Course Schedule Problem: Mastering Topological Sorting in Programming Interviews
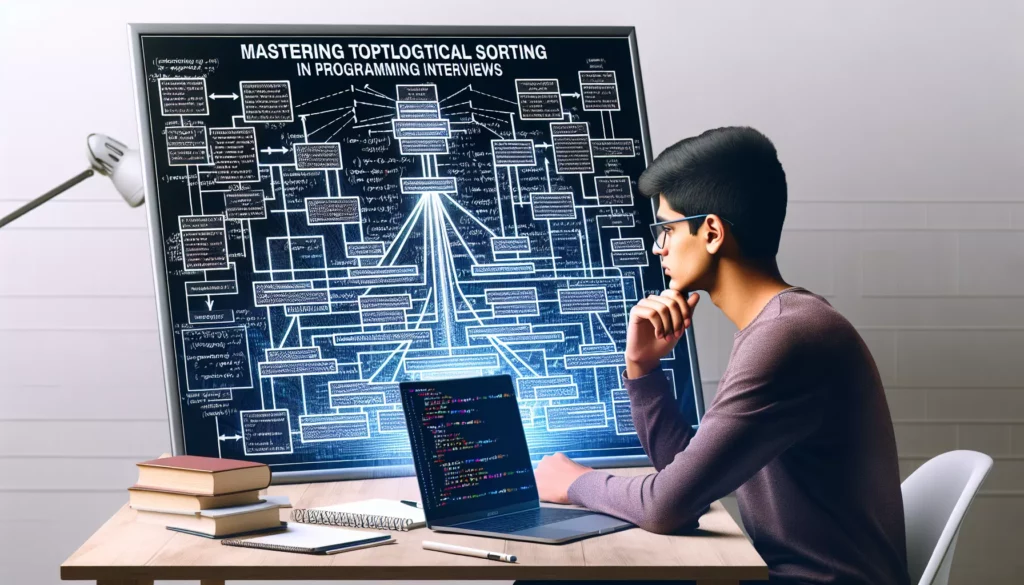
Welcome to another insightful tutorial from AlgoCademy! Today, we’re diving deep into a classic problem that often appears in coding interviews, especially those conducted by major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). We’ll be exploring the Course Schedule Problem, which is an excellent application of topological sorting and graph theory.
Understanding the Course Schedule Problem
Before we delve into the solution, let’s first understand what the Course Schedule Problem entails. Imagine you’re a student planning your academic schedule. You have a list of courses you need to take, but some courses have prerequisites. The challenge is to determine if it’s possible to complete all the courses given these prerequisites.
Here’s a formal definition of the problem:
There are a total of n courses you have to take, labeled from 0 to n-1. Some courses may have prerequisites, for example, to take course 0 you have to first take course 1, which is expressed as a pair: [0,1]. Given the total number of courses and a list of prerequisite pairs, is it possible for you to finish all courses?
This problem is a perfect application of topological sorting, a concept in graph theory that’s crucial for many real-world scenarios, including dependency resolution, build systems, and, of course, course scheduling.
Topological Sorting: The Key to Solving the Course Schedule Problem
Topological sorting is an algorithm for ordering the vertices of a directed acyclic graph (DAG) such that for every directed edge from vertex A to vertex B, A comes before B in the ordering. In the context of our course schedule problem, each course is a vertex, and each prerequisite relationship is a directed edge.
The key insight here is that if we can perform a topological sort on the graph representing our courses and prerequisites, then we can complete all courses. If we can’t (due to a cycle in the graph), then it’s impossible to complete all courses.
Implementing the Solution
Let’s implement this solution step by step. We’ll use Python for our implementation, but the concepts can be easily translated to other programming languages.
Step 1: Represent the Graph
First, we need to represent our courses and prerequisites as a graph. We’ll use an adjacency list representation.
from collections import defaultdict
def canFinish(numCourses, prerequisites):
# Create a graph represented as an adjacency list
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
# Rest of the code will go here
In this representation, each course is a key in our graph
dictionary, and its value is a list of its prerequisites.
