Deploying Your First Project: A Beginner’s Guide to Going Live
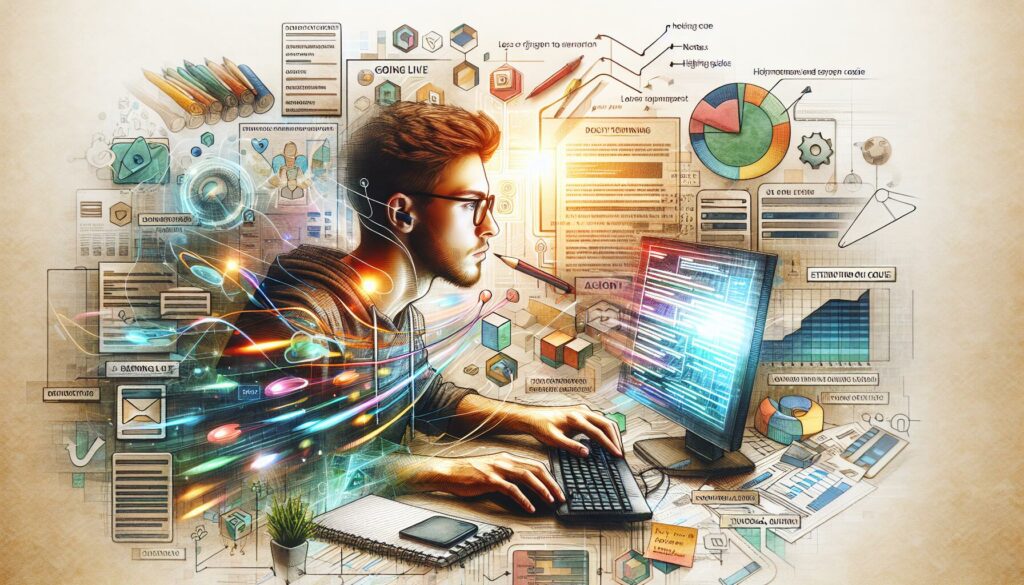
Welcome to the exciting world of project deployment! If you’ve been honing your coding skills and are ready to share your creation with the world, you’ve come to the right place. This comprehensive guide will walk you through the process of deploying your first project, from setting up cloud services to configuring domain names and SSL certificates. We’ll also cover best practices for managing deployments, handling updates, and monitoring performance. By the end of this article, you’ll have the knowledge and confidence to take your project live and showcase your hard work to the world.
Table of Contents
- Understanding Deployment
- Choosing a Cloud Service Provider
- Preparing Your Project for Deployment
- Setting Up Your Cloud Environment
- Deploying Your Project
- Configuring Your Domain Name
- Setting Up SSL Certificates
- Best Practices for Managing Deployments
- Handling Updates and Maintenance
- Monitoring Performance and Scaling
- Conclusion
1. Understanding Deployment
Before we dive into the technical details, let’s take a moment to understand what deployment means in the context of software development. Deployment is the process of making your application or website accessible to users over the internet. It involves taking your locally developed project and moving it to a server or cloud platform where it can be accessed by anyone with an internet connection.
Deployment typically involves several key steps:
- Preparing your code for a production environment
- Choosing and setting up a hosting platform
- Uploading your code to the server
- Configuring the server environment
- Setting up a domain name
- Ensuring security with SSL certificates
- Testing and monitoring the live application
Understanding these steps is crucial for a successful deployment, especially when you’re doing it for the first time. Let’s explore each of these aspects in detail.
2. Choosing a Cloud Service Provider
The first major decision you’ll need to make is choosing a cloud service provider. There are numerous options available, each with its own strengths and features. Here are some popular choices for beginners:
Amazon Web Services (AWS)
AWS is one of the most comprehensive and widely used cloud platforms. It offers a vast array of services, including EC2 for virtual servers, S3 for storage, and Lambda for serverless computing. AWS provides a free tier, making it an excellent choice for beginners to experiment without incurring costs.
Google Cloud Platform (GCP)
GCP offers a user-friendly interface and a range of services similar to AWS. It’s known for its strong data analytics and machine learning capabilities. GCP also provides a free tier and credits for new users.
