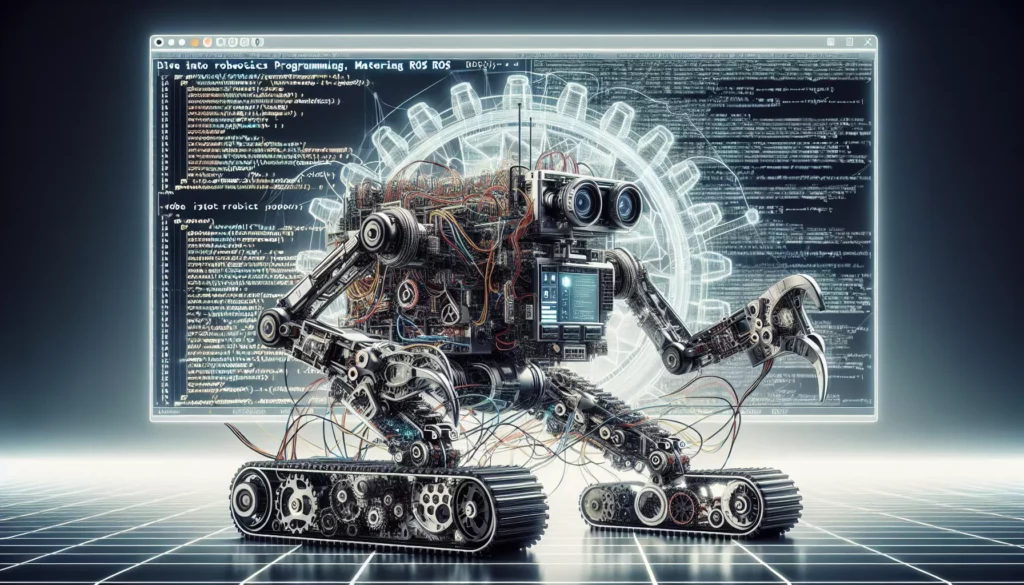
In the ever-evolving world of technology, robotics has emerged as a field that combines innovation, engineering, and programming in exciting ways. As we continue our journey through AlgoCademy’s comprehensive guide to coding education and programming skills development, it’s time to explore a fascinating and practical application of your coding skills: robotics programming. In this extensive guide, we’ll dive deep into the world of robotics programming, with a particular focus on ROS (Robot Operating System), a flexible framework for writing robot software.
Understanding Robotics Programming
Before we delve into the specifics of ROS, it’s essential to understand what robotics programming entails. Robotics programming is the process of developing software that controls and directs robots to perform specific tasks. It involves a combination of various disciplines, including:
- Computer Science
- Mechanical Engineering
- Electrical Engineering
- Mathematics
- Artificial Intelligence
The goal of robotics programming is to create intelligent machines capable of interacting with their environment, making decisions, and performing tasks autonomously or under human control.
Introduction to ROS (Robot Operating System)
ROS, or Robot Operating System, is not an operating system in the traditional sense like Windows or Linux. Instead, it’s a flexible framework for writing robot software. ROS provides a collection of tools, libraries, and conventions that aim to simplify the task of creating complex and robust robot behavior across a wide variety of robotic platforms.
Key Features of ROS
- Distributed Computing: ROS allows you to split your robot’s control system into many small, standalone programs that can run on different computers and communicate with each other.
- Modularity: You can build complex systems by combining smaller, reusable pieces of code.
- Language Independence: ROS supports multiple programming languages, including Python, C++, and Lisp.
- Tools and Visualization: ROS comes with a wide range of development and debugging tools.
- Large Community: Being open-source, ROS has a large and active community contributing to its development and providing support.
Setting Up ROS
Before we start programming with ROS, we need to set it up on our system. ROS is primarily designed to work on Unix-like platforms, with Ubuntu being the most widely supported operating system.
Installation Steps
- Choose a ROS distribution. As of 2023, ROS Noetic is the latest LTS (Long Term Support) version for ROS 1.
