Designing Algorithms for Fair Resource Sharing: A Comprehensive Guide
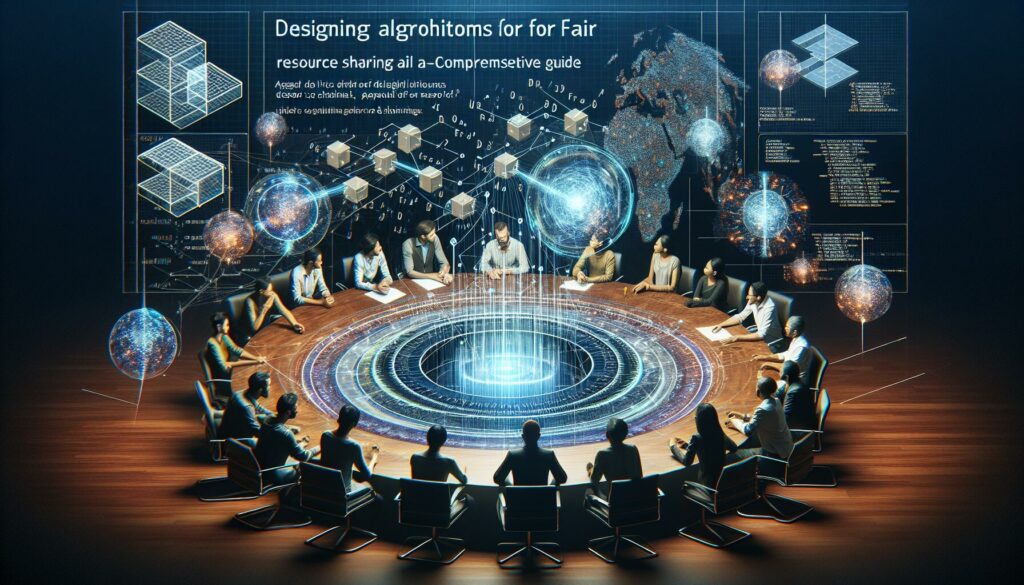
In today’s interconnected digital world, the efficient and fair allocation of resources is a critical challenge across various domains, from computer networks to cloud computing platforms. As our reliance on shared resources grows, so does the need for sophisticated algorithms that can ensure equitable distribution and optimal utilization. This article delves deep into the intricacies of designing algorithms for fair resource sharing, exploring key concepts, common approaches, and practical implementations.
Understanding Fair Resource Sharing
Before we dive into the algorithmic aspects, it’s essential to understand what we mean by “fair resource sharing.” In the context of computing and networking, fair resource sharing refers to the equitable allocation of limited resources among multiple users or processes. The goal is to maximize overall system efficiency while ensuring that no single user or process monopolizes the available resources at the expense of others.
Fair resource sharing is crucial in various scenarios, including:
- Network bandwidth allocation
- CPU scheduling in operating systems
- Disk I/O management
- Cloud computing resource allocation
- Distributed systems load balancing
Designing algorithms for fair resource sharing involves balancing multiple objectives, such as:
- Maximizing resource utilization
- Minimizing waiting times
- Ensuring fairness among users or processes
- Adapting to changing resource demands
- Preventing starvation of low-priority tasks
Key Concepts in Fair Resource Sharing
Before we explore specific algorithms, let’s familiarize ourselves with some fundamental concepts that underpin fair resource sharing:
1. Fairness Metrics
To design effective algorithms, we need ways to quantify and measure fairness. Some common fairness metrics include:
- Max-min fairness: Maximizes the minimum allocation among all users
- Proportional fairness: Balances efficiency and fairness by maximizing the product of allocations
- Jain’s fairness index: A numerical measure of fairness ranging from 0 (completely unfair) to 1 (perfectly fair)
2. Resource Types
Different resources have different characteristics that influence sharing algorithms:
- Divisible resources: Can be split into arbitrary amounts (e.g., CPU time, network bandwidth)
- Indivisible resources: Must be allocated as whole units (e.g., processors, memory pages)
- Renewable resources: Can be reused after release (e.g., CPU cycles)
- Consumable resources: Are depleted upon use (e.g., energy in battery-powered devices)
3. Workload Characteristics
The nature of the workload significantly impacts algorithm design:
- Static vs. dynamic: Are resource demands known in advance or do they change over time?
- Homogeneous vs. heterogeneous: Are all users/processes similar, or do they have diverse requirements?
- Periodic vs. aperiodic: Do resource requests follow a regular pattern or occur randomly?
