Debugging Your Own Code: A Guide to Fixing Problems When You’re Stuck
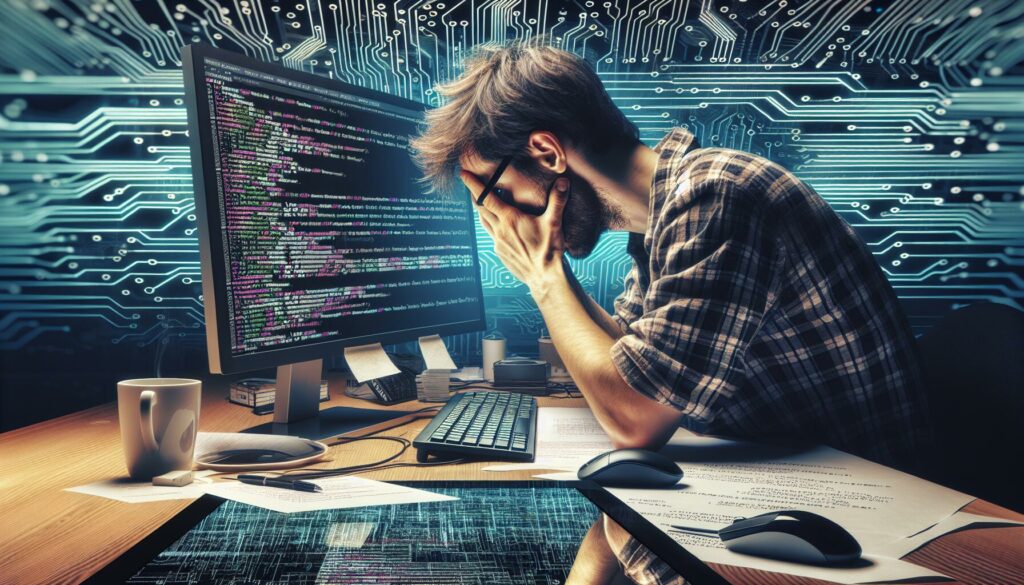
As a programmer, you’ll inevitably encounter bugs in your code. Whether you’re a beginner or an experienced developer, debugging is an essential skill that can save you hours of frustration and help you become a more efficient coder. In this comprehensive guide, we’ll explore various strategies for debugging your own code, with a focus on self-coded projects. We’ll cover the use of IDE tools, logging techniques, and how to test edge cases effectively. Along the way, we’ll provide real-world examples of common issues that arise when building projects from scratch.
Understanding the Importance of Debugging
Before we dive into specific debugging techniques, it’s crucial to understand why debugging is such an important skill for programmers. Debugging is not just about fixing errors; it’s about understanding how your code works, identifying potential issues, and improving your overall coding practices.
Here are some key reasons why mastering debugging is essential:
- Saves time and reduces frustration
- Improves code quality and reliability
- Enhances problem-solving skills
- Helps in understanding complex systems
- Prepares you for technical interviews and real-world scenarios
Setting Up Your Debugging Environment
Before you start debugging, it’s important to set up your development environment properly. This includes choosing the right Integrated Development Environment (IDE) and configuring it for optimal debugging.
Choosing the Right IDE
A good IDE can significantly streamline your debugging process. Some popular IDEs include:
- Visual Studio Code (VS Code): A versatile, lightweight IDE that supports multiple programming languages
- PyCharm: Ideal for Python development
- IntelliJ IDEA: Great for Java and other JVM languages
- Xcode: For iOS and macOS development
- Eclipse: A popular choice for Java development
Configuring Your IDE for Debugging
Once you’ve chosen your IDE, take some time to configure it for debugging. This typically involves:
- Setting up breakpoints
- Configuring watch variables
- Customizing the debug console
- Setting up keyboard shortcuts for common debugging actions
For example, in VS Code, you can set a breakpoint by clicking on the gutter (the area to the left of the line numbers) or by using the keyboard shortcut F9. To start debugging, you can press F5 or use the “Run and Debug” view in the sidebar.
Essential Debugging Strategies
Now that we have our environment set up, let’s explore some essential debugging strategies that can help you tackle various coding issues.
1. Using Breakpoints Effectively
Breakpoints are one of the most powerful tools in a debugger’s arsenal. They allow you to pause the execution of your code at specific points, giving you the opportunity to inspect variables, step through code line by line, and understand the flow of your program.
