Developing a Personalized Interview Prep Plan: Your Roadmap to Success
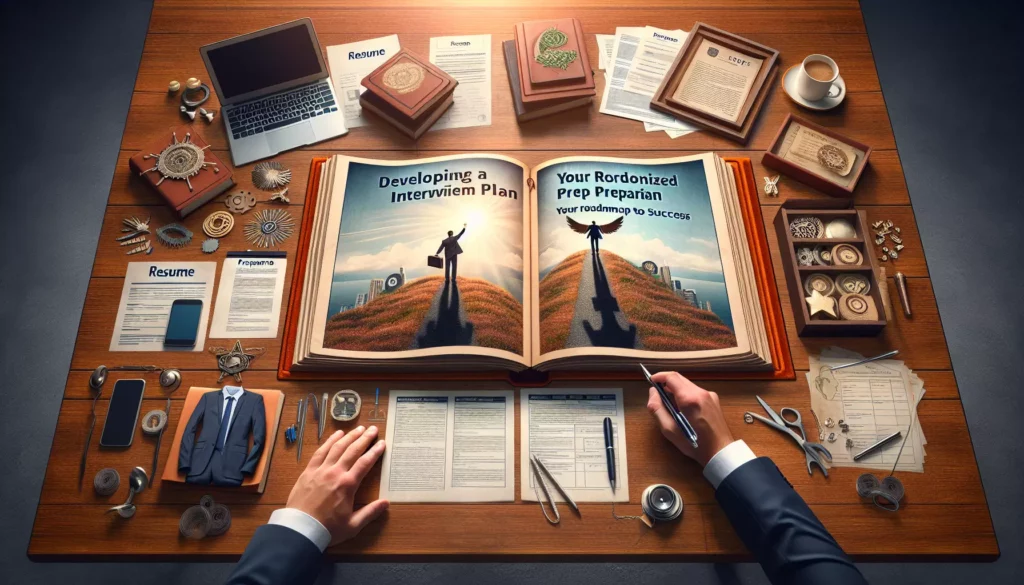
In the competitive world of tech, landing your dream job at a major company like Google, Amazon, or Facebook requires more than just coding skills. It demands a strategic approach to interview preparation. This comprehensive guide will walk you through the process of developing a personalized interview prep plan, tailored to your unique strengths, weaknesses, and career goals.
Why a Personalized Interview Prep Plan Matters
Every aspiring developer has a unique background, skill set, and learning style. A one-size-fits-all approach to interview preparation often falls short. By creating a personalized plan, you can:
- Focus on your specific areas of improvement
- Maximize your study time efficiency
- Build confidence in your abilities
- Tailor your preparation to the companies you’re targeting
- Track your progress and stay motivated
Let’s dive into the step-by-step process of crafting your personalized interview prep plan.
Step 1: Assess Your Current Skills
Before you can create an effective plan, you need to know where you stand. Start by conducting a thorough self-assessment of your coding skills and knowledge.
1.1 Take a Coding Assessment
Platforms like AlgoCademy offer comprehensive coding assessments that can help you identify your strengths and weaknesses across various topics. These assessments typically cover:
- Data Structures (Arrays, Linked Lists, Trees, Graphs, etc.)
- Algorithms (Sorting, Searching, Dynamic Programming, etc.)
- Problem-solving skills
- Time and space complexity analysis
1.2 Review Your Past Projects and Experiences
Reflect on your coding projects, internships, or work experiences. What technologies have you worked with? What challenges did you face, and how did you overcome them? This reflection will help you identify areas where you have practical experience and those where you need more exposure.
1.3 Identify Your Learning Style
Understanding how you learn best is crucial for effective preparation. Are you a visual learner who benefits from diagrams and flowcharts? Do you prefer hands-on coding exercises? Or do you learn best through discussions and explanations? Knowing your learning style will help you choose the most effective study materials and methods.
Step 2: Set Clear Goals
With a clear understanding of your current skills, it’s time to set specific, measurable, achievable, relevant, and time-bound (SMART) goals for your interview preparation.
2.1 Define Your Target Companies
Research the companies you’re interested in and make a list of your top choices. Each company may have slightly different interview processes and focus areas, so tailoring your preparation to your target companies is essential.
2.2 Identify the Required Skills
Based on job descriptions and insider information (from sites like Glassdoor or LinkedIn), create a list of skills and knowledge areas that your target companies prioritize. This might include:
- Specific programming languages (e.g., Java, Python, C++)
- Data structures and algorithms
- System design principles
- Specific technologies or frameworks
2.3 Set Timeframe and Milestones
Determine how much time you have for preparation and set realistic milestones. For example:
- Week 1-4: Strengthen fundamentals in data structures and algorithms
- Week 5-8: Focus on advanced topics and system design
- Week 9-12: Practice mock interviews and work on communication skills
Step 3: Create Your Study Plan
Now that you have clear goals, it’s time to create a structured study plan that addresses your weaknesses and builds on your strengths.
