Different Types of Technical Interviews: A Comprehensive Guide
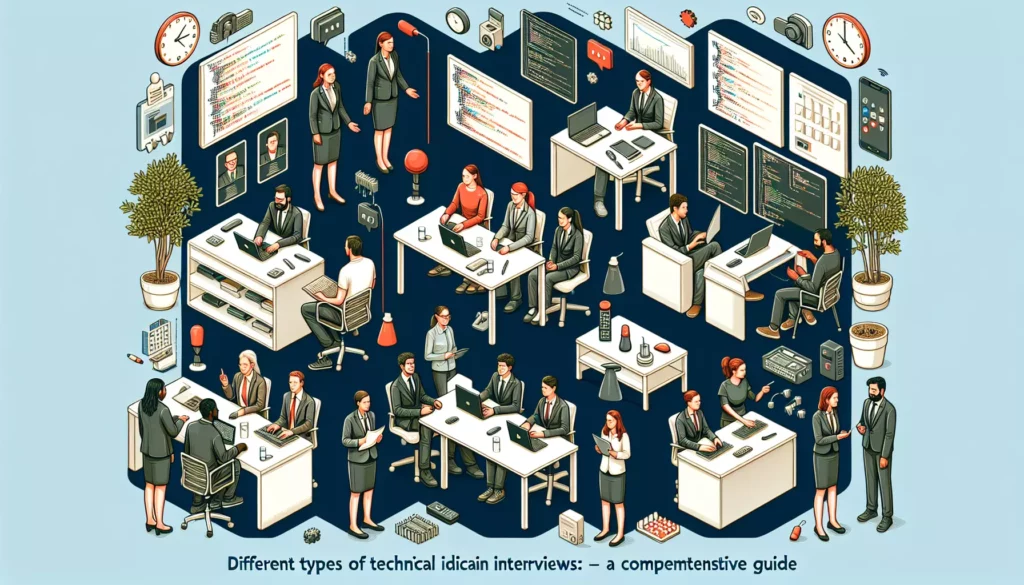
In the competitive world of software engineering, securing a position at a top tech company often involves navigating through various types of technical interviews. Whether you’re a fresh graduate or an experienced developer looking to make a career move, understanding the different interview formats can significantly boost your chances of success. This comprehensive guide will walk you through the most common types of technical interviews, with a particular focus on those used by major tech companies, often referred to as FAANG (Facebook/Meta, Amazon, Apple, Netflix, Google) and other industry leaders.
1. Coding Interviews
Coding interviews are perhaps the most common and feared type of technical interview. They’re designed to assess your problem-solving skills, coding proficiency, and ability to think on your feet.
What to Expect:
- You’ll be presented with one or more algorithmic problems to solve.
- You may be asked to write code on a whiteboard, in a shared online editor, or on paper.
- Time constraints are usually tight, ranging from 30 minutes to an hour per problem.
- You’ll need to explain your thought process and approach as you code.
How to Prepare:
- Practice coding problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Focus on data structures and algorithms.
- Learn to communicate your thought process clearly.
- Practice writing clean, efficient code under time pressure.
Here’s a simple example of a coding question you might encounter:
// Write a function that reverses a string. The input string is given as an array of characters s.
// You must do this by modifying the input array in-place with O(1) extra memory.
function reverseString(s: string[]): void {
let left = 0;
let right = s.length - 1;
while (left < right) {
// Swap characters
[s[left], s[right]] = [s[right], s[left]];
left++;
right--;
}
};
2. Take-Home Coding Challenges
Take-home coding challenges are becoming increasingly popular as they allow candidates to showcase their skills in a more relaxed environment.
What to Expect:
- You’ll be given a project or problem to solve within a specified timeframe (usually 1-7 days).
- The challenge may involve building a small application, implementing a specific feature, or solving a complex algorithmic problem.
- You’ll typically have access to your preferred development environment and resources.
How to Prepare:
- Practice time management to ensure you complete all requirements within the given timeframe.
- Focus on writing clean, well-documented, and easily maintainable code.
- Pay attention to edge cases and error handling.
- Include tests to demonstrate the robustness of your solution.
A take-home challenge might look something like this:
// Implement a RESTful API for a simple todo list application. The API should support the following operations:
// - Create a new todo item
// - Retrieve all todo items
// - Update an existing todo item
// - Delete a todo item
// Use any programming language and framework of your choice. Include appropriate tests and documentation.
// Example Todo Item structure:
// {
// "id": 1,
// "title": "Buy groceries",
// "completed": false,
// "createdAt": "2023-06-01T10:00:00Z"
// }
3. System Design Interviews
System design interviews are crucial for senior-level positions and assess your ability to design large-scale distributed systems.
What to Expect:
- You’ll be asked to design a complex system (e.g., a social media platform, a ride-sharing app, or a distributed file storage system).
- The focus is on high-level architecture, scalability, and trade-offs.
- You’ll need to ask clarifying questions and make assumptions about requirements.
- The interviewer may dive deeper into specific components of your design.
How to Prepare:
- Study common system design patterns and architectures.
- Understand concepts like load balancing, caching, database sharding, and microservices.
- Practice estimating system requirements (e.g., storage, bandwidth).
- Learn to communicate trade-offs between different design choices.
A system design question might be:
“Design a URL shortening service like bit.ly.”
