Converting Strings to Integers in JavaScript: A Comprehensive Guide
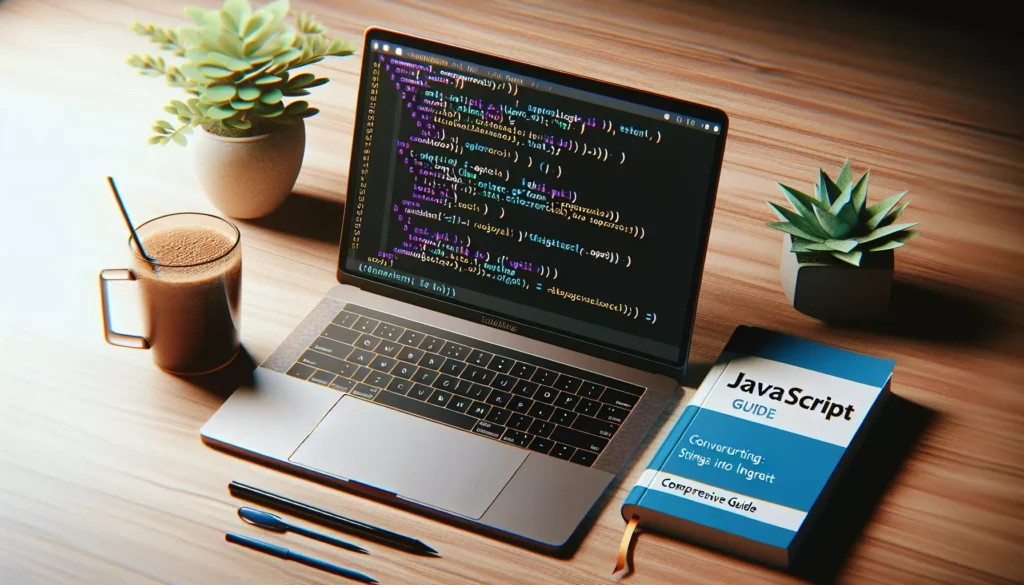
Converting strings to integers is a common task in JavaScript programming. Whether you’re working with user input, parsing data from an API, or manipulating strings in your code, knowing how to effectively convert strings to integers is essential. In this comprehensive guide, we’ll explore various methods and best practices for string to integer conversion in JavaScript.
Table of Contents
- Understanding the Basics
- The parseInt() Function
- The Number() Function
- The Unary Plus Operator
- Math.floor() Method
- Bitwise NOT Operator
- Handling Edge Cases and Error Checking
- Performance Considerations
- Best Practices and Common Pitfalls
- Real-world Examples
- Conclusion
1. Understanding the Basics
Before diving into the various methods of converting strings to integers, it’s important to understand what happens behind the scenes. In JavaScript, strings and numbers are two distinct data types. When you convert a string to an integer, you’re essentially changing the data type of the value.
JavaScript provides several built-in methods and operators to perform this conversion. Each method has its own nuances and use cases, which we’ll explore in detail.
2. The parseInt() Function
The parseInt()
function is one of the most commonly used methods for converting strings to integers in JavaScript. It parses a string argument and returns an integer of the specified radix or base.
Basic Syntax:
parseInt(string, radix)
Where:
string
is the value to parse. If this argument is not a string, it is converted to a string first.radix
is an integer between 2 and 36 that represents the numerical base used in the string. This parameter is optional, but it’s recommended to always specify it to avoid confusion.
Examples:
console.log(parseInt("10")); // Output: 10
console.log(parseInt("10.5")); // Output: 10
console.log(parseInt("10", 10)); // Output: 10 (base 10)
console.log(parseInt("1010", 2)); // Output: 10 (binary)
console.log(parseInt("FF", 16)); // Output: 255 (hexadecimal)
The parseInt()
function is particularly useful when you need to handle different number bases or when you want to extract an integer from the beginning of a string that might contain non-numeric characters.
