Creating a Study Plan for Coding Interviews: A Comprehensive Guide
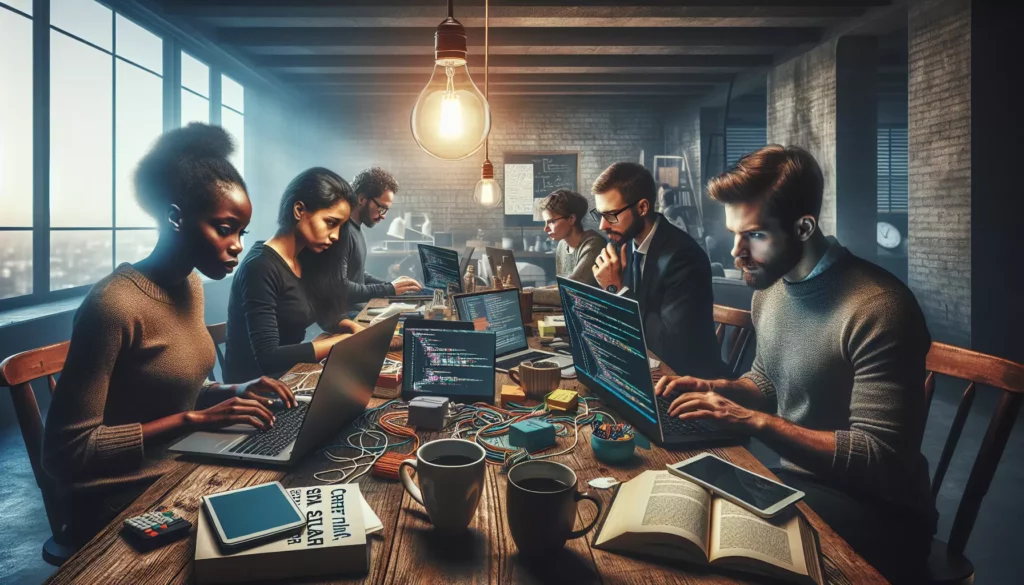
In the competitive world of software engineering, landing a job at a top tech company like Google, Amazon, or Facebook (often collectively referred to as FAANG) is a dream for many aspiring developers. However, the road to success in these coveted positions is paved with challenging coding interviews that test not only your programming skills but also your problem-solving abilities and algorithmic thinking. To navigate this journey successfully, you need a well-structured study plan that covers all the essential areas and prepares you for the rigorous interview process.
In this comprehensive guide, we’ll walk you through the process of creating an effective study plan for coding interviews. We’ll cover everything from assessing your current skill level to mastering specific topics and practicing mock interviews. By the end of this article, you’ll have a clear roadmap to follow as you prepare for your dream job in the tech industry.
1. Assess Your Current Skill Level
Before diving into your study plan, it’s crucial to understand where you stand in terms of your coding skills and knowledge. This self-assessment will help you identify your strengths and weaknesses, allowing you to tailor your study plan accordingly.
1.1. Take Online Coding Assessments
Start by taking online coding assessments on platforms like HackerRank, LeetCode, or CodeSignal. These platforms offer a variety of problems that simulate coding interview questions. Attempt a mix of easy, medium, and hard problems to gauge your current level.
1.2. Review Core Computer Science Concepts
Evaluate your understanding of fundamental computer science concepts, including:
- Data Structures (arrays, linked lists, trees, graphs, etc.)
- Algorithms (sorting, searching, dynamic programming, etc.)
- Time and Space Complexity Analysis
- Object-Oriented Programming (OOP) principles
- Database concepts and SQL
- Operating Systems basics
- Computer Networks fundamentals
1.3. Analyze Your Performance
After completing the assessments and reviewing core concepts, analyze your performance. Identify areas where you excel and those that need improvement. This analysis will form the foundation of your personalized study plan.
2. Set Clear Goals and Timelines
With a clear understanding of your current skill level, it’s time to set specific goals and establish a timeline for your preparation.
2.1. Define Your Target Companies
Research the companies you’re interested in and understand their interview processes. Different companies may focus on different aspects of coding and problem-solving, so tailoring your preparation to your target companies is essential.