- Set up your Ubuntu repositories to allow “restricted,” “universe,” and “multiverse.”
- Set up your sources.list and keys:
sudo sh -c 'echo "deb http://packages.ros.org/ros/ubuntu $(lsb_release -sc) main" > /etc/apt/sources.list.d/ros-latest.list'
sudo apt-key adv --keyserver 'hkp://keyserver.ubuntu.com:80' --recv-key C1CF6E31E6BADE8868B172B4F42ED6FBAB17C654
- Update your package index:
sudo apt update
- Install ROS:
sudo apt install ros-noetic-desktop-full
- Set up your environment:
echo "source /opt/ros/noetic/setup.bash" >> ~/.bashrc
source ~/.bashrc
With these steps completed, you should have ROS installed and ready to use on your system.
ROS Concepts and Architecture
To effectively use ROS, it’s crucial to understand its core concepts and architecture. ROS operates on a graph-based architecture where processing takes place in nodes that may receive, post, and multiplex sensor, control, state, planning, actuator, and other messages.
Key Concepts in ROS
- Nodes: Nodes are processes that perform computation. A robot control system usually comprises many nodes. For example, one node controls a laser range-finder, one node controls the wheel motors, one node performs localization, one node performs path planning, etc.
- Messages: Nodes communicate with each other by passing messages. A message is simply a data structure, comprising typed fields.
- Topics: Messages are routed via a transport system with publish/subscribe semantics. A node sends out a message by publishing it to a given topic. A node that is interested in a certain kind of data will subscribe to the appropriate topic.
- Services: The publish/subscribe model is a very flexible communication paradigm, but its many-to-many, one-way transport is not appropriate for request/reply interactions, which are often required in a distributed system. Request/reply is done via services, which are defined by a pair of message structures: one for the request and one for the reply.
- Master: The ROS Master provides name registration and lookup services to the rest of the nodes in the ROS system. It tracks publishers and subscribers to topics as well as services. The role of the Master is to enable individual ROS nodes to locate one another.
- Parameter Server: The Parameter Server allows data to be stored by key in a central location. It is currently part of the Master.
Writing Your First ROS Program
Now that we understand the basic concepts of ROS, let’s write a simple ROS program. We’ll create a publisher node that sends a simple message and a subscriber node that receives and prints the message.
Creating a ROS Workspace
First, we need to create a ROS workspace:
mkdir -p ~/catkin_ws/src
cd ~/catkin_ws/
catkin_make
source devel/setup.bash
Creating a ROS Package
Next, let’s create a ROS package:
cd ~/catkin_ws/src
catkin_create_pkg beginner_tutorials std_msgs rospy roscpp
Writing the Publisher Node
Create a new file called `talker.py` in the `beginner_tutorials/scripts` directory:
#!/usr/bin/env python
import rospy
from std_msgs.msg import String
def talker():
pub = rospy.Publisher('chatter', String, queue_size=10)
rospy.init_node('talker', anonymous=True)
rate = rospy.Rate(10) # 10hz
while not rospy.is_shutdown():
hello_str = "hello world %s" % rospy.get_time()
rospy.loginfo(hello_str)
pub.publish(hello_str)
rate.sleep()
if __name__ == '__main__':
try:
talker()
except rospy.ROSInterruptException:
pass
Writing the Subscriber Node
Create another file called `listener.py` in the same directory:
#!/usr/bin/env python
import rospy
from std_msgs.msg import String
def callback(data):
rospy.loginfo(rospy.get_caller_id() + "I heard %s", data.data)
def listener():
rospy.init_node('listener', anonymous=True)
rospy.Subscriber("chatter", String, callback)
rospy.spin()
if __name__ == '__main__':
listener()
Running the Nodes
To run these nodes, first make sure they’re executable:
chmod +x talker.py listener.py
Then, in separate terminal windows:
- Start the ROS master:
roscore
- Run the talker node:
rosrun beginner_tutorials talker.py
- Run the listener node:
rosrun beginner_tutorials listener.py
You should see the talker publishing messages and the listener receiving them.
