Debugging by Interpretive Dance: Expressing Code Flow Through Movement
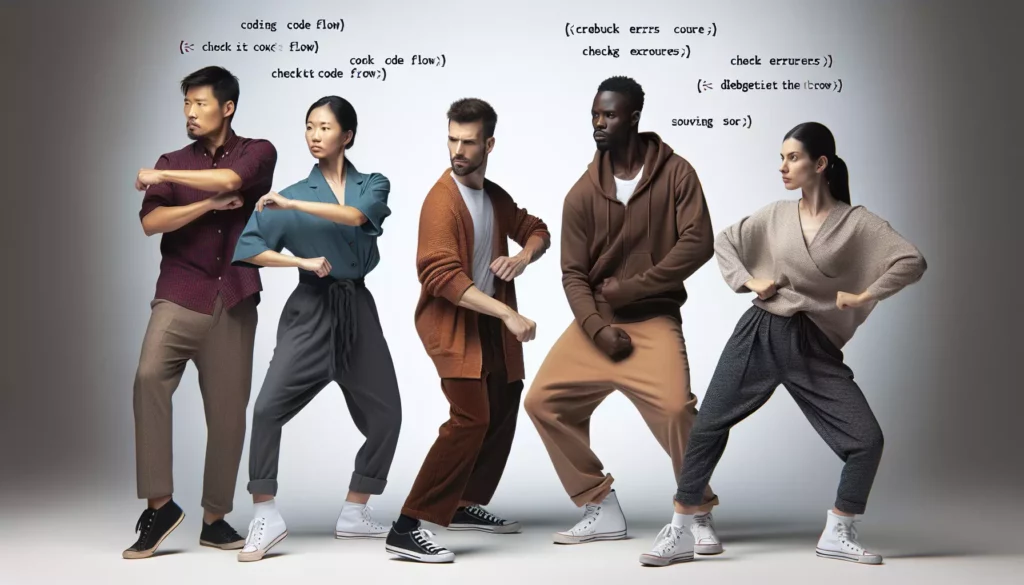
In the world of programming, debugging is often seen as a tedious and frustrating process. But what if we could transform this essential task into something more engaging, creative, and even fun? Enter the concept of “Debugging by Interpretive Dance” – a unique approach that combines the logical world of coding with the expressive art of movement. This innovative method not only offers a fresh perspective on understanding code flow but also serves as a powerful educational tool for both novice and experienced programmers alike.
The Intersection of Code and Choreography
At first glance, programming and dance might seem like polar opposites. One is rooted in logic and structure, while the other is often associated with creativity and emotion. However, both share fundamental similarities that make their combination not only possible but potentially revolutionary in the realm of coding education.
Similarities Between Code and Dance:
- Sequence and Flow: Both code and choreography rely on a specific sequence of steps or instructions.
- Rhythm and Timing: Just as dancers must maintain rhythm, code execution follows a precise timing.
- Structure and Patterns: Programming patterns find parallels in dance formations and routines.
- Expression and Communication: Both mediums aim to convey ideas or solve problems.
By leveraging these similarities, we can create a unique learning experience that engages multiple senses and learning styles, potentially improving comprehension and retention of complex programming concepts.
The Basics of Debugging by Interpretive Dance
Debugging by interpretive dance involves translating code execution and flow into physical movements. This method can be particularly useful for visualizing and understanding the step-by-step process of how a program runs, especially when trying to identify and fix errors.
Key Elements of the Dance-Debug Approach:
- Mapping Code Constructs to Movements
- Representing Variables and Data Types
- Illustrating Control Flow
- Expressing Function Calls and Returns
- Demonstrating Error States
1. Mapping Code Constructs to Movements
To begin, we need to establish a “movement vocabulary” that corresponds to different programming constructs. For example:
- Variable Declaration: A sweeping arm motion, as if clearing a space
- Assignment: A grabbing motion, followed by placing the “value” in the cleared space
- Conditional Statements: A fork in the path, with the dancer choosing a direction based on the condition
- Loops: Repetitive circular movements, with variations for different loop types
2. Representing Variables and Data Types
Different data types can be represented through distinct gestures or props:
- Integers: Holding up a number of fingers
- Strings: Miming the act of writing or using ribbon props
- Booleans: Thumbs up for true, thumbs down for false
- Arrays: A line of dancers, each representing an element
3. Illustrating Control Flow
Control flow can be demonstrated through the dancer’s path and direction:
- Sequential Execution: Moving in a straight line
- Branching: Taking different paths based on conditions
- Looping: Returning to a previous position and repeating movements
4. Expressing Function Calls and Returns
Function calls and returns can be choreographed as follows:
- Function Call: A leap or jump to a new area of the stage
- Parameter Passing: Handing off props or gestures to another dancer
- Return Value: Bringing back a prop or gesture to the original position
5. Demonstrating Error States
Errors and exceptions can be dramatically portrayed:
- Syntax Error: A stumble or misstep in the choreography
- Runtime Error: An abrupt stop or fall
- Infinite Loop: Continuous spinning until “manually” stopped
Practical Application: Dancing Through a Simple Algorithm
Let’s explore how we might dance through a simple bubble sort algorithm. Here’s the Python code for a bubble sort:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
unsorted_array = [64, 34, 25, 12, 22, 11, 90]
sorted_array = bubble_sort(unsorted_array)
print(sorted_array)
Now, let’s break down how this could be interpreted through dance:
- Setup: Dancers line up, each representing an element in the array. They hold numbered cards to show their values.
- Outer Loop: A lead dancer moves along the line, representing the ‘i’ variable.
- Inner Loop: For each position of the lead dancer, the line of “element dancers” perform the comparison and swap:
- Comparison: Adjacent dancers face each other and display their numbers.
