Debugging Strategies Every Programmer Should Know: Fix Bugs Faster During Coding Interviews
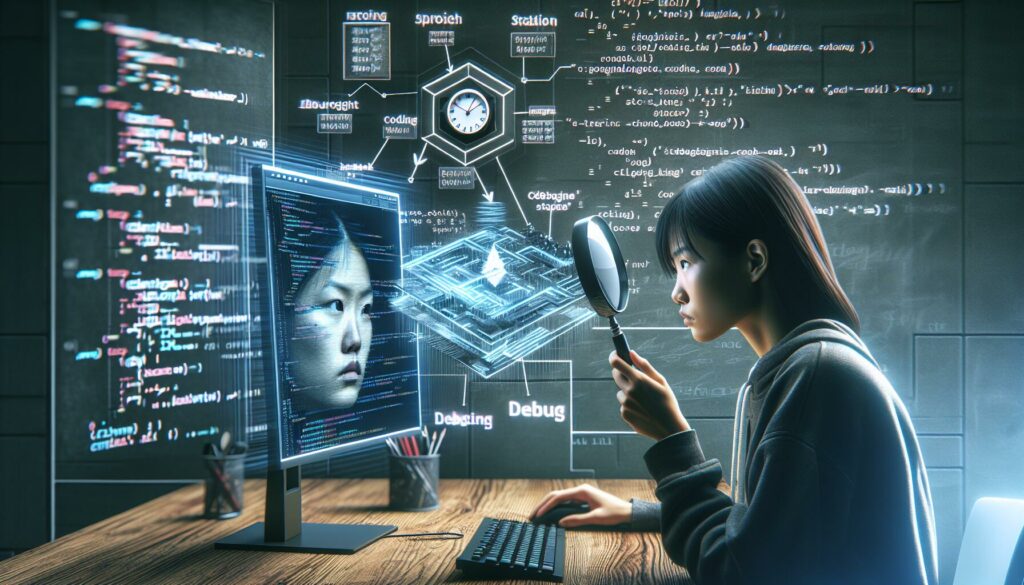
Debugging is an essential skill for every programmer, and it becomes even more crucial during coding interviews. The ability to quickly identify and fix bugs can make the difference between a successful interview and a missed opportunity. In this comprehensive guide, we’ll explore effective debugging strategies that will help you tackle bugs efficiently, especially in high-pressure interview situations.
Why Debugging Skills Matter in Coding Interviews
Before we dive into specific strategies, let’s understand why strong debugging skills are vital for coding interviews:
- Time Pressure: Interviews often have strict time limits, making quick bug identification crucial.
- Problem Complexity: Interview questions can be intricate, increasing the likelihood of bugs.
- Demonstration of Skills: Effective debugging showcases your problem-solving abilities and attention to detail.
- Realistic Scenarios: Debugging is a common task in real-world programming, making it a relevant interview skill.
Essential Debugging Strategies
1. Use Print Statements Effectively
Print statements are one of the simplest yet most powerful debugging tools at your disposal. They allow you to inspect the state of your program at various points during execution.
Best Practices for Using Print Statements:
- Be Strategic: Place print statements at key points in your code, such as function entry/exit points or before/after critical operations.
- Use Descriptive Labels: Include clear labels with your print statements to easily identify where the output is coming from.
- Print Variable Values: Output the values of relevant variables to understand their state at different stages of execution.
- Format Output: Use formatting to make your debug output easy to read and distinguish from regular program output.
Example:
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
print(f"DEBUG: left={left}, right={right}, mid={mid}, arr[mid]={arr[mid]}")
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Test the function
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
result = binary_search(arr, target)
print(f"Result: {result}")
In this example, the print statement inside the while loop helps you track the progress of the binary search algorithm, making it easier to identify any issues with the search logic.
2. Divide and Conquer: Isolate the Problem
When faced with a complex bug, breaking down the problem into smaller, manageable parts can be extremely helpful. This approach allows you to narrow down the source of the issue more quickly.
Steps for Dividing the Problem Space:
- Identify Functional Units: Break your code into logical sections or functions.
- Test Each Unit: Verify that each section works correctly in isolation.
- Use Binary Search Method: If you have a large codebase, use a binary search approach to isolate the bug. Start by commenting out half of the code and see if the bug persists. Repeat this process, narrowing down the problematic section.
- Check Inputs and Outputs: Verify that the inputs and outputs of each function are correct.
Example:
Suppose you have a function that’s supposed to calculate the factorial of a number, but it’s giving incorrect results:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n - 1)
# Test cases
print(factorial(5)) # Expected: 120, Actual: 120
print(factorial(0)) # Expected: 1, Actual: 1
print(factorial(3)) # Expected: 6, Actual: 6
print(factorial(10)) # Expected: 3628800, Actual: 3628800
To debug this function:
- Test the base cases (n = 0 and n = 1) separately.