Advanced ROS Concepts
As you become more comfortable with ROS basics, you can explore more advanced concepts:
1. Launch Files
Launch files allow you to start multiple nodes at once, set parameters, and configure your ROS setup. They’re written in XML and typically have a .launch extension.
2. TF (Transform) Library
TF is a package that lets you keep track of multiple coordinate frames over time. It’s particularly useful in robotics for tasks like navigation and manipulation.
3. Action Library
The actionlib package provides a standardized interface for preemptable tasks. This is useful for tasks that take a long time to execute and may need to be cancelled or preempted.
4. URDF (Unified Robot Description Format)
URDF is an XML format for representing a robot model. It’s used to describe the physical properties of a robot, including its kinematics, dynamics, and visual elements.
5. Gazebo Simulation
Gazebo is a powerful 3D simulation environment for robots that integrates well with ROS. It allows you to test your algorithms in a simulated environment before deploying them on real hardware.
Practical Applications of ROS
ROS is used in a wide variety of robotics applications, including:
- Autonomous Vehicles: ROS is widely used in the development of self-driving cars and other autonomous vehicles.
- Industrial Robotics: Many industrial robots use ROS for tasks like pick-and-place operations, welding, and quality control.
- Service Robots: Robots designed to interact with humans, such as healthcare robots or hotel service robots, often use ROS.
- Drones and UAVs: ROS is popular in the development of unmanned aerial vehicles for tasks like surveying, photography, and delivery.
- Research and Education: Many universities and research institutions use ROS for robotics research and education.
Challenges in Robotics Programming
While robotics programming with ROS offers exciting possibilities, it also comes with its own set of challenges:
- Real-time Constraints: Many robotic applications require real-time performance, which can be challenging to achieve in a distributed system like ROS.
- Safety and Reliability: Robots often operate in environments where safety is critical, requiring robust error handling and fail-safe mechanisms.
- Sensor Integration: Integrating and calibrating various sensors (cameras, LiDAR, IMUs, etc.) can be complex.
- Motion Planning: Developing efficient algorithms for path planning and obstacle avoidance is a ongoing challenge in robotics.
- Human-Robot Interaction: Creating intuitive interfaces for humans to interact with robots is an active area of research and development.
Future of Robotics Programming
The field of robotics is rapidly evolving, and so is robotics programming. Some trends to watch out for include:
- AI and Machine Learning Integration: Increasing use of AI and machine learning algorithms in robotics for tasks like perception, decision making, and learning from experience.
- Cloud Robotics: Leveraging cloud computing for tasks that require high computational power, enabling robots to offload complex computations.
- ROS 2: The next generation of ROS, designed to address some limitations of ROS 1, particularly in areas like real-time performance and security.
- Swarm Robotics: Programming for coordinated multi-robot systems is becoming increasingly important.
- Edge Computing: As robots become more autonomous, there’s a growing need for on-board processing capabilities, leading to advancements in edge computing for robotics.
Conclusion
Robotics programming, particularly with platforms like ROS, represents an exciting frontier in the world of coding and technology. It combines many of the skills we’ve explored throughout AlgoCademy’s curriculum – from basic programming concepts to advanced algorithms and system design.
As you dive into robotics programming, you’ll find that it’s not just about writing code. It’s about bringing that code to life in the physical world, creating machines that can sense, think, and act. This field offers endless opportunities for innovation and problem-solving, whether you’re interested in developing robots for manufacturing, healthcare, exploration, or any other domain.
Remember, the journey into robotics programming is a continuous learning process. The field is constantly evolving, with new tools, techniques, and applications emerging all the time. Stay curious, keep experimenting, and don’t be afraid to tackle complex challenges. With persistence and creativity, you can contribute to shaping the future of robotics and technology.
As you continue your journey with AlgoCademy, consider how you can apply the algorithmic thinking and problem-solving skills you’ve developed to the exciting world of robotics. Who knows? Your next coding project might just walk, roll, or fly!