Crafting Optimal Solutions from Brute Force Approaches
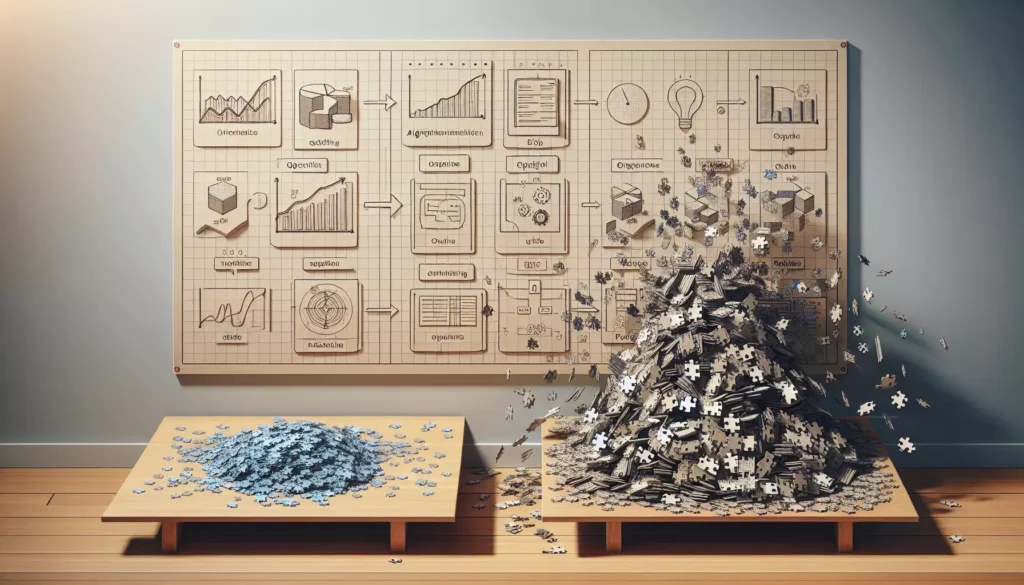
In the world of programming and algorithm design, the journey from a brute force solution to an optimal one is both challenging and rewarding. This process is at the heart of what we teach at AlgoCademy, where we focus on developing strong problem-solving skills and algorithmic thinking. In this comprehensive guide, we’ll explore how to transform basic, inefficient solutions into elegant, optimized algorithms – a crucial skill for any programmer aiming to excel in technical interviews and real-world software development.
Understanding Brute Force Approaches
Before we dive into optimization techniques, let’s first understand what a brute force approach is and why it’s often the starting point for problem-solving.
What is a Brute Force Approach?
A brute force approach is a straightforward method of solving a problem that relies on sheer computing power and trying every possibility rather than employing more advanced techniques or algorithms. While it’s often the most intuitive way to solve a problem, it’s rarely the most efficient.
Characteristics of Brute Force Solutions:
- Simplicity: They are usually the easiest to implement and understand.
- Guaranteed correctness: They always find the correct answer (given enough time and resources).
- Inefficiency: They often have poor time complexity, making them impractical for large inputs.
- Resource-intensive: They may require significant computational power or memory.
Example of a Brute Force Approach
Let’s consider a classic problem: finding all pairs of numbers in an array that sum to a given target. A brute force solution might look like this:
def find_pairs_brute_force(arr, target):
pairs = []
n = len(arr)
for i in range(n):
for j in range(i+1, n):
if arr[i] + arr[j] == target:
pairs.append((arr[i], arr[j]))
return pairs
# Example usage
array = [1, 5, 7, 1, 5, 3, 4, 2]
target = 6
result = find_pairs_brute_force(array, target)
print(result) # Output: [(1, 5), (1, 5), (4, 2)]
This solution works by checking every possible pair of numbers in the array. While it’s correct, it has a time complexity of O(n^2), which becomes problematic for large arrays.
The Importance of Optimization
While brute force solutions are a great starting point, they often fall short in real-world scenarios due to their inefficiency. Here’s why optimization matters:
- Performance: Optimized solutions run faster and use fewer resources, crucial for handling large datasets or real-time applications.
- Scalability: Efficient algorithms allow your solutions to scale with increasing input sizes.
- Cost-effectiveness: In cloud computing environments, more efficient algorithms directly translate to lower operational costs.
- User experience: Faster algorithms lead to more responsive applications and better user satisfaction.
- Interview success: Top tech companies (FAANG and beyond) expect candidates to optimize their solutions during technical interviews.
Strategies for Optimizing Brute Force Solutions
Now that we understand the importance of optimization, let’s explore some strategies to transform brute force approaches into more efficient solutions.
1. Identify Redundant Computations
Often, brute force solutions perform the same calculations multiple times. Identifying and eliminating these redundancies can significantly improve efficiency.
Example: Fibonacci Sequence
Consider a naive recursive implementation of the Fibonacci sequence:
def fibonacci_brute_force(n):
if n <= 1:
return n
return fibonacci_brute_force(n-1) + fibonacci_brute_force(n-2)
# Example usage
print(fibonacci_brute_force(30)) # This will take a long time
This implementation recalculates the same Fibonacci numbers multiple times. We can optimize it using memoization:
def fibonacci_optimized(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci_optimized(n-1, memo) + fibonacci_optimized(n-2, memo)
return memo[n]
# Example usage
print(fibonacci_optimized(30)) # Much faster!
2. Use Appropriate Data Structures
Choosing the right data structure can dramatically improve the efficiency of your algorithm. Each data structure has its strengths and weaknesses, and selecting the appropriate one can lead to significant optimizations.
