Debunking Myths: Coding Interviews Aren’t That Scary
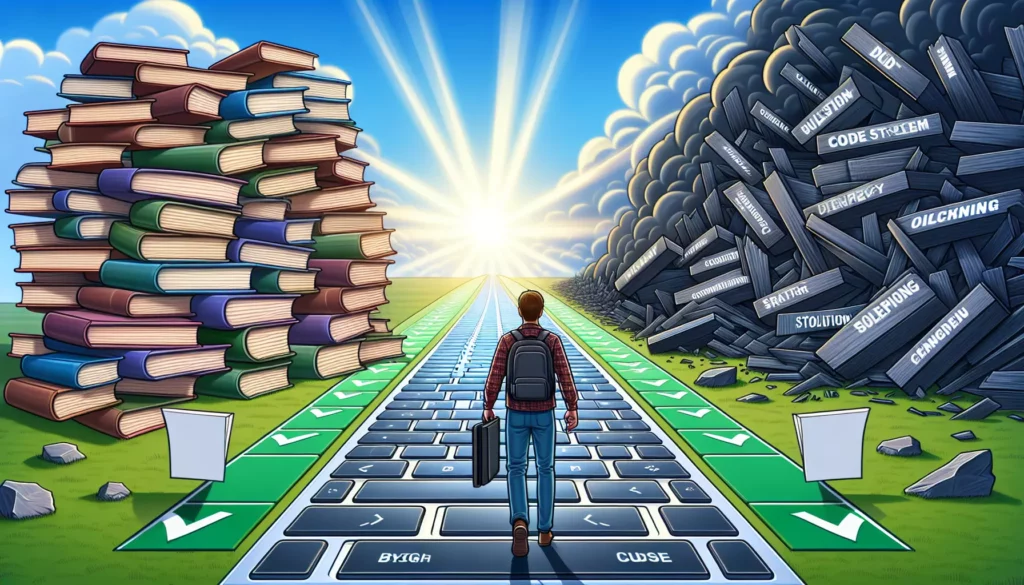
For many aspiring software engineers and developers, the mere thought of a coding interview can send shivers down their spine. The reputation of these technical assessments has grown to mythical proportions, often painted as grueling ordeals designed to break even the most talented programmers. However, it’s time to pull back the curtain and reveal the truth: coding interviews aren’t as terrifying as they’re made out to be. In this comprehensive guide, we’ll debunk common myths, provide practical strategies, and offer reassurance to those preparing for their next (or first) coding interview.
The Evolution of Coding Interviews
Before we dive into debunking myths, it’s essential to understand how coding interviews have evolved. In the early days of tech recruitment, interviews were often unstructured and relied heavily on puzzle-solving abilities that didn’t necessarily correlate with on-the-job performance. However, as the industry matured, so did the interview process.
Today’s coding interviews are designed to assess a candidate’s problem-solving skills, coding ability, and thought process. They’re not meant to be impossible challenges but rather opportunities for candidates to showcase their skills and potential.
Common Myths About Coding Interviews
Let’s address some of the most prevalent myths surrounding coding interviews and why they’re not as accurate as you might think:
Myth 1: You Need to Know Every Algorithm Under the Sun
Reality: While having a solid understanding of fundamental algorithms is important, interviewers are more interested in your problem-solving approach than your ability to recite obscure algorithms from memory.
Most coding interviews focus on common data structures and algorithms that are widely used in software development. These typically include:
- Arrays and strings
- Linked lists
- Trees and graphs
- Stacks and queues
- Sorting and searching algorithms
- Dynamic programming (in some cases)
Understanding these core concepts and being able to apply them to solve problems is far more valuable than memorizing every algorithm in existence.
Myth 2: You Must Provide a Perfect Solution Immediately
Reality: Interviewers are often more interested in your problem-solving process than in seeing a flawless solution right away.
