Dinic’s Algorithm: Mastering Maximum Flow in Network Graphs
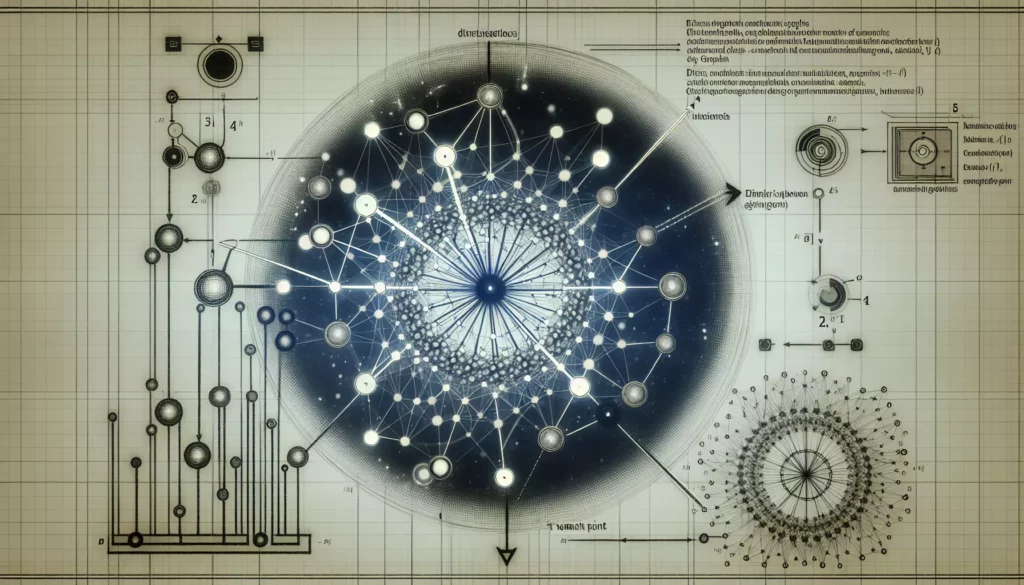
In the world of computer science and algorithms, network flow problems hold a special place due to their wide-ranging applications and intriguing complexity. Among the various algorithms designed to tackle these problems, Dinic’s Algorithm stands out as a powerful and efficient solution for finding the maximum flow in a network. In this comprehensive guide, we’ll dive deep into Dinic’s Algorithm, exploring its intricacies, implementation, and real-world applications.
Understanding Network Flow Problems
Before we delve into Dinic’s Algorithm, it’s crucial to understand the concept of network flow problems. A network flow problem involves determining the maximum amount of flow that can be pushed through a network from a source to a sink, subject to capacity constraints on the edges.
Imagine a network of pipes with varying capacities, where water flows from a source (like a reservoir) to a sink (like a water treatment plant). The goal is to find the maximum amount of water that can flow through this network without exceeding the capacity of any pipe. This analogy closely represents the essence of network flow problems in graph theory.
Key Components of a Flow Network
- Vertices (Nodes): Represent points in the network where flow can be redirected.
- Edges: Represent the connections between vertices, with each edge having a capacity.
- Source: The starting point of the flow.
- Sink: The endpoint where the flow terminates.
- Capacity: The maximum amount of flow that can pass through an edge.
- Flow: The actual amount passing through an edge, which must be less than or equal to the capacity.
Enter Dinic’s Algorithm
Dinic’s Algorithm, developed by Yefim Dinitz in 1970, is an efficient method for solving the maximum flow problem. It improves upon the Ford-Fulkerson method by introducing the concepts of level graphs and blocking flows, resulting in a significantly faster algorithm, especially for dense graphs.
Key Concepts in Dinic’s Algorithm
- Level Graph: A graph where each node is assigned a level based on its shortest distance from the source.
- Blocking Flow: A flow where every path from source to sink in the level graph contains at least one saturated edge.
- Augmenting Path: A path from source to sink in the residual graph where flow can be increased.
The Algorithm Steps
- Construct the level graph using Breadth-First Search (BFS).
- Find blocking flow in the level graph using Depth-First Search (DFS).
- Update the residual graph.
- Repeat steps 1-3 until no more augmenting paths are found.
Implementing Dinic’s Algorithm
Let’s dive into the implementation of Dinic’s Algorithm using C++. We’ll break down the code into manageable parts and explain each section in detail.
1. Setting Up the Data Structures
#include <vector>
#include <queue>
#include <limits>
const int INF = std::numeric_limits<int>::max();
struct Edge {
int v, u;
long long cap, flow;
Edge(int v, int u, long long cap) : v(v), u(u), cap(cap), flow(0) {}
};
struct Dinic {
int n, m = 0, s, t;
std::vector<Edge> edges;
std::vector<std::vector<int>> adj;
std::vector<int> level, ptr;
std::queue<int> q;
Dinic(int n, int s, int t) : n(n), s(s), t(t) {
adj.resize(n);
level.resize(n);
ptr.resize(n);
}
void add_edge(int v, int u, long long cap) {
edges.emplace_back(v, u, cap);
edges.emplace_back(u, v, 0);
adj[v].push_back(m);
adj[u].push_back(m + 1);
m += 2;
}
// ... (BFS, DFS, and max_flow functions will be added here)
};
In this setup, we define the Edge
structure to represent edges in our graph, and the Dinic
structure to encapsulate the algorithm’s functionality. The add_edge
function is used to add edges to the graph, creating both forward and backward edges for each connection.