Microsoft Azure
Azure is Microsoft’s cloud computing platform. It integrates well with other Microsoft products and offers a wide range of services. Azure provides free credits for new users and has a strong focus on enterprise solutions.
Heroku
Heroku is a platform-as-a-service (PaaS) that abstracts away much of the infrastructure management. It’s incredibly easy to use for beginners and supports a wide range of programming languages. Heroku offers a free tier, though with some limitations.
DigitalOcean
DigitalOcean provides virtual private servers (called “droplets”) at an affordable price. It’s known for its simplicity and developer-friendly approach. While it doesn’t offer a free tier, its pricing is straightforward and competitive.
When choosing a cloud provider, consider factors such as:
- Ease of use
- Cost (including free tier options)
- Scalability
- Available services and features
- Community support and documentation
- Compatibility with your project’s technology stack
For this guide, we’ll use AWS as an example, but the general principles apply to most cloud providers.
3. Preparing Your Project for Deployment
Before you can deploy your project, you need to ensure it’s ready for a production environment. Here are some key steps to prepare your project:
Version Control
If you haven’t already, set up version control for your project using Git. This will help you manage your code and make it easier to deploy and update your project. Create a repository on a platform like GitHub or GitLab to store your code.
Environment Variables
Use environment variables to store sensitive information like API keys, database credentials, and other configuration details. This keeps your sensitive data separate from your code and allows for easy configuration across different environments (development, staging, production).
Dependencies
Ensure all your project dependencies are properly documented. For Node.js projects, this means having an up-to-date package.json file. For Python, you might use a requirements.txt file. This allows your deployment environment to install the necessary packages.
Database Migrations
If your project uses a database, prepare any necessary database migrations. These scripts will set up your database schema in the production environment.
Static Files
Optimize and organize your static files (images, CSS, JavaScript). Consider using a content delivery network (CDN) for better performance.
Configuration Files
Create configuration files for different environments. For example, you might have separate config files for development and production settings.
Testing
Run thorough tests on your application to catch any bugs before deployment. This includes unit tests, integration tests, and if possible, end-to-end tests.
Build Process
If your project requires a build step (e.g., compiling TypeScript, bundling JavaScript), ensure you have a clear build process documented and automated if possible.
Example: Preparing a Node.js Application
Here’s an example of how you might structure a Node.js application for deployment:
project-root/
│
├── src/
│ ├── index.js
│ ├── routes/
│ └── models/
│
├── public/
│ ├── css/
│ ├── js/
│ └── images/
│
├── tests/
│
├── .env
├── .gitignore
├── package.json
├── README.md
└── Dockerfile
In this structure:
- The
src/
directory contains your application code. - The
public/
directory holds static assets. - The
tests/
directory contains your test files. - The
.env
file stores environment variables (make sure to add this to your .gitignore). - The
.gitignore
file specifies which files and directories should not be tracked by Git. - The
package.json
file lists your project dependencies and scripts. - The
README.md
file provides information about your project. - The
Dockerfile
defines how to build a Docker container for your application (if you’re using containerization).
4. Setting Up Your Cloud Environment
Now that your project is prepared, it’s time to set up your cloud environment. We’ll use AWS as an example, but the general steps are similar for other cloud providers.
Creating an AWS Account
- Go to the AWS website (aws.amazon.com) and click on “Create an AWS Account”.
- Follow the prompts to create your account. You’ll need to provide an email address, password, and credit card information (even for the free tier).
- Once your account is created, log in to the AWS Management Console.
Setting Up an EC2 Instance
EC2 (Elastic Compute Cloud) provides resizable compute capacity in the cloud. Here’s how to set up an EC2 instance:
- From the AWS Management Console, navigate to EC2.
- Click “Launch Instance”.
- Choose an Amazon Machine Image (AMI). For a Node.js application, you might choose an Ubuntu Server AMI.
- Select an instance type. The t2.micro is eligible for the free tier and is suitable for many small projects.