Common Algorithms for Fair Resource Sharing
Now that we have a foundation in the key concepts, let’s explore some widely-used algorithms for fair resource sharing:
1. Round Robin (RR)
Round Robin is one of the simplest and most widely used scheduling algorithms for fair resource sharing, particularly in CPU scheduling and network packet scheduling.
How it works:
- Allocate a fixed time quantum to each process or user in a circular order.
- If a process completes before its time quantum expires, the next process in the queue is scheduled immediately.
- If a process doesn’t complete within its time quantum, it’s moved to the back of the queue, and the next process is scheduled.
Advantages:
- Simple to implement and understand
- Ensures fairness by giving each process equal opportunity
- Prevents starvation of low-priority tasks
Disadvantages:
- Performance degrades with a large number of processes due to frequent context switching
- Not ideal for processes with varying CPU burst times
Implementation example (Python):
def round_robin(processes, time_quantum):
remaining_time = {p: processes[p] for p in processes}
ready_queue = list(processes.keys())
current_time = 0
while ready_queue:
process = ready_queue.pop(0)
if remaining_time[process] <= time_quantum:
current_time += remaining_time[process]
print(f"Process {process} completed at time {current_time}")
remaining_time[process] = 0
else:
current_time += time_quantum
remaining_time[process] -= time_quantum
ready_queue.append(process)
print(f"Process {process} executed for {time_quantum} units. Remaining time: {remaining_time[process]}")
# Example usage
processes = {"P1": 10, "P2": 5, "P3": 8}
time_quantum = 2
round_robin(processes, time_quantum)
2. Weighted Fair Queuing (WFQ)
Weighted Fair Queuing is an advanced scheduling algorithm that extends the concept of fair queuing by assigning weights to different queues or flows. It’s commonly used in network routers for bandwidth allocation.
How it works:
- Assign weights to different queues based on their priority or service level agreement (SLA).
- Calculate a virtual finish time for each packet based on its size and queue weight.
- Schedule packets in order of their virtual finish times.
Advantages:
- Provides fair bandwidth allocation based on assigned weights
- Supports differentiated services and quality of service (QoS) requirements
- Protects well-behaved flows from misbehaving ones
Disadvantages:
- More complex to implement compared to simpler algorithms
- May introduce some overhead in packet processing
Implementation example (Python):
import heapq
class Packet:
def __init__(self, flow_id, size, arrival_time):
self.flow_id = flow_id
self.size = size
self.arrival_time = arrival_time
self.finish_time = 0
class WFQ:
def __init__(self, weights):
self.weights = weights
self.virtual_time = 0
self.queues = {flow_id: [] for flow_id in weights}
self.schedule = []
def enqueue(self, packet):
self.queues[packet.flow_id].append(packet)
def calculate_finish_time(self, packet):
flow_id = packet.flow_id
if not self.queues[flow_id]:
start_time = max(self.virtual_time, packet.arrival_time)
else:
start_time = max(self.virtual_time, self.queues[flow_id][-1].finish_time)
return start_time + (packet.size / self.weights[flow_id])
def schedule_packets(self):
for flow_id, queue in self.queues.items():
if queue:
packet = queue[0]
packet.finish_time = self.calculate_finish_time(packet)
heapq.heappush(self.schedule, (packet.finish_time, packet))
while self.schedule:
finish_time, packet = heapq.heappop(self.schedule)
self.virtual_time = finish_time
yield packet
self.queues[packet.flow_id].pop(0)
if self.queues[packet.flow_id]:
next_packet = self.queues[packet.flow_id][0]
next_packet.finish_time = self.calculate_finish_time(next_packet)
heapq.heappush(self.schedule, (next_packet.finish_time, next_packet))
# Example usage
weights = {"Flow1": 2, "Flow2": 1, "Flow3": 3}
wfq = WFQ(weights)
packets = [
Packet("Flow1", 100, 0),
Packet("Flow2", 200, 0),
Packet("Flow3", 150, 0),
Packet("Flow1", 120, 1),
Packet("Flow2", 80, 2),
]
for packet in packets:
wfq.enqueue(packet)
for scheduled_packet in wfq.schedule_packets():
print(f"Scheduled: Flow {scheduled_packet.flow_id}, Size {scheduled_packet.size}, Finish Time {scheduled_packet.finish_time:.2f}")
3. Deficit Round Robin (DRR)
Deficit Round Robin is an enhancement of the Round Robin algorithm that addresses the issue of unfairness when dealing with variable-sized packets or tasks.
How it works:
- Assign a quantum (credit) to each queue.
- In each round, add the quantum to the deficit counter of each non-empty queue.
- Serve packets from the queue as long as their size is less than or equal to the deficit counter.
- Subtract the size of each served packet from the deficit counter.
