Deque in Python: A Comprehensive Guide to Efficient Data Structures
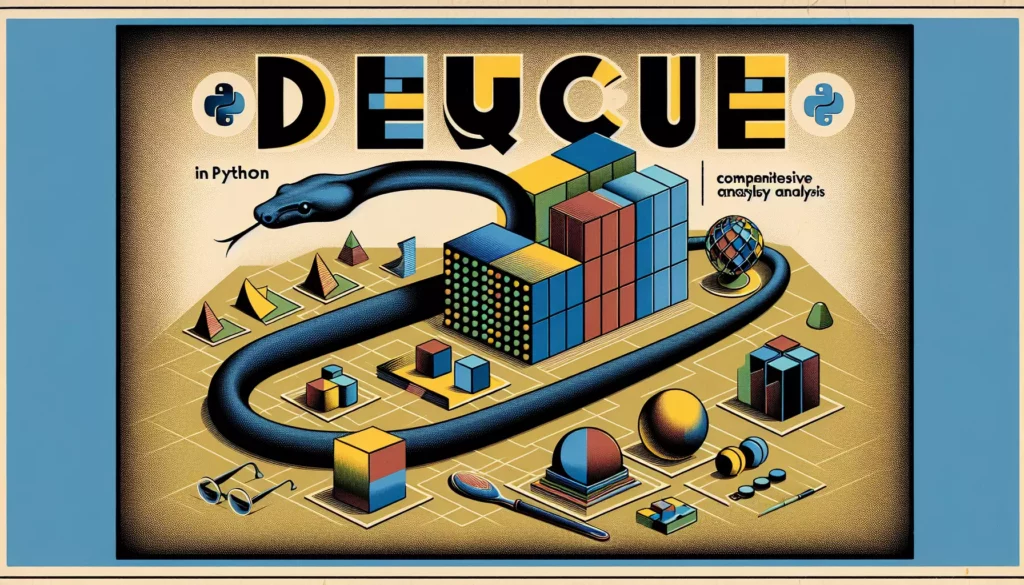
In the world of Python programming, efficient data structures play a crucial role in optimizing performance and solving complex problems. One such powerful data structure is the deque, short for “double-ended queue.” In this comprehensive guide, we’ll explore the deque data structure in Python, its features, use cases, and how it can improve your coding efficiency.
Table of Contents
- What is a Deque?
- Importing Deque in Python
- Creating a Deque
- Basic Operations with Deque
- Advanced Operations and Methods
- Deque vs. List: Performance Comparison
- Common Use Cases for Deque
- Tips and Tricks for Working with Deque
- Conclusion
1. What is a Deque?
A deque, pronounced “deck,” is a double-ended queue that allows efficient insertion and deletion of elements from both ends. It combines the features of a stack and a queue, providing a versatile data structure for various applications. The deque in Python is implemented as part of the collections
module and offers O(1) time complexity for append and pop operations on both ends.
Key characteristics of a deque include:
- Ability to add and remove elements from both ends
- Dynamic sizing, allowing it to grow or shrink as needed
- Thread-safe implementation
- Memory-efficient storage of elements
2. Importing Deque in Python
To use the deque data structure in Python, you need to import it from the collections
module. Here’s how you can do it:
from collections import deque
This import statement brings the deque class into your current namespace, allowing you to create and manipulate deque objects.
3. Creating a Deque
Creating a deque in Python is straightforward. You can initialize an empty deque or create one with initial values. Here are some examples:
# Creating an empty deque
empty_deque = deque()
# Creating a deque with initial values
numbers_deque = deque([1, 2, 3, 4, 5])
# Creating a deque with a string
string_deque = deque('Hello')
# Creating a deque with a maximum length (size-limited deque)
limited_deque = deque(maxlen=5)
In the last example, we create a size-limited deque with a maximum length of 5. When the deque reaches its maximum size, adding new elements will automatically remove elements from the opposite end to maintain the specified length.
