Developing Intuition for Choosing the Right Algorithm
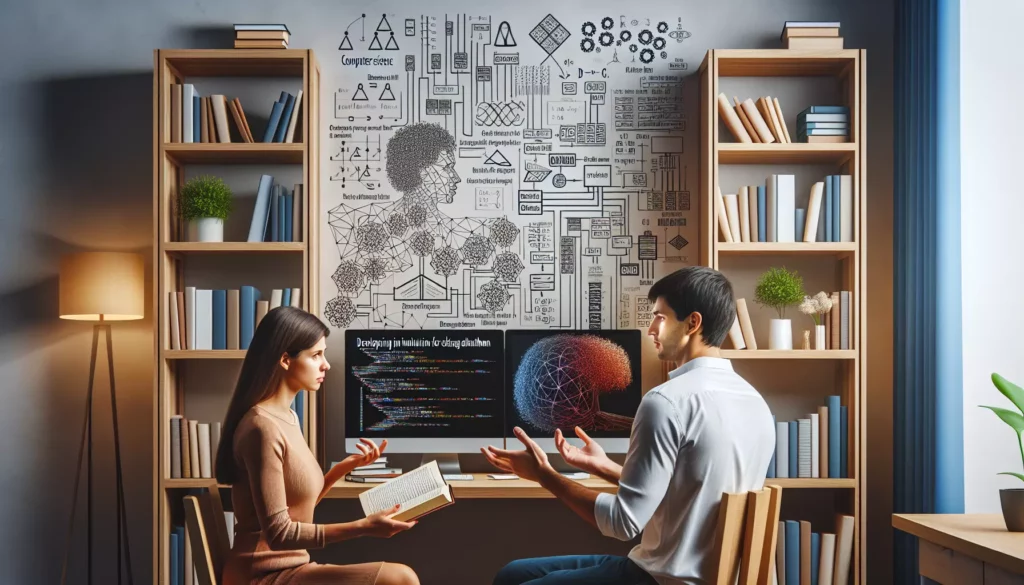
In the vast world of computer science and programming, algorithms serve as the backbone of efficient problem-solving. As you progress in your coding journey, particularly when preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), developing an intuition for choosing the right algorithm becomes crucial. This skill not only helps you solve problems more effectively but also demonstrates your depth of understanding to potential employers.
In this comprehensive guide, we’ll explore the process of developing algorithmic intuition, discuss various types of algorithms, and provide practical tips to help you make informed decisions when tackling coding challenges. Whether you’re a beginner looking to improve your problem-solving skills or an experienced developer preparing for technical interviews, this article will provide valuable insights to enhance your algorithmic thinking.
Understanding Algorithmic Intuition
Algorithmic intuition refers to the ability to quickly identify the most suitable algorithm or approach for solving a given problem. It’s not about memorizing a catalog of algorithms, but rather about understanding the fundamental principles and patterns that underlie different problem-solving techniques. Developing this intuition comes with practice, exposure to various problem types, and a deep understanding of algorithm complexity and trade-offs.
Why is Algorithmic Intuition Important?
- Efficient Problem-Solving: With good intuition, you can quickly narrow down the potential solutions and focus on the most promising approaches.
- Time Management: In time-constrained situations like coding interviews, the ability to quickly identify the right algorithm can be a game-changer.
- Code Optimization: Understanding which algorithm fits best helps in writing more efficient and optimized code.
- Adaptability: Strong algorithmic intuition allows you to adapt to new problems and scenarios more easily.
- Career Advancement: This skill is highly valued in the tech industry, particularly for roles that involve complex problem-solving and system design.
Key Factors in Choosing the Right Algorithm
When faced with a problem, several factors come into play when deciding which algorithm to use. Understanding these factors is crucial in developing your intuition:
1. Time Complexity
Time complexity is a measure of how the runtime of an algorithm grows with respect to the input size. It’s typically expressed using Big O notation.
- O(1): Constant time – ideal for small, fixed operations
- O(log n): Logarithmic time – often seen in divide-and-conquer algorithms
- O(n): Linear time – common in algorithms that process each input element once
- O(n log n): Linearithmic time – typical for efficient sorting algorithms
- O(n^2): Quadratic time – often seen in nested loops
- O(2^n): Exponential time – usually indicates a brute-force approach
Understanding these complexities helps you estimate how an algorithm will perform as the input size grows.
2. Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Sometimes, there’s a trade-off between time and space complexity.
- In-place algorithms: Modify the input directly, using O(1) extra space
- Out-of-place algorithms: Require additional space proportional to the input size
3. Input Size and Constraints
The size and nature of the input data can significantly influence algorithm choice:
- Small datasets might allow for more computationally intensive algorithms
- Large datasets may require more efficient algorithms to avoid time or memory limitations
- Specific input constraints (e.g., sorted data, limited range of values) can enable the use of specialized algorithms
4. Problem Type
Different categories of problems often have associated families of algorithms:
- Sorting: QuickSort, MergeSort, HeapSort
- Searching: Binary Search, Depth-First Search, Breadth-First Search
- Graph problems: Dijkstra’s algorithm, Bellman-Ford, Floyd-Warshall
- Dynamic Programming problems: often involve breaking down a problem into smaller subproblems
- String manipulation: KMP algorithm, Rabin-Karp algorithm
5. Required Operations
The types of operations needed can guide algorithm selection:
- Frequent insertions/deletions: Consider using a linked list or a dynamic array
- Fast lookups: Hash tables or balanced binary search trees might be appropriate
- Maintaining order: Sorted arrays or balanced binary search trees could be suitable
Common Algorithm Categories and Their Use Cases
To develop intuition, it’s helpful to understand the broad categories of algorithms and when they’re typically used:
1. Sorting Algorithms
- QuickSort: Efficient for average cases, in-place sorting
- MergeSort: Stable sorting, guaranteed O(n log n) time complexity
- HeapSort: In-place sorting with O(n log n) worst-case time complexity
- Counting Sort: Efficient for sorting integers with a limited range
2. Searching Algorithms
- Binary Search: Efficient for searching in sorted arrays
- Linear Search: Simple search for unsorted data
- Depth-First Search (DFS): Used in graph traversal, path finding
- Breadth-First Search (BFS): Used in graph traversal, shortest path in unweighted graphs
3. Graph Algorithms
- Dijkstra’s Algorithm: Finding shortest paths in weighted graphs
- Bellman-Ford Algorithm: Shortest paths with negative edge weights
- Kruskal’s Algorithm: Minimum spanning tree
- Topological Sort: Ordering tasks with dependencies
4. Dynamic Programming
Used for optimization problems with overlapping subproblems and optimal substructure. Common examples include:
- Fibonacci sequence calculation
- Longest Common Subsequence
- Knapsack problem
- Matrix Chain Multiplication
5. Greedy Algorithms
Used when a locally optimal choice leads to a globally optimal solution. Examples include:
- Huffman coding for data compression
- Dijkstra’s algorithm for shortest path
- Fractional Knapsack problem
6. Divide and Conquer
Breaking a problem into smaller subproblems, solving them, and combining the results. Examples include:
- MergeSort
- QuickSort
- Binary Search
- Strassen’s algorithm for matrix multiplication
Practical Tips for Developing Algorithmic Intuition
Now that we’ve covered the key factors and common algorithm categories, let’s explore some practical tips to help you develop your algorithmic intuition:
1. Practice Regularly
Consistent practice is key to developing intuition. Solve a variety of problems regularly:
- Use platforms like LeetCode, HackerRank, or CodeForces for diverse problem sets
- Participate in coding contests to challenge yourself under time pressure
- Implement algorithms from scratch to deepen your understanding
2. Analyze Multiple Solutions
For each problem you solve:
- Try to come up with multiple solutions
- Analyze the time and space complexity of each approach
- Compare your solution with other efficient solutions
- Understand why one solution might be preferred over another
3. Study Algorithm Design Techniques
Familiarize yourself with common algorithm design techniques:
- Greedy algorithms
- Dynamic programming
- Divide and conquer
- Backtracking
- Branch and bound
Understanding these techniques will help you recognize patterns in problems and apply appropriate strategies.
