Count of Range Sum: Mastering a Challenging Algorithm
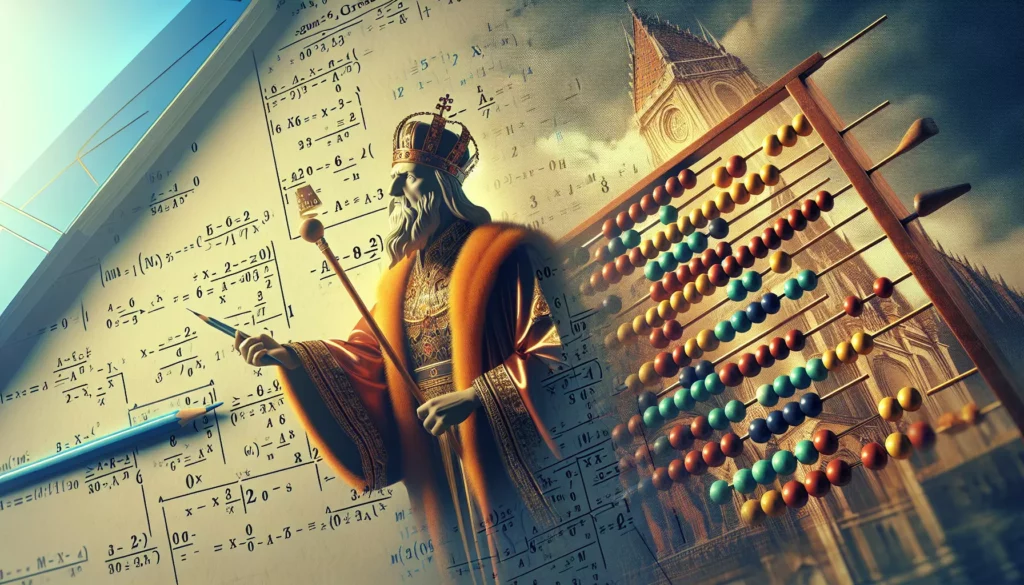
Welcome to another in-depth exploration of advanced algorithms on AlgoCademy! Today, we’re diving into a particularly challenging problem that often appears in technical interviews for top tech companies: the Count of Range Sum. This problem is a testament to the importance of algorithmic thinking and efficient problem-solving skills that are crucial for any aspiring software engineer.
Understanding the Problem
Before we delve into the solution, let’s clearly define the problem:
Given an integer array
nums
and two integerslower
andupper
, return the number of range sums that lie in[lower, upper]
inclusive.Range sum
S(i, j)
is defined as the sum of the elements innums
between indicesi
andj
inclusive, wherei <= j
.
This problem might seem straightforward at first glance, but it quickly becomes complex when we consider the constraints and the need for an efficient solution. A naive approach would be to calculate all possible range sums and count those within the given range, but this would result in a time complexity of O(n^2), which is too slow for large inputs.
The Challenges
The Count of Range Sum problem presents several challenges:
- Efficiency: We need to find a solution that’s more efficient than the naive O(n^2) approach.
- Range Handling: We must efficiently handle the range constraint [lower, upper].
- Prefix Sums: Understanding and utilizing prefix sums is crucial for an optimal solution.
- Data Structure Choice: Selecting the right data structure can significantly impact the algorithm’s performance.
Approach 1: Merge Sort Solution
One of the most efficient solutions to this problem involves using a modified merge sort algorithm. This approach leverages the divide-and-conquer paradigm and the properties of sorted arrays to achieve a time complexity of O(n log n).
Key Concepts
- Prefix Sums: We’ll use prefix sums to efficiently calculate range sums.
- Merge Sort: We’ll modify the merge sort algorithm to count range sums during the merge step.
- Two Pointers: We’ll use two pointers to efficiently count the number of valid range sums.
Implementation
Let’s break down the implementation step by step:
class Solution {
private int lower;
private int upper;
private int count;
private long[] temp;
public int countRangeSum(int[] nums, int lower, int upper) {
this.lower = lower;
this.upper = upper;
this.count = 0;
int n = nums.length;
long[] prefixSums = new long[n + 1];
this.temp = new long[n + 1];
// Calculate prefix sums
for (int i = 0; i < n; i++) {
prefixSums[i + 1] = prefixSums[i] + nums[i];
}
mergeSort(prefixSums, 0, n);
return count;
}
private void mergeSort(long[] arr, int left, int right) {
if (left >= right) return;
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
private void merge(long[] arr, int left, int mid, int right) {
int i = left, j = mid + 1, k = left;
int p = mid + 1, q = mid + 1;
while (i <= mid) {
while (p <= right && arr[p] - arr[i] < lower) p++;
while (q <= right && arr[q] - arr[i] <= upper) q++;
count += q - p;
while (j <= right && arr[j] < arr[i]) {
temp[k++] = arr[j++];
}
temp[k++] = arr[i++];
}
while (j <= right) {
temp[k++] = arr[j++];
}
System.arraycopy(temp, left, arr, left, right - left + 1);
}
}
Explanation
- We start by calculating the prefix sums of the input array. This allows us to compute any range sum in constant time.
- We then perform a merge sort on the prefix sums array.