- Test the recursive case with small inputs (e.g., n = 2, n = 3) to ensure the recursion works correctly.
- Add print statements to track the recursive calls and intermediate results.
def factorial(n):
print(f"DEBUG: Calculating factorial for n = {n}")
if n == 0 or n == 1:
print(f"DEBUG: Base case reached, returning 1")
return 1
else:
result = n * factorial(n - 1)
print(f"DEBUG: n = {n}, result = {result}")
return result
# Test case
print(factorial(5))
This approach helps you visualize the recursive process and identify any issues in the logic or base cases.
3. Rubber Duck Debugging
Rubber duck debugging is a method of debugging code by explaining it, line-by-line, to an inanimate object (traditionally, a rubber duck). This technique can be particularly useful in interview settings, as it allows you to verbalize your thought process and potentially spot errors you might have missed.
How to Practice Rubber Duck Debugging:
- Explain Your Code: Go through your code line by line, explaining what each part does.
- Be Detailed: Don’t skip any steps, no matter how obvious they may seem.
- Question Your Assumptions: As you explain, question whether each line is doing exactly what you think it should.
- Listen to Yourself: Often, the act of explaining out loud can help you realize where your logic might be flawed.
Example:
Let’s say you’re trying to debug a function that’s supposed to find the second largest element in an array:
def find_second_largest(arr):
if len(arr) < 2:
return None
largest = second_largest = float('-inf')
for num in arr:
if num > largest:
second_largest = largest
largest = num
elif num > second_largest and num != largest:
second_largest = num
return second_largest if second_largest != float('-inf') else None
# Test cases
print(find_second_largest([5, 2, 8, 1, 9])) # Expected: 8
print(find_second_largest([1, 1, 1, 1])) # Expected: None
print(find_second_largest([10])) # Expected: None
Now, let’s go through the rubber duck debugging process:
“Okay, rubber duck, let’s go through this function:
- First, we check if the array has less than 2 elements. If so, we return None because there can’t be a second largest element.
- We initialize two variables, largest and second_largest, to negative infinity. This ensures that any number in the array will be larger than these initial values.
- We iterate through each number in the array:
- If the current number is larger than largest, we update second_largest to be the old largest, and largest to be the current number.
- If the current number is larger than second_largest but not equal to largest, we update second_largest.
- After the loop, we return second_largest, but only if it’s been updated from its initial value. Otherwise, we return None.
Hmm… now that I say it out loud, I realize that this logic doesn’t handle duplicate values correctly. If the largest number appears more than once, we might incorrectly return None. Let’s fix that by removing the ‘and num != largest’ condition.”
After this rubber duck session, you might revise your code to:
def find_second_largest(arr):
if len(arr) < 2:
return None
largest = second_largest = float('-inf')
for num in arr:
if num > largest:
second_largest = largest
largest = num
elif num > second_largest:
second_largest = num
return second_largest if second_largest != float('-inf') else None
This revised version correctly handles cases with duplicate values.
4. Leverage Debugging Tools
While print statements are useful, many programming environments offer more sophisticated debugging tools. Familiarizing yourself with these tools can significantly speed up your debugging process.
Common Debugging Tools and Features:
- Breakpoints: Allow you to pause program execution at specific lines.
- Step-through Execution: Enables you to run your code line by line.
- Variable Inspection: Lets you view and sometimes modify variable values during execution.
- Call Stack Examination: Helps you understand the sequence of function calls that led to the current point in execution.
Example: Using Python’s pdb
Python’s built-in debugger, pdb, can be a powerful tool for debugging during interviews. Here’s how you might use it:
import pdb
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i = j = 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Test the function
arr = [38, 27, 43, 3, 9, 82, 10]
pdb.set_trace() # This will start the debugger
sorted_arr = merge_sort(arr)
print(sorted_arr)
When you run this code, it will pause at the pdb.set_trace()
line, allowing you to step through the merge sort algorithm, inspect variables, and identify any issues.
5. Write and Use Test Cases
Developing a set of comprehensive test cases is crucial for effective debugging. Test cases help you verify that your code works correctly for various inputs and edge cases.
Tips for Writing Effective Test Cases:
- Cover Edge Cases: Include tests for empty inputs, single-element inputs, and other boundary conditions.
- Test Normal Cases: Ensure your code works for typical, expected inputs.
- Include Large Inputs: Test how your code performs with larger datasets to catch performance issues.
- Use Assertion Statements: Implement assertions to automatically check if outputs match expected results.
