Debugging Like Sherlock: Applying Detective Techniques to Code Investigation
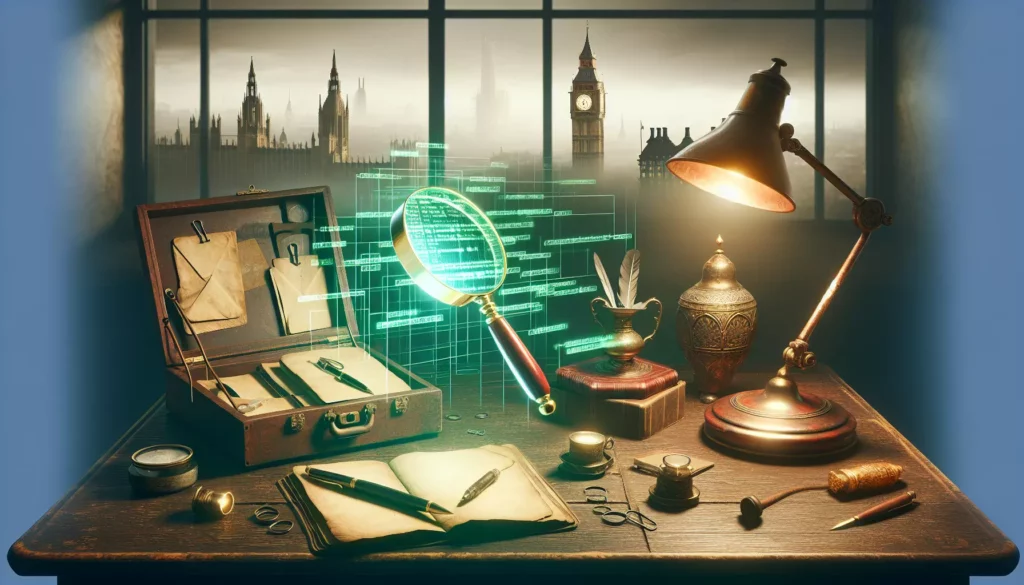
In the world of software development, bugs are the elusive criminals that every programmer must face. Just as Sherlock Holmes meticulously investigates crime scenes, developers must approach debugging with the same level of dedication and analytical prowess. This article will explore how you can channel your inner detective to become a master debugger, using techniques that would make even the great Sherlock Holmes proud.
The Art of Observation: Gathering Clues
Sherlock Holmes was renowned for his keen eye for detail. In debugging, this translates to carefully observing the symptoms of the bug. Before diving into the code, take a step back and gather as much information as possible:
- What is the expected behavior?
- What is the actual behavior?
- Under what conditions does the bug occur?
- Is the bug reproducible?
- Are there any error messages or logs?
By collecting these clues, you create a solid foundation for your investigation. Let’s look at an example of how this might play out in practice:
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
return total / len(numbers)
result = calculate_average([1, 2, 3, 4, 5])
print(f"The average is: {result}")
# Output: The average is: 3.0
result = calculate_average([])
print(f"The average of an empty list is: {result}")
# Output: ZeroDivisionError: division by zero
In this scenario, our function works correctly for non-empty lists but crashes when given an empty list. Our observation tells us that the bug is related to handling empty input.
The Deductive Method: Forming Hypotheses
Once you’ve gathered your clues, it’s time to form hypotheses about what might be causing the bug. Sherlock Holmes was famous for his deductive reasoning, and as a developer, you should cultivate this skill as well. Consider all possible explanations, no matter how unlikely they may seem at first.
For our example, we might form the following hypotheses:
- The function doesn’t check for empty input before dividing.
- There’s an issue with the loop that calculates the total.
- The len() function is not working correctly for empty lists.
By listing out potential causes, we create a roadmap for our investigation. This helps us approach the problem systematically rather than haphazardly poking around in the code.
The Process of Elimination: Testing Hypotheses
Sherlock Holmes often said, “When you have eliminated the impossible, whatever remains, however improbable, must be the truth.” In debugging, we follow a similar process of elimination. We test each hypothesis, ruling out those that don’t fit the evidence.
Let’s test our hypotheses:
- We can quickly confirm that there’s no check for empty input before the division operation.
- The loop for calculating the total seems correct, as it works for non-empty lists.
- We can easily verify that len([]) returns 0, ruling out an issue with the len() function.
Through this process, we’ve identified the most likely cause: the function doesn’t handle empty input properly. Now we can focus on fixing this specific issue.
The Art of Interrogation: Using Debugging Tools
Sherlock Holmes was a master of interrogation, extracting crucial information from witnesses and suspects. As a developer, your debugging tools are your means of interrogating the code. Let’s explore some essential debugging techniques:
1. Print Statements
The humble print statement is often underestimated but can be incredibly powerful. By strategically placing print statements, you can track the flow of your program and the values of variables at different points.
def calculate_average(numbers):
print(f"Input: {numbers}")
total = 0
for num in numbers:
total += num
print(f"Current total: {total}")
result = total / len(numbers)
print(f"Final result: {result}")
return result
calculate_average([1, 2, 3, 4, 5])
calculate_average([])
This enhanced version of our function will provide more insight into what’s happening at each step, making it easier to pinpoint where things go wrong.
2. Debugger
Most modern IDEs come with built-in debuggers that allow you to step through your code line by line, inspect variables, and set breakpoints. This is like having a magnifying glass for your code, allowing you to examine every detail in slow motion.
