Converting Recursive Solutions to Iterative: A Strategic Approach
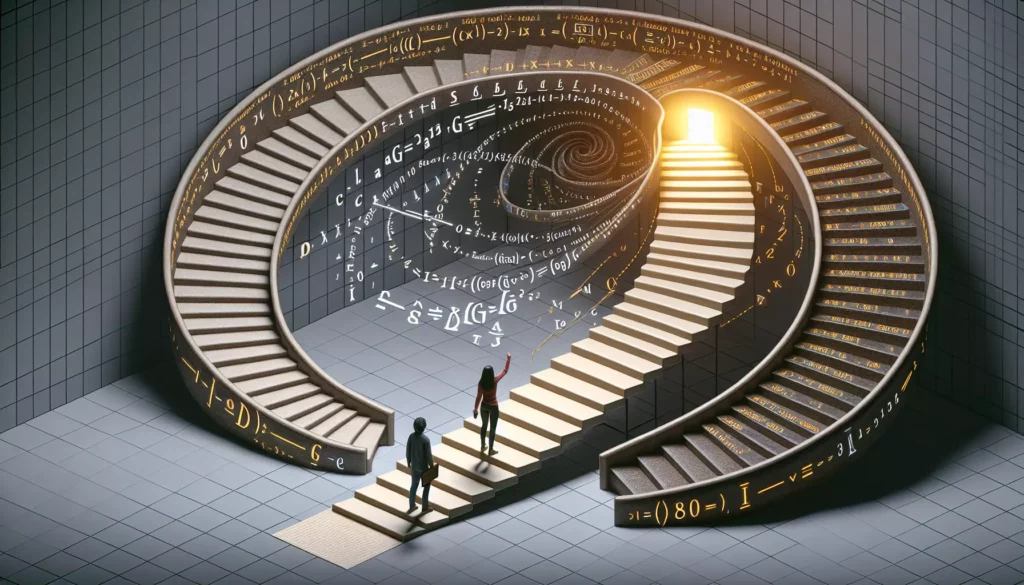
In the world of programming, recursion is a powerful and elegant technique for solving complex problems. However, it’s not always the most efficient or practical solution, especially when dealing with large datasets or in environments with limited stack space. This is where the ability to convert recursive solutions to iterative ones becomes a valuable skill. In this comprehensive guide, we’ll explore the strategic approach to transforming recursive algorithms into their iterative counterparts, providing you with the tools and understanding to optimize your code and tackle technical interviews with confidence.
Understanding Recursion and Iteration
Before we dive into the conversion process, let’s briefly review the concepts of recursion and iteration:
Recursion
Recursion is a programming technique where a function calls itself to solve a problem by breaking it down into smaller, similar subproblems. It’s often used for tasks that have a naturally recursive structure, such as traversing tree-like data structures or implementing divide-and-conquer algorithms.
Iteration
Iteration, on the other hand, uses loops (such as for, while, or do-while) to repeat a set of instructions until a condition is met. It’s generally more straightforward to implement and can be more efficient in terms of memory usage and execution time for certain problems.
Why Convert Recursive Solutions to Iterative?
There are several reasons why you might want to convert a recursive solution to an iterative one:
- Stack Overflow Prevention: Recursive calls consume stack space, which can lead to stack overflow errors for deeply nested recursions or large inputs.
- Performance Improvement: Iterative solutions often have better time and space complexity, especially for tail-recursive functions.
- Better Control Flow: Iterative solutions can provide more explicit control over the execution flow, making it easier to add additional logic or optimizations.
- Language/Environment Constraints: Some programming languages or environments may have limitations on recursion depth or may not optimize tail recursion.
The Strategic Approach to Conversion
Converting a recursive solution to an iterative one requires a systematic approach. Here’s a step-by-step strategy you can follow:
1. Identify the Recursive Pattern
The first step is to analyze the recursive function and identify its core components:
- Base case(s): The condition(s) that stop the recursion
- Recursive case(s): The part of the function that makes the recursive call(s)
- State: The information passed between recursive calls
2. Choose an Appropriate Data Structure
Based on the recursive pattern, select a data structure to simulate the function call stack:
- Stack: For linear recursion (single recursive call)
- Queue: For breadth-first traversals
- Custom structure: For more complex recursion patterns
3. Initialize the Iteration
Set up the initial state and the chosen data structure with the starting values.
4. Implement the Main Loop
Create a loop that continues until the data structure is empty. This loop will simulate the recursive calls.
5. Process Each Item
Within the loop, process each item from the data structure, simulating a single recursive call.
6. Handle Base and Recursive Cases
Implement logic to handle both base cases and recursive cases within the loop.
7. Update State and Continue
Update the state as necessary and add new items to the data structure to simulate further recursive calls.
8. Return the Result
Once the loop is complete, return the final result.
Example: Converting a Recursive Factorial Function
Let’s apply this strategy to convert a simple recursive factorial function to an iterative one.
Recursive Implementation
First, let’s look at the recursive implementation of the factorial function:
def factorial_recursive(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial_recursive(n - 1)
Step-by-Step Conversion
Now, let’s follow our strategic approach to convert this to an iterative solution:
1. Identify the Recursive Pattern
- Base case: n == 0 or n == 1
- Recursive case: n * factorial_recursive(n – 1)
- State: The current value of n
2. Choose an Appropriate Data Structure
For this simple linear recursion, we don’t actually need a stack. We can use a simple variable to keep track of the result.