- If a packet is too large to be served, leave it in the queue and move to the next queue.
- Reset the deficit counter to zero when a queue becomes empty.
Advantages:
- Provides fairness for variable-sized packets or tasks
- Low complexity (O(1) per packet)
- Easily allows for weighted fair queuing by assigning different quanta to queues
Disadvantages:
- May introduce some latency for large packets in low-bandwidth scenarios
- Requires careful tuning of quantum sizes for optimal performance
Implementation example (Python):
from collections import deque
class Flow:
def __init__(self, id, quantum):
self.id = id
self.quantum = quantum
self.deficit = 0
self.queue = deque()
class DRR:
def __init__(self, flows):
self.flows = flows
self.active_list = deque(flows)
def enqueue(self, flow_id, packet_size):
for flow in self.flows:
if flow.id == flow_id:
flow.queue.append(packet_size)
if flow not in self.active_list:
self.active_list.append(flow)
break
def schedule(self):
while self.active_list:
current_flow = self.active_list.popleft()
current_flow.deficit += current_flow.quantum
while current_flow.queue and current_flow.queue[0] <= current_flow.deficit:
packet_size = current_flow.queue.popleft()
current_flow.deficit -= packet_size
yield (current_flow.id, packet_size)
if current_flow.queue:
self.active_list.append(current_flow)
else:
current_flow.deficit = 0
# Example usage
flows = [
Flow("Flow1", 500),
Flow("Flow2", 500),
Flow("Flow3", 500)
]
drr = DRR(flows)
# Enqueue packets
drr.enqueue("Flow1", 200)
drr.enqueue("Flow1", 500)
drr.enqueue("Flow2", 400)
drr.enqueue("Flow2", 300)
drr.enqueue("Flow3", 100)
drr.enqueue("Flow3", 200)
drr.enqueue("Flow3", 300)
# Schedule packets
for flow_id, packet_size in drr.schedule():
print(f"Scheduled: Flow {flow_id}, Packet Size {packet_size}")
Advanced Techniques for Fair Resource Sharing
While the algorithms discussed above form the foundation of fair resource sharing, more advanced techniques have been developed to address complex scenarios and optimize resource allocation further:
1. Proportional Share Scheduling
Proportional Share Scheduling aims to allocate resources to processes or users in proportion to their assigned weights or shares. This approach is particularly useful in scenarios where different users or applications have varying importance or priority levels.
Key features:
- Assigns a number of shares to each user or process
- Allocates resources proportionally to the number of shares
- Supports dynamic adjustment of shares based on changing priorities
Implementation considerations:
- Use a virtual time concept to track resource usage
- Implement efficient data structures (e.g., red-black trees) for managing processes and their shares
- Consider using lottery scheduling as a randomized approximation of proportional share scheduling
2. Multi-Resource Fairness
In many real-world scenarios, multiple types of resources (e.g., CPU, memory, network bandwidth) need to be shared simultaneously. Multi-Resource Fairness algorithms address this challenge by considering the allocation of multiple resources holistically.
Key concepts:
- Dominant Resource Fairness (DRF): Allocates resources based on the dominant (most demanded) resource for each user
- Generalized Resource Fairness: Extends DRF to handle more complex resource constraints and preferences
Implementation challenges:
- Determining the relative importance of different resource types
- Handling dynamic changes in resource demands
- Balancing fairness with overall system efficiency
3. Fair Queuing with Priority and Shaping
This advanced technique combines fair queuing with traffic shaping and priority mechanisms to provide more fine-grained control over resource allocation, especially in network environments.
