Developing Algorithms for Real-Time Analytics: A Comprehensive Guide
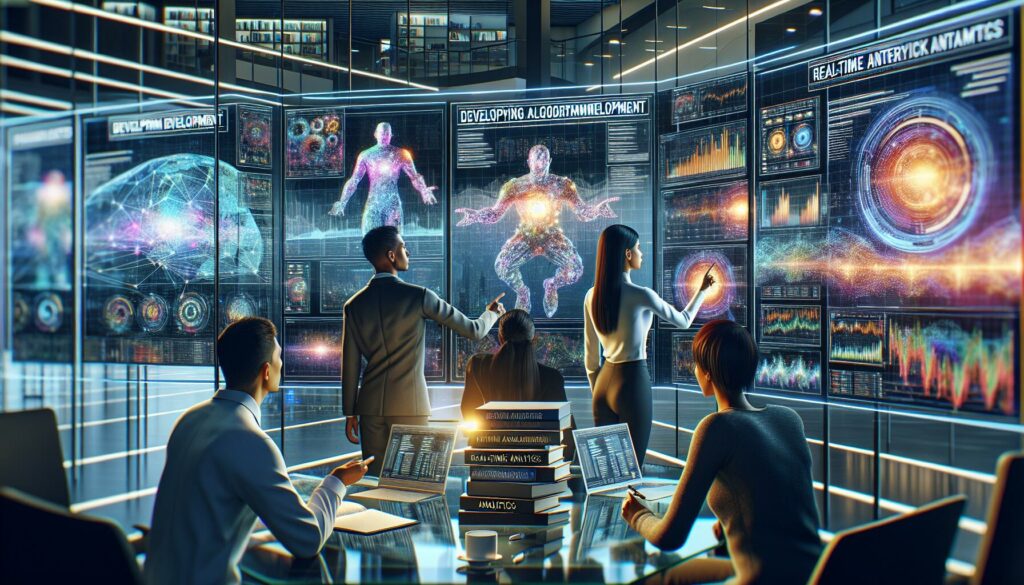
In the fast-paced world of modern technology, the ability to process and analyze data in real-time has become increasingly crucial. Real-time analytics allow businesses and organizations to make instant decisions based on up-to-the-minute information, providing a competitive edge in various industries. This article will delve into the intricacies of developing algorithms for real-time analytics, exploring key concepts, challenges, and best practices.
Understanding Real-Time Analytics
Before we dive into algorithm development, it’s essential to understand what real-time analytics entails. Real-time analytics refers to the ability to collect, process, and analyze data as it’s generated, allowing for immediate insights and actions. This differs from traditional batch processing, where data is collected over time and analyzed periodically.
Key Components of Real-Time Analytics
- Data Ingestion: Collecting data from various sources in real-time
- Stream Processing: Analyzing data on-the-fly as it flows through the system
- Complex Event Processing: Identifying patterns and correlations in real-time data streams
- Data Storage: Efficiently storing processed data for further analysis
- Visualization: Presenting insights in an easily digestible format
Challenges in Developing Real-Time Analytics Algorithms
Creating algorithms for real-time analytics presents unique challenges that developers must overcome:
1. Latency
One of the primary challenges in real-time analytics is minimizing latency. Algorithms must process data quickly enough to provide insights in near real-time, which can be particularly challenging when dealing with large volumes of data.
2. Scalability
As data volumes grow, algorithms need to scale efficiently to handle increased loads without sacrificing performance or accuracy.
3. Accuracy
Maintaining high levels of accuracy while processing data at high speeds is crucial. Algorithms must balance speed with precision to provide reliable insights.
4. Fault Tolerance
Real-time systems must be robust enough to handle failures and continue operating without significant disruption.
5. Data Quality
Ensuring the quality and consistency of incoming data is essential for accurate analysis.
Key Algorithms for Real-Time Analytics
Several algorithms are commonly used in real-time analytics. Let’s explore some of the most important ones:
1. Sliding Window Algorithm
The sliding window algorithm is fundamental in real-time analytics, particularly for time-series data. It maintains a “window” of recent data points and updates calculations as new data arrives and old data falls out of the window.