Step 2: Implement Depth-First Search (DFS)
We’ll use a depth-first search approach to detect cycles in our graph. If we encounter a cycle, it means there’s a circular dependency in our course prerequisites, making it impossible to complete all courses.
# Set to keep track of visited nodes
visited = set()
# Set to keep track of nodes in the current DFS path
path = set()
def dfs(course):
# If we've already processed this course, it's safe
if course in visited:
return True
# If we encounter a course already in our current path, we have a cycle
if course in path:
return False
# Add the course to our current path
path.add(course)
# Recursively check all prerequisites
for prereq in graph[course]:
if not dfs(prereq):
return False
# Remove the course from our current path
path.remove(course)
# Mark the course as fully processed
visited.add(course)
return True
# Check each course
for course in range(numCourses):
if not dfs(course):
return False
return True
This DFS implementation does a few key things:
- It keeps track of all visited nodes to avoid unnecessary re-processing.
- It maintains a set of nodes in the current DFS path to detect cycles.
- If it encounters a node already in the current path, it means there’s a cycle, and we return
False
. - If it successfully processes all prerequisites of a course without finding a cycle, it marks the course as visited and returns
True
.
Putting It All Together
Here’s the complete implementation of our solution:
from collections import defaultdict
def canFinish(numCourses, prerequisites):
# Create a graph represented as an adjacency list
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
# Set to keep track of visited nodes
visited = set()
# Set to keep track of nodes in the current DFS path
path = set()
def dfs(course):
# If we've already processed this course, it's safe
if course in visited:
return True
# If we encounter a course already in our current path, we have a cycle
if course in path:
return False
# Add the course to our current path
path.add(course)
# Recursively check all prerequisites
for prereq in graph[course]:
if not dfs(prereq):
return False
# Remove the course from our current path
path.remove(course)
# Mark the course as fully processed
visited.add(course)
return True
# Check each course
for course in range(numCourses):
if not dfs(course):
return False
return True
# Test the function
print(canFinish(2, [[1,0]])) # Should return True
print(canFinish(2, [[1,0],[0,1]])) # Should return False
Time and Space Complexity Analysis
Understanding the time and space complexity of our solution is crucial for optimizing our code and preparing for technical interviews. Let’s break it down:
Time Complexity
The time complexity of our solution is O(V + E), where V is the number of vertices (courses) and E is the number of edges (prerequisites) in our graph.
- We iterate through each course once in our main loop: O(V)
- For each course, we perform a DFS that explores all its prerequisites: O(E)
In the worst case, where we have to explore all edges for each vertex, this gives us O(V + E).
Space Complexity
The space complexity of our solution is also O(V + E).
- We store the graph as an adjacency list, which takes O(V + E) space.
- Our
visited
andpath
sets can contain at most V elements each: O(V) - The recursion stack in our DFS can go as deep as V in the worst case: O(V)
Adding these up, we get O(V + E) space complexity.
Common Variations and Follow-up Questions
In coding interviews, especially at top tech companies, interviewers often present variations of the original problem or ask follow-up questions. Here are some common ones for the Course Schedule Problem:
1. Course Schedule II
Instead of just determining if it’s possible to finish all courses, you’re asked to return the order in which the courses should be taken. This is essentially asking for the topological sort itself.
def findOrder(numCourses, prerequisites):
graph = defaultdict(list)
for course, prereq in prerequisites:
graph[course].append(prereq)
visited = set()
path = set()
order = []
def dfs(course):
if course in visited:
return True
if course in path:
return False
path.add(course)
for prereq in graph[course]:
if not dfs(prereq):
return False
path.remove(course)
visited.add(course)
order.append(course)
return True
for course in range(numCourses):
if not dfs(course):
return []
return order[::-1] # Reverse the order
2. Minimum Semesters to Complete All Courses
In this variation, you’re asked to determine the minimum number of semesters needed to complete all courses, assuming you can take any number of courses in a semester as long as you have completed all their prerequisites.
