Crossing Over: How Skills from Other Industries Can Boost Your Coding Career
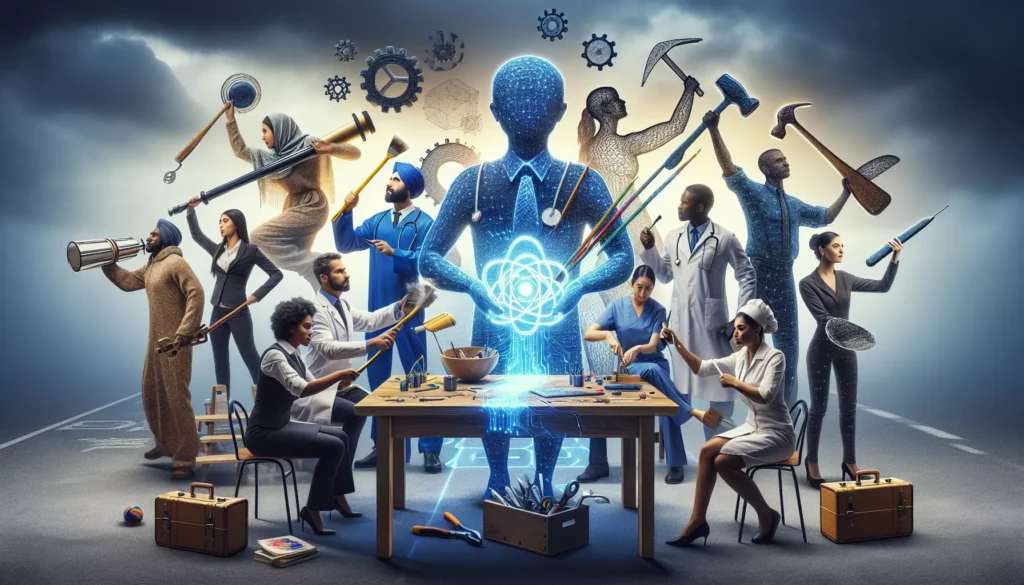
In the ever-evolving world of technology, the path to becoming a successful programmer isn’t always a straight line. While traditional computer science education remains valuable, there’s a growing recognition that skills and experiences from other industries can significantly enhance one’s coding career. This cross-pollination of expertise is not just beneficial—it’s becoming increasingly essential in a tech landscape that values diverse perspectives and interdisciplinary approaches.
At AlgoCademy, we’ve observed that some of our most successful learners come from varied backgrounds, bringing unique insights to their coding journey. Whether you’re a career changer or looking to augment your existing tech skills, understanding how to leverage your prior experiences can give you a significant edge. In this comprehensive guide, we’ll explore how skills from other industries can be applied to coding, and how they can make you a more well-rounded and effective programmer.
1. Problem-Solving Skills from Various Fields
Regardless of the industry, problem-solving is a universal skill that translates exceptionally well to programming. Let’s look at how different fields contribute to this essential coding competency:
Engineering and Mathematics
Engineers and mathematicians are trained to break down complex problems into smaller, manageable parts—a skill directly applicable to algorithmic thinking in programming. Their analytical approach to problem-solving aligns perfectly with the systematic nature of coding.
For example, an engineer’s experience with optimization problems can be invaluable when working on efficiency in algorithms. Consider this simple Python function that an engineer might optimize:
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
An engineer might recognize the potential for stack overflow with large inputs and optimize it to an iterative solution:
def factorial_optimized(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Business and Finance
Professionals from business and finance bring a unique perspective to problem-solving in coding. Their experience with data analysis, pattern recognition, and strategic thinking can be particularly useful in areas like business logic implementation and financial modeling in software.
A finance professional might approach a coding problem with a keen eye for efficiency and risk management. For instance, when designing a trading algorithm, they might emphasize error handling and validation:
def execute_trade(stock_symbol, quantity, price):
try:
if not validate_stock(stock_symbol):
raise ValueError("Invalid stock symbol")
if quantity <= 0:
raise ValueError("Quantity must be positive")
if price <= 0:
raise ValueError("Price must be positive")
# Execute trade logic here
print(f"Trade executed: {quantity} shares of {stock_symbol} at ${price}")
except ValueError as e:
print(f"Trade failed: {str(e)}")
except Exception as e:
print(f"Unexpected error: {str(e)}")
def validate_stock(symbol):
# Implementation of stock validation
pass
Creative Arts
Artists, musicians, and designers bring a different flavor to problem-solving in coding. Their ability to think outside the box and approach problems from unconventional angles can lead to innovative solutions. Creative professionals often excel in user interface design and user experience optimization.