3.1 Prioritize Topics
Based on your assessment results and the skills required by your target companies, create a prioritized list of topics to study. For example:
- Arrays and Strings
- Linked Lists
- Trees and Graphs
- Sorting and Searching
- Dynamic Programming
- System Design
3.2 Choose Your Resources
Select a mix of resources that cater to your learning style and cover the topics you need to master. Some popular options include:
- Online platforms: AlgoCademy, LeetCode, HackerRank
- Books: “Cracking the Coding Interview” by Gayle Laakmann McDowell
- Video courses: Coursera, edX, or YouTube tutorials
- Coding bootcamps or workshops
3.3 Create a Study Schedule
Develop a realistic study schedule that fits your daily routine. Be sure to include:
- Regular study sessions (e.g., 2 hours per day)
- Problem-solving practice
- Review and revision time
- Breaks to prevent burnout
Here’s an example of how you might structure your daily study routine:
// Sample Daily Study Routine
function dailyStudyRoutine() {
const morningSession = {
duration: 1,
activity: "Review previous day's concepts"
};
const afternoonSession = {
duration: 2,
activity: "Learn new topic and solve related problems"
};
const eveningSession = {
duration: 1,
activity: "Practice coding challenges on AlgoCademy"
};
return [morningSession, afternoonSession, eveningSession];
}
Step 4: Implement Effective Study Techniques
To make the most of your study time, incorporate these proven techniques into your preparation:
4.1 Active Recall
Instead of passively reading or watching videos, actively engage with the material. After learning a new concept, try to explain it in your own words or teach it to someone else. This helps reinforce your understanding and identifies areas where you need more clarity.
4.2 Spaced Repetition
Review topics at increasing intervals to improve long-term retention. For example, review a topic after 1 day, then 3 days, then a week, and so on. This technique helps move information from short-term to long-term memory.
4.3 Interleaving
Mix up your practice with different types of problems and topics. This approach improves your ability to choose the right technique for each problem, a crucial skill in interviews.
// Example of interleaved practice schedule
function interleavedPractice(topics) {
let schedule = [];
for (let i = 0; i < topics.length; i++) {
schedule.push({
topic: topics[i],
problems: getRandomProblems(topics[i], 2)
});
schedule.push({
topic: topics[(i + 1) % topics.length],
problems: getRandomProblems(topics[(i + 1) % topics.length], 2)
});
}
return schedule;
}
const topics = ["Arrays", "LinkedLists", "Trees", "DynamicProgramming"];
const practiceSchedule = interleavedPractice(topics);
4.4 Deliberate Practice
Focus on challenging problems that push you out of your comfort zone. Analyze your mistakes and learn from them. Platforms like AlgoCademy often provide detailed explanations and multiple approaches to problems, which can be invaluable for deliberate practice.
Step 5: Develop Your Problem-Solving Process
Having a structured approach to problem-solving is crucial for technical interviews. Develop and refine your process using these steps:
5.1 Understand the Problem
Before diving into coding, make sure you fully understand the problem requirements, constraints, and expected output. Ask clarifying questions if needed.
5.2 Plan Your Approach
Sketch out a high-level plan or algorithm before coding. Consider multiple approaches and discuss their trade-offs.
5.3 Implement the Solution
Write clean, well-organized code. Use meaningful variable names and add comments to explain your thought process.
5.4 Test and Debug
Run through test cases, including edge cases, to verify your solution. Debug any issues you encounter.
5.5 Optimize
Consider ways to improve the time and space complexity of your solution. Discuss potential optimizations with your interviewer.
// Example problem-solving framework
function solveProblem(problem) {
// Step 1: Understand the problem
const requirements = analyzeRequirements(problem);
const constraints = identifyConstraints(problem);
// Step 2: Plan your approach
const approach = planApproach(requirements, constraints);
// Step 3: Implement the solution
const solution = implementSolution(approach);
// Step 4: Test and debug
const testCases = generateTestCases(problem);
const debuggedSolution = testAndDebug(solution, testCases);
// Step 5: Optimize
const optimizedSolution = optimizeSolution(debuggedSolution);
return optimizedSolution;
}
Step 6: Practice Mock Interviews
As you progress in your preparation, incorporate mock interviews into your routine. This will help you:
- Get comfortable with the interview format
- Improve your communication skills
- Learn to manage time pressure
- Receive feedback on your performance
6.1 Use Online Platforms
Websites like Pramp or interviewing.io offer free peer-to-peer mock interviews. AlgoCademy also provides AI-powered mock interviews that can give you immediate feedback on your performance.
6.2 Practice with Friends or Mentors
Ask friends in the industry or find mentors who can conduct mock interviews for you. This personal touch can provide valuable insights and tailored feedback.
6.3 Record and Review
If possible, record your mock interviews (with permission) and review them later. This can help you identify areas for improvement in your communication and problem-solving approach.
Step 7: Continuously Evaluate and Adjust
Your interview prep plan should be flexible and adaptable. Regularly assess your progress and make adjustments as needed.
7.1 Track Your Progress
Keep a log of the problems you’ve solved, concepts you’ve mastered, and areas where you still struggle. Many platforms, including AlgoCademy, offer progress tracking features to help with this.
7.2 Seek Feedback
Regularly seek feedback from peers, mentors, or through online communities. This external perspective can help you identify blind spots in your preparation.
7.3 Stay Updated
The tech industry evolves rapidly. Stay informed about new trends, technologies, and interview processes at your target companies. Adjust your prep plan accordingly.
7.4 Celebrate Milestones
Acknowledge your progress and celebrate small wins along the way. This positive reinforcement can help maintain motivation during the challenging preparation process.
Conclusion: Your Path to Interview Success
Developing a personalized interview prep plan is a crucial step towards landing your dream job in the tech industry. By assessing your skills, setting clear goals, creating a structured study plan, and continuously refining your approach, you’ll be well-prepared to tackle even the most challenging technical interviews.
Remember, the key to success lies not just in accumulating knowledge, but in developing the problem-solving skills and confidence to apply that knowledge effectively under pressure. Platforms like AlgoCademy can provide the structure, resources, and practice you need to turn your personalized plan into interview success.
As you embark on this journey, stay committed to your goals, be patient with yourself, and trust in the process. With dedication and the right approach, you’ll be well on your way to impressing interviewers and landing that coveted position at your dream tech company.
Happy coding, and best of luck with your interview preparation!