2.2. Set a Target Date
Determine when you want to be ready for interviews. This could be based on application deadlines, personal goals, or your current job situation. Having a target date will help you structure your study plan and stay motivated.
2.3. Break Down Your Goals
Divide your overall goal into smaller, manageable objectives. For example:
- Master a new data structure every week
- Solve 5 coding problems daily
- Complete one system design question per week
- Review one computer science concept daily
3. Create a Structured Curriculum
Now that you have your goals in place, it’s time to create a structured curriculum that covers all the essential topics for coding interviews.
3.1. Data Structures and Algorithms
This is the core of most coding interviews. Ensure your curriculum covers the following data structures and algorithms:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
- Tries
Algorithms:
- Sorting (QuickSort, MergeSort, HeapSort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Divide and Conquer
- Recursion
3.2. Problem-Solving Techniques
Learn and practice various problem-solving techniques, including:
- Two-pointer technique
- Sliding window
- Fast and slow pointers
- Merge intervals
- Cyclic sort
- In-place reversal of a linked list
- Tree traversals (DFS and BFS)
- Graph algorithms (Dijkstra’s, Floyd-Warshall, etc.)
3.3. System Design
For more senior positions or certain companies, system design questions are crucial. Include the following topics in your curriculum:
- Scalability concepts
- Load balancing
- Caching
- Database sharding
- Microservices architecture
- API design
- Distributed systems
3.4. Programming Languages
Choose one or two programming languages to focus on during your preparation. Popular choices include:
- Python
- Java
- C++
- JavaScript
Ensure you have a deep understanding of the language(s) you choose, including their standard libraries and built-in data structures.
3.5. Object-Oriented Programming
Review and practice OOP concepts, including:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Design patterns
3.6. Database and SQL
Brush up on database concepts and SQL queries:
- ACID properties
- Indexing
- Normalization
- Join operations
- Subqueries
- Aggregation functions
4. Develop a Study Schedule
With your curriculum in place, create a study schedule that fits your lifestyle and learning preferences.
4.1. Allocate Time for Each Topic
Divide your available study time among the different topics based on their importance and your current skill level. For example:
- 50% on Data Structures and Algorithms
- 20% on Problem-Solving Techniques
- 15% on System Design
- 10% on Programming Language Proficiency
- 5% on Miscellaneous Topics (OOP, Databases, etc.)
4.2. Create a Weekly Schedule
Design a weekly schedule that includes:
- Daily problem-solving sessions
- Dedicated time for learning new concepts
- Review sessions for previously learned material
- Mock interview practice
4.3. Use the Pomodoro Technique
Implement the Pomodoro Technique to maintain focus and avoid burnout. This involves studying for 25-minute intervals followed by 5-minute breaks, with a longer break after every four Pomodoros.
5. Utilize Effective Learning Resources
To maximize your learning efficiency, use a combination of high-quality resources:
5.1. Online Platforms
Leverage coding platforms that offer structured learning paths and practice problems:
- LeetCode
- HackerRank
- AlgoExpert
- InterviewBit
5.2. Books
Supplement your online learning with comprehensive books on algorithms and interview preparation:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “Introduction to Algorithms” by Thomas H. Cormen, et al.
- “Elements of Programming Interviews” by Adnan Aziz, et al.
5.3. Video Courses
Enroll in video courses that provide in-depth explanations and visualizations:
- Coursera’s “Algorithms Specialization” by Stanford University
- MIT OpenCourseWare’s “Introduction to Algorithms”
- Udemy’s “Master the Coding Interview: Data Structures + Algorithms”
5.4. GitHub Repositories
Explore curated GitHub repositories that compile interview preparation resources:
- TheAlgorithms
- coding-interview-university
- tech-interview-handbook
6. Implement Active Learning Techniques
To ensure that you’re not just passively consuming information, incorporate active learning techniques into your study plan:
6.1. Implement from Scratch
Instead of relying solely on built-in data structures and algorithms, implement them from scratch. This deepens your understanding and prepares you for questions that require custom implementations.
6.2. Teach Others
Explain concepts to others, either in person or through online forums. Teaching reinforces your understanding and highlights areas where you need more clarity.