A designer might approach a coding challenge with a focus on the end-user experience. For example, when creating a simple command-line interface, they might emphasize clarity and user-friendliness:
def get_user_input():
print("\n=== Welcome to the Task Manager ===")
print("1. Add a new task")
print("2. View all tasks")
print("3. Mark a task as complete")
print("4. Exit")
while True:
choice = input("\nEnter your choice (1-4): ")
if choice in ['1', '2', '3', '4']:
return int(choice)
else:
print("Invalid input. Please enter a number between 1 and 4.")
2. Communication Skills from Service Industries
Effective communication is crucial in programming, especially in collaborative environments. Professionals from service industries bring valuable skills in this area:
Customer Service
Customer service professionals excel at understanding user needs and translating them into actionable items. This skill is invaluable in requirements gathering and user story creation in software development.
For instance, a former customer service rep might excel at writing clear, user-friendly documentation:
"""
User Registration Function
This function handles the registration of new users in the system.
Parameters:
- username (str): The desired username for the new account. Must be unique.
- email (str): The email address associated with the account. Must be valid and unique.
- password (str): The password for the account. Must be at least 8 characters long.
Returns:
- dict: A dictionary containing the status of the registration and any relevant messages.
Example usage:
result = register_user("john_doe", "john@example.com", "securepass123")
print(result)
# Output: {'status': 'success', 'message': 'User registered successfully'}
"""
def register_user(username, email, password):
# Implementation here
pass
Education
Educators bring strong skills in explaining complex concepts in understandable terms. This is particularly useful in code commenting, documentation, and mentoring junior developers.
An educator might write especially clear and instructive comments in their code:
# The Sieve of Eratosthenes is an ancient algorithm for finding all prime numbers up to a given limit.
# It works by iteratively marking the multiples of each prime number as composite (not prime).
def sieve_of_eratosthenes(n):
# Initialize a boolean array "is_prime[0..n]" and mark all entries as true.
# A value in is_prime[i] will finally be false if i is Not a prime, else true.
is_prime = [True] * (n + 1)
is_prime[0] = is_prime[1] = False # 0 and 1 are not prime numbers
for i in range(2, int(n**0.5) + 1):
if is_prime[i]:
# Update all multiples of i starting from i*i
for j in range(i*i, n+1, i):
is_prime[j] = False
# Collect all prime numbers
primes = [i for i in range(2, n+1) if is_prime[i]]
return primes
# Example usage
print(sieve_of_eratosthenes(30))
# Output: [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
Sales and Marketing
Professionals from sales and marketing backgrounds excel at persuasion and storytelling. These skills are valuable in pitching ideas, presenting projects, and even in writing compelling user interfaces.
A marketer might approach UI text with a focus on engagement and call-to-action:
def display_welcome_message():
print("🚀 Welcome to CodeMaster Pro! 🚀")
print("Unlock your coding potential today!")
print("\nWhat would you like to do?")
print("1. Start a new project (It's quick and easy!)")
print("2. Continue where you left off (Your code misses you!)")
print("3. Explore tutorials (Learn something amazing!)")
choice = input("\nEnter your choice (1-3): ")
return choice
3. Analytical Skills from Research and Academia
The ability to analyze complex systems and data is crucial in programming. Professionals from research and academic backgrounds bring strong analytical skills to the table:
Scientific Research
Researchers are trained to form hypotheses, design experiments, and analyze results—skills that translate well to debugging, testing, and optimizing code.