For example, in Python, you can use the pdb module to set breakpoints and step through your code:
import pdb
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
pdb.set_trace() # This will pause execution and start the debugger
return total / len(numbers)
calculate_average([1, 2, 3, 4, 5])
calculate_average([])
When the debugger pauses at the set_trace() line, you can inspect the values of ‘total’ and ‘numbers’, step to the next line, or continue execution.
3. Logging
For more complex applications, especially those running in production, logging is essential. It’s like leaving a trail of breadcrumbs that you can follow later to understand what happened.
import logging
logging.basicConfig(level=logging.DEBUG)
def calculate_average(numbers):
logging.debug(f"Calculating average for: {numbers}")
total = 0
for num in numbers:
total += num
logging.debug(f"Current total: {total}")
try:
result = total / len(numbers)
logging.debug(f"Calculated average: {result}")
return result
except ZeroDivisionError:
logging.error("Attempted to calculate average of empty list")
return None
calculate_average([1, 2, 3, 4, 5])
calculate_average([])
This version uses logging to record what’s happening at each step, which can be especially useful for diagnosing issues in larger applications.
The Power of Deduction: Analyzing the Evidence
Now that we’ve gathered our evidence through various debugging techniques, it’s time to analyze it and draw conclusions. Sherlock Holmes was famous for his ability to see connections that others missed. In debugging, this means looking at the data you’ve collected and identifying patterns or anomalies.
In our example, the evidence clearly points to a problem with dividing by zero when the input list is empty. Armed with this knowledge, we can now implement a fix:
def calculate_average(numbers):
if not numbers:
return None # or raise an exception, depending on your requirements
total = sum(numbers)
return total / len(numbers)
print(calculate_average([1, 2, 3, 4, 5])) # Output: 3.0
print(calculate_average([])) # Output: None
This improved version handles empty inputs gracefully, returning None instead of raising an exception. We’ve also simplified the function by using the built-in sum() function, which is more efficient for larger lists.
The Importance of the Experiment: Testing Your Solution
Sherlock Holmes often conducted experiments to test his theories. In the world of programming, this translates to thorough testing of your bug fixes. It’s not enough to fix the immediate issue; you need to ensure that your solution doesn’t introduce new problems and works in all scenarios.
Here’s how we might test our improved calculate_average function:
import unittest
class TestCalculateAverage(unittest.TestCase):
def test_normal_list(self):
self.assertEqual(calculate_average([1, 2, 3, 4, 5]), 3.0)
def test_empty_list(self):
self.assertIsNone(calculate_average([]))
def test_single_item_list(self):
self.assertEqual(calculate_average([42]), 42.0)
def test_negative_numbers(self):
self.assertEqual(calculate_average([-1, -2, -3, -4, -5]), -3.0)
def test_float_numbers(self):
self.assertAlmostEqual(calculate_average([1.5, 2.5, 3.5]), 2.5)
if __name__ == '__main__':
unittest.main()
By writing comprehensive tests, we can be confident that our function works correctly in various scenarios, including edge cases.
The Case of the Recurring Bug: Preventing Future Issues
A true detective doesn’t just solve the current case; they also work to prevent future crimes. In the world of debugging, this means implementing practices that will help catch and prevent bugs before they make it into production code.
1. Code Reviews
Just as Sherlock Holmes often collaborated with Dr. Watson, developers should work together through code reviews. A fresh pair of eyes can often spot issues that the original author missed.
2. Static Analysis Tools
Tools like pylint for Python or ESLint for JavaScript can automatically detect potential issues in your code. It’s like having a junior detective constantly scanning your codebase for suspicious activity.
3. Continuous Integration and Testing
Implementing a CI/CD pipeline that runs your test suite automatically on every commit can catch bugs early in the development process.
4. Error Monitoring
For applications in production, using error monitoring tools can alert you to issues as soon as they occur, allowing you to investigate and fix problems quickly.
The Elementary Principles of Debugging
As we conclude our journey through the world of debugging, let’s summarize the key principles that every code detective should keep in mind:
- Observe Carefully: Gather all available information about the bug before starting your investigation.
- Form Hypotheses: Use deductive reasoning to come up with potential explanations for the bug.
- Test Systematically: Eliminate impossible explanations and focus on the most likely causes.
- Use Your Tools: Leverage debugging tools and techniques to interrogate your code effectively.
- Analyze Thoroughly: Look for patterns and connections in the evidence you’ve gathered.
- Test Your Solutions: Ensure your fix works in all scenarios and doesn’t introduce new problems.
- Prevent Future Issues: Implement practices that will help catch and prevent bugs in the future.
By applying these principles, you can approach debugging with the same methodical precision that Sherlock Holmes applied to his cases. Remember, in the words of the great detective himself, “The game is afoot!” Happy debugging!
Conclusion: The Never-Ending Mystery
Debugging, like detective work, is an ongoing process. As software systems grow more complex, new types of bugs will emerge, presenting fresh challenges for developers to solve. But by approaching these challenges with a detective’s mindset – observant, analytical, and persistent – you’ll be well-equipped to tackle even the most perplexing coding conundrums.
Remember that every bug you encounter is an opportunity to learn and improve your skills. Each debugging session makes you a better developer, honing your problem-solving abilities and deepening your understanding of the systems you work with.
So the next time you’re faced with a particularly tricky bug, don your deerstalker cap, grab your magnifying glass (or debugger), and dive into the code with the enthusiasm of Sherlock Holmes embarking on a new case. The thrill of the chase and the satisfaction of solving the mystery await you!
And always remember: “When you have eliminated the impossible, whatever remains, however improbable, must be the truth.” Happy sleuthing, code detectives!