Data Structures 101: Understanding Arrays, HashMaps, and More
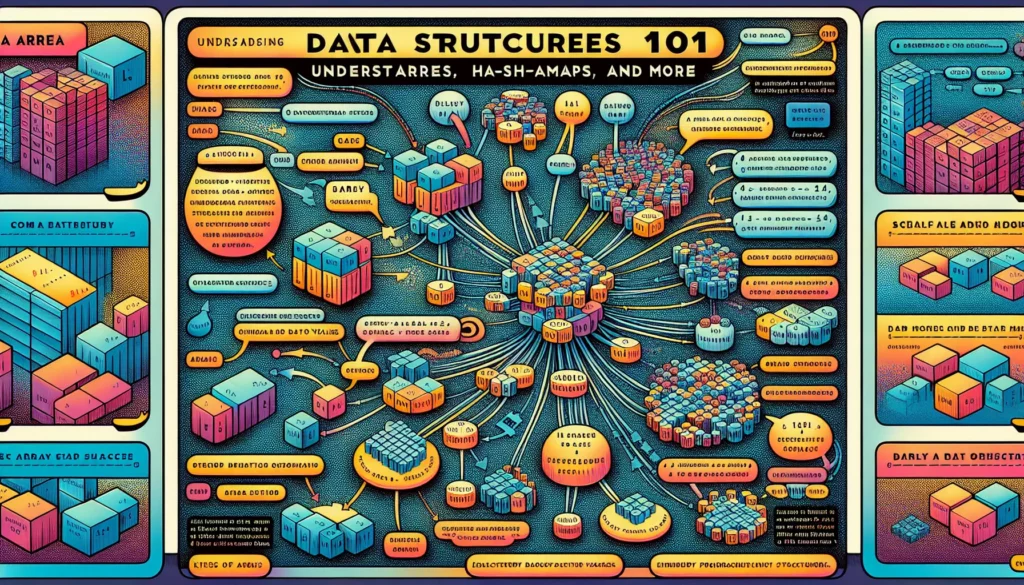
In the world of programming, data structures are fundamental building blocks that allow us to organize and manipulate data efficiently. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, having a solid grasp of data structures is crucial. In this comprehensive guide, we’ll dive deep into some of the most important data structures, including arrays, HashMaps, and more. By the end of this article, you’ll have a better understanding of how these structures work and when to use them in your coding projects.
1. Arrays: The Foundation of Data Structures
Arrays are one of the most basic and widely used data structures in programming. They provide a simple way to store and access multiple elements of the same data type in contiguous memory locations.
Key Characteristics of Arrays:
- Fixed size (in most programming languages)
- Elements are stored in contiguous memory locations
- Random access (constant time complexity for element retrieval)
- Homogeneous data (all elements must be of the same data type)
Types of Arrays:
- One-dimensional arrays (vectors)
- Multi-dimensional arrays (matrices)
Common Operations and Time Complexities:
- Access: O(1)
- Search: O(n) for unsorted arrays, O(log n) for sorted arrays (using binary search)
- Insertion: O(n) (worst case, when inserting at the beginning)
- Deletion: O(n) (worst case, when deleting from the beginning)
Example of Array Declaration and Usage in Java:
// Declaring and initializing an array
int[] numbers = new int[5];
// Assigning values to array elements
numbers[0] = 10;
numbers[1] = 20;
numbers[2] = 30;
numbers[3] = 40;
numbers[4] = 50;
// Accessing array elements
System.out.println("Third element: " + numbers[2]);
// Iterating through an array
for (int i = 0; i < numbers.length; i++) {
System.out.println("Element at index " + i + ": " + numbers[i]);
}
When to Use Arrays:
- When you need to store a fixed number of elements of the same data type
- When you require fast access to elements by their index
- For implementing other data structures like stacks, queues, and matrices
- In algorithms that require sequential data processing
2. HashMaps: Efficient Key-Value Pair Storage
HashMaps, also known as hash tables or dictionaries in some programming languages, are powerful data structures that allow you to store key-value pairs. They provide fast access, insertion, and deletion operations, making them ideal for many applications.