4. Basic Operations with Deque
Deque supports various operations for adding, removing, and accessing elements. Let’s explore some of the basic operations:
4.1. Adding Elements
# Adding elements to the right end
numbers_deque = deque([1, 2, 3])
numbers_deque.append(4)
print(numbers_deque) # Output: deque([1, 2, 3, 4])
# Adding elements to the left end
numbers_deque.appendleft(0)
print(numbers_deque) # Output: deque([0, 1, 2, 3, 4])
# Adding multiple elements to the right end
numbers_deque.extend([5, 6, 7])
print(numbers_deque) # Output: deque([0, 1, 2, 3, 4, 5, 6, 7])
# Adding multiple elements to the left end
numbers_deque.extendleft([-2, -1])
print(numbers_deque) # Output: deque([-1, -2, 0, 1, 2, 3, 4, 5, 6, 7])
4.2. Removing Elements
# Removing elements from the right end
numbers_deque = deque([1, 2, 3, 4, 5])
popped_right = numbers_deque.pop()
print(popped_right) # Output: 5
print(numbers_deque) # Output: deque([1, 2, 3, 4])
# Removing elements from the left end
popped_left = numbers_deque.popleft()
print(popped_left) # Output: 1
print(numbers_deque) # Output: deque([2, 3, 4])
4.3. Accessing Elements
numbers_deque = deque([1, 2, 3, 4, 5])
# Accessing elements by index
print(numbers_deque[0]) # Output: 1
print(numbers_deque[-1]) # Output: 5
# Iterating through the deque
for item in numbers_deque:
print(item, end=' ') # Output: 1 2 3 4 5
5. Advanced Operations and Methods
Deque provides several advanced operations and methods that can be useful in various scenarios. Let’s explore some of them:
5.1. Rotating the Deque
The rotate()
method allows you to shift the elements of the deque to the right or left by a specified number of positions.
numbers_deque = deque([1, 2, 3, 4, 5])
# Rotate to the right by 2 positions
numbers_deque.rotate(2)
print(numbers_deque) # Output: deque([4, 5, 1, 2, 3])
# Rotate to the left by 1 position
numbers_deque.rotate(-1)
print(numbers_deque) # Output: deque([5, 1, 2, 3, 4])
5.2. Counting Elements
The count()
method returns the number of occurrences of a specified element in the deque.
numbers_deque = deque([1, 2, 2, 3, 2, 4, 2, 5])
count_2 = numbers_deque.count(2)
print(count_2) # Output: 4
5.3. Reversing the Deque
The reverse()
method reverses the order of elements in the deque in-place.
numbers_deque = deque([1, 2, 3, 4, 5])
numbers_deque.reverse()
print(numbers_deque) # Output: deque([5, 4, 3, 2, 1])
5.4. Clearing the Deque
The clear()
method removes all elements from the deque, leaving it empty.
numbers_deque = deque([1, 2, 3, 4, 5])
numbers_deque.clear()
print(numbers_deque) # Output: deque([])
5.5. Copying the Deque
The copy()
method creates a shallow copy of the deque.
original_deque = deque([1, 2, 3, 4, 5])
copied_deque = original_deque.copy()
print(copied_deque) # Output: deque([1, 2, 3, 4, 5])
6. Deque vs. List: Performance Comparison
One of the main advantages of using a deque over a regular Python list is its performance for certain operations. Let’s compare the time complexity of common operations between deque and list:
Operation | Deque | List |
---|---|---|
Append to right | O(1) | O(1) amortized |
Append to left | O(1) | O(n) |
Pop from right | O(1) | O(1) |
Pop from left | O(1) | O(n) |
Random access | O(n) | O(1) |
As we can see, deque outperforms list for operations involving the left end of the collection. This makes deque an excellent choice for scenarios where you need to frequently add or remove elements from both ends of the collection.
Here’s a simple benchmark to illustrate the performance difference:
import time
from collections import deque
def benchmark_append_left(data_structure, n):
start_time = time.time()
for i in range(n):
data_structure.insert(0, i) # For list
# data_structure.appendleft(i) # For deque
end_time = time.time()
return end_time - start_time
n = 100000
list_time = benchmark_append_left([], n)
deque_time = benchmark_append_left(deque(), n)
print(f"Time taken for list: {list_time:.6f} seconds")
print(f"Time taken for deque: {deque_time:.6f} seconds")
print(f"Deque is {list_time / deque_time:.2f}x faster")
Running this benchmark will show that deque is significantly faster than list for left-side operations, especially as the number of elements increases.
