Computation Theory: Exploring Automata, Turing Machines, and Formal Languages
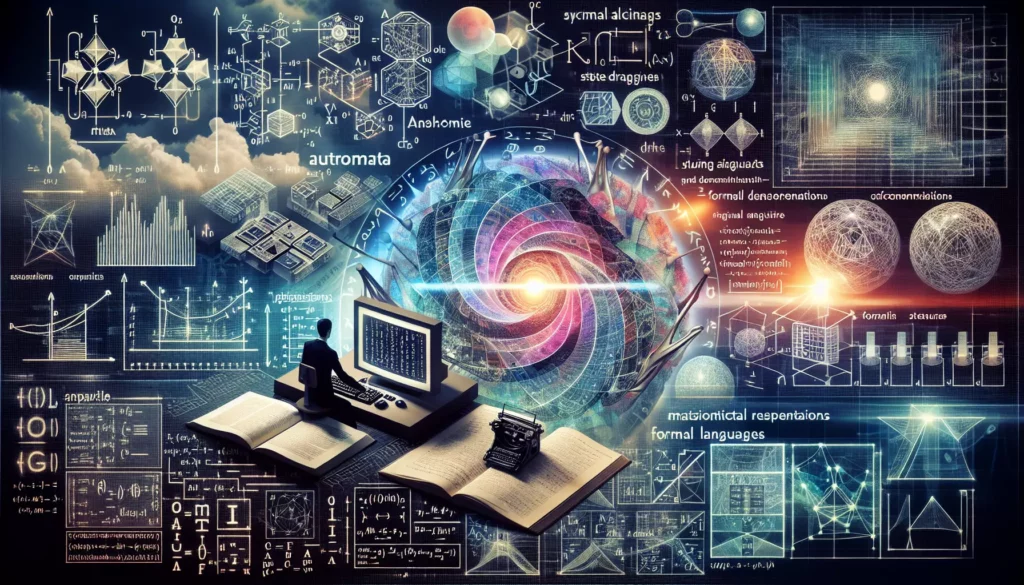
In the ever-evolving world of computer science and programming, understanding the fundamental principles that govern computation is crucial. Computation theory, a branch of theoretical computer science, provides the foundation for understanding how computers process information and solve problems. This article delves into the fascinating realm of computation theory, exploring key concepts such as automata theory, Turing machines, and formal language theory. Whether you’re a beginner programmer or an experienced developer preparing for technical interviews at top tech companies, grasping these concepts will enhance your problem-solving skills and deepen your understanding of algorithmic thinking.
What is Computation Theory?
Computation theory, also known as theory of computation, is a field that focuses on the study of fundamental problems in computer science. It aims to answer questions about what can be computed, how efficiently it can be computed, and what the limitations of computation are. By exploring these concepts, we gain insights into the capabilities and limitations of computers and algorithms.
The study of computation theory is essential for several reasons:
- It provides a theoretical foundation for understanding the nature of computation
- It helps in designing efficient algorithms and data structures
- It enables us to classify problems based on their complexity
- It contributes to the development of new programming languages and computer architectures
Now, let’s dive into the three main areas of computation theory: automata theory, Turing machines, and formal language theory.
Automata Theory
Automata theory is the study of abstract machines and the problems they can solve. These abstract machines, called automata, are mathematical models of computation that define a set of rules for processing input and producing output. Automata theory plays a crucial role in understanding the capabilities and limitations of different types of computational devices.
Types of Automata
There are several types of automata, each with different levels of computational power:
- Finite Automata (FA): The simplest type of automata, capable of recognizing regular languages.
- Pushdown Automata (PDA): More powerful than FA, capable of recognizing context-free languages.
- Turing Machines: The most powerful type of automata, capable of recognizing recursively enumerable languages.
Finite Automata
Finite automata, also known as finite state machines, are the simplest type of automata. They consist of a finite number of states and transitions between these states based on input symbols. Finite automata can be either deterministic (DFA) or non-deterministic (NFA).