- Configure instance details, add storage, and add tags as needed.
- Configure the security group to allow inbound traffic on ports 22 (SSH), 80 (HTTP), and 443 (HTTPS).
- Review and launch the instance.
- Create a new key pair or use an existing one. This key pair will allow you to securely connect to your instance.
Connecting to Your EC2 Instance
Once your instance is running, you can connect to it using SSH:
ssh -i /path/to/your-key-pair.pem ec2-user@your-instance-public-dns
Replace /path/to/your-key-pair.pem
with the path to your key pair file, and your-instance-public-dns
with your instance’s public DNS name, which you can find in the EC2 dashboard.
Setting Up the Server Environment
Once connected to your EC2 instance, you’ll need to set up the environment for your application. For a Node.js application, this might involve:
- Updating the system:
sudo apt update && sudo apt upgrade -y
- Installing Node.js and npm:
curl -sL https://deb.nodesource.com/setup_14.x | sudo -E bash - sudo apt-get install -y nodejs
- Installing any other necessary software (e.g., database systems, web servers).
5. Deploying Your Project
With your cloud environment set up, you’re ready to deploy your project. Here’s a general process for deploying a Node.js application:
Transferring Your Code
You can transfer your code to the EC2 instance using various methods. One common approach is to use Git:
- Install Git on your EC2 instance:
sudo apt-get install git
- Clone your repository:
git clone https://github.com/your-username/your-repo.git
Installing Dependencies
Navigate to your project directory and install dependencies:
cd your-repo
npm install
Setting Up Environment Variables
Create a .env
file in your project directory and add your environment variables:
touch .env
nano .env
Add your variables, for example:
PORT=3000
DATABASE_URL=mongodb://localhost:27017/your-database
API_KEY=your-api-key
Starting Your Application
You can start your application using Node.js directly, but for production, it’s better to use a process manager like PM2:
- Install PM2:
npm install pm2 -g
- Start your application:
pm2 start src/index.js --name your-app-name
- Configure PM2 to start on system reboot:
pm2 startup systemd sudo env PATH=$PATH:/usr/bin /usr/lib/node_modules/pm2/bin/pm2 startup systemd -u ubuntu --hp /home/ubuntu pm2 save
Setting Up a Reverse Proxy
To handle incoming HTTP requests and forward them to your Node.js application, you’ll need to set up a reverse proxy. Nginx is a popular choice:
- Install Nginx:
sudo apt-get install nginx
- Create a new Nginx configuration file:
sudo nano /etc/nginx/sites-available/your-app
- Add the following configuration (adjust as needed):
server { listen 80; server_name your-domain.com; location / { proxy_pass http://localhost:3000; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } }
- Create a symbolic link to enable the site:
sudo ln -s /etc/nginx/sites-available/your-app /etc/nginx/sites-enabled/
- Test the Nginx configuration:
sudo nginx -t
- If the test is successful, restart Nginx:
sudo systemctl restart nginx
6. Configuring Your Domain Name
To make your application accessible via a user-friendly domain name, you’ll need to configure your domain settings:
Purchasing a Domain
If you don’t already have a domain, you can purchase one from a domain registrar like Namecheap, GoDaddy, or Google Domains.
Configuring DNS
- In your domain registrar’s dashboard, locate the DNS settings.
- Add an A record that points to your EC2 instance’s public IP address. For example:
Type: A Host: @ Value: your-ec2-public-ip TTL: 3600
- If you want to use a subdomain (e.g., www), add a CNAME record:
Type: CNAME Host: www Value: your-domain.com TTL: 3600
DNS changes can take up to 48 hours to propagate, but often occur much faster.
7. Setting Up SSL Certificates
Securing your site with HTTPS is crucial for protecting user data and improving search engine rankings. Let’s Encrypt provides free SSL certificates that are easy to set up:
- Install Certbot, a tool for obtaining Let’s Encrypt certificates:
sudo apt-get update sudo apt-get install certbot python3-certbot-nginx
- Obtain and install a certificate:
sudo certbot --nginx -d your-domain.com -d www.your-domain.com
- Follow the prompts to complete the certificate installation.