- Swap: If the left number is greater, the dancers exchange positions with an exaggerated crossing motion.
- Repeat: The lead dancer continues down the line, with fewer comparisons needed each pass (represented by fewer dancers participating).
- Completion: The dance ends when the lead dancer completes a pass without any swaps occurring.
This dance representation allows viewers to visually track the progress of the sorting algorithm, making the concept more tangible and easier to grasp.
Benefits of Debugging by Interpretive Dance
Incorporating dance into the debugging process offers several advantages:
1. Enhanced Visualization
By physically acting out the code execution, programmers can gain a clearer picture of how their algorithms work. This visual and kinesthetic approach can reveal insights that might be missed when simply reading code on a screen.
2. Improved Memory Retention
The act of physically performing the code flow can enhance memory retention. This multi-sensory learning experience engages more areas of the brain, potentially leading to better recall of programming concepts and patterns.
3. Collaborative Debugging
Debugging by interpretive dance can be a group activity, fostering collaboration and communication among team members. It allows programmers to literally “walk through” the code together, promoting shared understanding and problem-solving.
4. Stress Relief
Debugging can be a frustrating process. Introducing an element of physical activity and creativity can help alleviate stress and make the debugging process more enjoyable.
5. Accessible Learning Tool
This method can be particularly beneficial for kinesthetic learners who struggle with traditional, text-based learning approaches. It provides an alternative way to understand and internalize programming concepts.
Challenges and Considerations
While debugging by interpretive dance offers numerous benefits, it’s important to acknowledge potential challenges:
1. Complexity Limitations
As programs become more complex, translating them into dance movements may become increasingly difficult or impractical. This method might be most effective for fundamental concepts or smaller code segments.
2. Physical Space Requirements
Performing debug dances requires adequate space, which may not always be available in typical office or learning environments.
3. Learning Curve
Both instructors and learners need to become familiar with the movement vocabulary and how it maps to code constructs. This initial learning phase might require some time and patience.
4. Potential for Misinterpretation
There’s a risk that the dance interpretation might oversimplify or misrepresent certain coding concepts. Care must be taken to ensure accuracy in the translation from code to movement.
Integrating Dance-Debugging into Coding Education
To effectively incorporate debugging by interpretive dance into coding education, consider the following strategies:
1. Start with Basic Concepts
Begin by applying the dance-debug method to fundamental programming concepts like variables, loops, and conditional statements. This lays a strong foundation for more complex interpretations later.
2. Create a Standard Movement Vocabulary
Develop and document a standardized set of movements that correspond to common programming constructs. This ensures consistency and helps learners build familiarity with the method.
3. Incorporate Video Tutorials
Create video demonstrations of dance-debug interpretations for various algorithms and coding patterns. These can serve as reference materials for students and complement traditional learning resources.
4. Encourage Student Choreography
As students become more comfortable with the concept, encourage them to create their own dance interpretations of code. This creative process can deepen their understanding and engagement with the material.
5. Use as a Complementary Tool
Position dance-debugging as a complementary method rather than a replacement for traditional debugging techniques. It should enhance, not substitute, existing learning approaches.
Real-World Applications and Case Studies
While debugging by interpretive dance might seem unconventional, similar kinesthetic learning approaches have shown promise in various educational settings:
Case Study 1: Computer Science Unplugged
The “Computer Science Unplugged” project, developed by Tim Bell and colleagues, uses physical activities to teach computer science concepts without computers. Their approach, which includes elements of movement and dance, has been successfully implemented in schools worldwide.
Case Study 2: Algorithm Visualization in Education
Research by Schweitzer and Brown (2007) on algorithm visualization in education found that active engagement methods, including kinesthetic learning, led to better understanding and retention of algorithmic concepts among students.
Case Study 3: Dance Your PhD
While not directly related to coding, the annual “Dance Your PhD” contest challenges doctoral students to explain their research through dance. This initiative demonstrates the potential of using movement to convey complex, technical concepts.
Future Possibilities and Research Directions
The concept of debugging by interpretive dance opens up exciting possibilities for future development and research:
1. VR and AR Integration
Virtual and Augmented Reality technologies could be used to create immersive environments where learners can interact with code through movement, blending the physical and digital realms of debugging.
2. AI-Powered Movement Analysis
Machine learning algorithms could be developed to analyze and provide feedback on dance-debug performances, helping learners refine their understanding of code flow.
3. Cross-Disciplinary Studies
Research collaborations between computer science educators and dance or movement therapists could yield insights into the most effective ways to represent code through motion.
4. Accessibility and Inclusion
Exploring how dance-debugging can be adapted for learners with different physical abilities could lead to more inclusive coding education practices.
Conclusion: Embracing Creativity in Coding Education
Debugging by interpretive dance represents a bold step towards more creative, engaging, and holistic approaches to coding education. By bridging the gap between the logical world of programming and the expressive realm of movement, we open new pathways for learning and understanding complex computational concepts.
While it may not replace traditional debugging methods, this innovative approach offers a valuable complement to existing techniques. It encourages learners to think about code in new ways, fosters collaboration, and makes the often daunting task of debugging more approachable and even enjoyable.
As we continue to explore and refine this method, we may discover that the dance of debugging not only enhances our understanding of code but also cultivates a deeper appreciation for the artistry inherent in programming. In embracing this creative approach, we remind ourselves that at its core, coding is not just about writing instructions for machines – it’s about expressing ideas, solving problems, and bringing imagination to life.
So, the next time you find yourself stuck on a particularly tricky bug, why not step away from the keyboard, clear some space, and let your code dance? You might just find that the solution was waiting for you in the rhythm of your own movements all along.