Here’s a simple example of a DFA that recognizes strings ending with “ab”:
Q = {q0, q1, q2} // Set of states
Σ = {a, b} // Input alphabet
q0 = q0 // Initial state
F = {q2} // Set of final states
Transition function δ:
δ(q0, a) = q1
δ(q0, b) = q0
δ(q1, a) = q1
δ(q1, b) = q2
δ(q2, a) = q1
δ(q2, b) = q0
This DFA has three states (q0, q1, q2) and transitions defined for input symbols ‘a’ and ‘b’. The state q2 is the final state, reached when the input string ends with “ab”.
Pushdown Automata
Pushdown automata (PDA) are more powerful than finite automata. They include a stack, which allows them to recognize context-free languages. PDAs are useful for parsing programming languages and evaluating arithmetic expressions.
A simple example of a PDA that recognizes the language L = {a^n b^n | n ≥ 0} (strings with equal numbers of ‘a’s followed by ‘b’s):
Q = {q0, q1, q2} // Set of states
Σ = {a, b} // Input alphabet
Γ = {A, Z} // Stack alphabet
q0 = q0 // Initial state
Z = Z // Initial stack symbol
F = {q2} // Set of final states
Transition function δ:
δ(q0, a, Z) = (q0, AZ) // Push 'A' for each 'a'
δ(q0, a, A) = (q0, AA)
δ(q0, b, A) = (q1, ε) // Pop 'A' for each 'b'
δ(q1, b, A) = (q1, ε)
δ(q1, ε, Z) = (q2, Z) // Accept when stack is empty except for 'Z'
This PDA uses the stack to keep track of the number of ‘a’s encountered, and then pops the stack for each ‘b’, ensuring that the numbers match.
Turing Machines
Turing machines, introduced by Alan Turing in 1936, are the most powerful computational model in the theory of computation. They are capable of simulating any computer algorithm and are used to define the concept of computability.
Components of a Turing Machine
A Turing machine consists of:
- An infinite tape divided into cells, each containing a symbol
- A read/write head that can move left or right on the tape
- A finite set of states
- A finite set of symbols (alphabet)
- A transition function that determines the machine’s behavior
Example: Turing Machine for Binary Addition
Let’s consider a simple Turing machine that performs binary addition of two numbers:
Q = {q0, q1, q2, qf} // Set of states
Σ = {0, 1, +, =} // Input alphabet
Γ = {0, 1, +, =, B} // Tape alphabet (B represents blank)
q0 = q0 // Initial state
qf = qf // Final state
Transition function δ:
δ(q0, 0) = (q0, 0, R) // Move right until '+'
δ(q0, 1) = (q0, 1, R)
δ(q0, +) = (q1, +, R) // Start addition
δ(q1, 0) = (q1, 0, R) // Move right until '='
δ(q1, 1) = (q1, 1, R)
δ(q1, =) = (q2, =, L) // Move left to start adding
δ(q2, 0) = (q2, 0, L) // Add 0 + 0 = 0, carry 0
δ(q2, 1) = (q2, 1, L) // Add 1 + 0 = 1 or 0 + 1 = 1, carry 0
δ(q2, +) = (qf, +, R) // Finish addition
// Additional transitions for carrying and handling 1 + 1 case
This Turing machine performs binary addition by moving back and forth on the tape, updating symbols as it goes. The actual implementation would require more states and transitions to handle all cases, including carrying.
The Church-Turing Thesis
The Church-Turing thesis states that any computation that can be carried out by an algorithm can be performed by a Turing machine. This thesis is fundamental to our understanding of computability and has significant implications for computer science and mathematics.
Formal Language Theory
Formal language theory is the study of formal languages and their relation to automata. It provides a framework for understanding the structure and properties of programming languages, as well as natural languages to some extent.
The Chomsky Hierarchy
The Chomsky hierarchy, introduced by Noam Chomsky, classifies formal languages into four types based on their generative power:
- Type 0 (Recursively Enumerable): Recognized by Turing machines
- Type 1 (Context-Sensitive): Recognized by linear bounded automata
- Type 2 (Context-Free): Recognized by pushdown automata
- Type 3 (Regular): Recognized by finite automata
Each type is a proper subset of the types above it, forming a hierarchy of increasing complexity and expressive power.
Regular Languages
Regular languages are the simplest class of formal languages in the Chomsky hierarchy. They can be described by regular expressions and are recognized by finite automata.
