Data Structures: The Building Blocks of Efficient Programming
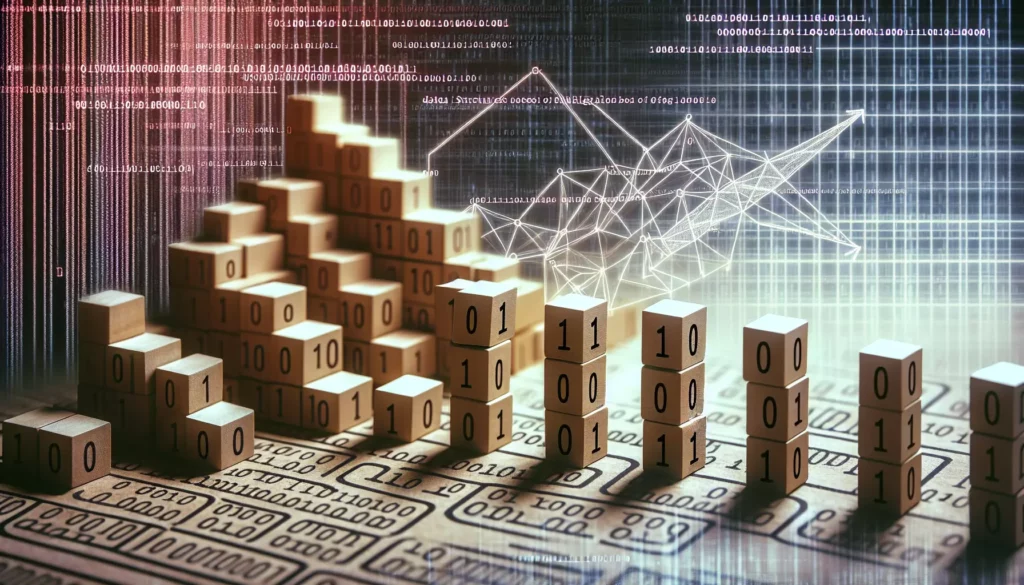
In the world of programming, data structures are as fundamental as the alphabet is to language. They form the backbone of efficient code and are crucial for solving complex computational problems. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, understanding data structures is essential. In this comprehensive guide, we’ll explore what data structures are, why they’re important, and how they’re used in real-world applications.
What Are Data Structures?
At its core, a data structure is a particular way of organizing data in a computer so that it can be used effectively. Think of data structures as containers that store and organize data, each with its own set of rules for adding, accessing, and removing information. Just as you might organize your closet differently depending on the types of clothes you have and how often you need to access them, programmers choose different data structures based on the specific needs of their algorithms and applications.
Key Characteristics of Data Structures
- Organization: How data is arranged and related to other pieces of data.
- Operations: The set of actions that can be performed on the data, such as insertion, deletion, and searching.
- Performance: How efficiently these operations can be carried out in terms of time and space complexity.
Why Are Data Structures Important in Programming?
The importance of data structures in programming cannot be overstated. They are critical for several reasons:
1. Efficiency and Performance
Choosing the right data structure can dramatically improve the efficiency of your algorithms. For example, searching for an element in a sorted array using binary search is much faster than linear search in an unsorted array. The difference in performance can be the distinction between an application that runs smoothly and one that lags or crashes under heavy loads.
2. Code Organization and Clarity
Data structures provide a clear and organized way to manage data. This organization leads to cleaner, more readable code, which is easier to maintain and debug. When you use appropriate data structures, your code’s intent becomes more apparent, making it easier for other developers (including your future self) to understand and work with.
3. Problem-Solving
Many complex problems become more manageable when you apply the right data structure. For instance, graph data structures are excellent for solving network-related problems, while hash tables are ideal for quick lookups and caching mechanisms.
4. Memory Management
Efficient data structures help in better memory utilization. By choosing the appropriate structure, you can minimize memory waste and optimize storage, which is crucial in environments with limited resources, such as embedded systems or mobile devices.
5. Abstraction and Modularity
Data structures provide a level of abstraction that allows programmers to focus on the high-level functionality of their code rather than low-level implementation details. This abstraction promotes modularity, making it easier to develop and maintain large-scale software systems.
Common Types of Data Structures
Let’s explore some of the most commonly used data structures in programming:
1. Arrays
Arrays are the simplest and most widely used data structure. They store elements of the same type in contiguous memory locations, allowing for constant-time access to any element using its index.
// Example of an array in Java
int[] numbers = {1, 2, 3, 4, 5};
System.out.println(numbers[2]); // Outputs: 3
2. Linked Lists
Linked lists consist of nodes, where each node contains data and a reference (or link) to the next node in the sequence. They are excellent for frequent insertions and deletions, especially at the beginning of the list.
