Counting Sort: A Linear Time Sorting Algorithm
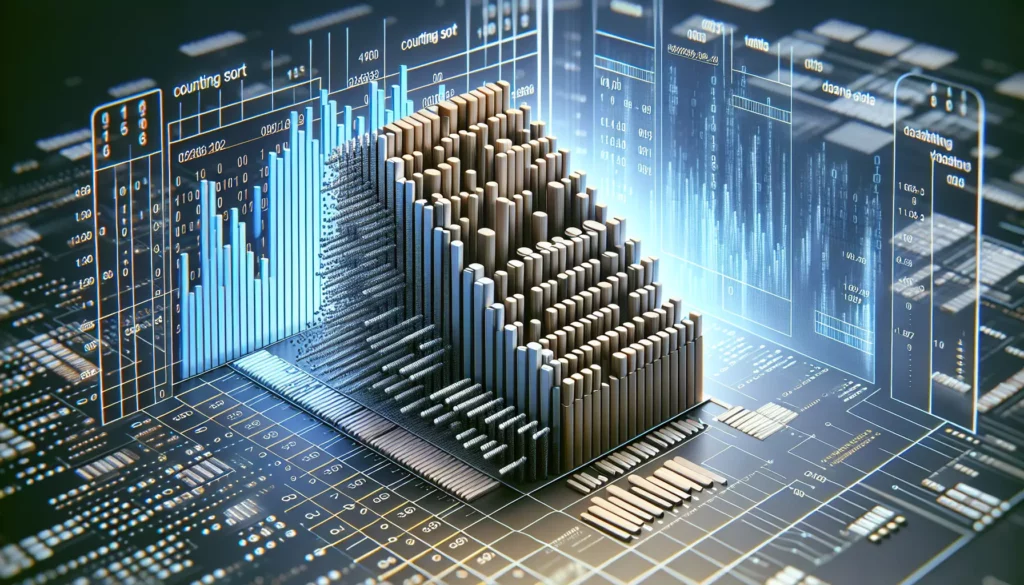
Sorting algorithms are fundamental in computer science, and among the various sorting techniques, Counting Sort stands out for its efficiency in specific scenarios. This article will delve deep into the Counting Sort algorithm, exploring its mechanics, implementation, time complexity, and practical applications.
Table of Contents
- Introduction to Counting Sort
- How Counting Sort Works
- Implementation of Counting Sort
- Time and Space Complexity
- Advantages of Counting Sort
- Disadvantages and Limitations
- Practical Applications
- Comparison with Other Sorting Algorithms
- Variations and Improvements
- Conclusion
1. Introduction to Counting Sort
Counting Sort is a non-comparison-based sorting algorithm that works efficiently when dealing with a limited range of input values. Unlike popular algorithms such as Quick Sort or Merge Sort, which rely on comparing elements, Counting Sort uses the actual values of the elements to sort them.
The key idea behind Counting Sort is to determine, for each element, how many elements are smaller than it. This information directly yields the position of each element in the sorted output. Counting Sort is particularly effective when the range of potential values in the input array is not significantly larger than the number of elements to be sorted.
2. How Counting Sort Works
The Counting Sort algorithm operates in three main steps:
- Counting Phase: Count the occurrences of each element in the input array.
- Accumulation Phase: Modify the count array to store the actual position of each element in the output array.
- Placement Phase: Build the output array using the modified count array.
Let’s break down these steps in more detail:
2.1 Counting Phase
In this phase, we create a count array to store the count of each unique element in the input array. The size of the count array is determined by the range of input values.
For example, if we have an input array [4, 2, 2, 8, 3, 3, 1] with values ranging from 1 to 8, we would create a count array of size 8. After counting, our count array would look like this:
Index: 0 1 2 3 4 5 6 7 8
Count: 0 1 2 2 1 0 0 0 1
This indicates that we have one 1, two 2s, two 3s, one 4, and one 8 in our input array.
2.2 Accumulation Phase
In this phase, we modify the count array so that each element stores the actual position of the corresponding element in the sorted output. We do this by adding each count to the sum of all previous counts.
Continuing our example, after the accumulation phase, our count array would become:
Index: 0 1 2 3 4 5 6 7 8
Count: 0 1 3 5 6 6 6 6 7
Now, each count represents the position of the last occurrence of its index value in the sorted output.
2.3 Placement Phase
In the final phase, we build the output array. We iterate through the input array from right to left (to maintain stability), use the count array to determine the correct position for each element, place the element in that position in the output array, and decrement the count.
After this phase, we have our sorted array: [1, 2, 2, 3, 3, 4, 8]
3. Implementation of Counting Sort
Let’s implement the Counting Sort algorithm in Python:
def counting_sort(arr):
# Find the range of input values
max_val = max(arr)
min_val = min(arr)
range_of_values = max_val - min_val + 1
# Initialize the count array
count = [0] * range_of_values
# Counting phase
for num in arr:
count[num - min_val] += 1
# Accumulation phase
for i in range(1, len(count)):
count[i] += count[i-1]
# Placement phase
output = [0] * len(arr)
for num in reversed(arr):
index = count[num - min_val] - 1
output[index] = num
count[num - min_val] -= 1
return output
# Example usage
arr = [4, 2, 2, 8, 3, 3, 1]
sorted_arr = counting_sort(arr)
print(sorted_arr) # Output: [1, 2, 2, 3, 3, 4, 8]
This implementation works for both positive and negative integers. It first finds the range of values in the input array to determine the size of the count array. The rest of the implementation follows the three phases described earlier.