3. Initialize the Iteration
We’ll start with the initial value of n and a result variable set to 1.
4. Implement the Main Loop
We’ll use a while loop that continues as long as n is greater than 1.
5. Process Each Item
In each iteration, we’ll multiply the result by the current value of n.
6. Handle Base and Recursive Cases
The base case is implicitly handled by the loop condition. The recursive case is simulated by the multiplication in each iteration.
7. Update State and Continue
We’ll decrement n in each iteration to move towards the base case.
8. Return the Result
After the loop completes, we’ll return the final result.
Iterative Implementation
Here’s the resulting iterative implementation:
def factorial_iterative(n):
result = 1
while n > 1:
result *= n
n -= 1
return result
This iterative version accomplishes the same task as the recursive one but without the risk of stack overflow for large inputs.
More Complex Example: Tree Traversal
Let’s look at a more complex example: converting a recursive in-order binary tree traversal to an iterative solution.
Recursive Implementation
First, the recursive version:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal_recursive(root):
result = []
if root:
result.extend(inorder_traversal_recursive(root.left))
result.append(root.val)
result.extend(inorder_traversal_recursive(root.right))
return result
Step-by-Step Conversion
Now, let’s apply our strategy to convert this to an iterative solution:
1. Identify the Recursive Pattern
- Base case: root is None
- Recursive cases: Traverse left subtree, process current node, traverse right subtree
- State: The current node being processed
2. Choose an Appropriate Data Structure
We’ll use a stack to keep track of nodes to be processed.
3. Initialize the Iteration
We’ll start with an empty result list and a stack containing the root node (if it exists).
4. Implement the Main Loop
We’ll use a while loop that continues as long as there are nodes in the stack or we’re currently processing a node.
5. Process Each Item
We’ll traverse to the leftmost node, then process nodes in the order: current node, right child, parent.
6. Handle Base and Recursive Cases
The base case is handled by checking for None nodes. The recursive cases are simulated by pushing nodes onto the stack and processing them in the correct order.
7. Update State and Continue
We’ll update the current node and stack as we traverse the tree.
8. Return the Result
After processing all nodes, we’ll return the result list.
Iterative Implementation
Here’s the resulting iterative implementation:
def inorder_traversal_iterative(root):
result = []
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
result.append(current.val)
current = current.right
return result
This iterative version accomplishes the same in-order traversal as the recursive one but without the risk of stack overflow for deep trees.
Common Challenges and Solutions
When converting recursive solutions to iterative ones, you may encounter some common challenges. Here are a few, along with strategies to overcome them:
1. Managing Complex State
Challenge: Some recursive algorithms maintain complex state across recursive calls.
Solution: Use a custom object or tuple to encapsulate all necessary state information, and push/pop these objects from your stack or queue.
2. Handling Multiple Recursive Calls
Challenge: Functions with multiple recursive calls (e.g., quicksort) can be tricky to convert.
Solution: Use a stack to manage multiple paths of execution. Push all potential recursive calls onto the stack and process them one by one.
3. Maintaining Order of Operations
Challenge: Ensuring that operations occur in the same order as in the recursive version.
Solution: Carefully design your loop structure and stack operations to mimic the order of the recursive calls.
4. Handling Tail Recursion
Challenge: Identifying and optimizing tail-recursive functions.
Solution: Tail-recursive functions can often be converted to a simple loop by updating the function parameters in-place.
Performance Considerations
While iterative solutions often provide performance benefits, it’s important to consider the following:
- Time Complexity: In most cases, the time complexity remains the same when converting from recursive to iterative.
- Space Complexity: Iterative solutions typically have better space complexity, especially for deep recursions.
- Readability: Recursive solutions can sometimes be more concise and easier to understand. Consider the trade-off between performance and code clarity.
- Tail Call Optimization: Some languages and compilers optimize tail-recursive functions. In these cases, the performance difference may be negligible.
Best Practices for Conversion
To ensure successful and efficient conversions, keep these best practices in mind:
- Start Simple: Begin with straightforward recursive functions to build your conversion skills.
- Maintain Functionality: Ensure your iterative solution produces identical results to the recursive version.
- Use Helper Functions: Break down complex conversions into smaller, manageable helper functions.
- Test Thoroughly: Implement comprehensive test cases to verify the correctness of your converted function.
- Consider Edge Cases: Pay special attention to edge cases, especially those related to empty or minimal inputs.
- Optimize Gradually: First focus on a correct conversion, then optimize for performance if necessary.
- Document Your Approach: Clearly comment your iterative solution, explaining how it mirrors the recursive logic.
Conclusion
Converting recursive solutions to iterative ones is a valuable skill that can significantly improve the efficiency and robustness of your code. By following the strategic approach outlined in this guide and practicing with various examples, you’ll be well-equipped to tackle this common challenge in technical interviews and real-world programming scenarios.
Remember that the goal is not always to convert every recursive solution to an iterative one. Sometimes, recursion provides the most elegant and maintainable solution. The key is to understand both approaches and make informed decisions based on the specific requirements of your project and the constraints of your environment.
As you continue to develop your skills in this area, you’ll find that the ability to think both recursively and iteratively enhances your overall problem-solving capabilities. This flexibility will serve you well in your journey as a programmer, whether you’re preparing for technical interviews at top tech companies or working on complex software projects.
Keep practicing, stay curious, and don’t hesitate to tackle increasingly complex conversions. With time and experience, you’ll develop an intuition for when and how to apply these techniques effectively, making you a more versatile and valuable programmer.