Key components:
- Priority queues: Allow high-priority traffic to be served first
- Token bucket algorithm: Shapes traffic to conform to specified rate limits
- Hierarchical Fair Service Curve (HFSC): Provides service guarantees for both real-time and non-real-time traffic
Implementation example (Python):
import heapq
import time
class Packet:
def __init__(self, flow_id, size, priority, arrival_time):
self.flow_id = flow_id
self.size = size
self.priority = priority
self.arrival_time = arrival_time
self.finish_time = 0
class PriorityFairQueue:
def __init__(self, weights, rate_limits):
self.weights = weights
self.rate_limits = rate_limits
self.virtual_time = 0
self.queues = {flow_id: [] for flow_id in weights}
self.schedule = []
self.tokens = {flow_id: rate_limits[flow_id] for flow_id in rate_limits}
self.last_update = time.time()
def enqueue(self, packet):
self.queues[packet.flow_id].append(packet)
def update_tokens(self):
current_time = time.time()
elapsed = current_time - self.last_update
for flow_id in self.tokens:
self.tokens[flow_id] = min(
self.tokens[flow_id] + elapsed * self.rate_limits[flow_id],
self.rate_limits[flow_id]
)
self.last_update = current_time
def calculate_finish_time(self, packet):
flow_id = packet.flow_id
if not self.queues[flow_id]:
start_time = max(self.virtual_time, packet.arrival_time)
else:
start_time = max(self.virtual_time, self.queues[flow_id][-1].finish_time)
return start_time + (packet.size / (self.weights[flow_id] * packet.priority))
def schedule_packets(self):
self.update_tokens()
for flow_id, queue in self.queues.items():
if queue and self.tokens[flow_id] >= queue[0].size:
packet = queue[0]
packet.finish_time = self.calculate_finish_time(packet)
heapq.heappush(self.schedule, (packet.priority, packet.finish_time, packet))
while self.schedule:
_, finish_time, packet = heapq.heappop(self.schedule)
self.virtual_time = finish_time
self.tokens[packet.flow_id] -= packet.size
yield packet
self.queues[packet.flow_id].pop(0)
self.update_tokens()
if self.queues[packet.flow_id] and self.tokens[packet.flow_id] >= self.queues[packet.flow_id][0].size:
next_packet = self.queues[packet.flow_id][0]
next_packet.finish_time = self.calculate_finish_time(next_packet)
heapq.heappush(self.schedule, (next_packet.priority, next_packet.finish_time, next_packet))
# Example usage
weights = {"Flow1": 2, "Flow2": 1, "Flow3": 3}
rate_limits = {"Flow1": 100, "Flow2": 50, "Flow3": 150}
pfq = PriorityFairQueue(weights, rate_limits)
packets = [
Packet("Flow1", 80, 2, 0),
Packet("Flow2", 120, 1, 0),
Packet("Flow3", 100, 3, 0),
Packet("Flow1", 90, 2, 1),
Packet("Flow2", 60, 1, 2),
]
for packet in packets:
pfq.enqueue(packet)
for scheduled_packet in pfq.schedule_packets():
print(f"Scheduled: Flow {scheduled_packet.flow_id}, Size {scheduled_packet.size}, Priority {scheduled_packet.priority}, Finish Time {scheduled_packet.finish_time:.2f}")
Challenges and Future Directions
As technology evolves and resource sharing scenarios become more complex, several challenges and opportunities emerge in the field of fair resource sharing algorithms:
1. Scalability
With the increasing scale of distributed systems and cloud environments, designing algorithms that can efficiently handle millions of users or processes while maintaining fairness is a significant challenge.
Potential solutions:
- Hierarchical scheduling approaches
- Distributed fair sharing algorithms
- Approximation techniques for large-scale systems
2. Heterogeneous Resources
Modern computing environments often involve diverse types of resources with different characteristics (e.g., CPUs, GPUs, specialized hardware accelerators). Designing fair sharing algorithms that can handle this heterogeneity is an active area of research.
Research directions:
- Multi-dimensional resource allocation models
- Resource equivalence metrics for heterogeneous systems
- Adaptive algorithms that can learn resource relationships
3. Dynamic and Unpredictable Workloads
In many real-world scenarios, resource demands can change rapidly and unpredictably. Developing algorithms that can quickly adapt to these changes while maintaining fairness is crucial.
Approaches to explore:
- Online learning techniques for resource allocation
- Predictive models for anticipating workload changes
- Robust optimization methods for handling uncertainty
4. Energy-Aware Fair Sharing
As energy efficiency becomes increasingly important, integrating energy considerations into fair resource sharing algorithms is a growing area of interest.
Research opportunities:
- Energy-proportional fair sharing algorithms
- Trade-offs between fairness, performance, and energy consumption
- Green computing metrics for fair resource allocation
Conclusion
Designing algorithms for fair resource sharing is a critical and evolving field in computer science and engineering. From the foundational concepts of Round Robin and Weighted Fair Queuing to advanced techniques like Multi-Resource Fairness and energy-aware scheduling, the domain continues to expand to meet the challenges of modern computing environments.
As we’ve explored in this article, effective fair resource sharing algorithms must balance multiple objectives, adapt to diverse scenarios, and scale to meet the demands of large-scale systems. By understanding the core principles, implementing robust algorithms, and staying abreast of emerging techniques, developers and system designers can create more efficient, fair, and responsive systems that optimize resource utilization while ensuring equitable access for all users and processes.
As technology continues to advance, the field of fair resource sharing algorithms will undoubtedly see further innovations, addressing the challenges of scalability, heterogeneity, and dynamic workloads. By embracing these developments and continuing to refine our approaches, we can build more efficient, fair, and sustainable computing systems that benefit users across a wide range of applications and domains.