In fact, many interviewers appreciate candidates who think out loud, ask clarifying questions, and iterate on their solutions. This demonstrates:
- Critical thinking skills
- Ability to communicate complex ideas
- Adaptability and willingness to improve
Remember, in real-world software development, problems are rarely solved in isolation or on the first try. The ability to collaborate, seek feedback, and refine solutions is highly valued.
Myth 3: Only Geniuses Pass Coding Interviews
Reality: Success in coding interviews is more about preparation, practice, and perseverance than innate genius.
Many successful software engineers will tell you that they’ve failed interviews before eventually landing their dream jobs. The key is to:
- Consistently practice coding problems
- Learn from each interview experience
- Develop a growth mindset
Platforms like AlgoCademy are designed to help learners of all levels improve their skills and prepare for coding interviews through structured learning paths and interactive problem-solving exercises.
Myth 4: Coding Interviews Are Just About Writing Code
Reality: While coding is a crucial component, interviews often assess a range of skills beyond just writing code.
Modern coding interviews may include:
- System design discussions
- Behavioral questions
- Collaborative problem-solving sessions
- Code reviews
These diverse elements aim to evaluate a candidate’s ability to work in a team, communicate effectively, and think about larger architectural concerns—all essential skills for a successful software engineer.
Strategies for Coding Interview Success
Now that we’ve dispelled some common myths, let’s explore strategies to help you approach coding interviews with confidence:
1. Practice, Practice, Practice
Consistent practice is the cornerstone of interview preparation. Here’s how to make the most of your practice sessions:
- Solve a variety of problems: Focus on different data structures and algorithms to broaden your problem-solving toolkit.
- Time yourself: Many interviews have time constraints, so get comfortable solving problems under pressure.
- Use platforms like AlgoCademy: Take advantage of structured learning paths and AI-powered assistance to guide your practice.
2. Master the Art of Problem-Solving
Develop a systematic approach to tackling coding problems:
- Clarify the problem: Ask questions to ensure you understand the requirements fully.
- Break it down: Divide the problem into smaller, manageable sub-problems.
- Plan your approach: Outline your solution before diving into coding.
- Code with clarity: Write clean, readable code that’s easy to understand and maintain.
- Test your solution: Consider edge cases and potential issues in your implementation.
3. Communicate Effectively
Clear communication is crucial during coding interviews:
- Think out loud: Share your thought process as you work through the problem.
- Explain your decisions: Justify why you chose a particular approach or data structure.
- Be open to feedback: Show that you can incorporate suggestions and improve your solution.
4. Learn from Your Mistakes
Every interview, whether successful or not, is a learning opportunity:
- Reflect on your performance: Identify areas where you struggled or could improve.
- Seek feedback: Ask interviewers for constructive criticism when possible.
- Revisit challenging problems: After the interview, try to solve any problems that gave you difficulty.
Real-World Coding Interview Examples
To further demystify the coding interview process, let’s look at some examples of the types of problems you might encounter:
Example 1: String Manipulation
Problem: Write a function to determine if two strings are anagrams of each other.
This problem tests your ability to work with strings and basic data structures. Here’s a Python solution:
def are_anagrams(str1, str2):
# Remove spaces and convert to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if lengths are different
if len(str1) != len(str2):
return False
# Create character frequency dictionaries
char_count1 = {}
char_count2 = {}
# Count character frequencies
for char in str1:
char_count1[char] = char_count1.get(char, 0) + 1
for char in str2:
char_count2[char] = char_count2.get(char, 0) + 1
# Compare frequency dictionaries
return char_count1 == char_count2
# Test the function
print(are_anagrams("listen", "silent")) # True
print(are_anagrams("hello", "world")) # False
This solution demonstrates:
- String manipulation
- Use of dictionaries (hash maps)
- Attention to edge cases (different lengths, case sensitivity)
Example 2: Tree Traversal
Problem: Implement an in-order traversal of a binary tree.