Example: Two Sum Problem
Let’s optimize our earlier brute force solution for finding pairs that sum to a target:
def find_pairs_optimized(arr, target):
seen = {}
pairs = []
for num in arr:
complement = target - num
if complement in seen:
pairs.append((complement, num))
seen[num] = True
return pairs
# Example usage
array = [1, 5, 7, 1, 5, 3, 4, 2]
target = 6
result = find_pairs_optimized(array, target)
print(result) # Output: [(1, 5), (1, 5), (4, 2)]
By using a hash table (dictionary in Python), we’ve reduced the time complexity from O(n^2) to O(n).
3. Leverage Mathematical Properties
Many problems have underlying mathematical properties that can be exploited for optimization. Understanding these properties can lead to elegant and efficient solutions.
Example: Finding Prime Numbers
A brute force approach to find prime numbers up to n might look like this:
def is_prime_brute_force(n):
if n < 2:
return False
for i in range(2, n):
if n % i == 0:
return False
return True
def find_primes_brute_force(n):
return [num for num in range(2, n+1) if is_prime_brute_force(num)]
# Example usage
print(find_primes_brute_force(30)) # Output: [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
We can optimize this using the Sieve of Eratosthenes, which leverages the fact that multiples of primes are not prime:
def sieve_of_eratosthenes(n):
primes = [True] * (n + 1)
primes[0] = primes[1] = False
for i in range(2, int(n**0.5) + 1):
if primes[i]:
for j in range(i*i, n+1, i):
primes[j] = False
return [num for num in range(2, n+1) if primes[num]]
# Example usage
print(sieve_of_eratosthenes(30)) # Output: [2, 3, 5, 7, 11, 13, 17, 19, 23, 29]
This optimized solution has a time complexity of O(n log log n), which is much more efficient than the O(n^2) of the brute force approach.
4. Divide and Conquer
The divide and conquer strategy involves breaking a problem into smaller subproblems, solving them independently, and then combining the results. This approach often leads to more efficient recursive solutions.
Example: Merge Sort
Consider sorting an array. A simple brute force approach might use bubble sort:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
print(bubble_sort([64, 34, 25, 12, 22, 11, 90]))
We can optimize this using the divide and conquer strategy of merge sort:
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] <= right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
# Example usage
print(merge_sort([64, 34, 25, 12, 22, 11, 90]))
Merge sort has a time complexity of O(n log n), which is significantly better than bubble sort’s O(n^2), especially for large arrays.
5. Dynamic Programming
Dynamic programming is a powerful technique for optimizing recursive solutions by storing the results of expensive function calls and reusing them when needed. It’s particularly useful for problems with overlapping subproblems.
Example: Longest Common Subsequence
Let’s consider finding the longest common subsequence (LCS) of two strings. A naive recursive approach might look like this:
def lcs_recursive(X, Y, m, n):
if m == 0 or n == 0:
return 0
elif X[m-1] == Y[n-1]:
return 1 + lcs_recursive(X, Y, m-1, n-1)
else:
return max(lcs_recursive(X, Y, m, n-1), lcs_recursive(X, Y, m-1, n))
# Example usage
X = "AGGTAB"
Y = "GXTXAYB"
print(lcs_recursive(X, Y, len(X), len(Y))) # Output: 4
This recursive solution has an exponential time complexity. We can optimize it using dynamic programming:
def lcs_dp(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
# Example usage
X = "AGGTAB"
Y = "GXTXAYB"
print(lcs_dp(X, Y)) # Output: 4
The dynamic programming solution has a time complexity of O(mn), which is much more efficient than the exponential complexity of the recursive approach.
Best Practices for Optimization
As you work on optimizing your solutions, keep these best practices in mind:
- Start with a working solution: Begin with a brute force approach to ensure you understand the problem and have a correct solution.
- Analyze the current solution: Identify bottlenecks and inefficiencies in your brute force approach.
- Consider multiple approaches: There’s often more than one way to optimize a solution. Explore different strategies.
- Use profiling tools: Leverage profiling tools to identify which parts of your code are consuming the most time and resources.
- Test thoroughly: Ensure your optimized solution still produces correct results for all test cases.
- Balance readability and efficiency: While optimization is important, maintain code readability. An overly complex solution can be hard to maintain.
- Document your optimization: Explain your optimization process and the reasoning behind your choices.
- Consider space-time tradeoffs: Sometimes, using more memory can significantly reduce time complexity. Evaluate these tradeoffs based on your specific requirements.
Real-world Applications
The skills you develop in optimizing algorithms have wide-ranging applications in the tech industry:
- Database Query Optimization: Improving the efficiency of database queries can significantly enhance the performance of data-intensive applications.
- Machine Learning: Optimizing machine learning algorithms can lead to faster training times and more efficient model inference.
- Web Development: Efficient algorithms are crucial for creating responsive web applications, especially those dealing with large datasets or real-time processing.
- Game Development: Optimized algorithms are essential for rendering graphics, simulating physics, and managing game state in real-time.
- Financial Systems: In high-frequency trading and risk analysis, even small optimizations can lead to significant competitive advantages.
- Bioinformatics: Efficient algorithms are crucial for analyzing large genomic datasets and performing complex biological simulations.
Conclusion
The journey from brute force to optimal solutions is a fundamental aspect of algorithmic thinking and a key focus at AlgoCademy. By mastering these optimization techniques, you’ll not only improve your problem-solving skills but also enhance your ability to create efficient, scalable software solutions. Remember, optimization is an iterative process that requires practice and creativity.
As you continue your coding education and prepare for technical interviews, especially for top tech companies, focus on developing your ability to recognize optimization opportunities and apply these strategies effectively. With consistent practice and a deep understanding of these concepts, you’ll be well-equipped to tackle complex programming challenges and excel in your software development career.
Keep coding, keep optimizing, and never stop learning!