Cyber-Physical Systems: Bridging the Gap Between Computation and Physical Processes
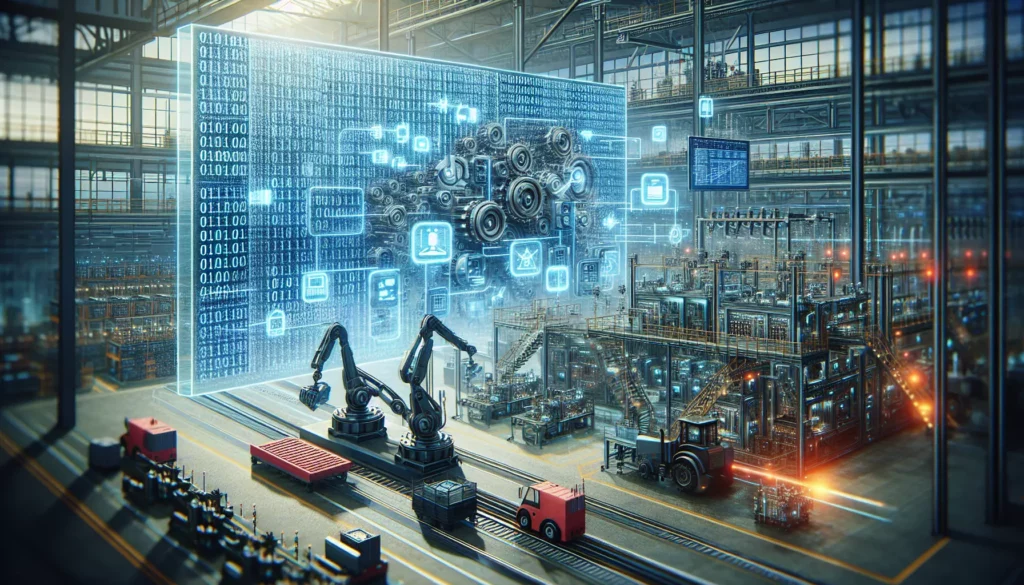
In today’s rapidly evolving technological landscape, the integration of computation with physical processes has become increasingly important. This fusion has given rise to a field known as Cyber-Physical Systems (CPS). As we delve into this fascinating area, we’ll explore its significance, applications, challenges, and how it relates to the broader context of coding education and programming skills development.
What are Cyber-Physical Systems?
Cyber-Physical Systems represent a new generation of systems that tightly integrate computational elements with physical processes. Unlike traditional embedded systems, CPS are designed to interact more deeply with the physical world, often through feedback loops where physical processes affect computations and vice versa.
Key characteristics of Cyber-Physical Systems include:
- Integration of computation, networking, and physical processes
- Real-time operation and feedback
- Distributed and often autonomous control
- High reliability and safety requirements
- Scalability and adaptability
Applications of Cyber-Physical Systems
The applications of CPS are vast and diverse, spanning multiple industries and sectors. Some prominent examples include:
1. Smart Manufacturing
In Industry 4.0, CPS play a crucial role in creating smart factories. They enable real-time monitoring of production processes, predictive maintenance, and adaptive manufacturing systems that can respond to changes in demand or supply chain disruptions.
2. Autonomous Vehicles
Self-driving cars are a prime example of CPS in action. They integrate sensors, control systems, and decision-making algorithms to navigate complex environments safely and efficiently.
3. Smart Grids
In the energy sector, CPS enable the creation of intelligent power grids that can balance supply and demand, integrate renewable energy sources, and respond to outages or fluctuations in real-time.
4. Healthcare Systems
CPS in healthcare include remote patient monitoring systems, smart medical devices, and robotic surgery systems that combine sensing, computation, and physical actuation.
5. Smart Cities
Urban infrastructure is being transformed by CPS, from traffic management systems to smart buildings that optimize energy usage and environmental conditions.
Challenges in Developing Cyber-Physical Systems
While the potential of CPS is immense, their development comes with significant challenges:
1. Complexity
CPS often involve intricate interactions between software, hardware, and physical processes, making them inherently complex to design and validate.
2. Real-Time Constraints
Many CPS applications require strict real-time performance, which can be challenging to achieve consistently, especially in distributed systems.
3. Safety and Security
As CPS often control critical infrastructure or operate in safety-critical environments, ensuring their security and reliability is paramount but challenging.
4. Interoperability
CPS often need to interact with legacy systems and diverse technologies, requiring careful consideration of standards and protocols.
5. Scalability
Designing CPS that can scale from small, localized applications to large, distributed systems presents significant engineering challenges.
The Role of Programming Skills in CPS Development
Developing effective Cyber-Physical Systems requires a broad range of programming skills and knowledge. This is where platforms like AlgoCademy can play a crucial role in preparing the next generation of CPS engineers and developers.
1. Algorithmic Thinking
CPS often require sophisticated algorithms for control, optimization, and decision-making. Strong algorithmic thinking skills are essential for designing efficient and effective CPS. AlgoCademy’s focus on algorithmic problem-solving provides a solid foundation for this aspect of CPS development.
2. Real-Time Programming
Many CPS operate under strict real-time constraints. Understanding real-time programming concepts, such as task scheduling, priority inversion, and deadlock prevention, is crucial. While AlgoCademy may not directly cover these topics, the problem-solving skills it fosters are transferable to learning real-time programming concepts.
3. Distributed Systems
CPS often involve distributed computation and control. Knowledge of distributed algorithms, communication protocols, and synchronization techniques is vital. AlgoCademy’s coverage of data structures and algorithms provides a good starting point for understanding these more advanced concepts.