This problem tests your understanding of tree data structures and recursive algorithms. Here’s a Java solution:
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
}
}
class Solution {
public List<Integer> inorderTraversal(TreeNode root) {
List<Integer> result = new ArrayList<>();
inorderHelper(root, result);
return result;
}
private void inorderHelper(TreeNode node, List<Integer> result) {
if (node == null) {
return;
}
inorderHelper(node.left, result);
result.add(node.val);
inorderHelper(node.right, result);
}
}
// Usage
TreeNode root = new TreeNode(1);
root.left = new TreeNode(2);
root.right = new TreeNode(3);
root.left.left = new TreeNode(4);
root.left.right = new TreeNode(5);
Solution solution = new Solution();
List<Integer> traversalResult = solution.inorderTraversal(root);
System.out.println(traversalResult); // [4, 2, 5, 1, 3]
This solution showcases:
- Understanding of tree structures
- Recursive problem-solving
- Proper use of helper methods
Preparing for System Design Questions
As mentioned earlier, many coding interviews now include system design questions, especially for more senior positions. These questions assess your ability to think about large-scale software architecture and design. Here are some tips for tackling system design questions:
1. Clarify Requirements
Start by asking questions to understand the scope and constraints of the system you’re designing. Consider:
- Scale: How many users? How much data?
- Features: What are the core functionalities?
- Performance: What are the latency requirements?
- Reliability: What level of availability is needed?
2. Outline High-Level Architecture
Sketch out the main components of your system. This might include:
- Client-side components
- Application servers
- Databases
- Caching layers
- Load balancers
- Content Delivery Networks (CDNs)
3. Deep Dive into Components
Choose key components to discuss in more detail. Explain your choices for:
- Data storage (SQL vs NoSQL)
- Caching strategies
- API design
- Scalability solutions (horizontal vs vertical scaling)
4. Address Bottlenecks and Trade-offs
Discuss potential issues and how you’d address them:
- Single points of failure
- Data consistency vs availability
- Read vs write performance
Behavioral Interview Preparation
Many coding interviews also include behavioral questions to assess your soft skills and cultural fit. Here are some tips for preparing:
1. Use the STAR Method
When answering behavioral questions, use the STAR method:
- Situation: Describe the context.
- Task: Explain your responsibility.
- Action: Detail the steps you took.
- Result: Share the outcome and what you learned.
2. Prepare Stories
Have a few relevant stories ready that demonstrate:
- Leadership and teamwork
- Handling conflicts
- Overcoming technical challenges
- Learning from failures
3. Research the Company
Understand the company’s values and culture to align your responses accordingly.
The Role of Platforms Like AlgoCademy
Preparing for coding interviews can be overwhelming, but platforms like AlgoCademy are designed to make the process more manageable and less intimidating. Here’s how AlgoCademy can help:
1. Structured Learning Paths
AlgoCademy offers curated learning paths that guide you through essential topics, ensuring you cover all the necessary ground for interview preparation.
2. Interactive Problem-Solving
Practice coding problems in an environment that simulates real interview conditions, complete with time tracking and immediate feedback.
3. AI-Powered Assistance
Get personalized hints and explanations when you’re stuck, helping you learn and improve rather than getting discouraged.
4. Progress Tracking
Monitor your improvement over time and identify areas that need more focus.
5. Community Support
Connect with other learners, share experiences, and learn from peers who are on the same journey.
Conclusion: Embrace the Challenge
Coding interviews may seem daunting, but they’re not insurmountable obstacles. By debunking common myths, understanding what to expect, and preparing systematically, you can approach these interviews with confidence and even excitement.
Remember:
- Interviews are opportunities to showcase your skills and learn.
- Consistent practice and a growth mindset are key to success.
- Communication and problem-solving skills are just as important as coding ability.
- Every interview, regardless of the outcome, is a valuable learning experience.
By leveraging resources like AlgoCademy and applying the strategies discussed in this article, you can transform coding interviews from intimidating hurdles into exciting challenges that propel your career forward. Embrace the process, stay curious, and keep coding. Your dream job in tech is within reach!