Handling Leading Zeros:
It’s important to note that parseInt()
treats strings with leading zeros as octal (base 8) in ECMAScript 3 and older. To avoid this, always specify the radix:
console.log(parseInt("08")); // Output: 0 (in older browsers)
console.log(parseInt("08", 10)); // Output: 8 (always correct)
3. The Number() Function
The Number()
function converts a string to a number, which can be either an integer or a floating-point number, depending on the input.
Basic Syntax:
Number(value)
Examples:
console.log(Number("10")); // Output: 10
console.log(Number("10.5")); // Output: 10.5
console.log(Number("10.0")); // Output: 10
console.log(Number("10.00")); // Output: 10
console.log(Number("10e2")); // Output: 1000
console.log(Number("0xFF")); // Output: 255
The Number()
function is more strict than parseInt()
when it comes to parsing. It will return NaN
(Not a Number) for inputs that cannot be fully converted to a number.
console.log(Number("10px")); // Output: NaN
console.log(parseInt("10px")); // Output: 10
4. The Unary Plus Operator
The unary plus operator (+
) is a quick and concise way to convert a string to a number. It works similarly to the Number()but with a more compact syntax.
Examples:
console.log(+"10"); // Output: 10
console.log(+"10.5"); // Output: 10.5
console.log(+"10e2"); // Output: 1000
console.log(+"0xFF"); // Output: 255
console.log(+"10px"); // Output: NaN
The unary plus operator is often used for its brevity, especially in situations where you’re confident that the input will be a valid number string.
5. Math.floor() Method
While not directly a string-to-integer conversion method, Math.floor()
can be used in combination with other methods to ensure you get an integer result, especially when dealing with floating-point numbers.
Examples:
console.log(Math.floor("10.5")); // Output: 10
console.log(Math.floor("10.9")); // Output: 10
console.log(Math.floor("-10.5")); // Output: -11
Note that Math.floor()
automatically coerces its argument to a number, so you don’t need to use Number()
or the unary plus operator explicitly.
6. Bitwise NOT Operator
The bitwise NOT operator (~
) can be used as a trick to convert a string to an integer. This method is less common and should be used with caution, as it’s not immediately clear what it does.
Example:
console.log(~~"10"); // Output: 10
console.log(~~"10.5"); // Output: 10
console.log(~~"-10.5"); // Output: -10
This method works by applying the bitwise NOT operator twice. The first ~
converts the string to a number and inverts all the bits, and the second ~
inverts them back, effectively truncating any decimal places.
7. Handling Edge Cases and Error Checking
When converting strings to integers, it’s crucial to handle edge cases and implement proper error checking to ensure your code is robust and reliable.
Handling NaN:
Always check for NaN
results, especially when using methods like Number()
or the unary plus operator:
function safeConvert(str) {
const num = Number(str);
if (isNaN(num)) {
console.error("Invalid number:", str);
return null;
}
return num;
}
console.log(safeConvert("10")); // Output: 10
console.log(safeConvert("abc")); // Output: null (and logs an error)
Handling Infinity:
Be aware that very large numbers might result in Infinity
:
console.log(Number("1e1000")); // Output: Infinity
Handling Empty Strings:
Different methods handle empty strings differently:
console.log(parseInt("")); // Output: NaN
console.log(Number("")); // Output: 0
console.log(+""); // Output: 0
Handling Whitespace:
Most conversion methods automatically trim whitespace, but it’s good to be aware of this behavior:
console.log(parseInt(" 10 ")); // Output: 10
console.log(Number(" 10 ")); // Output: 10
console.log(+" 10 "); // Output: 10
8. Performance Considerations
When it comes to performance, the differences between these methods are generally negligible for most applications. However, in high-performance scenarios or when dealing with large datasets, these differences can add up.
Benchmark:
Here’s a simple benchmark to compare the performance of different conversion methods:
function benchmark(fn, iterations = 1000000) {
const start = performance.now();
for (let i = 0; i < iterations; i++) {
fn("12345");
}
const end = performance.now();
console.log(`${fn.name}: ${end - start} ms`);
}
benchmark(parseInt);
benchmark(Number);
benchmark(str => +str);
benchmark(str => ~~str);
benchmark(str => Math.floor(str));
The results may vary depending on the JavaScript engine and the specific use case, but generally, the unary plus operator and Number()
tend to be the fastest methods.