Key Characteristics of HashMaps:
- Store data as key-value pairs
- Keys must be unique
- Fast access, insertion, and deletion (average case)
- No particular order of elements
How HashMaps Work:
- A hash function is used to compute an index from the key
- The key-value pair is stored at the computed index in an array
- Collision resolution techniques (e.g., chaining or open addressing) are used to handle multiple keys hashing to the same index
Common Operations and Time Complexities:
- Access: O(1) average case, O(n) worst case
- Insertion: O(1) average case, O(n) worst case
- Deletion: O(1) average case, O(n) worst case
- Search: O(1) average case, O(n) worst case
Example of HashMap Usage in Java:
import java.util.HashMap;
// Creating a HashMap
HashMap<String, Integer> ageMap = new HashMap<>();
// Adding key-value pairs
ageMap.put("Alice", 25);
ageMap.put("Bob", 30);
ageMap.put("Charlie", 35);
// Accessing values
System.out.println("Bob's age: " + ageMap.get("Bob"));
// Updating values
ageMap.put("Alice", 26);
// Removing key-value pairs
ageMap.remove("Charlie");
// Checking if a key exists
if (ageMap.containsKey("David")) {
System.out.println("David's age is in the map");
} else {
System.out.println("David's age is not in the map");
}
// Iterating through a HashMap
for (String name : ageMap.keySet()) {
System.out.println(name + " is " + ageMap.get(name) + " years old");
}
When to Use HashMaps:
- When you need to store and retrieve data based on unique keys
- For implementing caches or memoization in algorithms
- When you require fast lookups, insertions, and deletions
- For counting occurrences of elements in a dataset
3. Linked Lists: Dynamic and Flexible Data Storage
Linked lists are linear data structures where elements are stored in nodes, and each node points to the next node in the sequence. Unlike arrays, linked lists do not require contiguous memory allocation, making them more flexible for dynamic data storage.
Key Characteristics of Linked Lists:
- Dynamic size (can grow or shrink easily)
- Non-contiguous memory allocation
- Efficient insertion and deletion at any position
- Sequential access (no random access)
Types of Linked Lists:
- Singly Linked List: Each node has data and a reference to the next node
- Doubly Linked List: Each node has data, a reference to the next node, and a reference to the previous node
- Circular Linked List: The last node points back to the first node, forming a circle
Common Operations and Time Complexities:
- Access: O(n)
- Search: O(n)
- Insertion at beginning: O(1)
- Insertion at end: O(n) for singly linked list, O(1) for doubly linked list with tail pointer
- Deletion: O(1) if the node is given, O(n) if we need to search for the node
Example of Singly Linked List Implementation in Java:
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
class SinglyLinkedList {
Node head;
public void insert(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void display() {
Node current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
}
// Usage
SinglyLinkedList list = new SinglyLinkedList();
list.insert(10);
list.insert(20);
list.insert(30);
list.display(); // Output: 10 -> 20 -> 30 -> null
When to Use Linked Lists:
- When you need frequent insertions and deletions at any position in the list
- When you don’t know the size of the data structure in advance
- For implementing other data structures like stacks, queues, and graphs
- When memory usage needs to be efficient (no need for contiguous memory)
4. Stacks: Last-In-First-Out (LIFO) Data Structure
Stacks are abstract data types that follow the Last-In-First-Out (LIFO) principle. They are commonly used in various algorithms and applications, including function call management, expression evaluation, and backtracking algorithms.
Key Characteristics of Stacks:
- LIFO order: The last element added is the first one to be removed
- Restricted access: Elements can only be added or removed from the top of the stack
- Dynamic size (can grow or shrink as needed)
Common Operations and Time Complexities:
- Push (add an element): O(1)
- Pop (remove the top element): O(1)
- Peek (view the top element without removing it): O(1)
- isEmpty (check if the stack is empty): O(1)
Example of Stack Implementation in Java:
import java.util.ArrayList;
class Stack<T> {
private ArrayList<T> stack;
public Stack() {
stack = new ArrayList<>();
}
public void push(T item) {
stack.add(item);
}
public T pop() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
return stack.remove(stack.size() - 1);
}
public T peek() {
if (isEmpty()) {
throw new IllegalStateException("Stack is empty");
}
return stack.get(stack.size() - 1);
}
public boolean isEmpty() {
return stack.isEmpty();
}
public int size() {
return stack.size();
}
}
// Usage
Stack<Integer> stack = new Stack<>();
stack.push(10);
stack.push(20);
stack.push(30);
System.out.println("Top element: " + stack.peek()); // Output: 30
System.out.println("Popped element: " + stack.pop()); // Output: 30
System.out.println("Stack size: " + stack.size()); // Output: 2
When to Use Stacks:
- For implementing undo/redo functionality in applications
- In depth-first search algorithms for graph traversal
- For parsing and evaluating arithmetic expressions
- In backtracking algorithms
5. Queues: First-In-First-Out (FIFO) Data Structure
Queues are abstract data types that follow the First-In-First-Out (FIFO) principle. They are commonly used in scenarios where tasks or data need to be processed in the order they arrive, such as in job scheduling systems or breadth-first search algorithms.