Example: Testing a Binary Search Function
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Test cases
def test_binary_search():
# Test normal case
assert binary_search([1, 3, 5, 7, 9], 5) == 2, "Failed on normal case"
# Test first element
assert binary_search([1, 3, 5, 7, 9], 1) == 0, "Failed on first element"
# Test last element
assert binary_search([1, 3, 5, 7, 9], 9) == 4, "Failed on last element"
# Test element not in array
assert binary_search([1, 3, 5, 7, 9], 4) == -1, "Failed on element not in array"
# Test empty array
assert binary_search([], 5) == -1, "Failed on empty array"
# Test single-element array
assert binary_search([1], 1) == 0, "Failed on single-element array"
print("All test cases passed!")
test_binary_search()
By running these test cases, you can quickly identify if your binary search implementation has any issues with specific scenarios.
Common Bugs in Coding Interview Problems and How to Fix Them
Now that we’ve covered general debugging strategies, let’s look at some common bugs that often appear in coding interview problems and how to systematically identify and fix them.
1. Off-by-One Errors
Off-by-one errors occur when a loop iterates one time too many or too few. These are particularly common in array manipulation and string processing problems.
Example:
def print_array_elements(arr):
for i in range(len(arr)):
print(arr[i])
# This will cause an IndexError for the last iteration
print_array_elements([1, 2, 3])
How to Fix:
- Double-check loop conditions, especially when using < vs <=.
- Be careful with zero-based indexing.
- Use print statements or debugger to check loop variables at each iteration.
Corrected Version:
def print_array_elements(arr):
for i in range(len(arr)):
print(arr[i])
2. Infinite Loops
Infinite loops occur when the loop condition never becomes false. These can be particularly tricky in recursive functions or while loops.
Example:
def find_element(arr, target):
left, right = 0, len(arr) - 1
while left < right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid
else:
right = mid
return -1
How to Fix:
- Ensure that the loop variable is being updated in each iteration.
- Check that the loop condition will eventually be met.
- Use print statements to track the loop variables and see if they’re changing as expected.
Corrected Version:
def find_element(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3. Stack Overflow in Recursive Functions
Stack overflow errors can occur in recursive functions when the recursion goes too deep, often due to missing or incorrect base cases.
Example:
def factorial(n):
return n * factorial(n - 1)
# This will cause a stack overflow
print(factorial(5))
How to Fix:
- Ensure that your recursive function has a proper base case.
- Check that the recursive calls are moving towards the base case.
- Consider using an iterative approach instead of recursion for very large inputs.
Corrected Version:
def factorial(n):
if n == 0 or n == 1:
return 1
return n * factorial(n - 1)
print(factorial(5)) # Correctly prints 120
4. Unintended Integer Division
In languages like Python 2, division of integers results in integer division, which can lead to unexpected results.
Example:
def average(a, b):
return (a + b) / 2
print(average(3, 4)) # In Python 2, this would print 3 instead of 3.5
How to Fix:
- Cast one of the operands to a float to force float division.
- In Python 3, use
//
for intentional integer division and/
for float division.
Corrected Version:
def average(a, b):
return (a + b) / 2.0 # or use float(a + b) / 2 in Python 2
print(average(3, 4)) # Correctly prints 3.5
5. Mutable Default Arguments
In Python, using mutable objects (like lists or dictionaries) as default arguments can lead to unexpected behavior.
Example:
def append_to_list(item, lst=[]):
lst.append(item)
return lst
print(append_to_list(1)) # [1]
print(append_to_list(2)) # [1, 2] (Unexpected!)
How to Fix:
- Use None as the default argument and create the mutable object inside the function.
- Be aware of this behavior when reviewing or writing functions with default arguments.
Corrected Version:
def append_to_list(item, lst=None):
if lst is None:
lst = []
lst.append(item)
return lst
print(append_to_list(1)) # [1]
print(append_to_list(2)) # [2]
Conclusion
Debugging is an essential skill for any programmer, and it becomes even more critical during coding interviews. By mastering strategies like using print statements effectively, dividing the problem space, rubber duck debugging, leveraging debugging tools, and writing comprehensive test cases, you’ll be well-equipped to tackle bugs quickly and efficiently.
Remember that debugging is not just about fixing errors; it’s about understanding your code deeply and being able to reason about its behavior. This understanding is exactly what interviewers are looking for when they present you with coding challenges.
As you prepare for coding interviews, make debugging an integral part of your practice routine. Don’t just solve problems; intentionally introduce bugs into your solutions and practice finding and fixing them. This will not only improve your debugging skills but also deepen your understanding of the algorithms and data structures you’re working with.
Lastly, stay calm when you encounter bugs during an interview. View them as opportunities to showcase your problem-solving skills rather than as setbacks. With practice and the strategies outlined in this guide, you’ll be well-prepared to handle any debugging challenges that come your way during coding interviews.