9. Best Practices and Common Pitfalls
When converting strings to integers in JavaScript, following best practices can help you write more reliable and maintainable code. Here are some tips and common pitfalls to avoid:
Best Practices:
- Always specify the radix with parseInt(): This prevents unexpected behavior with leading zeros.
- Use Number() or unary plus for simple conversions: These methods are concise and generally faster.
- Implement error checking: Always handle potential NaN results in your code.
- Be consistent: Choose one method and use it consistently throughout your codebase for better readability.
- Document your choice: If you’re using a less common method (like bitwise NOT), comment your code to explain why.
Common Pitfalls:
- Forgetting to handle NaN: Always check for NaN results to prevent errors from propagating through your code.
- Assuming all inputs are valid: User input or data from external sources may not always be in the expected format.
- Ignoring decimal places: Be aware that most integer conversion methods truncate decimal places rather than rounding.
- Overlooking locale-specific number formats: Different locales may use different decimal and thousand separators.
10. Real-world Examples
Let’s look at some real-world scenarios where string to integer conversion is commonly used:
Parsing User Input:
function calculateArea() {
const length = document.getElementById("length").value;
const width = document.getElementById("width").value;
const area = Number(length) * Number(width);
if (isNaN(area)) {
document.getElementById("result").textContent = "Please enter valid numbers.";
} else {
document.getElementById("result").textContent = `The area is ${area} square units.`;
}
}
Working with API Data:
async function fetchUserAge(userId) {
try {
const response = await fetch(`https://api.example.com/users/${userId}`);
const data = await response.json();
const age = parseInt(data.age, 10);
if (isNaN(age)) {
console.error("Invalid age data received");
return null;
}
return age;
} catch (error) {
console.error("Error fetching user data:", error);
return null;
}
}
Parsing CSV Data:
function parseCSV(csvString) {
const rows = csvString.split("\n");
return rows.map(row => {
const columns = row.split(",");
return {
id: parseInt(columns[0], 10),
name: columns[1],
age: parseInt(columns[2], 10),
salary: parseFloat(columns[3])
};
});
}
const csvData = `
1,John Doe,30,50000.00
2,Jane Smith,28,55000.50
3,Bob Johnson,35,60000.75
`;
console.log(parseCSV(csvData));
Implementing a Simple Calculator:
function calculate(operation, a, b) {
const num1 = Number(a);
const num2 = Number(b);
if (isNaN(num1) || isNaN(num2)) {
return "Invalid input";
}
switch (operation) {
case "add":
return num1 + num2;
case "subtract":
return num1 - num2;
case "multiply":
return num1 * num2;
case "divide":
return num2 !== 0 ? num1 / num2 : "Cannot divide by zero";
default:
return "Invalid operation";
}
}
console.log(calculate("add", "10", "5")); // Output: 15
console.log(calculate("divide", "10", "0")); // Output: Cannot divide by zero
console.log(calculate("multiply", "abc", "5")); // Output: Invalid input
11. Conclusion
Converting strings to integers is a fundamental operation in JavaScript programming. We’ve explored various methods, including parseInt()
, Number()
, the unary plus operator, Math.floor()
, and even the bitwise NOT operator. Each method has its strengths and use cases, and choosing the right one depends on your specific requirements.
Remember to always handle potential errors and edge cases, especially when dealing with user input or external data. Performance differences between these methods are generally minimal, so focus on code readability and consistency in your projects.
By mastering string to integer conversion techniques, you’ll be better equipped to handle numeric data in your JavaScript applications, leading to more robust and reliable code.
As you continue to develop your JavaScript skills, keep experimenting with these methods and stay updated with the latest ECMAScript features that might introduce new ways of handling numeric conversions. Happy coding!