7. Common Use Cases for Deque
Deque is a versatile data structure that can be used in various scenarios. Here are some common use cases:
7.1. Implementing a Queue
Deque can be used to implement an efficient queue data structure, where elements are added to one end and removed from the other.
from collections import deque
class Queue:
def __init__(self):
self.items = deque()
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.popleft()
return None
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.dequeue()) # Output: 2
print(queue.size()) # Output: 1
7.2. Implementing a Stack
Deque can also be used to implement an efficient stack data structure, where elements are added and removed from the same end.
from collections import deque
class Stack:
def __init__(self):
self.items = deque()
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
return None
def is_empty(self):
return len(self.items) == 0
def size(self):
return len(self.items)
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.pop()) # Output: 2
print(stack.size()) # Output: 1
7.3. Sliding Window Problems
Deque is particularly useful for solving sliding window problems, where you need to maintain a window of elements and perform operations on it as it slides through a larger collection.
from collections import deque
def max_sliding_window(nums, k):
result = []
window = deque()
for i, num in enumerate(nums):
# Remove indices that are out of the current window
if window and window[0] == i - k:
window.popleft()
# Remove smaller elements from the right
while window and nums[window[-1]] < num:
window.pop()
window.append(i)
# Add the maximum of the current window to the result
if i >= k - 1:
result.append(nums[window[0]])
return result
# Example usage
nums = [1, 3, -1, -3, 5, 3, 6, 7]
k = 3
print(max_sliding_window(nums, k))
# Output: [3, 3, 5, 5, 6, 7]
7.4. Palindrome Checking
Deque can be used to efficiently check if a string is a palindrome by comparing characters from both ends.
from collections import deque
def is_palindrome(s):
char_deque = deque(c.lower() for c in s if c.isalnum())
while len(char_deque) > 1:
if char_deque.popleft() != char_deque.pop():
return False
return True
# Example usage
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
print(is_palindrome("race a car")) # Output: False
8. Tips and Tricks for Working with Deque
Here are some tips and tricks to help you make the most of the deque data structure in Python:
8.1. Use maxlen for Circular Buffers
When creating a deque with a fixed maximum length, it behaves like a circular buffer. This is useful for maintaining a sliding window of recent items.
from collections import deque
recent_items = deque(maxlen=5)
for i in range(10):
recent_items.append(i)
print(recent_items) # Output: deque([5, 6, 7, 8, 9], maxlen=5)
8.2. Combine rotate() and slicing for Efficient Rotation
You can use the rotate()
method in combination with slicing to efficiently rotate a portion of the deque.
from collections import deque
d = deque([1, 2, 3, 4, 5, 6, 7, 8, 9])
d.rotate(-2)
d[:5] = sorted(d[:5])
print(d) # Output: deque([3, 4, 5, 6, 7, 8, 9, 1, 2])
8.3. Use deque for File I/O
Deque can be useful for efficient line-by-line file reading, especially when you need to keep track of the last N lines.
from collections import deque
def tail(filename, n=10):
with open(filename, 'r') as f:
return deque(f, n)
# Usage
last_lines = tail('example.txt', 5)
for line in last_lines:
print(line.strip())
8.4. Implement a Circular List
You can use deque to implement a circular list, where indexing wraps around to the beginning when it reaches the end.
from collections import deque
class CircularList:
def __init__(self, iterable):
self.items = deque(iterable)
def __getitem__(self, index):
return self.items[index % len(self.items)]
def __len__(self):
return len(self.items)
# Usage
cl = CircularList([1, 2, 3, 4, 5])
print(cl[2]) # Output: 3
print(cl[5]) # Output: 1
print(cl[-1]) # Output: 5
8.5. Use deque for Breadth-First Search
Deque is an excellent choice for implementing breadth-first search algorithms in graph traversal.
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
# Example usage
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
bfs(graph, 'A') # Output: A B C D E F
9. Conclusion
The deque data structure in Python is a powerful and versatile tool that offers efficient operations for both ends of a collection. Its ability to perform O(1) insertions and deletions at both ends makes it an excellent choice for implementing queues, stacks, and solving various algorithmic problems.
Throughout this comprehensive guide, we’ve explored the basics of creating and manipulating deques, advanced operations, performance comparisons with lists, and common use cases. We’ve also provided tips and tricks to help you leverage the full potential of deques in your Python projects.
By mastering the deque data structure, you’ll be able to write more efficient and elegant code for a wide range of applications, from simple queue implementations to complex graph algorithms. As you continue to work with deques, you’ll discover even more creative ways to use this powerful data structure in your Python programming journey.