Your approach might include:
- Clarify requirements (e.g., expected traffic, length of shortened URLs)
- Design the high-level system architecture
- Choose appropriate data storage solutions
- Discuss scaling strategies
- Address potential issues like collision handling and analytics
4. Behavioral Interviews
While not strictly technical, behavioral interviews are an essential part of the hiring process at many tech companies.
What to Expect:
- Questions about your past experiences, challenges you’ve faced, and how you’ve handled them.
- Scenarios to assess your problem-solving skills, teamwork, and cultural fit.
- Discussions about your career goals and motivations.
How to Prepare:
- Use the STAR method (Situation, Task, Action, Result) to structure your responses.
- Prepare examples of projects you’ve worked on, challenges you’ve overcome, and leadership experiences.
- Research the company’s values and culture to align your responses.
- Practice common behavioral questions with a friend or mentor.
Example behavioral question:
“Tell me about a time when you had to work with a difficult team member. How did you handle the situation?”
5. Pair Programming Interviews
Pair programming interviews simulate real-world collaborative coding scenarios and assess your ability to work with others.
What to Expect:
- You’ll work on a coding problem with an interviewer or another candidate.
- The focus is on communication, collaboration, and problem-solving.
- You may be asked to alternate between “driver” (writing code) and “navigator” (providing direction) roles.
How to Prepare:
- Practice pair programming with friends or coding groups.
- Work on verbalizing your thought process as you code.
- Learn to give and receive constructive feedback.
- Be open to different approaches and ideas.
6. Technical Phone Screens
Technical phone screens are often the first step in the interview process and serve as a preliminary assessment of your skills.
What to Expect:
- A mix of technical questions and simple coding problems.
- Questions about your background and experience.
- You may be asked to write code in a shared online editor.
How to Prepare:
- Review fundamental CS concepts and common algorithms.
- Practice explaining technical concepts clearly and concisely.
- Ensure you have a quiet environment and a stable internet connection.
- Have a pen and paper ready for notes or diagrams.
7. Architecture Interviews
Architecture interviews are common for senior and lead positions and focus on your ability to design and evolve complex software systems.
What to Expect:
- Discussion of architectural patterns and best practices.
- Questions about trade-offs between different architectural choices.
- Scenarios involving legacy system migration or greenfield projects.
How to Prepare:
- Study various architectural styles (e.g., microservices, event-driven, serverless).
- Understand principles of software architecture (e.g., SOLID, DRY, KISS).
- Practice diagramming architectures and explaining your design decisions.
- Stay updated on current trends and technologies in software architecture.
8. Domain-Specific Interviews
Some companies conduct interviews that focus on specific domains or technologies relevant to their business.
What to Expect:
- In-depth questions about a particular technology stack or domain (e.g., machine learning, mobile development, blockchain).
- Practical problems related to the specific domain.
- Discussion of relevant tools, frameworks, and best practices.
How to Prepare:
- Research the company’s tech stack and domain focus.
- Brush up on specific technologies and concepts relevant to the role.
- Stay informed about the latest trends and developments in the domain.
- Prepare examples of projects or experiences related to the domain.
9. Whiteboard Design Sessions
Whiteboard design sessions are a hybrid of coding and system design interviews, often focusing on designing and implementing a specific component or feature.
What to Expect:
- You’ll be asked to design and implement a solution to a problem on a whiteboard.
- The focus is on your thought process, problem-solving approach, and ability to explain your decisions.
- You may need to write pseudo-code or actual code as part of your solution.
How to Prepare:
- Practice explaining your thought process out loud as you solve problems.
- Work on your ability to break down complex problems into smaller, manageable parts.
- Learn to manage your time effectively, balancing design and implementation.
- Get comfortable with writing and diagramming on a whiteboard.
10. Culture Fit Interviews
While not strictly technical, culture fit interviews are crucial in many tech companies’ hiring processes.
What to Expect:
- Questions about your work style, values, and how you handle various situations.
- Discussions about the company’s culture and how you might fit in.
- Scenarios to assess your alignment with the company’s values and ways of working.
How to Prepare:
- Research the company’s culture, values, and mission.
- Reflect on your own values and how they align with the company’s.
- Prepare examples of how you’ve contributed to positive work environments in the past.
- Be authentic and honest in your responses.
Conclusion
Navigating the various types of technical interviews can be challenging, but with proper preparation and practice, you can significantly improve your chances of success. Remember that different companies may use different combinations of these interview types, so it’s essential to research the specific process for each company you’re applying to.
Key takeaways for success across all interview types include:
- Strong fundamental knowledge of data structures, algorithms, and system design principles
- Clear communication of your thought process and problem-solving approach
- Ability to write clean, efficient code
- Familiarity with common interview patterns and questions
- Practice, practice, practice!
Platforms like AlgoCademy can be invaluable resources in your preparation journey, offering interactive coding tutorials, AI-powered assistance, and step-by-step guidance to help you develop the skills needed to excel in technical interviews.
Remember, the goal of these interviews is not just to test your technical skills but also to assess your problem-solving abilities, communication skills, and how you approach challenges. Stay calm, think logically, and don’t hesitate to ask clarifying questions when needed. With thorough preparation and the right mindset, you’ll be well-equipped to tackle any technical interview that comes your way. Good luck!