A researcher might approach testing with a scientific mindset:
import unittest
class TestStringMethods(unittest.TestCase):
def setUp(self):
# This method is called before each test
self.test_string = "Hello, World!"
def test_upper(self):
# Hypothesis: The upper() method should convert all characters to uppercase
self.assertEqual(self.test_string.upper(), "HELLO, WORLD!")
def test_lower(self):
# Hypothesis: The lower() method should convert all characters to lowercase
self.assertEqual(self.test_string.lower(), "hello, world!")
def test_split(self):
# Hypothesis: The split() method should divide the string into a list of substrings
self.assertEqual(self.test_string.split(', '), ['Hello', 'World!'])
def tearDown(self):
# This method is called after each test
pass
if __name__ == '__main__':
unittest.main()
Data Analysis
Data analysts bring skills in pattern recognition, statistical analysis, and data visualization. These skills are invaluable in areas like machine learning, big data processing, and algorithm optimization.
A data analyst might approach a coding problem with a focus on efficient data handling and insightful analysis:
import pandas as pd
import matplotlib.pyplot as plt
def analyze_sales_data(file_path):
# Load the data
df = pd.read_csv(file_path)
# Perform basic analysis
total_sales = df['sales'].sum()
avg_sales = df['sales'].mean()
max_sale = df['sales'].max()
print(f"Total Sales: ${total_sales:.2f}")
print(f"Average Sale: ${avg_sales:.2f}")
print(f"Highest Sale: ${max_sale:.2f}")
# Visualize sales trend
df['date'] = pd.to_datetime(df['date'])
df.set_index('date', inplace=True)
df['sales'].resample('M').sum().plot(kind='bar')
plt.title('Monthly Sales Trend')
plt.xlabel('Month')
plt.ylabel('Total Sales ($)')
plt.show()
# Usage
analyze_sales_data('sales_data.csv')
Philosophy and Logic
Philosophers and logicians bring strong skills in critical thinking, logical reasoning, and argument construction. These skills are particularly useful in areas like algorithm design and formal verification of software.
A philosopher might approach a coding problem by first clearly defining the problem and its logical structure:
def is_valid_argument(premises, conclusion):
"""
Evaluate the validity of a logical argument.
An argument is valid if the conclusion necessarily follows from the premises.
This is a simplified representation and does not cover all aspects of logical validity.
Args:
premises (list): A list of boolean values representing the truth of each premise.
conclusion (bool): A boolean value representing the truth of the conclusion.
Returns:
bool: True if the argument is valid, False otherwise.
"""
# If all premises are true, the conclusion must be true for the argument to be valid
if all(premises) and not conclusion:
return False
# If any premise is false, the argument is vacuously valid
return True
# Example usage
premises = [True, True, False]
conclusion = True
print(f"Is the argument valid? {is_valid_argument(premises, conclusion)}")
4. Project Management Skills from Various Industries
Effective project management is crucial in software development. Professionals from industries that emphasize project management bring valuable skills:
Construction and Engineering
Professionals from construction and engineering are skilled in managing complex projects with multiple dependencies. These skills translate well to software project management, particularly in areas like sprint planning and resource allocation.
An engineer might approach a software project with a structured, phased approach:
class SoftwareProject:
def __init__(self, name):
self.name = name
self.phases = ["Requirements", "Design", "Implementation", "Testing", "Deployment"]
self.current_phase = 0
self.completed = False
def start_next_phase(self):
if self.current_phase < len(self.phases) - 1:
self.current_phase += 1
print(f"Starting {self.phases[self.current_phase]} phase for {self.name}")
else:
self.completed = True
print(f"Project {self.name} completed!")
def project_status(self):
if self.completed:
return f"{self.name} is completed."
else:
return f"{self.name} is in {self.phases[self.current_phase]} phase."
# Usage
project = SoftwareProject("E-commerce Platform")
print(project.project_status()) # E-commerce Platform is in Requirements phase.
project.start_next_phase() # Starting Design phase for E-commerce Platform
project.start_next_phase() # Starting Implementation phase for E-commerce Platform
print(project.project_status()) # E-commerce Platform is in Implementation phase.
Event Planning
Event planners excel at coordinating multiple tasks, managing timelines, and handling unexpected issues. These skills are valuable in agile development environments and in managing software releases.