4. Time and Space Complexity
One of the most attractive features of Counting Sort is its time complexity. Let’s analyze it in detail:
4.1 Time Complexity
- Counting Phase: O(n), where n is the number of elements in the input array.
- Accumulation Phase: O(k), where k is the range of input values.
- Placement Phase: O(n)
The overall time complexity is O(n + k). When k is O(n), the time complexity becomes O(n), making Counting Sort a linear time sorting algorithm.
4.2 Space Complexity
The space complexity of Counting Sort is O(n + k):
- O(n) for the output array
- O(k) for the count array
This makes Counting Sort less space-efficient than in-place sorting algorithms like Quick Sort when k is large.
5. Advantages of Counting Sort
Counting Sort offers several advantages in specific scenarios:
- Linear Time Complexity: When the range of input values is not significantly larger than the number of elements, Counting Sort provides O(n) time complexity, outperforming comparison-based sorting algorithms.
- Stability: Counting Sort is a stable sorting algorithm, meaning it preserves the relative order of equal elements in the sorted output.
- Simplicity: The algorithm is straightforward to understand and implement.
- Efficient for Small Integers: It’s particularly efficient when sorting small integers or elements that can be converted to small integers.
6. Disadvantages and Limitations
Despite its advantages, Counting Sort has some limitations:
- Limited Range: It’s only efficient when the range of potential values is not significantly larger than the number of elements to be sorted.
- Not In-Place: Counting Sort requires additional space proportional to the range of input values, making it less memory-efficient for large ranges.
- Integer-Only: In its basic form, Counting Sort only works with integers or data that can be mapped to integers.
- Not Suitable for Large Ranges: If the range of values is very large compared to the number of elements, Counting Sort becomes inefficient in both time and space.
7. Practical Applications
Counting Sort finds applications in various scenarios:
- Sorting Student Scores: When sorting test scores within a fixed range (e.g., 0-100), Counting Sort can be very efficient.
- Sorting Ages: In demographic data analysis, sorting ages (typically within a range of 0-120) can be done efficiently using Counting Sort.
- Sorting Characters: When sorting strings or characters (with a known character set), Counting Sort can be used effectively.
- Radix Sort: Counting Sort is often used as a subroutine in Radix Sort, which can sort integers with a larger range.
- Bucket Sort: Counting Sort can be used within each bucket in the Bucket Sort algorithm.
8. Comparison with Other Sorting Algorithms
Let’s compare Counting Sort with some other popular sorting algorithms:
8.1 Counting Sort vs. Quick Sort
- Time Complexity: Counting Sort: O(n+k), Quick Sort: O(n log n) on average
- Space Complexity: Counting Sort: O(n+k), Quick Sort: O(log n) for the call stack
- Stability: Counting Sort is stable, Quick Sort is not
- In-Place: Counting Sort is not in-place, Quick Sort is in-place
Counting Sort outperforms Quick Sort when k is O(n), but Quick Sort is more versatile and efficient for a wider range of inputs.
8.2 Counting Sort vs. Merge Sort
- Time Complexity: Counting Sort: O(n+k), Merge Sort: O(n log n)
- Space Complexity: Counting Sort: O(n+k), Merge Sort: O(n)
- Stability: Both are stable
- In-Place: Neither is in-place in their standard implementations
Merge Sort is more versatile, but Counting Sort is faster for small ranges of integers.
8.3 Counting Sort vs. Heap Sort
- Time Complexity: Counting Sort: O(n+k), Heap Sort: O(n log n)
- Space Complexity: Counting Sort: O(n+k), Heap Sort: O(1)
- Stability: Counting Sort is stable, Heap Sort is not
- In-Place: Counting Sort is not in-place, Heap Sort is in-place
Heap Sort is more space-efficient and works well for a wider range of inputs, but Counting Sort is faster for small ranges of integers.
9. Variations and Improvements
Several variations and improvements have been proposed for Counting Sort:
9.1 Generalized Counting Sort
This variation allows Counting Sort to work with negative numbers and non-integer values by using a mapping function.
9.2 In-Place Counting Sort
While not commonly used, in-place variations of Counting Sort have been developed to reduce space complexity at the cost of increased time complexity.
9.3 Parallel Counting Sort
Parallelized versions of Counting Sort have been proposed to take advantage of multi-core processors and distributed systems.
9.4 Counting Sort with Bucket Sort
For larger ranges, Counting Sort can be combined with Bucket Sort to create a more efficient algorithm for certain distributions of data.
10. Conclusion
Counting Sort is a powerful and efficient sorting algorithm when used in the right context. Its linear time complexity makes it an excellent choice for sorting integers or data that can be mapped to integers within a small range. While it has limitations, particularly in terms of space complexity and the range of values it can efficiently handle, Counting Sort remains an important tool in a programmer’s arsenal.
Understanding the mechanics, advantages, and limitations of Counting Sort allows developers to make informed decisions about when to use this algorithm. In scenarios where the input constraints align with Counting Sort’s strengths, it can provide significant performance improvements over comparison-based sorting algorithms.
As with all algorithms, the key to effective use lies in recognizing the characteristics of your data and the requirements of your specific problem. By mastering Counting Sort and understanding its place in the broader landscape of sorting algorithms, you’ll be better equipped to choose the right tool for each sorting task you encounter.