4. Learn to Recognize Problem Patterns
Many problems fall into recognizable patterns. Learn to identify these patterns:
- Two-pointer technique
- Sliding window
- Tree traversal patterns
- Graph traversal patterns
- Interval problems
5. Implement Data Structures from Scratch
Understanding the internals of data structures helps in choosing the right one for a given problem:
- Implement arrays, linked lists, stacks, and queues
- Build your own hash table
- Implement tree structures like binary search trees and heaps
- Create graph representations (adjacency list, adjacency matrix)
6. Analyze Real-World Systems
Study how algorithms are applied in real-world systems:
- Database indexing techniques
- Load balancing algorithms in distributed systems
- Caching strategies in web applications
- Recommendation systems in e-commerce platforms
7. Teach Others
Explaining concepts to others can solidify your understanding:
- Participate in coding forums and help others solve problems
- Write blog posts explaining algorithms
- Conduct coding workshops or study groups
Case Studies: Applying Algorithmic Intuition
Let’s look at a few examples to see how algorithmic intuition can be applied in practice:
Case Study 1: Finding a Number in a Sorted Array
Problem: Given a sorted array of integers and a target integer, find if the target exists in the array.
Intuition: The key information here is that the array is sorted. This immediately suggests that we can use a more efficient algorithm than simple linear search.
Solution: Binary Search is the optimal choice here. It has a time complexity of O(log n), which is much better than the O(n) complexity of linear search for large arrays.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return True
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return False
Case Study 2: Finding the Shortest Path in a Weighted Graph
Problem: Given a weighted graph and two nodes, find the shortest path between them.
Intuition: This is a classic shortest path problem in a weighted graph. The presence of weights rules out a simple BFS approach.
Solution: Dijkstra’s algorithm is an excellent choice for finding the shortest path in a weighted graph with non-negative weights. If negative weights are possible, we might consider the Bellman-Ford algorithm instead.
import heapq
def dijkstra(graph, start, end):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
return current_distance
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return float('infinity') # If no path is found
Case Study 3: Finding the Longest Increasing Subsequence
Problem: Given an unsorted array of integers, find the length of the longest increasing subsequence.
Intuition: This problem has an optimal substructure (the solution can be constructed from optimal solutions of its subproblems) and overlapping subproblems, which are hallmarks of dynamic programming problems.
Solution: We can use dynamic programming to solve this efficiently in O(n^2) time, or even better, we can use a combination of dynamic programming and binary search to achieve O(n log n) time complexity.
def longest_increasing_subsequence(arr):
if not arr:
return 0
# dp[i] represents the length of the longest increasing subsequence
# that ends with arr[i]
dp = [1] * len(arr)
for i in range(1, len(arr)):
for j in range(i):
if arr[i] > arr[j]:
dp[i] = max(dp[i], dp[j] + 1)
return max(dp)
Common Pitfalls in Algorithm Selection
As you develop your algorithmic intuition, be aware of these common pitfalls:
1. Overcomplicating Solutions
Sometimes, a simple solution is the best. Don’t always reach for complex algorithms if a straightforward approach will suffice.
2. Ignoring Problem Constraints
Always pay attention to the constraints given in the problem statement. They often provide clues about which algorithms are suitable.
3. Premature Optimization
Don’t optimize prematurely. Start with a working solution, then optimize if necessary.
4. Neglecting Edge Cases
Consider edge cases when choosing an algorithm. Some algorithms may perform poorly or fail entirely for certain edge cases.
5. Forgetting About Space Complexity
Time complexity is important, but don’t forget about space complexity, especially when dealing with large datasets or memory-constrained environments.
Conclusion
Developing intuition for choosing the right algorithm is a crucial skill for any programmer, especially those preparing for technical interviews at top tech companies. It’s a skill that comes with practice, experience, and a deep understanding of various algorithmic approaches.
Remember, the goal is not to memorize solutions to specific problems, but to develop a problem-solving mindset that allows you to approach new challenges with confidence. By understanding the fundamental principles behind different algorithms, recognizing common problem patterns, and practicing regularly, you’ll gradually build the intuition needed to tackle a wide range of coding challenges.
As you continue your journey in algorithm mastery, keep challenging yourself with diverse problems, analyze your solutions critically, and always strive to understand the why behind each approach. With time and dedication, you’ll find yourself naturally gravitating towards efficient solutions, a skill that will serve you well throughout your programming career.
Happy coding, and may your algorithmic intuition grow stronger with each problem you solve!