- During the merge step, we use two pointers (p and q) to find the range of elements that satisfy the lower and upper bounds.
- The count of valid range sums is incremented by q – p in each iteration.
- We also perform the standard merge operation to sort the array.
Time and Space Complexity
- Time Complexity: O(n log n), where n is the length of the input array. This comes from the merge sort algorithm.
- Space Complexity: O(n) for the temporary array used in merge sort.
Approach 2: Binary Indexed Tree (Fenwick Tree) Solution
Another efficient approach to solving the Count of Range Sum problem involves using a Binary Indexed Tree (BIT), also known as a Fenwick Tree. This data structure allows us to perform range sum queries and updates in O(log n) time.
Key Concepts
- Binary Indexed Tree: A data structure that efficiently updates elements and calculates prefix sums in a table of numbers.
- Discretization: A technique to map the continuous range of sums to a discrete set of indices.
- Coordinate Compression: A method to reduce the range of values we need to consider, making the BIT more efficient.
Implementation
class Solution {
private int[] bit;
private int n;
public int countRangeSum(int[] nums, int lower, int upper) {
int n = nums.length;
long[] prefixSums = new long[n + 1];
for (int i = 0; i < n; i++) {
prefixSums[i + 1] = prefixSums[i] + nums[i];
}
Set<Long> allNumbers = new TreeSet<>();
for (long sum : prefixSums) {
allNumbers.add(sum);
allNumbers.add(sum - lower);
allNumbers.add(sum - upper);
}
Map<Long, Integer> ranks = new HashMap<>();
int rank = 0;
for (long num : allNumbers) {
ranks.put(num, ++rank);
}
this.n = ranks.size();
this.bit = new int[this.n + 1];
int count = 0;
for (long sum : prefixSums) {
count += query(ranks.get(sum - lower)) - query(ranks.get(sum - upper) - 1);
update(ranks.get(sum), 1);
}
return count;
}
private void update(int index, int val) {
while (index <= n) {
bit[index] += val;
index += index & (-index);
}
}
private int query(int index) {
int sum = 0;
while (index > 0) {
sum += bit[index];
index -= index & (-index);
}
return sum;
}
}
Explanation
- We start by calculating the prefix sums of the input array.
- We perform coordinate compression by collecting all unique values (including the lower and upper bound differences) and mapping them to ranks.
- We initialize a Binary Indexed Tree with the size of the compressed coordinate space.
- We iterate through the prefix sums, querying the BIT for the count of elements in the range [sum – upper, sum – lower] and updating the BIT with the current sum.
- The final count gives us the number of range sums within the specified bounds.
Time and Space Complexity
- Time Complexity: O(n log n), where n is the length of the input array. This comes from the sorting step in coordinate compression and the log n operations on the BIT.
- Space Complexity: O(n) for the BIT and the coordinate mapping.
Comparing the Approaches
Both the Merge Sort and Binary Indexed Tree approaches offer efficient solutions to the Count of Range Sum problem, with some trade-offs:
- Merge Sort Approach:
- Pros: Conceptually simpler, doesn’t require additional data structures.
- Cons: Requires careful implementation of the merge step.
- Binary Indexed Tree Approach:
- Pros: More flexible, can handle dynamic updates if needed.
- Cons: Requires understanding of an additional data structure (BIT) and coordinate compression.
Both approaches achieve the same time complexity of O(n log n), making them suitable for large inputs. The choice between them might depend on the specific requirements of the problem or personal preference.
Common Pitfalls and Tips
When tackling the Count of Range Sum problem, be aware of these common pitfalls:
- Integer Overflow: Be cautious of integer overflow when calculating sums. Use long integers where necessary.
- Edge Cases: Consider edge cases like empty arrays or when lower == upper.
- Off-by-One Errors: Be careful with array indices, especially when dealing with prefix sums.
- Efficiency: Avoid naive O(n^2) solutions, as they will likely exceed time limits for large inputs.
Here are some tips to help you solve this problem effectively:
- Practice implementing merge sort and binary indexed trees separately before tackling this problem.
- Use pen and paper to visualize the algorithm, especially for the merge sort approach.
- Test your solution with various input sizes to ensure it scales well.
- Consider the trade-offs between different approaches and be prepared to explain your choice in an interview setting.
Related Problems
If you found the Count of Range Sum problem interesting, you might want to explore these related problems:
- Reverse Pairs: Another problem that can be solved efficiently using a modified merge sort.
- Count of Smaller Numbers After Self: A problem that uses similar techniques to Count of Range Sum.
- Range Sum Query – Mutable: A problem where Binary Indexed Trees can be effectively applied.
- The Skyline Problem: A more complex problem that often uses similar divide-and-conquer techniques.
Conclusion
The Count of Range Sum problem is a challenging algorithmic task that tests your ability to think creatively and implement efficient solutions. By mastering this problem, you’re not only preparing for technical interviews but also honing your skills in algorithm design and data structure manipulation.
Remember, the key to solving complex problems like this is to break them down into smaller, manageable parts. Start with a clear understanding of the problem, consider multiple approaches, and always think about efficiency. With practice, you’ll find yourself tackling even the most challenging algorithmic problems with confidence.
Keep coding, keep learning, and don’t hesitate to revisit this problem as you continue to grow your skills. Happy coding!