4. Embedded Systems Programming
Many CPS components run on embedded hardware with limited resources. Proficiency in low-level programming languages like C and assembly, as well as understanding hardware-software interactions, is often necessary. While AlgoCademy may focus more on high-level languages, the fundamental programming concepts it teaches are applicable across different languages and paradigms.
5. Data Processing and Analysis
CPS generate and process vast amounts of data. Skills in data structures, algorithms for efficient data processing, and techniques for data analysis and visualization are crucial. These are core areas covered in AlgoCademy’s curriculum.
6. Machine Learning and AI
Many advanced CPS incorporate machine learning and AI techniques for adaptive control, pattern recognition, and decision-making. AlgoCademy’s coverage of algorithms provides a foundation for understanding and implementing these advanced techniques.
Integrating CPS Concepts into Coding Education
While platforms like AlgoCademy primarily focus on general programming skills and interview preparation, there’s potential to integrate CPS-specific concepts into coding education. Here are some ways this could be approached:
1. CPS-Focused Problem Sets
Develop problem sets that simulate CPS scenarios. For example, create coding challenges that involve optimizing a simulated traffic control system or designing an algorithm for a smart home energy management system.
2. Real-Time Programming Modules
Introduce modules that cover the basics of real-time programming. This could include concepts like task scheduling, interrupt handling, and deadline-driven algorithms.
3. Hardware-Software Integration Projects
Create projects that combine software development with simple hardware interactions. This could involve using microcontrollers or single-board computers to create small-scale CPS prototypes.
4. Distributed Systems Simulations
Develop simulations of distributed systems to help learners understand the challenges of coordinating multiple computational nodes in a CPS context.
5. Data Processing for CPS
Expand on existing data structure and algorithm content to include scenarios relevant to CPS, such as efficient processing of sensor data streams or real-time data fusion algorithms.
Code Example: Simple CPS Simulation
To illustrate some basic concepts of Cyber-Physical Systems, let’s look at a simple Python simulation of a temperature control system. This example demonstrates the interaction between a computational element (the controller) and a physical process (the room temperature).
import random
import time
class Room:
def __init__(self, initial_temp):
self.temperature = initial_temp
self.heater_on = False
def update(self):
if self.heater_on:
self.temperature += 0.5
else:
self.temperature -= 0.1
# Simulate some random fluctuation
self.temperature += random.uniform(-0.1, 0.1)
class TemperatureController:
def __init__(self, target_temp):
self.target_temp = target_temp
def control(self, room):
if room.temperature < self.target_temp - 1:
room.heater_on = True
elif room.temperature > self.target_temp + 1:
room.heater_on = False
def simulate_temperature_control():
room = Room(20) # Start at 20 degrees
controller = TemperatureController(22) # Target temperature is 22 degrees
for _ in range(100): # Simulate for 100 time steps
room.update()
controller.control(room)
print(f"Time: {_}, Temperature: {room.temperature:.2f}, Heater: {'On' if room.heater_on else 'Off'}")
time.sleep(0.1) # Slow down the simulation for readability
simulate_temperature_control()
This simple simulation demonstrates several key aspects of Cyber-Physical Systems:
- Integration of physical processes (room temperature changes) with computation (temperature controller)
- Real-time operation and feedback loop
- Handling of continuous physical quantities (temperature) in a discrete computational system
- Dealing with uncertainty and fluctuations in the physical world
While this is a highly simplified example, it illustrates the basic principles of how computational elements interact with physical processes in a CPS.
The Future of Cyber-Physical Systems
As we look to the future, Cyber-Physical Systems are poised to play an increasingly important role in our lives and industries. Some emerging trends and areas of development include:
1. AI-Enhanced CPS
The integration of artificial intelligence and machine learning techniques is making CPS more adaptive and capable of handling complex, unpredictable environments.
2. Edge Computing in CPS
Moving computation closer to the data source through edge computing is enabling faster response times and reduced bandwidth requirements in large-scale CPS deployments.
3. 5G and Beyond
The rollout of 5G networks and future communication technologies will enable new classes of CPS applications that require ultra-low latency and high reliability.
4. Human-CPS Interaction
As CPS become more prevalent, designing effective and intuitive interfaces for human interaction with these systems is becoming increasingly important.
5. Resilient and Self-Healing CPS
Developing CPS that can adapt to failures, cyber-attacks, and changing environments is a key area of ongoing research.
Conclusion
Cyber-Physical Systems represent a frontier where the digital and physical worlds converge. As we’ve explored, they offer immense potential across various sectors, from manufacturing and transportation to healthcare and urban infrastructure. However, developing effective CPS requires a broad skill set that spans computer science, engineering, and domain-specific knowledge.
For aspiring programmers and computer scientists, platforms like AlgoCademy provide a solid foundation in the algorithmic thinking and problem-solving skills that are crucial for CPS development. As the field continues to evolve, integrating CPS-specific concepts into coding education will become increasingly important.
The journey from learning basic coding skills to developing complex Cyber-Physical Systems is a challenging but rewarding one. It requires continuous learning, adaptability, and a mindset that embraces the intersection of digital and physical realms. As we move further into the era of smart, interconnected systems, the ability to design, implement, and maintain Cyber-Physical Systems will become an increasingly valuable skill in the tech industry and beyond.
Whether you’re just starting your coding journey or looking to expand your skills into the realm of CPS, remember that every line of code you write is a step towards bridging the gap between computation and the physical world. The future of technology lies in this integration, and those who can navigate this complex landscape will be well-positioned to shape the smart, interconnected world of tomorrow.