// Example of a linked list node in Python
class Node:
def __init__(self, data):
self.data = data
self.next = None
# Creating a simple linked list
head = Node(1)
head.next = Node(2)
head.next.next = Node(3)
3. Stacks
Stacks follow the Last-In-First-Out (LIFO) principle. They are used in scenarios where you need to reverse the order of elements or keep track of function calls (call stack).
// Example of a stack in C++
#include <stack>
std::stack<int> myStack;
myStack.push(1);
myStack.push(2);
myStack.push(3);
std::cout << myStack.top(); // Outputs: 3
4. Queues
Queues follow the First-In-First-Out (FIFO) principle. They are useful in scenarios like task scheduling or managing requests in a system.
// Example of a queue in JavaScript
let queue = [];
queue.push(1); // Enqueue
queue.push(2);
queue.push(3);
console.log(queue.shift()); // Dequeue, outputs: 1
5. Trees
Trees are hierarchical structures consisting of nodes with a parent-child relationship. They are used in various applications, including file systems, HTML DOM, and expression parsing.
// Example of a binary tree node in Java
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int x) { val = x; }
}
6. Graphs
Graphs consist of vertices (nodes) and edges connecting these vertices. They are used to represent networks, relationships, and complex systems.
// Example of an adjacency list representation of a graph in Python
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
7. Hash Tables
Hash tables provide fast insertion, deletion, and lookup operations. They are implemented using an array and a hash function, which maps keys to array indices.
// Example of a hash table (dictionary) in Python
my_dict = {'apple': 1, 'banana': 2, 'orange': 3}
print(my_dict['banana']) # Outputs: 2
Choosing the Right Data Structure
Selecting the appropriate data structure for a given problem is a crucial skill for any programmer. Here are some factors to consider:
- Nature of the problem: What operations will be performed most frequently?
- Time complexity: How fast do these operations need to be?
- Space complexity: How much memory is available?
- Ease of implementation: Is the added complexity worth the performance gain?
For example, if you need fast lookups and don’t care about the order of elements, a hash table might be ideal. If you need to maintain a sorted list with frequent insertions and deletions, a balanced binary search tree could be more appropriate.
Data Structures in Technical Interviews
Understanding data structures is crucial for technical interviews, especially at top tech companies. Interviewers often ask questions that require you to:
- Identify the most suitable data structure for a given problem.
- Implement a data structure from scratch.
- Analyze the time and space complexity of operations on different data structures.
- Optimize a solution by changing the underlying data structure.
For instance, a common interview question might ask you to implement a least recently used (LRU) cache, which requires a combination of a hash table for fast lookups and a doubly linked list for quick removals and additions.
Real-World Applications of Data Structures
Data structures are not just theoretical concepts; they have numerous practical applications in software development:
1. Social Networks
Graph data structures are used to represent connections between users, enabling features like friend suggestions and degrees of separation.
2. File Systems
Tree structures are used to organize files and directories in operating systems.
3. Databases
B-trees and hash indexes are used to optimize database queries and storage.
4. Compilers and Interpreters
Abstract Syntax Trees (ASTs) represent the structure of program code during compilation or interpretation.
5. Gaming
Quadtrees and octrees are used for spatial partitioning in game development, improving collision detection and rendering efficiency.
6. Machine Learning
Decision trees and random forests are data structures used in various machine learning algorithms.
Advanced Data Structures
As you progress in your programming journey, you’ll encounter more sophisticated data structures that solve specific problems efficiently:
1. Trie
A tree-like structure used for efficient retrieval of keys in a dataset of strings. It’s particularly useful in applications like autocomplete and spell checkers.
2. Segment Tree
A tree data structure used for storing information about intervals or segments. It allows for efficient query operations on arrays.
3. Bloom Filter
A space-efficient probabilistic data structure used to test whether an element is a member of a set. It’s commonly used in caching systems and databases.
4. Skip List
A probabilistic data structure that allows for fast search within an ordered sequence of elements. It’s an alternative to balanced trees with simpler implementation.
Conclusion
Data structures are the foundational building blocks of efficient algorithms and well-designed software systems. They provide the means to organize, store, and retrieve data in ways that can dramatically impact the performance and capabilities of your programs. As you continue to develop your programming skills, investing time in understanding and mastering various data structures will pay dividends in your ability to solve complex problems and write efficient code.
Remember, the journey to mastering data structures is ongoing. Each problem you encounter is an opportunity to apply your knowledge and further refine your skills. Whether you’re preparing for technical interviews at top tech companies or building the next revolutionary application, a solid grasp of data structures will be your key to success in the world of programming.
Keep practicing, experimenting with different data structures, and analyzing their performance in various scenarios. As you do, you’ll develop an intuition for choosing the right structure for each unique problem you face. Happy coding!