6.3. Write Blog Posts
Document your learning journey by writing blog posts about the concepts you’re studying. This helps consolidate your knowledge and creates a valuable resource for future reference.
6.4. Participate in Coding Competitions
Join online coding competitions on platforms like Codeforces or TopCoder. These competitions simulate the time pressure of real interviews and expose you to a wide range of problems.
7. Practice, Practice, Practice
Consistent practice is the key to success in coding interviews. Incorporate the following practice techniques into your study plan:
7.1. Daily Coding Problems
Solve at least one coding problem every day. Start with easier problems and gradually increase the difficulty as you improve.
7.2. Timed Practice Sessions
Simulate interview conditions by setting a timer (usually 45-60 minutes) and solving problems without interruptions.
7.3. Mock Interviews
Conduct mock interviews with friends, mentors, or through platforms like Pramp or interviewing.io. This helps you get comfortable with explaining your thought process and coding under pressure.
7.4. Review and Optimize Solutions
After solving a problem, review your solution and look for ways to optimize it. Compare your approach with other solutions and learn from different perspectives.
8. Focus on Communication and Soft Skills
Technical skills alone are not enough to ace coding interviews. Develop your communication and soft skills to stand out:
8.1. Practice Thinking Aloud
Get comfortable explaining your thought process as you solve problems. This helps interviewers understand your approach and problem-solving skills.
8.2. Learn to Ask Clarifying Questions
Don’t hesitate to ask for clarification on problem requirements or constraints. This shows that you’re thorough and helps you avoid misunderstandings.
8.3. Develop a Problem-Solving Framework
Create a structured approach to tackling coding problems:
- Understand the problem
- Identify the inputs and outputs
- Consider edge cases
- Develop a high-level approach
- Implement the solution
- Test and debug
- Analyze time and space complexity
- Discuss potential optimizations
9. Stay Consistent and Motivated
Preparing for coding interviews is a marathon, not a sprint. Implement strategies to stay consistent and motivated throughout your preparation:
9.1. Track Your Progress
Use a spreadsheet or a habit-tracking app to monitor your daily progress. Seeing your consistent efforts can be a great motivator.
9.2. Join Study Groups
Connect with other aspiring developers who are also preparing for coding interviews. Share resources, discuss problems, and motivate each other.
9.3. Celebrate Small Wins
Acknowledge your progress, no matter how small. Solved a difficult problem? Mastered a new concept? Celebrate these achievements to maintain a positive mindset.
9.4. Take Regular Breaks
Avoid burnout by taking regular breaks and engaging in activities you enjoy. A refreshed mind is more effective at problem-solving and retaining information.
10. Continuously Refine Your Study Plan
As you progress through your preparation, regularly assess and refine your study plan:
10.1. Review Your Progress
Every few weeks, review your progress against your initial goals. Are you on track? Are there areas where you’re falling behind?
10.2. Adjust Your Focus
Based on your progress review, adjust the time allocation for different topics. You may need to spend more time on challenging areas or explore advanced topics if you’re progressing faster than expected.
10.3. Stay Updated with Industry Trends
Keep an eye on the latest trends in coding interviews. New types of questions or focus areas may emerge, and you’ll want to incorporate these into your study plan.
10.4. Seek Feedback
If possible, seek feedback from experienced developers or mentors. They can provide valuable insights into your preparation and suggest areas for improvement.
Conclusion
Creating a comprehensive study plan for coding interviews is a crucial step towards landing your dream job in the tech industry. By following the steps outlined in this guide – from assessing your current skills to continuously refining your plan – you’ll be well-prepared to tackle even the most challenging coding interviews.
Remember that consistency is key. Stick to your study plan, practice regularly, and stay motivated. With dedication and the right approach, you’ll be well on your way to impressing interviewers and securing a position at top tech companies.
As you embark on this journey, keep in mind that the skills you’re developing are not just for passing interviews. The problem-solving abilities, algorithmic thinking, and deep understanding of computer science concepts will serve you well throughout your career as a software engineer.
Good luck with your preparation, and may your hard work lead you to success in your coding interviews and beyond!