Here’s a simple implementation of a sliding window algorithm in Python:
class SlidingWindow:
def __init__(self, window_size):
self.window_size = window_size
self.window = []
def add_element(self, element):
if len(self.window) >= self.window_size:
self.window.pop(0)
self.window.append(element)
def get_average(self):
return sum(self.window) / len(self.window) if self.window else 0
# Usage
window = SlidingWindow(5)
for i in range(10):
window.add_element(i)
print(f"Current window: {window.window}, Average: {window.get_average()}")
2. Exponential Moving Average (EMA)
The Exponential Moving Average is useful for smoothing time series data and giving more weight to recent observations. It’s particularly effective in real-time analytics for detecting trends and reducing noise in data streams.
def exponential_moving_average(data, alpha):
ema = [data[0]] # Start with the first data point
for i in range(1, len(data)):
ema.append(alpha * data[i] + (1 - alpha) * ema[i-1])
return ema
# Usage
data = [1, 3, 5, 7, 9, 11, 13, 15]
alpha = 0.3
ema_result = exponential_moving_average(data, alpha)
print(f"EMA result: {ema_result}")
3. Count-Min Sketch
The Count-Min Sketch algorithm is a probabilistic data structure used for summarizing streaming data. It’s particularly useful for counting or estimating the frequency of events in a stream.
import mmh3
import numpy as np
class CountMinSketch:
def __init__(self, width, depth):
self.width = width
self.depth = depth
self.sketch = np.zeros((depth, width), dtype=int)
def add(self, item, count=1):
for i in range(self.depth):
j = mmh3.hash(item, i) % self.width
self.sketch[i, j] += count
def estimate(self, item):
return min(self.sketch[i, mmh3.hash(item, i) % self.width] for i in range(self.depth))
# Usage
cms = CountMinSketch(10, 5)
cms.add("apple", 3)
cms.add("banana", 2)
cms.add("apple", 1)
print(f"Estimated count for 'apple': {cms.estimate('apple')}")
print(f"Estimated count for 'banana': {cms.estimate('banana')}")
4. Reservoir Sampling
Reservoir sampling is an algorithm used for randomly selecting a fixed-size sample from a stream of data of unknown or unbounded size. This is particularly useful in real-time analytics when you need to maintain a representative sample of a large data stream.
import random
def reservoir_sampling(stream, k):
reservoir = []
for i, item in enumerate(stream):
if len(reservoir) < k:
reservoir.append(item)
else:
j = random.randint(0, i)
if j < k:
reservoir[j] = item
return reservoir
# Usage
stream = range(1000000) # Simulating a large stream of data
sample_size = 10
sample = reservoir_sampling(stream, sample_size)
print(f"Random sample of size {sample_size}: {sample}")
Best Practices for Developing Real-Time Analytics Algorithms
When developing algorithms for real-time analytics, consider the following best practices:
1. Optimize for Speed
In real-time analytics, speed is crucial. Optimize your algorithms to process data as quickly as possible without sacrificing accuracy. This may involve using efficient data structures, parallelizing computations where possible, and minimizing unnecessary operations.
2. Use Approximation Techniques
In some cases, it may be acceptable to use approximation techniques that trade a small amount of accuracy for significant gains in speed. Algorithms like Count-Min Sketch and HyperLogLog are examples of probabilistic data structures that provide efficient approximations for certain types of queries.
3. Implement Incremental Processing
Design your algorithms to process data incrementally whenever possible. This allows for continuous updates as new data arrives, rather than recomputing results from scratch each time.
4. Consider Data Expiration
In many real-time analytics scenarios, older data becomes less relevant over time. Implement mechanisms to expire or downweight older data to maintain relevance and manage memory usage.
5. Plan for Scalability
Design your algorithms with scalability in mind from the start. This may involve using distributed computing frameworks or implementing algorithms that can be easily parallelized.
6. Implement Robust Error Handling
Real-time systems must be resilient to errors and unexpected inputs. Implement thorough error handling and data validation to ensure your algorithms can handle anomalies gracefully.
7. Use Efficient Data Structures
Choose appropriate data structures that support fast insertions, deletions, and lookups. For example, using a circular buffer for a sliding window can be more efficient than a standard array or list.
8. Leverage In-Memory Processing
Whenever possible, process data in-memory to minimize I/O operations and reduce latency. This is particularly important for real-time analytics where speed is critical.
Advanced Techniques in Real-Time Analytics
As you become more proficient in developing real-time analytics algorithms, consider exploring these advanced techniques:
1. Sketch Algorithms
In addition to Count-Min Sketch, other sketch algorithms like HyperLogLog for cardinality estimation and Bloom filters for membership testing can be valuable in real-time analytics scenarios.
2. Online Machine Learning
Implement online machine learning algorithms that can update models incrementally as new data arrives, rather than requiring periodic batch retraining.
3. Complex Event Processing (CEP)
Develop algorithms for identifying complex patterns and correlations across multiple data streams in real-time.