An event planner might approach sprint planning with a focus on timelines and task coordination:
import datetime
class SprintPlanner:
def __init__(self, sprint_name, start_date, duration_days):
self.sprint_name = sprint_name
self.start_date = start_date
self.end_date = start_date + datetime.timedelta(days=duration_days)
self.tasks = []
def add_task(self, task_name, estimated_hours):
self.tasks.append({"name": task_name, "hours": estimated_hours})
def generate_schedule(self):
print(f"Sprint: {self.sprint_name}")
print(f"Duration: {self.start_date.strftime('%Y-%m-%d')} to {self.end_date.strftime('%Y-%m-%d')}")
print("\nTask Schedule:")
current_date = self.start_date
for task in self.tasks:
print(f"{current_date.strftime('%Y-%m-%d')}: {task['name']} ({task['hours']} hours)")
current_date += datetime.timedelta(days=1)
# Usage
sprint = SprintPlanner("Sprint 1", datetime.date(2023, 6, 1), 14)
sprint.add_task("User Authentication", 16)
sprint.add_task("Database Schema Design", 8)
sprint.add_task("API Development", 24)
sprint.generate_schedule()
5. Creativity and Design Skills from Arts and Media
In an era where user experience is paramount, creativity and design skills are increasingly valuable in programming. Professionals from arts and media bring unique perspectives:
Graphic Design
Graphic designers bring strong visual skills that are invaluable in front-end development, user interface design, and data visualization.
A graphic designer might approach a coding task with a focus on visual aesthetics and user experience:
import tkinter as tk
from tkinter import ttk
class StylishGUI:
def __init__(self, master):
self.master = master
master.title("Stylish App")
master.geometry("300x200")
master.configure(bg='#f0f0f0')
style = ttk.Style()
style.theme_use('clam')
style.configure("TButton",
foreground="#ffffff",
background="#4CAF50",
font=("Arial", 12),
padding=10)
self.label = tk.Label(master, text="Welcome to Stylish App!",
font=("Arial", 16), bg='#f0f0f0')
self.label.pack(pady=20)
self.button = ttk.Button(master, text="Click Me!", command=self.on_button_click)
self.button.pack()
def on_button_click(self):
self.label.config(text="Thanks for clicking!")
root = tk.Tk()
my_gui = StylishGUI(root)
root.mainloop()
Film and Video Production
Professionals from film and video production bring skills in storytelling, pacing, and visual narrative. These skills can be applied to creating engaging user interfaces and crafting intuitive user flows in applications.
A filmmaker might approach a user onboarding process with a focus on narrative and engagement:
class UserOnboarding:
def __init__(self):
self.steps = [
"Welcome to our app!",
"Let's set up your profile.",
"Choose your interests.",
"Connect with friends.",
"You're all set!"
]
self.current_step = 0
def next_step(self):
if self.current_step < len(self.steps) - 1:
self.current_step += 1
return self.steps[self.current_step]
else:
return "Onboarding complete!"
def display_progress(self):
progress = (self.current_step + 1) / len(self.steps) * 100
bar_length = 20
filled_length = int(bar_length * progress // 100)
bar = 'â–ˆ' * filled_length + '-' * (bar_length - filled_length)
return f"[{bar}] {progress:.0f}%"
# Usage
onboarding = UserOnboarding()
print(onboarding.steps[0]) # Welcome to our app!
print(onboarding.display_progress()) # [████----------------] 20%
print(onboarding.next_step()) # Let's set up your profile.
print(onboarding.display_progress()) # [████████--------------] 40%
Music Production
Musicians and music producers bring skills in pattern recognition, rhythm, and harmony. These skills can be applied to areas like algorithm design, particularly in areas involving patterns and sequences.
A musician might approach a coding problem with an ear for patterns and repetition:
def generate_rhythm(beats, pattern):
"""
Generate a rhythmic pattern.
Args:
beats (int): The number of beats in the pattern.
pattern (str): A string of 'x' and '.' representing hits and rests.
Returns:
str: The complete rhythmic pattern.
"""
full_pattern = pattern * (beats // len(pattern))
remainder = beats % len(pattern)
return full_pattern + pattern[:remainder]
def play_rhythm(rhythm):
"""
Simulate playing a rhythm.
Args:
rhythm (str): A string of 'x' and '.' representing hits and rests.