Here’s an example of how to use breakpoints effectively:
def calculate_factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * calculate_factorial(n - 1)
# Set a breakpoint on the line below
result = calculate_factorial(5)
print(f"The factorial of 5 is: {result}")
In this example, you would set a breakpoint on the line where the calculate_factorial
function is called. When you run the debugger, it will pause at this point, allowing you to step into the function and observe how the recursive calls work.
2. Step-by-Step Execution
Most debuggers offer different ways to step through your code:
- Step Over: Execute the current line and move to the next line
- Step Into: If the current line contains a function call, step into that function
- Step Out: If you’re inside a function, execute the rest of the function and return to the calling code
Using these stepping commands, you can navigate through your code and observe its behavior in detail.
3. Watching Variables
Variable watching allows you to track the values of specific variables as your code executes. This is particularly useful when dealing with complex algorithms or data structures.
For instance, if you’re implementing a sorting algorithm, you might want to watch the array being sorted at each step:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Set a breakpoint here and watch the 'arr' variable
sorted_array = bubble_sort([64, 34, 25, 12, 22, 11, 90])
print(f"Sorted array: {sorted_array}")
By watching the arr
variable, you can observe how the array changes after each swap operation.
4. Using Print Statements for Debugging
While not as sophisticated as using a debugger, print statements can be a quick and effective way to debug your code, especially for simple issues or when you’re working in an environment where a full debugger isn’t available.
Here’s an example of using print statements to debug a function:
def divide_numbers(a, b):
print(f"Dividing {a} by {b}") # Debug print
if b == 0:
print("Error: Division by zero!") # Debug print
return None
result = a / b
print(f"Result: {result}") # Debug print
return result
# Test the function
print(divide_numbers(10, 2))
print(divide_numbers(10, 0))
These print statements help you understand the flow of the function and identify potential issues, such as division by zero.
5. Logging for More Complex Debugging
For larger projects or when debugging in production environments, using a logging framework is often more appropriate than print statements. Python’s built-in logging
module is a great example:
import logging
logging.basicConfig(level=logging.DEBUG, format='%(asctime)s - %(levelname)s - %(message)s')
def complex_function(x, y):
logging.debug(f"Starting complex_function with x={x}, y={y}")
try:
result = x / y
logging.info(f"Operation successful. Result: {result}")
return result
except ZeroDivisionError:
logging.error("Division by zero occurred")
return None
# Test the function
complex_function(10, 2)
complex_function(10, 0)
Logging provides several advantages over print statements:
- Different log levels (DEBUG, INFO, WARNING, ERROR, CRITICAL)
- Timestamp information
- Ability to log to files or other outputs
- Can be easily enabled/disabled without removing code
Advanced Debugging Techniques
As you become more comfortable with basic debugging techniques, you can start exploring more advanced strategies to tackle complex issues.
1. Debugging Multithreaded Code
Debugging multithreaded code can be challenging due to race conditions and synchronization issues. Many IDEs offer special tools for debugging multithreaded applications. For example, in PyCharm, you can use the “Threads” view to see all active threads and switch between them during debugging.
Here’s an example of a simple multithreaded program that might need debugging:
import threading
import time
counter = 0
def increment_counter():
global counter
local_counter = counter
time.sleep(0.1) # Simulate some work
counter = local_counter + 1
threads = []
for _ in range(10):
thread = threading.Thread(target=increment_counter)
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
print(f"Final counter value: {counter}")
When debugging this code, you might need to set breakpoints inside the increment_counter
function and examine how different threads interact with the shared counter
variable.
2. Remote Debugging
Remote debugging allows you to debug code running on a different machine or in a different environment. This is particularly useful when debugging issues that only occur in production or on specific hardware.
For example, VS Code supports remote debugging for Python. You would typically:
- Install the ptvsd package on the remote machine
- Modify your code to enable the debug server
- Configure VS Code to connect to the remote debugger
Here’s a simple example of how you might set up remote debugging in your Python code:
import ptvsd
# Allow other computers to attach to ptvsd at this IP address and port
ptvsd.enable_attach(address=("0.0.0.0", 5678))
# Pause the program until a remote debugger is attached
ptvsd.wait_for_attach()
# Your code here
print("Remote debugging is now enabled")
3. Debugging Memory Issues
Memory-related bugs, such as memory leaks or buffer overflows, can be some of the most challenging to debug. Tools like Valgrind for C/C++ or memory_profiler for Python can help identify these issues.