4. Anomaly Detection
Implement real-time anomaly detection algorithms to identify unusual patterns or behaviors as they occur.
5. Time Series Forecasting
Develop algorithms for real-time forecasting of time series data, such as ARIMA models or exponential smoothing techniques adapted for streaming data.
Implementing Real-Time Analytics in Practice
When implementing real-time analytics algorithms in production systems, consider the following aspects:
1. Choosing the Right Technology Stack
Select appropriate technologies for each component of your real-time analytics pipeline. This may include:
- Stream processing frameworks like Apache Kafka Streams, Apache Flink, or Apache Spark Streaming
- In-memory databases like Redis or Apache Ignite for fast data access
- Time-series databases like InfluxDB or TimescaleDB for efficient storage and querying of time-series data
- Visualization tools like Grafana or Tableau for real-time dashboards
2. Ensuring Data Quality
Implement data validation and cleansing steps to ensure the quality of incoming data. This may involve:
- Filtering out invalid or corrupt data
- Normalizing data formats
- Handling missing values
- Detecting and addressing outliers
3. Monitoring and Alerting
Set up comprehensive monitoring for your real-time analytics system, including:
- Performance metrics (latency, throughput, resource utilization)
- Data quality metrics
- Alerting mechanisms for anomalies or system issues
4. Scaling Considerations
Plan for scaling your real-time analytics system as data volumes grow. This may involve:
- Horizontal scaling of processing nodes
- Partitioning data streams for parallel processing
- Implementing load balancing mechanisms
5. Security and Compliance
Ensure that your real-time analytics system adheres to relevant security and compliance requirements, including:
- Data encryption in transit and at rest
- Access control and authentication mechanisms
- Compliance with data protection regulations (e.g., GDPR, CCPA)
Case Studies: Real-Time Analytics in Action
To illustrate the practical applications of real-time analytics algorithms, let’s examine a few case studies:
1. E-commerce Fraud Detection
An e-commerce platform implements real-time fraud detection using a combination of sliding window algorithms and machine learning models. The system analyzes user behavior, transaction patterns, and historical data to flag potentially fraudulent transactions in real-time, allowing for immediate intervention.
2. Social Media Sentiment Analysis
A social media monitoring tool uses real-time analytics to perform sentiment analysis on incoming posts and comments. The system employs natural language processing algorithms and sliding windows to track sentiment trends and detect sudden shifts in public opinion.
3. IoT Sensor Monitoring
An industrial IoT system uses real-time analytics to monitor sensor data from manufacturing equipment. The system employs exponential moving averages and anomaly detection algorithms to identify potential equipment failures or process inefficiencies in real-time, enabling proactive maintenance and optimizing production.
4. Financial Market Analysis
A financial trading platform uses real-time analytics to process market data streams and identify trading opportunities. The system employs complex event processing algorithms to detect patterns across multiple data streams and generate trading signals with low latency.
Future Trends in Real-Time Analytics
As technology continues to evolve, several trends are shaping the future of real-time analytics:
1. Edge Computing
The rise of edge computing is enabling real-time analytics to be performed closer to the data source, reducing latency and bandwidth requirements. This is particularly relevant for IoT applications and scenarios with limited connectivity.
2. AI and Machine Learning Integration
Advanced AI and machine learning techniques are being increasingly integrated into real-time analytics systems, enabling more sophisticated analysis and predictive capabilities.
3. 5G and Improved Connectivity
The rollout of 5G networks is expected to significantly enhance the capabilities of real-time analytics systems, particularly for mobile and IoT applications, by providing faster and more reliable data transmission.
4. Quantum Computing
While still in its early stages, quantum computing has the potential to revolutionize certain aspects of real-time analytics by enabling complex computations that are currently infeasible with classical computers.
Conclusion
Developing algorithms for real-time analytics is a challenging but rewarding field that sits at the intersection of computer science, data engineering, and domain-specific expertise. By understanding the core concepts, mastering key algorithms, and following best practices, developers can create powerful real-time analytics systems that provide immediate insights and drive data-driven decision-making.
As the volume and velocity of data continue to grow, the importance of real-time analytics will only increase. By staying abreast of emerging trends and continuously refining their skills, developers can position themselves at the forefront of this exciting and rapidly evolving field.
Remember that developing effective real-time analytics algorithms requires not only technical skill but also a deep understanding of the problem domain and the specific requirements of each use case. As you continue to explore and implement real-time analytics solutions, always strive to balance performance, accuracy, and scalability to create systems that provide genuine value in today’s fast-paced, data-driven world.