"""
for beat in rhythm:
if beat == 'x':
print("*", end="") # Represent a hit
else:
print("-", end="") # Represent a rest
print() # New line at the end
# Usage
basic_pattern = "x.x."
full_rhythm = generate_rhythm(16, basic_pattern)
play_rhythm(full_rhythm)
# Output: *-*-*-*-*-*-*-*-
6. Adaptability and Learning Skills from Diverse Backgrounds
Perhaps the most valuable skill that professionals from other industries bring to coding is adaptability. The ability to learn quickly, adapt to new situations, and apply existing knowledge in novel ways is crucial in the fast-paced world of technology.
Career Changers
Those who have successfully changed careers demonstrate a high capacity for learning and adaptability. These skills are invaluable in the ever-evolving field of programming.
A career changer might approach learning a new programming concept with a structured, goal-oriented approach:
class LearningPlan:
def __init__(self, topic):
self.topic = topic
self.resources = []
self.progress = 0
def add_resource(self, resource_name, resource_type):
self.resources.append({"name": resource_name, "type": resource_type, "completed": False})
def complete_resource(self, resource_name):
for resource in self.resources:
if resource["name"] == resource_name:
resource["completed"] = True
self.update_progress()
break
def update_progress(self):
completed = sum(1 for resource in self.resources if resource["completed"])
self.progress = (completed / len(self.resources)) * 100 if self.resources else 0
def display_plan(self):
print(f"Learning Plan for {self.topic}")
print(f"Progress: {self.progress:.2f}%")
print("\nResources:")
for resource in self.resources:
status = "✓" if resource["completed"] else " "
print(f"[{status}] {resource['name']} ({resource['type']})")
# Usage
plan = LearningPlan("Python Data Structures")
plan.add_resource("Lists and Tuples Tutorial", "Video")
plan.add_resource("Dictionary Deep Dive", "Article")
plan.add_resource("Sets and Frozen Sets", "Book Chapter")
plan.complete_resource("Lists and Tuples Tutorial")
plan.display_plan()
Multilingual Individuals
People who speak multiple languages often have an easier time learning new programming languages. Their experience with different grammatical structures and syntax rules can be advantageous when learning various programming paradigms.
A multilingual person might approach learning multiple programming languages with a comparative method:
class LanguageComparison:
def __init__(self):
self.languages = {}
def add_language(self, name, features):
self.languages[name] = features
def compare_feature(self, feature):
print(f"Comparison of {feature}:")
for lang, features in self.languages.items():
if feature in features:
print(f"{lang}: {features[feature]}")
else:
print(f"{lang}: Not specified")
print()
# Usage
comparison = LanguageComparison()
comparison.add_language("Python", {
"type_system": "Dynamic",
"paradigm": "Multi-paradigm",
"syntax": "Indentation-based"
})
comparison.add_language("Java", {
"type_system": "Static",
"paradigm": "Object-oriented",
"syntax": "Curly brace"
})
comparison.add_language("JavaScript", {
"type_system": "Dynamic",
"paradigm": "Multi-paradigm",
"syntax": "Curly brace"
})
comparison.compare_feature("type_system")
comparison.compare_feature("paradigm")
Conclusion: The Power of Diverse Experiences in Coding
As we’ve explored throughout this article, the skills and experiences from various industries can significantly enhance one’s coding abilities. From problem-solving and communication to project management and creativity, each background brings unique strengths to the table.
At AlgoCademy, we believe in the power of diverse perspectives in coding education. Our platform is designed to accommodate learners from all backgrounds, providing interactive coding tutorials and AI-powered assistance that can help you leverage your unique skills in your coding journey.
Remember, your past experiences are not baggage to be discarded as you enter the world of programming—they are valuable assets that can set you apart in the tech industry. Embrace your diverse background, and use it to fuel your growth as a programmer.
Whether you’re a seasoned professional looking to change careers or someone adding coding skills to your existing repertoire, AlgoCademy is here to support your journey. Our resources, from beginner-level coding tutorials to advanced preparation for technical interviews at major tech companies, are designed to help you succeed.
So, as you continue your coding education, don’t forget to draw upon your unique experiences. They may just be the key to unlocking your full potential as a programmer. Happy coding!