Key Characteristics of Queues:
- FIFO order: The first element added is the first one to be removed
- Two ends: Elements are added at the rear (enqueue) and removed from the front (dequeue)
- Dynamic size (can grow or shrink as needed)
Common Operations and Time Complexities:
- Enqueue (add an element): O(1)
- Dequeue (remove the front element): O(1)
- Peek (view the front element without removing it): O(1)
- isEmpty (check if the queue is empty): O(1)
Example of Queue Implementation in Java:
import java.util.LinkedList;
class Queue<T> {
private LinkedList<T> queue;
public Queue() {
queue = new LinkedList<>();
}
public void enqueue(T item) {
queue.addLast(item);
}
public T dequeue() {
if (isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
return queue.removeFirst();
}
public T peek() {
if (isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
return queue.getFirst();
}
public boolean isEmpty() {
return queue.isEmpty();
}
public int size() {
return queue.size();
}
}
// Usage
Queue<String> queue = new Queue<>();
queue.enqueue("Alice");
queue.enqueue("Bob");
queue.enqueue("Charlie");
System.out.println("Front element: " + queue.peek()); // Output: Alice
System.out.println("Dequeued element: " + queue.dequeue()); // Output: Alice
System.out.println("Queue size: " + queue.size()); // Output: 2
When to Use Queues:
- In breadth-first search algorithms for graph traversal
- For implementing job scheduling systems
- In print spooling systems
- For handling asynchronous data transfer (buffering)
6. Trees: Hierarchical Data Structures
Trees are non-linear data structures that represent hierarchical relationships between elements. They consist of nodes connected by edges, with a special node called the root at the top of the hierarchy. Trees are widely used in various applications, including file systems, organization charts, and search algorithms.
Key Characteristics of Trees:
- Hierarchical structure with a root node
- Each node can have zero or more child nodes
- Nodes with no children are called leaf nodes
- Subtrees: Any node and its descendants form a subtree
Types of Trees:
- Binary Trees: Each node has at most two children
- Binary Search Trees (BST): A binary tree with ordered nodes
- AVL Trees: Self-balancing binary search trees
- Red-Black Trees: Another type of self-balancing binary search tree
- B-Trees: Multiway search trees used in databases and file systems
Example of Binary Search Tree Implementation in Java:
class Node {
int data;
Node left, right;
public Node(int data) {
this.data = data;
left = right = null;
}
}
class BinarySearchTree {
Node root;
public BinarySearchTree() {
root = null;
}
public void insert(int data) {
root = insertRec(root, data);
}
private Node insertRec(Node root, int data) {
if (root == null) {
root = new Node(data);
return root;
}
if (data < root.data) {
root.left = insertRec(root.left, data);
} else if (data > root.data) {
root.right = insertRec(root.right, data);
}
return root;
}
public void inorderTraversal() {
inorderTraversalRec(root);
}
private void inorderTraversalRec(Node root) {
if (root != null) {
inorderTraversalRec(root.left);
System.out.print(root.data + " ");
inorderTraversalRec(root.right);
}
}
}
// Usage
BinarySearchTree bst = new BinarySearchTree();
bst.insert(50);
bst.insert(30);
bst.insert(70);
bst.insert(20);
bst.insert(40);
bst.insert(60);
bst.insert(80);
System.out.println("Inorder traversal of the BST:");
bst.inorderTraversal(); // Output: 20 30 40 50 60 70 80
When to Use Trees:
- For representing hierarchical data (e.g., file systems, organization charts)
- In search algorithms (e.g., binary search trees for efficient searching)
- For implementing expression parsers and evaluators
- In network routing algorithms
Conclusion
Understanding data structures is crucial for any programmer, especially those preparing for technical interviews at major tech companies. Arrays, HashMaps, linked lists, stacks, queues, and trees are fundamental building blocks that allow us to organize and manipulate data efficiently in various scenarios.
By mastering these data structures, you’ll be better equipped to solve complex programming problems, optimize algorithms, and design efficient software systems. As you continue your journey in coding education and skill development, make sure to practice implementing and using these data structures in different programming challenges and projects.
Remember that choosing the right data structure for a given problem is often the key to developing efficient and elegant solutions. As you gain more experience, you’ll develop an intuition for when to use each data structure based on the specific requirements of your programming tasks.
Keep practicing, experimenting, and exploring more advanced data structures to further enhance your programming skills and prepare yourself for success in technical interviews and real-world software development challenges.