2. Implementing the BFS for Level Graph Construction
bool bfs() {
while (!q.empty()) {
int v = q.front();
q.pop();
for (int id : adj[v]) {
if (edges[id].cap - edges[id].flow < 1)
continue;
if (level[edges[id].u] != -1)
continue;
level[edges[id].u] = level[v] + 1;
q.push(edges[id].u);
}
}
return level[t] != -1;
}
void bfs_init() {
std::fill(level.begin(), level.end(), -1);
level[s] = 0;
q.push(s);
}
The BFS function constructs the level graph by assigning levels to nodes based on their distance from the source. The bfs_init
function initializes the BFS process.
3. Implementing the DFS for Finding Blocking Flow
long long dfs(int v, long long pushed) {
if (pushed == 0)
return 0;
if (v == t)
return pushed;
for (int& cid = ptr[v]; cid < (int)adj[v].size(); cid++) {
int id = adj[v][cid];
int u = edges[id].u;
if (level[v] + 1 != level[u] || edges[id].cap - edges[id].flow < 1)
continue;
long long tr = dfs(u, std::min(pushed, edges[id].cap - edges[id].flow));
if (tr == 0)
continue;
edges[id].flow += tr;
edges[id ^ 1].flow -= tr;
return tr;
}
return 0;
}
The DFS function finds augmenting paths in the level graph and pushes flow along these paths. It uses the concept of blocking flow to efficiently find the maximum flow that can be pushed through the current level graph.
4. Putting It All Together: The Max Flow Function
long long max_flow() {
long long flow = 0;
while (true) {
bfs_init();
if (!bfs())
break;
std::fill(ptr.begin(), ptr.end(), 0);
while (long long pushed = dfs(s, INF)) {
flow += pushed;
}
}
return flow;
}
The max_flow
function orchestrates the entire process, repeatedly constructing level graphs and finding blocking flows until no more augmenting paths can be found.
Time Complexity Analysis
Dinic’s Algorithm has a time complexity of O(V²E) for general graphs, where V is the number of vertices and E is the number of edges. However, for unit capacity networks, it achieves an impressive O(min(V²/³, E½) E) time complexity, making it particularly efficient for certain types of flow problems.
Comparison with Other Max Flow Algorithms
- Ford-Fulkerson: O(EF), where F is the maximum flow. Can be slow for large capacities.
- Edmonds-Karp: O(VE²). Guaranteed to terminate but can be slow for dense graphs.
- Push-Relabel: O(V²E) or O(V³) depending on the implementation. Generally faster in practice for dense graphs.
Dinic’s Algorithm often outperforms these alternatives, especially for networks with unit capacities or when the maximum flow is not extremely large.
Real-World Applications of Dinic’s Algorithm
The efficiency and versatility of Dinic’s Algorithm make it suitable for a wide range of practical applications:
1. Network Infrastructure Optimization
In telecommunications and computer networks, Dinic’s Algorithm can be used to determine the maximum data flow between different nodes, helping in the design and optimization of network infrastructure.
2. Transportation and Logistics
The algorithm can model and solve complex transportation problems, such as finding the maximum number of vehicles that can flow through a road network or optimizing the flow of goods in a supply chain.
3. Bipartite Matching
Dinic’s Algorithm can efficiently solve bipartite matching problems, which have applications in job assignment, resource allocation, and pattern recognition.
4. Image Segmentation
In computer vision, the algorithm can be applied to image segmentation problems, helping to separate objects from backgrounds in digital images.
5. Sports Tournament Scheduling
The algorithm can be used to determine if a given tournament schedule is feasible based on constraints like venue availability and team travel times.
Advanced Techniques and Optimizations
While the basic implementation of Dinic’s Algorithm is powerful, several advanced techniques can further enhance its performance:
1. Dynamic Tree Implementation
By using dynamic trees to maintain the residual graph, the time complexity for unit capacity networks can be improved to O(VE log V).
2. Scaling Algorithm
A scaling approach can be used to handle networks with large capacities more efficiently, reducing the number of augmenting path searches.
3. Capacity Scaling
This technique involves initially considering only the high-capacity edges and gradually including lower-capacity edges, potentially speeding up the algorithm for networks with varying edge capacities.
4. Parallel Implementation
Dinic’s Algorithm can be parallelized to take advantage of multi-core processors, significantly speeding up computations for large networks.
Common Pitfalls and How to Avoid Them
When implementing Dinic’s Algorithm, be aware of these common issues:
- Integer Overflow: Use long long for flow values to handle large capacities.
- Not Resetting Data Structures: Ensure proper initialization of level and ptr arrays between iterations.
- Incorrect Edge Representation: Always create both forward and backward edges for each connection.
- Inefficient BFS Implementation: Use a queue for BFS to maintain O(E) time complexity.
Practice Problems to Master Dinic’s Algorithm
To truly grasp Dinic’s Algorithm, practice is key. Here are some recommended problems from various online judges:
- Petya and Graph (Codeforces)
- Fast Maximum Flow (SPOJ)
- Sabotage (UVa Online Judge)
- Maximum Flow (HackerRank)
Conclusion
Dinic’s Algorithm is a powerful tool in the arsenal of any computer scientist or programmer dealing with network flow problems. Its efficiency and versatility make it an essential algorithm to master, particularly for those preparing for technical interviews at top tech companies.
By understanding the core concepts, implementing the algorithm, and practicing with real-world problems, you’ll not only enhance your problem-solving skills but also gain valuable insights into graph theory and network optimization. As you continue your journey in algorithm mastery, remember that Dinic’s Algorithm is just one piece of the puzzle – there’s always more to learn and explore in the fascinating world of computer science.
Keep coding, keep learning, and may your flows always be maximum!