For example, using memory_profiler in Python:
from memory_profiler import profile
@profile
def memory_hungry_function():
big_list = [i for i in range(1000000)]
del big_list
memory_hungry_function()
Running this script with python -m memory_profiler script.py
will show you a line-by-line analysis of memory usage.
Common Debugging Scenarios and Solutions
Let’s look at some common debugging scenarios you might encounter when building projects from scratch, along with strategies to solve them.
1. Infinite Loops
Infinite loops are a common issue, especially when working with recursive functions or complex loop conditions.
Example of an infinite loop:
def count_down(n):
while n > 0:
print(n)
n + 1 # Oops! Should be n - 1
count_down(5)
To debug this:
- Set a breakpoint inside the loop
- Use the debugger to step through the loop and watch the value of
n
- You’ll quickly see that
n
is increasing instead of decreasing
2. Off-by-One Errors
Off-by-one errors are mistakes in the boundary conditions of loops or array indexing.
Example:
def print_list_items(lst):
for i in range(len(lst)):
print(lst[i + 1]) # Oops! This will cause an IndexError
print_list_items([1, 2, 3, 4, 5])
To debug this:
- Set a breakpoint at the beginning of the function
- Step through the loop, watching the values of
i
andlst[i + 1]
- You’ll see that on the last iteration,
i + 1
is out of bounds
3. Unexpected Type Errors
In dynamically typed languages like Python, type errors can sometimes be surprising.
Example:
def add_numbers(a, b):
return a + b
result = add_numbers(5, "10")
print(result)
To debug this:
- Set a breakpoint inside the
add_numbers
function - Inspect the types of
a
andb
- You’ll see that
b
is a string, not a number
4. Debugging API Calls
When working with external APIs, it’s important to debug both the request you’re sending and the response you’re receiving.
Example:
import requests
def get_user_data(user_id):
response = requests.get(f"https://api.example.com/users/{user_id}")
return response.json()
user_data = get_user_data(123)
print(user_data['name'])
To debug this:
- Set a breakpoint after the API call
- Inspect the
response
object to check the status code and raw content - Examine the structure of the parsed JSON to ensure it matches your expectations
Best Practices for Effective Debugging
As you develop your debugging skills, keep these best practices in mind:
1. Reproduce the Bug Consistently
Before you start debugging, make sure you can reliably reproduce the issue. Create a minimal test case that demonstrates the problem.
2. Isolate the Problem
Try to narrow down the source of the bug as much as possible. Comment out sections of code or use binary search to identify the problematic area.
3. Use Version Control
Tools like Git can be invaluable for debugging. You can use git bisect
to find which commit introduced a bug, or easily revert to a working state if needed.
4. Write Unit Tests
Unit tests can help prevent bugs and make it easier to isolate issues when they do occur. Here’s a simple example using Python’s unittest
framework:
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-2, -3), -5)
def test_add_mixed_numbers(self):
self.assertEqual(add_numbers(-2, 3), 1)
if __name__ == '__main__':
unittest.main()
5. Use Debugging Tools Wisely
While print statements can be useful, try to leverage the full power of your IDE’s debugging tools. Learn keyboard shortcuts and advanced features to speed up your debugging process.
6. Take Breaks
Sometimes, the best debugging technique is to step away from the problem for a while. A fresh perspective can often lead to new insights.
Conclusion
Debugging is an essential skill for any programmer, and mastering it can significantly improve your coding efficiency and the quality of your projects. By understanding and applying the strategies discussed in this guide, you’ll be better equipped to tackle a wide range of coding challenges.
Remember that debugging is not just about fixing errors—it’s about understanding your code more deeply and improving your problem-solving skills. As you continue to practice and refine your debugging techniques, you’ll find that you’re not only fixing bugs more quickly but also writing better, more robust code from the start.
Whether you’re preparing for technical interviews, working on personal projects, or contributing to large-scale applications, the debugging skills you develop will serve you well throughout your programming career. Keep practicing, stay curious, and don’t be afraid to dive deep into your code to uncover and resolve issues. Happy debugging!