- Certbot will automatically modify your Nginx configuration to use the new certificate.
- Test automatic renewal:
sudo certbot renew --dry-run
Certbot sets up a cron job to automatically renew your certificates before they expire.
8. Best Practices for Managing Deployments
As you become more comfortable with deployments, consider adopting these best practices:
Use Version Control
Always use version control (like Git) to track changes to your codebase. This allows you to easily roll back changes if needed and collaborate with others.
Implement Continuous Integration/Continuous Deployment (CI/CD)
Set up a CI/CD pipeline to automate your testing and deployment process. Tools like Jenkins, GitLab CI, or GitHub Actions can help with this.
Use Environment-Specific Configurations
Maintain separate configurations for development, staging, and production environments. This helps prevent accidental changes to production settings.
Implement Logging
Set up comprehensive logging for your application. This will help you diagnose issues in production. Consider using a centralized logging service like ELK Stack (Elasticsearch, Logstash, Kibana) or a managed service like Loggly.
Regular Backups
Implement a backup strategy for your data and configurations. Regularly test your ability to restore from these backups.
Security Best Practices
- Keep your systems and dependencies up to date.
- Use strong, unique passwords and consider implementing two-factor authentication.
- Regularly audit your security settings and access logs.
- Use the principle of least privilege when setting up user accounts and permissions.
9. Handling Updates and Maintenance
Maintaining your deployed application is an ongoing process. Here are some tips for handling updates and maintenance:
Rolling Updates
When deploying updates, consider using a rolling update strategy to minimize downtime. This involves updating instances one at a time, ensuring that your service remains available throughout the update process.
Database Migrations
When making changes to your database schema, use migration scripts to apply these changes. This ensures that your database stays in sync with your application code.
Monitoring and Alerts
Set up monitoring for your application and infrastructure. Use tools like AWS CloudWatch or Prometheus to track key metrics and set up alerts for any anomalies.
Regular Maintenance
Schedule regular maintenance windows for tasks like system updates, database optimizations, and security audits.
Rollback Plan
Always have a rollback plan in case an update causes unexpected issues. This might involve keeping the previous version of your application ready to deploy or maintaining database snapshots.
10. Monitoring Performance and Scaling
As your application grows, you’ll need to monitor its performance and potentially scale your infrastructure:
Performance Monitoring
Use application performance monitoring (APM) tools like New Relic, Datadog, or AWS X-Ray to gain insights into your application’s performance. These tools can help you identify bottlenecks and optimize your code.
Load Testing
Regularly perform load tests to understand how your application behaves under stress. Tools like Apache JMeter or Gatling can simulate high traffic scenarios.
Scaling Strategies
- Vertical Scaling: Increase the resources (CPU, RAM) of your existing servers.
- Horizontal Scaling: Add more servers to distribute the load.
- Auto Scaling: Use cloud provider features like AWS Auto Scaling to automatically adjust the number of instances based on demand.
Caching
Implement caching strategies to reduce database load and improve response times. Consider using Redis or Memcached for in-memory caching.
Content Delivery Network (CDN)
Use a CDN like Cloudflare or AWS CloudFront to serve static assets from locations closer to your users, reducing latency and improving load times.
11. Conclusion
Congratulations! You’ve now learned the essentials of deploying your first project. From setting up your cloud environment to configuring your domain and implementing best practices, you’re well on your way to becoming a deployment expert.
Remember, deployment is an ongoing process. As your project grows and evolves, you’ll continue to refine your deployment strategies and learn new techniques. Stay curious, keep learning, and don’t be afraid to experiment with new tools and approaches.
Deployment can seem daunting at first, but with practice, it becomes an integral and exciting part of the development process. It’s the bridge that takes your code from your local machine to the world, allowing you to share your creations with users across the globe.
As you continue your journey in software development, remember that platforms like AlgoCademy are here to support you. Whether you’re working on algorithmic challenges, preparing for technical interviews, or exploring advanced deployment techniques, there’s always more to learn and discover in the world of coding.
Happy deploying, and may your projects reach new heights!