from collections import deque
def minSemesters(numCourses, prerequisites):
graph = defaultdict(list)
in_degree = [0] * numCourses
for course, prereq in prerequisites:
graph[prereq].append(course)
in_degree[course] += 1
queue = deque([i for i in range(numCourses) if in_degree[i] == 0])
semesters = 0
while queue:
semesters += 1
for _ in range(len(queue)):
course = queue.popleft()
for next_course in graph[course]:
in_degree[next_course] -= 1
if in_degree[next_course] == 0:
queue.append(next_course)
return semesters if sum(in_degree) == 0 else -1
3. Course Schedule with Limited Slots
In this variation, each semester has a limited number of slots for courses. You need to determine if it’s possible to complete all courses within a given number of semesters.
def canFinishWithLimitedSlots(numCourses, prerequisites, semesters, slotsPerSemester):
graph = defaultdict(list)
in_degree = [0] * numCourses
for course, prereq in prerequisites:
graph[prereq].append(course)
in_degree[course] += 1
queue = deque([i for i in range(numCourses) if in_degree[i] == 0])
courses_taken = 0
for _ in range(semesters):
slots = slotsPerSemester
for _ in range(len(queue)):
if slots == 0:
break
course = queue.popleft()
courses_taken += 1
slots -= 1
for next_course in graph[course]:
in_degree[next_course] -= 1
if in_degree[next_course] == 0:
queue.append(next_course)
return courses_taken == numCourses
Real-world Applications
The Course Schedule Problem and its variations have numerous real-world applications beyond academic scheduling. Understanding these can help you appreciate the importance of this problem and may even give you an edge in interviews. Here are some practical applications:
1. Build Systems
In software development, build systems need to compile source files in the correct order based on their dependencies. This is essentially a topological sort problem, similar to our course schedule.
2. Task Scheduling in Project Management
When managing complex projects with interdependent tasks, determining a feasible schedule is crucial. The course schedule algorithm can be adapted to create project timelines.
3. Package Managers
Software package managers need to resolve dependencies and install packages in the correct order. This is another application of topological sorting.
4. Data Processing Pipelines
In data engineering, processing pipelines often have stages that depend on the output of previous stages. Determining the order of execution is a topological sort problem.
5. Circuit Design
In electronic circuit design, components need to be evaluated in a specific order based on their connections. This ordering can be determined using topological sorting.
Interview Tips and Tricks
When tackling the Course Schedule Problem or its variations in an interview setting, keep these tips in mind:
- Clarify the Problem: Make sure you understand all the constraints and requirements. Ask questions if anything is unclear.
- Start with the Basics: Begin by explaining how you would represent the courses and prerequisites as a graph.
- Explain Your Approach: Before diving into coding, explain your high-level approach. Mention that you’ll be using topological sorting and why it’s appropriate for this problem.
- Consider Edge Cases: Discuss what happens if there are no prerequisites, or if there’s a cycle in the prerequisites.
- Optimize: If you have time after implementing a working solution, consider ways to optimize it. Can you reduce space usage? Can you make it more efficient for sparse graphs?
- Test Your Code: After implementing your solution, walk through a few test cases to ensure it works correctly.
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution.
- Think About Follow-ups: If the interviewer doesn’t ask, you can volunteer ideas about how you might modify your solution for variations of the problem.
Conclusion
The Course Schedule Problem is a fantastic example of how graph theory and algorithms apply to real-world scenarios. By mastering this problem and its variations, you’re not just preparing for coding interviews; you’re developing problem-solving skills that are valuable in many areas of software development and beyond.
Remember, the key to success in coding interviews is not just about memorizing solutions, but understanding the underlying concepts and being able to apply them flexibly to different scenarios. Keep practicing, and don’t hesitate to explore more advanced graph algorithms and their applications.
At AlgoCademy, we’re committed to helping you develop these crucial skills. Our interactive coding tutorials, AI-powered assistance, and comprehensive resources are designed to take you from a coding beginner to a confident technical interview candidate. Keep exploring, keep coding, and remember – every challenge is an opportunity to learn and grow!
Happy coding, and best of luck with your interviews!