Properties of regular languages:
- Closed under union, intersection, and complement
- Closed under concatenation and Kleene star
- Decidable for emptiness, finiteness, and equivalence
Example of a regular language: All binary strings ending with “01”
Regular Expression: (0|1)*01
Context-Free Languages
Context-free languages are more powerful than regular languages and are recognized by pushdown automata. They are crucial in the design of programming languages and parsing algorithms.
Properties of context-free languages:
- Closed under union, concatenation, and Kleene star
- Not closed under intersection or complement
- Decidable for emptiness and finiteness, but not for equivalence
Example of a context-free language: Well-balanced parentheses
Grammar:
S → (S) | SS | ε
Context-Sensitive Languages
Context-sensitive languages are more powerful than context-free languages and are recognized by linear bounded automata. They allow for more complex dependencies between parts of a string.
Properties of context-sensitive languages:
- Closed under union, intersection, concatenation, and Kleene star
- Closed under complement (proven by Immerman and Szelepcsényi)
- Decidable for emptiness, but undecidable for equivalence
Example of a context-sensitive language: a^n b^n c^n (equal numbers of a’s, b’s, and c’s)
Recursively Enumerable Languages
Recursively enumerable languages are the most general class of languages in the Chomsky hierarchy. They are recognized by Turing machines and include all decidable and some undecidable problems.
Properties of recursively enumerable languages:
- Closed under union, intersection, and concatenation
- Not closed under complement
- Undecidable for emptiness, finiteness, and equivalence
Example of a recursively enumerable language: The halting problem (undecidable)
Applications in Programming and Problem Solving
Understanding computation theory has numerous practical applications in programming and problem-solving:
1. Compiler Design
Formal language theory and automata are essential in designing compilers and interpreters. Lexical analysis uses finite automata to tokenize source code, while parsing often employs context-free grammars and pushdown automata.
2. Regular Expressions
Regular expressions, based on the theory of regular languages, are widely used in text processing, pattern matching, and input validation.
3. Complexity Analysis
Computation theory provides the foundation for analyzing the time and space complexity of algorithms, helping developers create more efficient solutions.
4. Formal Verification
Techniques from computation theory are used in formal verification of software and hardware systems, ensuring correctness and reliability.
5. Natural Language Processing
Formal language theory contributes to the development of natural language processing algorithms and models.
Preparing for Technical Interviews
For those preparing for technical interviews at top tech companies, a solid understanding of computation theory can be a significant advantage:
1. Problem Classification
Recognizing the type of problem (e.g., regular, context-free, or more complex) can help in choosing the appropriate approach and data structures.
2. Algorithm Design
Knowledge of automata and formal languages can inspire creative solutions to complex problems, especially those involving pattern matching or state transitions.
3. Complexity Analysis
Understanding the theoretical limits of computation helps in analyzing and optimizing the time and space complexity of solutions.
4. Language Design Questions
Some interviews may include questions about designing domain-specific languages or parsers, where knowledge of formal language theory is directly applicable.
5. Demonstrating Depth of Knowledge
Discussing concepts from computation theory can showcase a candidate’s strong theoretical foundation and passion for computer science.
Conclusion
Computation theory, encompassing automata theory, Turing machines, and formal language theory, forms the backbone of computer science. It provides a deep understanding of the capabilities and limitations of computation, which is invaluable for both theoretical research and practical software development.
For learners and professionals in the field of computer science and programming, studying computation theory offers numerous benefits:
- Enhanced problem-solving skills
- Improved algorithm design and analysis capabilities
- Better understanding of programming languages and compilers
- Stronger foundation for tackling complex computational problems
- Advantage in technical interviews and advanced computer science topics
As you progress in your coding journey, from beginner tutorials to preparing for technical interviews at major tech companies, keep in mind the fundamental principles of computation theory. They will not only deepen your understanding of how computers work but also elevate your skills as a programmer and problem solver.
Remember, platforms like AlgoCademy offer resources and interactive coding tutorials that can help you apply these theoretical concepts to practical programming challenges. By combining theoretical knowledge with hands-on coding practice, you’ll be well-equipped to tackle complex problems and excel in your programming career.